使用 RepositoryProvider 简化父子组件的传值
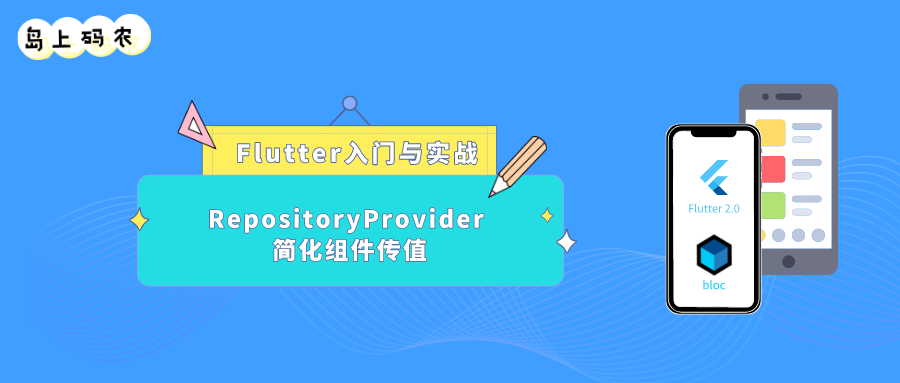
前言
在实际开发过程中,经常会遇到父子组件传值的情况,通常来说会有三种方式:
构造函数传值:父组件将子组件需要的对象通过构造函数传递给子组件;
单例对象:构建单例对象,使得父子组件使用的是同一个对象;
容器:将对象存入容器中,父子组件使用的时候直接从容器中获取。
第一种方式的缺陷是如果组件嵌套很深,传递数据对象需要层层传递,将导致代码很难维护。第二种方式需要自己构建单例类,而实际上要传递的对象可能存在很多个实例。第三种和单例类似,如果往容器存储不定数量的实例对象是不合适的。flutter_bloc 提供了一种基于组件的依赖注入方式解决这类问题,通过使用 RepositoryProvider
,可以为组件树的子组件提供共享对象,这个共享对象只限在组件树中使用,可以通过 Provider
的方式访问该对象。
RepositoryProvider 定义
Repository
实际上是 Provider
的一个子类,通过注册单例的方式实现组件树对象共享,因此其注册的对象会随着 Provider
的注销而销毁,而且这个对象无需是 Bloc
子类。因此在无法使用 Bloc
传输共享对象的时候,可以使用 RepositoryProvider
来完成。RepositoryProvider 有两种方式创建对象共享,create
和 value
方式,其中 create
是通过调用一个方法创建新的对象,而 value
是共享一个已有的对象。RepositoryProvider
