【Vuex 源码学习】第十二篇 - Vuex 插件机制的实现
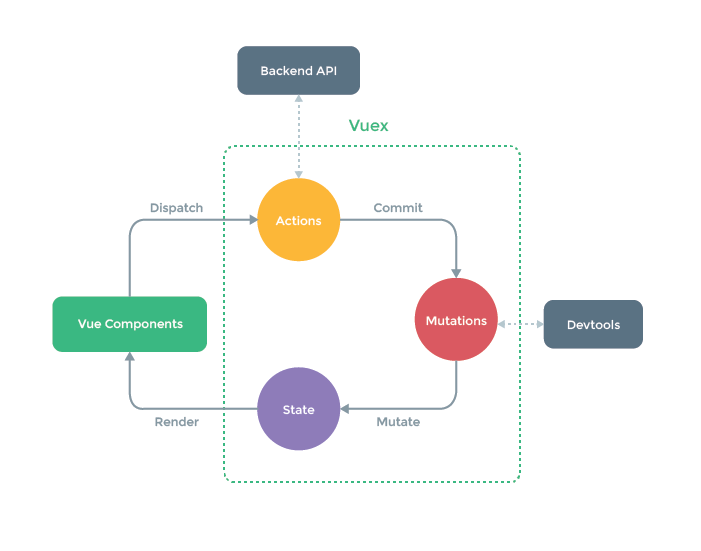
一,前言
上一篇,主要介绍了 Vuex 插件的开发,主要涉及以下几个点:
Vuex 插件的使用介绍;
Vuex 插件开发和使用分析;
Vuex 插件机制的分析;
本篇,继续介绍 Vuex 插件机制的实现;
二,Vuex 插件机制分析
通过官方 Vuex 插件所提供的插件机制,创建并实现了一个简易的 Vuex 插件;
Vuex 插件的实现,主要使用 Vuex 提供的以下方法:
Vuex 插件的 plugins 数组,提供插件注册功能;
store.subscribe:状态变更时的订阅回调功能;
store.replaceState:状态替换功能;
本篇继续以 vuex 持久化插件 vuex-persists 为例,逐步实现 Vuex 插件提供的机制核心逻辑;
三,Vuex 插件机制实现
1,Vuex 插件的注册
当
new Vuex.Store
初始化时,处理 options.plugins 数组中注册的插件函数(高阶函数);收集 Vuex 插件中,通过 store.subscribe 订阅状态变化事件的回调函数 fn 保存到
