SpringBoot 整合 Drools
发布于: 15 小时前
概述
目前 SpringBoot 框架最为流行,因此,掌握 SpringBoot 整合 Drools 非常重要。
得益于 Spring Boot 框架优秀的可插拔式扩展的开发模式,引入 Drools 也非常简单。
项目配置
Drools 整合 SpringBoot 需要引入 kie-spring 依赖
<dependency>
<groupId>org.kie</groupId>
<artifactId>kie-spring</artifactId>
<version>7.57.0.Final</version>
</dependency>
复制代码
配置 Drools 的 KieContainer、KieBase、KieSession
@Slf4j
@Configuration
public class DroolsConfiguration {
private static final String RULES_PATH = "rules/";
@Bean
@ConditionalOnMissingBean(KieFileSystem.class)
public KieFileSystem kieFileSystem() {
KieFileSystem kieFileSystem = KieServices.Factory.get().newKieFileSystem();
for (Resource ruleFile : getRuleFiles()) {
kieFileSystem.write(ResourceFactory.newClassPathResource(RULES_PATH + ruleFile.getFilename(), "UTF-8"));
}
return kieFileSystem;
}
@Bean
@ConditionalOnMissingBean(KieContainer.class)
public KieContainer kieContainer() {
KieRepository kieRepository = KieServices.Factory.get().getRepository();
kieRepository.addKieModule(kieRepository::getDefaultReleaseId);
KieBuilder kieBuilder = KieServices.Factory.get().newKieBuilder(kieFileSystem());
kieBuilder.buildAll();
return KieServices.Factory.get().newKieContainer(kieRepository.getDefaultReleaseId());
}
@Bean
@ConditionalOnMissingBean(KieBase.class)
public KieBase kieBase() {
return kieContainer().getKieBase();
}
@Bean
@ConditionalOnMissingBean(KieSession.class)
public KieSession kieSession() {
return kieContainer().newKieSession();
}
@Bean
@ConditionalOnMissingBean(KModuleBeanFactoryPostProcessor.class)
public KModuleBeanFactoryPostProcessor kModuleBeanFactoryPostProcessor(){
return new KModuleBeanFactoryPostProcessor();
}
/**
* 获取规则文件
*
* @return
*/
private Resource[] getRuleFiles() {
Resource[] resources = new Resource[0];
ResourcePatternResolver resourcePatternResolver = new PathMatchingResourcePatternResolver();
try {
resources = resourcePatternResolver.getResources("classpath*:" + RULES_PATH + "**/*.*");
} catch (IOException e) {
log.error("加载规则文件失败", e);
}
return resources;
}
}
复制代码
测试控制器 TestController
@RestController
@RequestMapping("/test")
public class TestController {
@Autowired
private TestService testService;
@GetMapping
public void test(){
testService.test();
}
}
复制代码
测试服务 TestService
@Slf4j
@Service
public class TestService {
@Autowired
private KieSession kieSession;
public void test() {
Person person = new Person();
person.setName("LeifChen");
person.setAge(18);
kieSession.insert(person);
int count = kieSession.fireAllRules();
log.info("总执行了{}条规则", count);
}
}
复制代码
规则文件存放于 src/main/resources 目录下的 rules 路径
package rules.hello
import com.chen.drools.bean.Person
rule "test001"
when
eval(true)
then
System.out.println("hello drools");
end
rule "test002"
when
$p:Person(age==18)
then
System.out.println("匹配年龄为18的人:" + $p);
$p.setAge(20);
update($p);
end
rule "test003"
when
$p:Person(age==20)
then
System.out.println("匹配年龄为20的人:" + $p);
end
复制代码
启动 SpringBoot 服务,在浏览器打开地址
localhost:8080/test
,查看控制台输出结果,说明 SpringBoot 整合 Drools 成功
参考
《Drools 规则引擎技术指南》——来志辉
划线
评论
复制
发布于: 15 小时前阅读数: 8
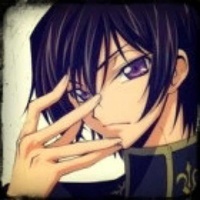
LeifChen
关注
持续学习与分享。 2017.10.29 加入
Java 开发
评论