的定义如下:
RepositoryProviderSingleChildWidget 本身是一个空的 Mixin:
,注释上写着其用途是为了方便 MultiRepositoryProvider
推断RepositoryProvider
的类型设计。可以看到实际上 RepositoryProvider
就是 Provider
,只是将静态方法 of
的listen
参数默认设置为 false
了,也就是不监听状态对象的变化。我们在子组件中通过两种方式访问共享对象:
如果有多个对象需要共享,可以使用MultiRepositoryProvider
,使用方式也和 MultiProvider 相同 :
RepositoryProvider 应用
回顾一下我们之前使用使用 BLoC 构建 Flutter 的页面实例的代码,在里面我们页面分成了三个部分:
头像及背景图:
_getBannerWithAvatar
;个人资料:
_getPersonalProfile
;个人数据统计:
_getPersonalStatistic
。
分别使用了三个构建组件的函数完成。对应的界面如下所示:
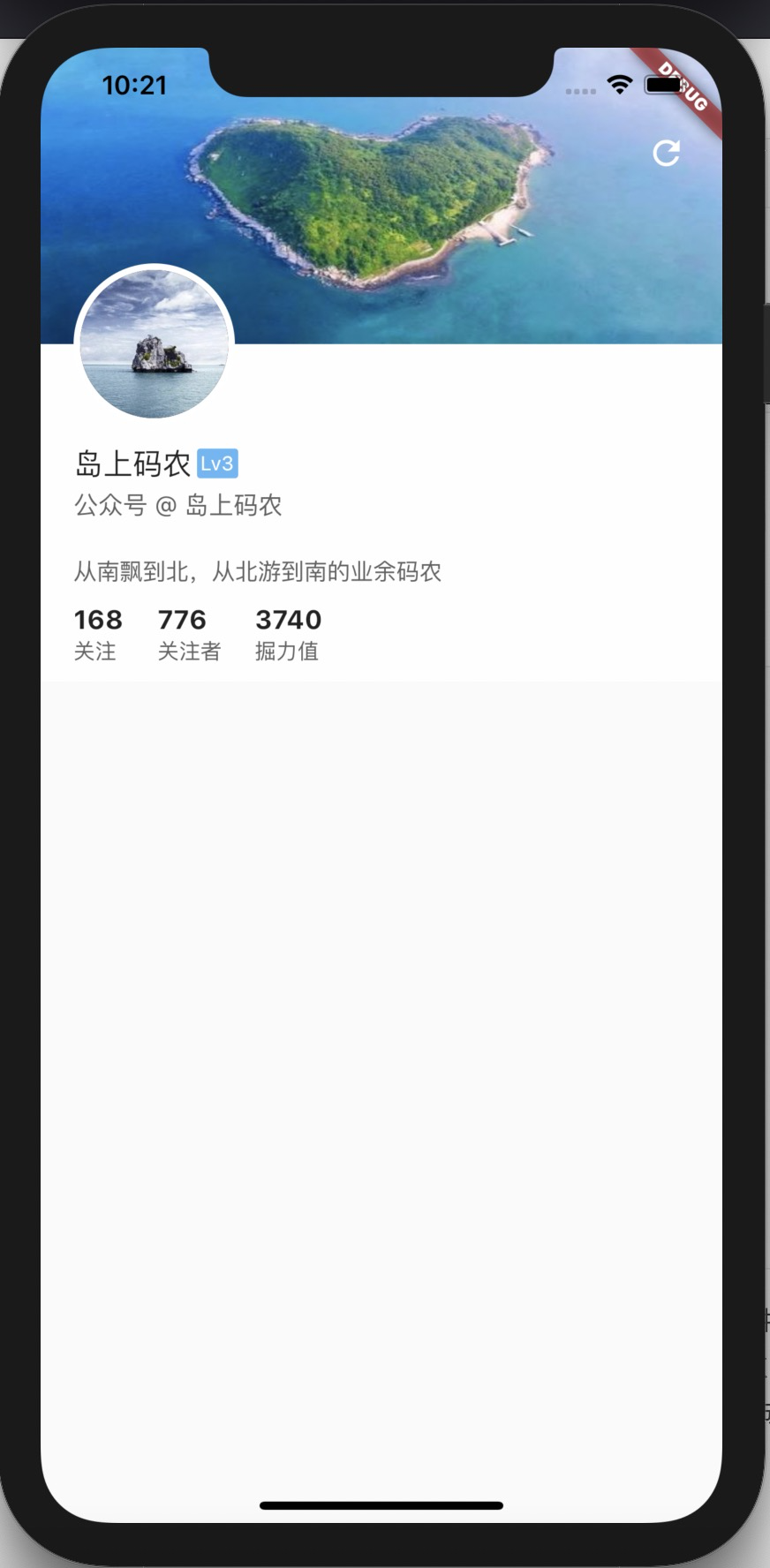
可以看到,每个函数都需要把 personalProfile
这个对象通过函数的参数传递,而如果函数中的组件还有下级组件需要这个对象,还需要继续往下传递。这要是需要修改对象传值的方式,需要沿着组件树逐级修改,维护起来会很不方便。我们改造一下,将三个函数构建组件分别换成自定义的 Widget,并且将个人统计区换成两级组件,改造后的组件树如下所示(省略了装饰类的层级)。
