假如只剩下 canvas 标签
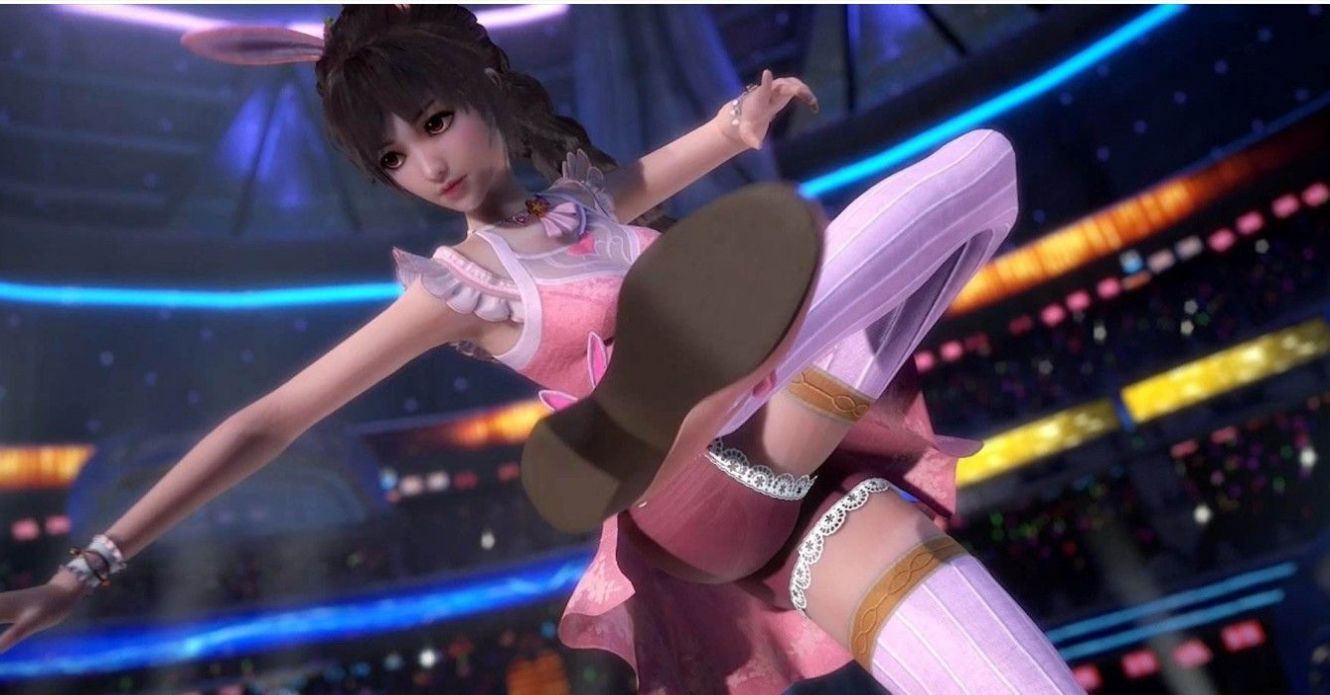
笔者正在进行“前端百题斩”的系列文章撰写,让每位前端工程师掌握高频知识点,为工作助力。关注公众号“执鸢者”,用知识武装自己的头脑。
一、背景
如果只剩下 canvas 标签,该如何去绘制页面中的内容呢?这也许是一个伪命题,但是用 canvas 确事能够帮助完成很多事。今天就用 canvas+AST 语法树构建一个信息流样式。
![]()
二、绘制流程
将整个绘制流程分为三部分:基本元素、AST 语法树、主函数类。基本元素指的是图片、文字、矩形、圆等;AST 语法树在本处值得就是包含一些属性的 js 对象;主函数类指对外暴露的接口,通过调用实现最终绘制。
2.1 基本元素
不管多么复杂的事物肯定都是由一系列简单的元素组成,例如汽车肯定是通过一些简单的机械零配件组成;电脑也是通过电阻、电容等零配件组成。网页也不例外,也是通过文字、图片、矩形等组成。
2.1.1 加载图片
图片是一个页面中的灵魂元素,在页面中占据绝大部分空间。
2.1.2 绘制文字
文字能够提高页面的可读性,让观察该页面的每一个人都能够快速了解该页面的思想。
2.1.3 绘制矩形
通过矩形元素能够与文字等元素配合达到意想不到的效果。
2.1.4 绘制圆
圆与矩形承担的角色一致,也是在页面中比较重要的角色。
2.2 AST 树