_subscribes
数组中;
2,subscribe 状态订阅实现
当状态变化时,即 mutation 方法执行时,依次执行
_subscribes
中保存的处理函数 fn,传入当前 mutation 和当前根状态 rootState;
测试 Vuex 插件的初始化和状态变化时间订阅:
在 Vuex 插件初始化过程中 plugins 插件被依次执行,并收集插件中通过 store.subscribe 进行状态变更订阅的回调函数 fn;
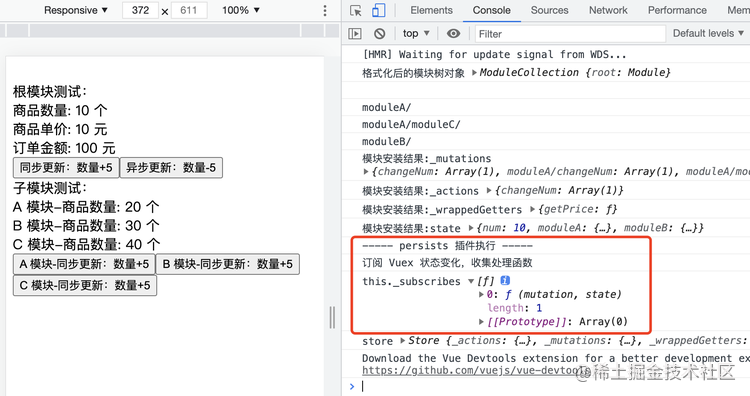
点击按钮更新 Vuex 中的状态,在对应 mutation 方法执行时,依次调用订阅函数,通知 Vuex 插件状态发生变化:
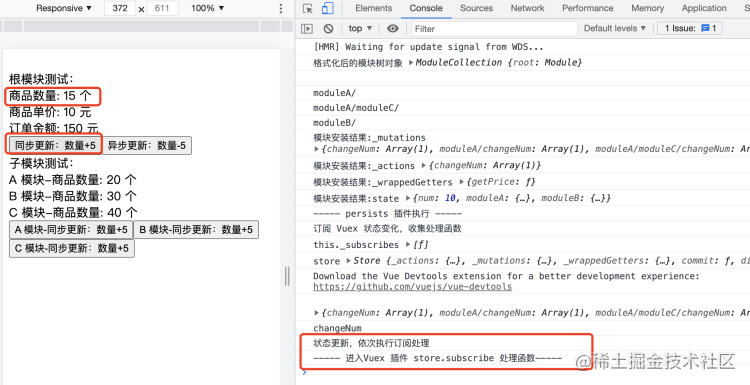
订阅回调中处理:将当前最新的根状态保存至本地存储:
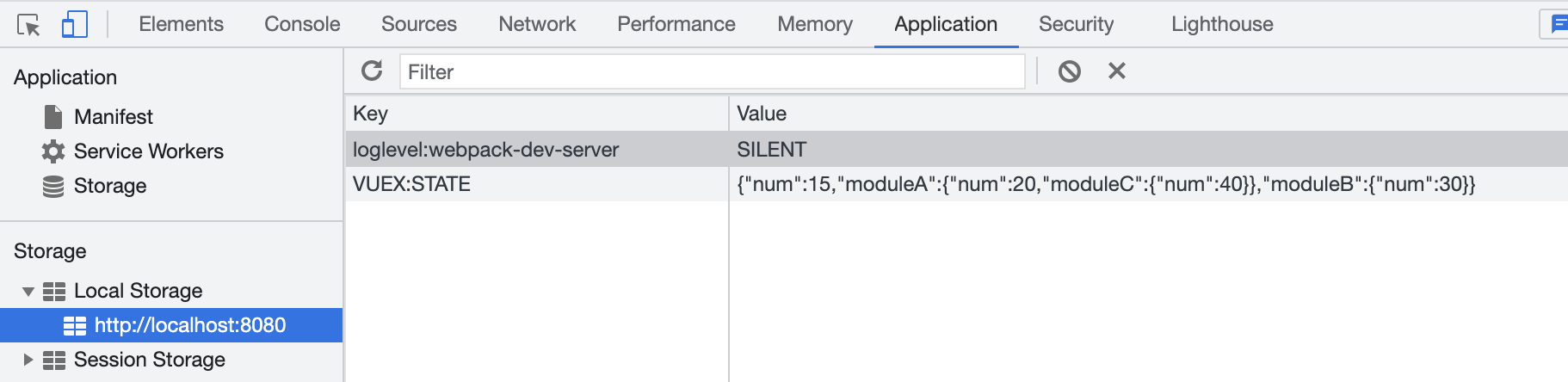
3,replaceState 状态替换实现
当状态更新时,将最新状态保存至本地存储中;
