Android 自定义 view 之模仿登录界面文本输入框(华为云 APP)
==================================================================
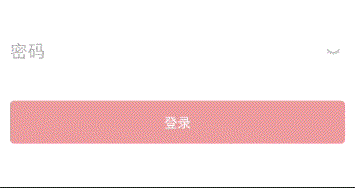
===================================================================
1.组合多个控件完成此输入框静态效果
2.hint 值上浮下潜动画
3.一些功能
===================================================================
public class MyEditVIew extends RelativeLayout {
public MyEditVIew(Context context) {
super(context,null);
}
public MyEditVIew(Context context, AttributeSet attrs) {
super(context, attrs,0);
}
public MyEditVIew(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
}
}
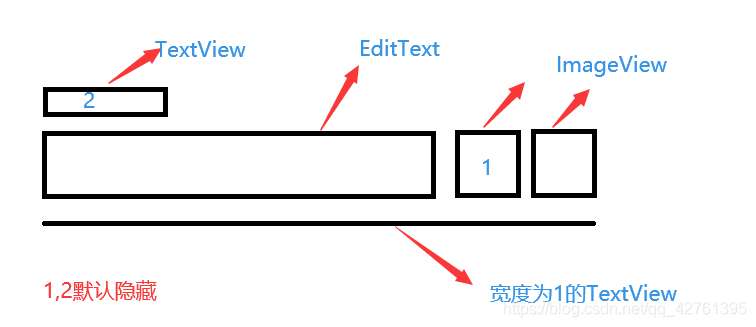
//代码在最后源码部分
LayoutInflater.from(context).inflate(R.layout.my_edit_view, this);
//小提示文字出现动画
private void minTextshow(TextView textView) {
AnimationSet animationSet = new AnimationSet(true);
Animation mHiddenAction = new TranslateAnimation(Animation.RELATIVE_TO_SELF,
0.0f, Animation.RELATIVE_TO_SELF, 0.0f,
Animation.RELATIVE_TO_SELF, 1.0f, Animation.RELATIVE_TO_SELF,
0.0f);
Animation alphaAnimation = new AlphaAnimation(0, 1f);
animationSet.addAnimation(mHiddenAction);
animationSet.addAnimation(alphaAnimation);
animationSet.setDuration(300);
textview.startAnimation(animationSet);
}
//小提示文字隐藏动画
private void minTexthide(TextView textView) {
AnimationSet animationSet = new AnimationSet(true);
Animation mShowAction = new TranslateAnimation(Animation.RELATIVE_TO_SELF, 0.0f,
Animation.RELATIVE_TO_SELF, 0.0f, Animation.RELATIVE_TO_SELF,
0.0f, Animation.RELATIVE_TO_SELF, 1.0f);
mShowAction.setRepeatMode(Animation.REVERSE);
Animation alphaAnimation = new AlphaAnimation(1f, 0);
animationSet.addAnimation(mShowAction);
animationSet.addAnimation(alphaAnimation);
animationSet.setRepeatMode(Animation.REVERSE);
animationSet.setDuration(300);
textview.startAnimation(animationSet);
CountDownTimer countDownTimer = new CountDownTimer(300, 300) {
@Override
public void onTick(long millisUntilFinished) {
}
@Override
public void onFinish() {
textview.setText("");
}
}.start();
}
主要代码
//设置文字非加密
HideReturnsTransformationMethod method = HideReturnsTransformationMethod.getInstance();
edittext.setTransformationMethod(method);
//设置文字加密
TransformationMethod method = PasswordTransformationMethod.getInstance();
edittext.setTransformationMethod(method);
1.将光标移到最后
//将光标移到最后
edittext.setSelection(edittext.getText().toString().length());
2.将键盘中的回车和空格去除
public static void setEditTextInputSpace(EditText editText) {
InputFilter filter = new InputFilter() {
@Override
public CharSequence filter(CharSequence source, int start, int end, Spanned dest, int dstart, int dend) {
if (source.equals(" ") || source.toString().contentEquals("\n")) {
return "";
} else {
return null;
}
}
};
editText.setFilters(new InputFilter[]{filter});
}
3.给自定义 view 对外提供一个获取值的方法
public String getText() {
return edittext.getText().toString();
}
1.MyEditVIew.java
public class MyEditVIew extends RelativeLayout {
private TextView textview;
private EditText edittext;
private boolean mtextisshow; //文字是否显示判断
private boolean imgisshow; //图片是否显示判断
private String hintText;
private ImageView imageView;
private ImageView iV_clean;
public MyEditVIew(Context context) {
super(context,null);
}
public MyEditVIew(Context context, AttributeSet attrs) {
super(context, attrs,0);
init(context, attrs);
setEditTextInputSpace(edittext);
textAddChanged();
imageOnClick();
}
public MyEditVIew(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, at
trs, defStyleAttr);
}
//打气布局,获取自定义属性的值
private void init(Context context, AttributeSet attrs) {
LayoutInflater.from(context).inflate(R.layout.my_edit_view, this);
textview = findViewById(R.id.textview);
edittext = findViewById(R.id.edittext);
imageView = findViewById(R.id.imageView);
iV_clean=findViewById(R.id.iV_clean);
TypedArray ta = context.obtainStyledAttributes(attrs, R.styleable.MyEditVIew);
hintText = ta.getString(R.styleable.MyEditVIew_myhintText);
}
//文字输入监听以及一些逻辑处理(未优化)
private void textAddChanged(){
edittext.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
}
@Override
public void afterTextChanged(Editable s) {
int textSum = s.toString().trim().length();
if (textSum == 0) {
if (mtextisshow == true) {
minTexthide(textview);
mtextisshow = false;
iV_clean.setVisibility(INVISIBLE);
edittext.setHint(hintText);
}
} else {
if (imgisshow) {
//设置文字非加密
HideReturnsTransformationMethod method = HideReturnsTransformationMethod.getInstance();
edittext.setTransformationMethod(method);
edittext.setSelection(edittext.getText().toString().length());
} else {
//设置文字加密
TransformationMethod method = PasswordTransformationMethod.getInstance();
edittext.setTransformationMethod(method);
//将光标移到最后
edittext.setSelection(edittext.getText().toString().length());
}
if (mtextisshow == false) {
textview.setText(hintText);
minTextshow(textview);
iV_clean.setVisibility(VISIBLE);
mtextisshow = true;
}
}
}
});
}
//两个图片的点击事件,加密,清除文字
private void imageOnClick(){
imageView.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
if (imgisshow) {
imageView.setImageResource(R.mipmap.password_show);
HideReturnsTransformationMethod method = HideReturnsTransformationMethod.getInstance();
edittext.setTransformationMethod(method);
edittext.setSelection(edittext.getText().toString().length());
imgisshow = false;
} else {
imageView.setImageResource(R.mipmap.pwd_invisible);
评论