实现服务器和客户端数据交互,Java Socket 有妙招
摘要:在 Java SDK 中,对于 Socket 原生提供了支持,它分为 ServerSocket 和 Socket。
本文分享自华为云社区《Java Socket 如何实现服务器和客户端数据交互》,作者: jackwangcumt 。
1 Socket 概述
根据百度百科的定义,Socket 译为套接字,它是对网络中不同主机上的应用进程之间进行双向通信的端点的抽象。一个 Socket 实例就是网络上进程通信的一端,提供了应用层进程利用网络协议交换数据的机制。Socket 向上连接各种应用进程,向下连接各种网络协议,是应用程序通过网络协议进行通信的接口。其示意图如下下图所示:
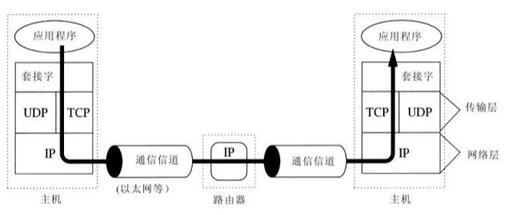
(来自《Java TCP/IP Socket 编程》)
从上图可知,套接字 Socket 在 OSI 七层模型中处于应用层和传输层之间,是一个重要的接口。一般来说,传输层协议有 TCP 协议和 UDP 协议。这两个协议底层都基于 IP 网络层协议。
2 Java Socket 实现
在 Java SDK 中,对于 Socket 原生提供了支持,它分为 ServerSocket 和 Socket,其中 ServerSocket 发起一个服务端的 Socket,其中需要提供一个端口号,如果给定 0,则自动申请可用的端口。当然了,也可以指定具体的端口号(有一定的范围,不超过 65535 )。不过这里需要注意,传入的端口不能被其他应用占用,否则启动服务失败。下面给出一个简单的 ServerSocket 示例,代码如下:
启动该 Server 端,并开始监听客户端的连接。当客户端没有连接时,服务器线程池 pool 并未启动单独的线程。下面给出客户端的 Java Socket 实现,具体的示例代码如下:
从代码可知,try (Socket socket = new Socket(IP, PORT)) 是一种包含资源释放的 try-with-resource 机制,它会自动进行资源释放,而不需要手动进行释放。Socket 对象要想和服务器通信,必须要明确服务器的 IP 地址和端口,否则不能正确通信,Socket 启动时,也会在主机上占用自己的端口。我们首先启动 Server 端,然后可以同时启动 10 个以上的 Client 端,比如 13 个,那么超过 Executors.newFixedThreadPool(10)限定的数量 10 后,将进入 queued tasks 队列中进行排队等待。通过打印 pool 对象,可以看出当前的状态,比如[Running, pool size = 4, active threads = 4, queued tasks = 0, completed tasks = 0]说明当前在运行状态,线程池大小为 10,激活的线程为 10,等待的任务线程 queued tasks 为 0。
下面给出 Server 端相关输出示例:
客户端相关输入输出界面如下:
版权声明: 本文为 InfoQ 作者【华为云开发者社区】的原创文章。
原文链接:【http://xie.infoq.cn/article/677d2ef4c5a09f411d6cdbe93】。文章转载请联系作者。
评论