当页面刷新时,读取本地存储并重新设置 Vuex 状态,刷新状态持久化;
插件初始化时,读取本都存储,进行 Vuex 状态同步:
添加 replaceState 实现,并更新插件逻辑,统一使用最新状态进行处理:
备注:虽然通过 replaceState 实现了 Vuex 插件状态的更新,但此时 Vuex 中的逻辑处理中,依旧使用的是module.state
或 rootState
旧状态,需要根据模块路径重新获取对应的新状态进行处理;否则页面不会更新;(涉及 mutation、getter、_subscribes 回调传参等相关处理逻辑需更新);
4,插件效果测试
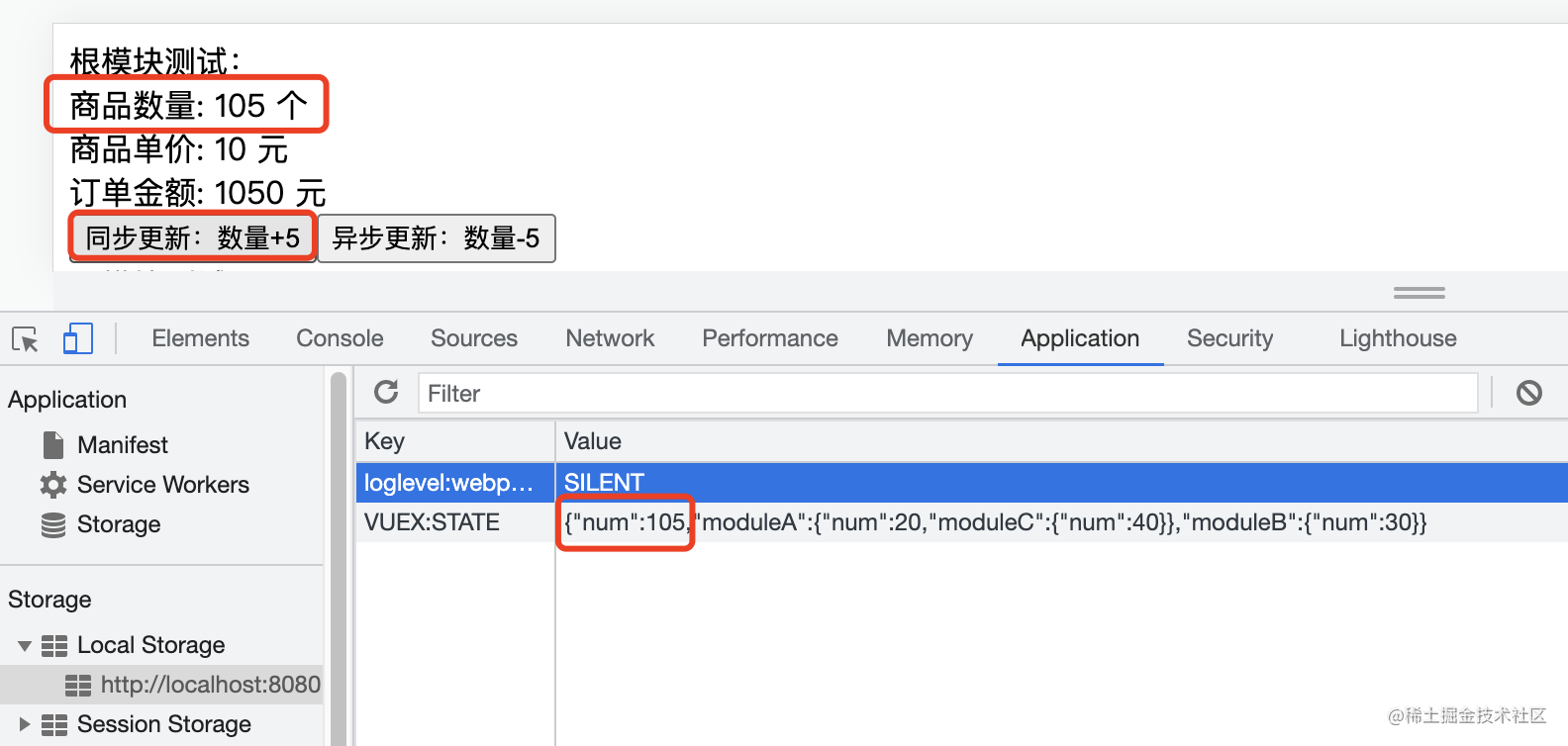
点击按钮更新状态,视图与本地存储同步变化;
页面刷新后,状态持久化成功;
四,结尾
本篇,主要介绍了 Vuex 插件机制的实现,主要涉及以下几个点:
Vuex 插件机制分析;
Vuex 插件机制核心逻辑实现:plugins 插件注册、subscribe 订阅收集、replaceState 状态替换;
下一篇,继续介绍 Vuex 辅助函数的实现;
评论