iOS 中的 Category
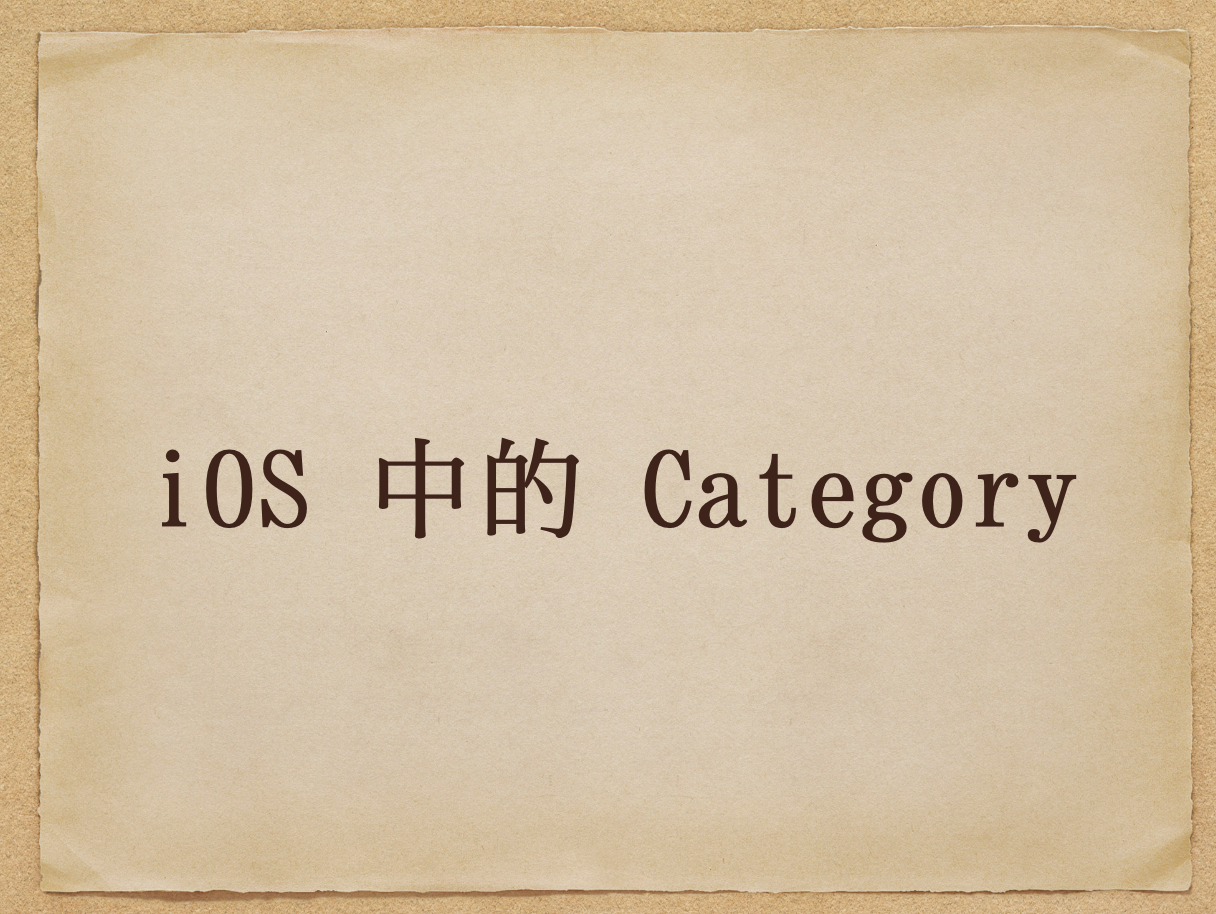
什么是 Category
在 OC 中,扩展一个类的方式有两种
继承
分类
可以在不修改原来类的基础上,为这个类扩充一些方法
一个庞大的类可以分模块开发
Category 的格式
通过 Category 给某类添加方法,分为声明和实现俩部分
创建 Category 时,必须给 Category 的名称加上专用前缀
创建 Category 的方法时,必须给方法名称加上专用前缀
分类声明
分类实现
Category 的类型
Class-continuation 类型的 Category
它必须定义在其所接续的那个类的实现文件中
此分类可以声明属性,且此分类没有特定的是现实文件,其中方法都定义在主实现文件中
一般存放不需要对外公开的属性(例子中的 age)
如果某属性在主接口中声明为“只读”,而类内部要用 setter 方法修改此属性,那么就在 Class-continuation 分类中将其扩展为 “readwrite”(例子中的 friends)
若想使类遵循的协议不为人知道,则可以在 Class-continuation 分类中声明
普通类型的 Category
Category 的运用
在开发中,类的实现文件特别大,难于管理与维护,因此经常使用分类机制把类的实现代码划分成易于管理的小块,以便单独检视
实现文件里,所有的方法都写在一个类,内容太多,所以我们可根据其不同功能分成多个分类
Category 的注意事项
Category 只能添加方法,不能添加属性。因为 Category 中的 @property,只会生成 setter/getter 的方法声明,不会生成实现及私有的成员变量 (在.m 文件(Class-continuation)中的分类可以声明属性,同时也可以生成 setter、getter 方法)
Xcode 会有警告

运行程序会崩溃

如果分类中有和原类中同名的方法,程序只会调用分类里的方法,如果多个分类中都有和原类中同名的方法,程序只会由编译器决定,编译器最后一个执行的方法来响应调用优先级(Category->本类->父类)
log:
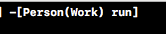
版权声明: 本文为 InfoQ 作者【NewBoy】的原创文章。
原文链接:【http://xie.infoq.cn/article/6dfcfd52d8bad7f08dde279ad】。文章转载请联系作者。
评论