golang 源码学习 --context
发布于: 1 小时前
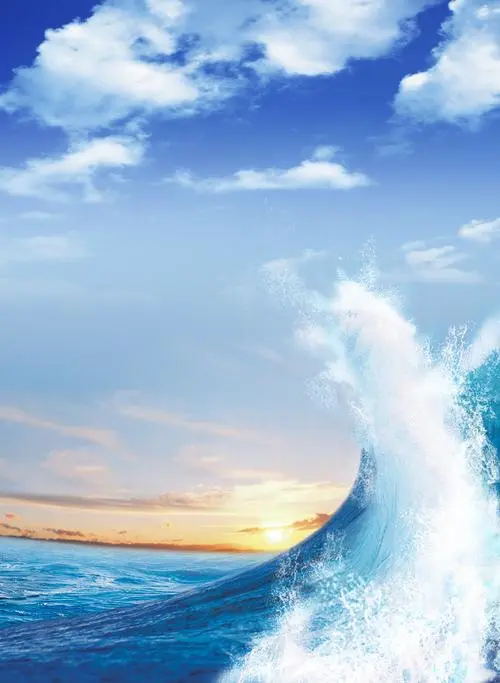
一.前言
在工作中,几乎每一个函数都有用到 context,context 结合 jaeger 完成了一次分布式请求的追踪,但是一直知其然不知其所以然,今天决定照着 golang 源码好好的探究一下 golang 中的 context。
二.源码分析
2.1 接口定义
context 携带 deadline,取消信号和其他跨越 api 边境的值,且可以被多个 goroutine 同时调用。
type Context interface {
//deadline返回ctx完成工作应该被取消的时间,当deadline没有被设置返回ok==false
Deadline() (deadline time.Time, ok bool)
//返回一个代表context完成的管道,若是context无法关闭,done返回nil
//WithCancel 安排 Done 在调用 cancel 时关闭;
//WithDeadline 安排 Done 在截止日期到期时关闭; WithTimeout 安排 Done 在超时过后关闭。
Done() <-chan struct{}
//若done没有关闭,err返回nil
//若doneclosed。err返回非空解释,如canceled如果context被cancel,DeadlineExceeded如果context
//的deadline超时
Err() error
//用于存储与此上下文关联的key值
Value(key interface{}) interface{}
}
复制代码
2.2 context 使用
2.2.1 emptyCtx|backgroud|todo
空上下文,没有截止时间,永远不会取消
type emptyCtx int
func (*emptyCtx) Deadline() (deadline time.Time, ok bool) {
return
}
func (*emptyCtx) Done() <-chan struct{} {
return nil
}
func (*emptyCtx) Err() error {
return nil
}
func (*emptyCtx) Value(key interface{}) interface{} {
return nil
}
func (e *emptyCtx) String() string {
switch e {
case background:
return "context.Background"
case todo:
return "context.TODO"
}
return "unknown empty Context"
}
复制代码
借由空指针实现了两个基础的 context
var (
background = new(emptyCtx)
todo = new(emptyCtx)
)
//它通常由主函数、初始化和测试使用,并作为传入请求的顶级context。
func Background() Context {
return background
}
//当不清楚用什么context或者周围的函数还没有拓展到接收context参数的时候使用
func TODO() Context {
return todo
}
复制代码
2.2.2 cancelCtx
2.2.2.1 基础结构
//canceler指的是一种可以直接取消的上下文
type canceler interface {
cancel(removeFromParent bool, err error)
Done() <-chan struct{}
}
// &cancelCtxKey is the key that a cancelCtx returns itself for.
var cancelCtxKey int
//可以取消的上下文,取消的时候还会将所有由该上下文派生的的子上下文一并取消
type cancelCtx struct {
Context
mu sync.Mutex // 保护并发
done atomic.Value //Value 提供一致类型值的原子加载和存储。Value 的零值从 Load 返回 nil。调用 Store 后,不得复制 Value。第一次使用后不得复制 Value。
children map[canceler]struct{} // 存储此上下文下的子上下文
err error // set to non-nil by the first cancel call
}
//获取cancelcontext的值
func (c *cancelCtx) Value(key interface{}) interface{} {
//特殊值,返回自己
if key == &cancelCtxKey {
return c
}
return c.Context.Value(key)
}
//发送结束信号
//由于done的类型特性,保证了原子的加载和存储功能
//若是done为空,需要为done赋值,开启锁用于保证并发安全性
func (c *cancelCtx) Done() <-chan struct{} {
d := c.done.Load()
if d != nil {
return d.(chan struct{})
}
c.mu.Lock()
defer c.mu.Unlock()
d = c.done.Load()
if d == nil {
d = make(chan struct{})
c.done.Store(d)
}
return d.(chan struct{})
}
//返回error
func (c *cancelCtx) Err() error {
c.mu.Lock()
err := c.err
c.mu.Unlock()
return err
}
//带cancel功能的context是可嵌套的
//a作为b,c的父级
//b,c可以派生自己的子级
//当父context取消的同时会取消所有由父context派生的子context
func (c *cancelCtx) cancel(removeFromParent bool, err error) {
if err == nil {
panic("context: internal error: missing cancel error")
}
c.mu.Lock()
if c.err != nil {
c.mu.Unlock()
return // already canceled
}
c.err = err
d, _ := c.done.Load().(chan struct{})
if d == nil {
c.done.Store(closedchan)
} else {
close(d)
}
for child := range c.children {
// NOTE: acquiring the child's lock while holding parent's lock.