AST 抽象语法树是源代码语法结构的一种抽象表示。它以树状的形式表现编程语言的语法结构,树上的每个节点都表示源代码中的一种结构。例如,在 Vue 中,将模板语法转换为 AST 抽象语法树,然后再将抽象语法树转换为 HTML 结构,咱们在利用 canvas 绘制页面时也利用 AST 抽象语法树来表示页面中的内容,实现的类型有 rect(矩形)、img(图片)、text(文字)、circle(圆)。
本次将绘制的内容包含静态页面部分和动画部分,所以将利用两个 canvas 实现,每个 canvas 将对应一个 AST 树,分别为静态部分 AST 树和动态部分 AST 树。
2.2.1 静态部分 AST 树
本次绘制的页面中静态部分的 AST 树如下所示,包含矩形、图片、文字。
2.2.2 动态部分 AST 树
本次绘制的页面中动画部分的 AST 树动态生成,由一系列动态颜色的圆组成。
2.3 主函数类
除了上述一些基本元素类,将通过一个主函数类对外进行暴露。
2.4 内容绘制
前面的准备工作已经完成,下面将各个函数和 AST 树联动起来,达到想要的效果。
2.4.1 静态内容绘制
先将静态部分的内容绘制好,作为页面的基石。
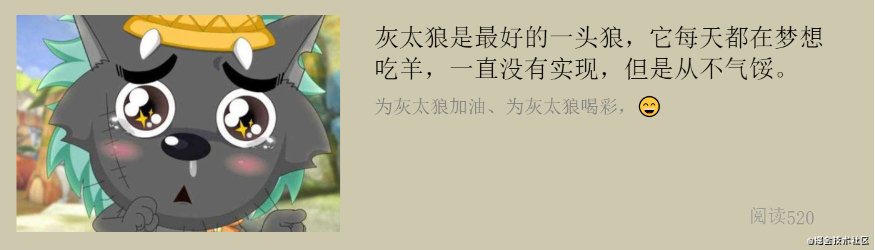
2.4.2 绘制动画跑马灯
再给该部分内容来点动画效果,更加激动人心。
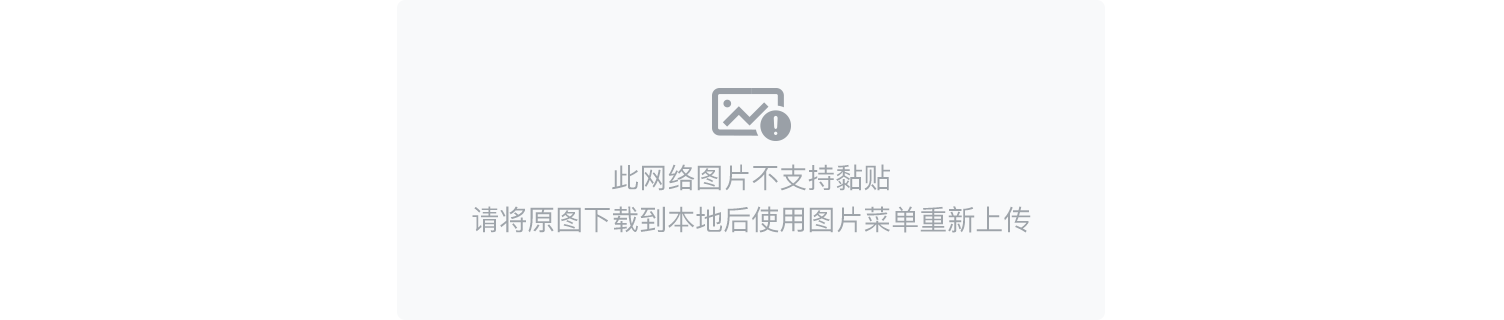
本文对应源码,关注公众号“执鸢者”,回复“canvas”获取
如果觉得这篇文章还不错,来个分享、点赞吧,让更多的人也看到
版权声明: 本文为 InfoQ 作者【执鸢者】的原创文章。
原文链接:【http://xie.infoq.cn/article/b09c03618ef70d5035d9fe2e5】。文章转载请联系作者。
评论