Python 协程
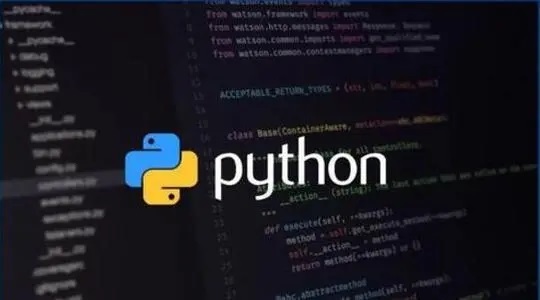
协程
参考资料
http://python.jobbole.com/86481/
http://python.jobbole.com/87310/
http://segmentfault.com/a/1190000009781688
迭代器
可迭代(Iterable):直接作用于 for 循环的变量
迭代器(Iterator):不但可以作用于 for 循环,还可以被 next 调用
list 是典型的可迭代对象,但不是迭代器
通过 isinstance 判断
iterable 和 iterator 可以转换
通过 iter 函数
生成器
generator:一边循环一边计算下一个元素的机制/算法
需要满足三个条件
每次调用都生产出 for 循环需要的下一个元素或者
如果达到最后一个后,爆出 StopIteration 异常
可以被 next 函数调用
如何生成一个生成器
直接使用
如果函数中包含 yield,则这个函数就叫生成器
next 调用函数,遇到 yield 返回
协程
历史历程
3.4 引入协程,用 yield 实现
3.5 引入协程语法
实现的协程比较好的包有 asyncio,tornado,gevent
定义:协程 是为非抢占式多任务产生子程序的计算机程序组件,协程允许不同入口点在不用位置暂停或者执行程序。
从技术角度讲,协程就是一个你可以暂停执行的函数,或者干脆把协程理解成生成器
协程的实现:
yield 返回
send 调用
协程的四个状态
inspect.getgeneratorstate(...) 函数确定,该函数会返回下述字符串中的一个:
GEN_CREATED:等待开始执行
GEN_RUNNING:解释器正在执行
GEN_SUSPENED:在 yield 表达式处暂停
GEN_CLOSED:执行结束
next 预激(prime)
代码案例 v2
协程终止
协程中未处理的异常会向上冒泡,传给 next 函数或 send 方法的调用方(即触发协程的对象)
终止协程的一种方式:发送某个哨符值,让协程退出。内置的 None 和 Ellipsis 等常量经常用作哨符值==。
yield from
调用协程为了得到返回值,协程必须正常终止
生成器正常终止会发出 StopIteration 异常,异常对象的 vlaue 属性保存返回值
yield from 从内部捕获 StopIterator 异常
案例 v03
委派生成器
包含 yield from 表达式的生成器函数
委派生成器在 yield from 表达式处暂停,调用方可以直接把数据发给子生成器
子生成器再把产出的值发给调用方
子生成器在最后,解释器会抛出 StopIteration,并且把返回值附加到异常对象上
案例 v04
asyncio
python3.4 开始引入标准库当中,内置对异步 io 的支持
asyncio 本身是一个消息循环
步骤:
创建消息循环
把协程导入
关闭
asyn and await
为了更好的表示异步 io
python3.5 引入
让协程代码更简洁
使用上,可以简单的进行替换
用 async 替换 @asyncio.coroutine
用 await 替换 yield from
aiohttp
asyncio 实现单线程的并发 io,在客户端用处不大
在服务器端可以 asyncio+coroutine 配合,因为 http 是 io 操作
asyncio 实现了 tcp,udp,ssl 等协议
aiohttp 是给予 asyncio 实现的 http 框架
pip install aiohttp 安装
concurrent.futures
python3 新增的库
类似其他语言的线程池的概念
利用 multiprocessiong 实现真正的并行计算
核心原理:以子进程的形式,并行运行多个 python 解释器,从而令 python 程序可以利用多核 CPU 来提升运行速度。由于子进程与主解释器相分离,所以他们的全局解释器锁也是相互独立的。每个子进程都能够完整的使用一个 CPU 内核。
concurrent.futures.Executor
ThreadPoolExecutor
ProcessPoolExecutor
执行的时候需要自行选择
sunmit(fn, args, kwargs)
fn: 异步执行的函数
args, kwargs 参数
current 中 map 函数
map(fn, *iterables, timeout=None)
跟 map 函数类似
函数需要异步执行
timeout:超时时间
map 跟 submit 使用一个就行
版权声明: 本文为 InfoQ 作者【若尘】的原创文章。
原文链接:【http://xie.infoq.cn/article/b8bf4e7e25ec51268235e422c】。文章转载请联系作者。
评论