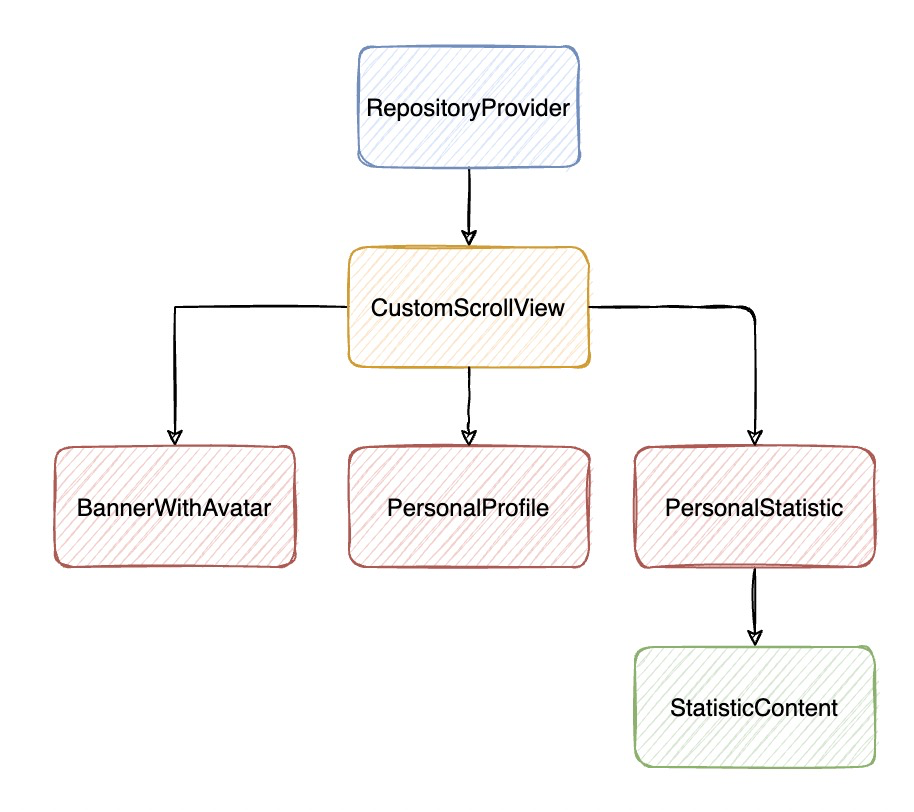
拆解完之后,我们就可以简化personalProfile
的传值了。
这里使用value
模式是因为 personalProfile
已经被创建了。然后在需要使用 personalProfile
的地方,使用context.read<PersonalEntity>()
就可以从 RepositoryProvider
中取出personalProfile
对象了,从而使得各个子组件无需再传递该对象。以 BannerWithAvatar 为例,如下所示:
可以看到整个代码更简洁也更易于维护了。具体源码已上传至:BLoC 状态管理代码,大家可以下载下来参考。
总结
本篇介绍了 RepositoryProvider
的使用,实际上 RepositoryProvider
借用Provider
实现了一个组件树上的局部共享对象容器。通过这个容器,为RepositoryProvider
的子组件树注入了共享对象,使得子组件可以从 context
中或使用RepositoryProvider.of
静态方法获取共享对象。通过这种方式避免了组件树的层层传值,使得代码更为简洁和易于维护。
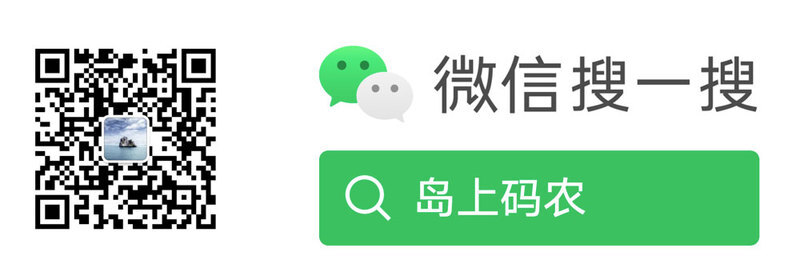
版权声明: 本文为 InfoQ 作者【岛上码农】的原创文章。
原文链接:【http://xie.infoq.cn/article/f8ca9a3beadcb5bfcff64c104】。文章转载请联系作者。
评论