child.cancel(false, err)
}
c.children = nil
c.mu.Unlock()
if removeFromParent {
removeChild(c.Context, c)
}
}
复制代码
2.2.2.2 应用
//WithCancel 返回带有新 Done channel的父级副本。
//当调用返回的取消函数或父context的 Done channel关闭时,返回的上下文的 Done 通道关闭,以先发生者为准。
//取消此上下文会释放与其关联的资源,因此一旦此上下文中运行的操作完成,代码应立即调用取消。
func WithCancel(parent Context) (ctx Context, cancel CancelFunc) {
c := newCancelCtx(parent)
propagateCancel(parent, &c)
return &c, func() { c.cancel(true, Canceled) }
}
//返回一个初始化的 cancelCtx.
func newCancelCtx(parent Context) cancelCtx {
return cancelCtx{Context: parent}
}
//当父级取消的同时需要取消父context派生的子context
//所以要为父子context建立联系
func propagateCancel(parent Context, child canceler) {
done := parent.Done()
if done == nil {
//若父永远不取消就没有必要进行关联
return // parent is never canceled
}
select {
case <-done:
// 父context已经取消就得把刚创建的子取消
child.cancel(false, parent.Err())
return
default:
}
//获取父context底层的cancelctx
if p, ok := parentCancelCtx(parent); ok {
p.mu.Lock()
if p.err != nil {
// 父context已经取消就得把刚创建的子取消
child.cancel(false, p.err)
} else {
if p.children == nil {
//子空,说明是第一次派生,需要初始化map
p.children = make(map[canceler]struct{})
}
p.children[child] = struct{}{}
}
p.mu.Unlock()
} else {
//若父进程没有cancelcontext,派生协程数+1,监听结束
atomic.AddInt32(&goroutines, +1)
go func() {
select {
case <-parent.Done():
child.cancel(false, parent.Err())
case <-child.Done():
}
}()
}
}
复制代码
2.2.3 timerCtx
2.2.3.1 基础结构
ype timerCtx struct {
cancelCtx
timer *time.Timer // Under cancelCtx.mu.
deadline time.Time
}
//deadline代表存在的deadline时间,ok代表是否有
func (c *timerCtx) Deadline() (deadline time.Time, ok bool) {
return c.deadline, true
}
func (c *timerCtx) String() string {
return contextName(c.cancelCtx.Context) + ".WithDeadline(" +
c.deadline.String() + " [" +
time.Until(c.deadline).String() + "])"
}
func (c *timerCtx) cancel(removeFromParent bool, err error) {
c.cancelCtx.cancel(false, err)
if removeFromParent {
// Remove this timerCtx from its parent cancelCtx's children.
removeChild(c.cancelCtx.Context, c)
}
c.mu.Lock()
if c.timer != nil {
c.timer.Stop()
c.timer = nil
}
c.mu.Unlock()
}
复制代码
2.2.3.2 应用
func WithTimeout(parent Context, timeout time.Duration) (Context, CancelFunc) {
return WithDeadline(parent, time.Now().Add(timeout))
}
func WithDeadline(parent Context, d time.Time) (Context, CancelFunc) {
if parent == nil {
panic("cannot create context from nil parent")
}
if cur, ok := parent.Deadline(); ok && cur.Before(d) {
// The current deadline is already sooner than the new one.
return WithCancel(parent)
}
//设置timerCtx的deadline
c := &timerCtx{
cancelCtx: newCancelCtx(parent),
deadline: d,
}
//为context传播创建条件
propagateCancel(parent, c)
dur := time.Until(d)
if dur <= 0 {
c.cancel(true, DeadlineExceeded) // 如果已经超过了截止时间,直接取消
return c, func() { c.cancel(false, Canceled) }
}
c.mu.Lock()
defer c.mu.Unlock()
if c.err == nil {
//进行时间计数,当时间到达以后会调用取消接口
c.timer = time.AfterFunc(dur, func() {
c.cancel(true, DeadlineExceeded)
})
}
return c, func() { c.cancel(true, Canceled) }
}
复制代码
2.2.4 valueCtx
2.2.4.1 基础结构
type valueCtx struct {
Context
key, val interface{}
}
// stringify tries a bit to stringify v, without using fmt, since we don't
// want context depending on the unicode tables. This is only used by
// *valueCtx.String().
func stringify(v interface{}) string {
switch s := v.(type) {
case stringer:
return s.String()
case string:
return s
}
return "<not Stringer>"
}
func (c *valueCtx) String() string {
return contextName(c.Context) + ".WithValue(type " +
reflectlite.TypeOf(c.key).String() +
", val " + stringify(c.val) + ")"
}
func (c *valueCtx) Value(key interface{}) interface{} {
if c.key == key {
return c.val
}
//父context的值子也可获取
return c.Context.Value(key)
}
复制代码
2.2.4.2 应用
func WithValue(parent Context, key, val interface{}) Context {
if parent == nil {
panic("cannot create context from nil parent")
}
if key == nil {
panic("nil key")
}
if !reflectlite.TypeOf(key).Comparable() {
panic("key is not comparable")
}
return &valueCtx{parent, key, val}
}
复制代码
划线
评论
复制
发布于: 1 小时前阅读数: 3
版权声明: 本文为 InfoQ 作者【en】的原创文章。
原文链接:【http://xie.infoq.cn/article/f6fa2996979edc4cdeff03923】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
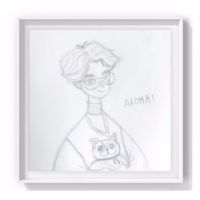
en
关注
努力分享对他人有价值的知识 2018.06.14 加入
还未添加个人简介
评论