Mybatis 学习笔记 --Mybatis 实现 CRUD,springboot 注解面试题
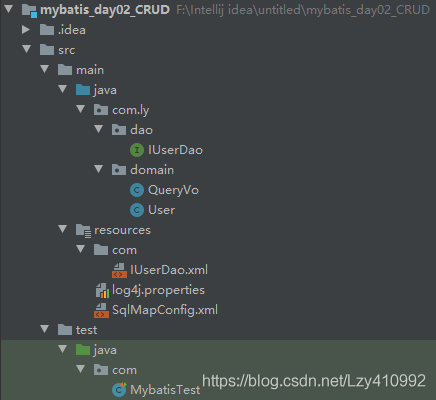
在 pom.xml 文件中添加相应的依赖 jar 包
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>mybatis_day02_CRUD</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<dependencies>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.4.5</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.11</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.12</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.10</version>
</dependency>
</dependencies>
</project>
配置 SqlMapConfig.xml:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<properties>
<property name="driver" value="com.mysql.cj.jdbc.Driver"></property>
<property name="url" value="jdbc:mysql://localhost:3306/mybatis?serverTimezone=GMT%2B8&useUnicode=true&characterEncoding=utf-8"></property>
<property name="username" value="root"></property>
<property name="password" value="123456"></property>
</properties>
<environments default="mysql">
<environment id="mysql">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="${driver}"></property>
<property name="url" value="${url}"></property>
<property name="username" value="${username}"></property>
<property name="password" value="${password}"></property>
</dataSource>
</environment>
</environments>
<mappers>
<mapper resource="com/IUserDao.xml"/>
</mappers>
</configuration>
配置封装数据库数据的 User 类:
/**
@Author: Ly
@Date: 2020-07-12 11:15
*/
public class User {
private Integer id;
private String username;
private String address;
private String sex;
private Date birthday;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", username='" + username + ''' +
", address='" + address + ''' +
", sex='" + sex + ''' +
", birthday=" + birthday +
'}';
}
}
定义封装查询条件的 QueryVo 类:
OGNL(Object Graphic Navigation Language)
表达式 ,即对象图导航语言。它是通过对象的取值方法来获取数据。在写法上把 get 给省略了。在开发中如果想实现复杂查询 ,查询条件不仅包括用户查询条件,还包括其它的查询条件(比如将用户购买商品信息也作为查询条件),这时可以使用 pojo 包装对象传递输入参数。
public class QueryVo {
private User user;
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
}
配置持久层接口 IUserDao:
/**
@Author: Ly
@Date: 2020-07-12 11:29
*/
public interface IUserDao {
/**
查询所有用户
@return
*/
List<User> findAll();
/**
保存用户
@param user
*/
void saveUser(User user);
/**
更新用户
@param user
*/
void updateUser(User user);
/**
根据 id 删除用户
@param userId
*/
void deleteUser(Integer userId);
/**
根据 id 查询一个用户 id
@param userId
*/
User findById(Integer userId);
/**
模糊查询用户信息
@param username
*/
List<User> findByName(String username);
/**
查询总用户数
*/
int findTotal();
/**
根据 queryVo 查询中的条件查询用户
*/
List<User> findUserByVo(QueryVo Vo);
}
配置接口映射文件 IUserDao.xml:
需要注意的是:其中的参数符号 #{username},#{birthday},#{birthday},#{sex},#{address}必须和 User 类的每个属性名一一对应,不可以乱写。
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.ly.dao.IUserDao">
<select id="findAll" resultType="com.ly.domain.User">
select * from user
</select>
<!--<insert id="saveUser" parameterType="com.ly.domain.User">
insert into user(us
ername,address,sex,birthday)values(#{username},#{address},#{sex},#{birthday})
</insert>-->
<insert id="saveUser" parameterType="com.ly.domain.User">
<selectKey keyProperty="id" keyColumn="id" resultType="int" order="AFTER">
select last_insert_id();
</selectKey>
insert into user(username,address,sex,birthday)values(#{username},#{address},#{sex},#{birthday})
</insert>
<update id="updateUser" parameterType="com.ly.domain.User">
update user set username=#{username},address=#{address},sex=#{sex},birthday=#{birthday} where id=#{id}
</update>
<delete id="deleteUser" parameterType="java.lang.Integer">
delete from user where id=#{id}
</delete>
<select id="findById" parameterType="INT" resultType="com.ly.domain.User">
select * from user where id=#{id}
</select>
<select id="findByName" parameterType="String" resultType="com.ly.domain.User">
select * from user where username like #{username}
</select>
<select id="findTotal" resultType="int">
select count(id) from user
</select>
<select id="findUserByVo" parameterType="com.ly.domain.QueryVo" resultType="com.ly.domain.User">
select * from user where username like #{user.username}
</select>
</mapper>
同时,在之前我们类的属性与数据库中的属性也是一一对应的,如果在没有对应,那么在执行查询操作时会发现属性名称不对应的数据无法查询出来,这时我们有两个解决方式:
1.对数据库查询语句进行操作:起别名 (这种方式的执行效率是最高的)
2.采用配置的方式:
<resultMap id="userMap" type="com...ClassName"> </resultMap>
(执行效率低,开发效率高)
<mapper namespace="com.ly.dao.IUserDao">
<resultMap id="userMap" type="com.ly.domain.User">
<id property="id" column="id"></id>
<!--非主键字段的对应
property:对应实体类中的属性名称
column:对应数据库表中的属性名称-->
<result property="username" column="username"></result>
<result property="address" column="address"></result>
<result property="sex" column="sex"></result>
<result property="birthday" column="birthday"></result>
</resultMap>
<select id="findAll" resultMap="userMap">
select * from user
</select>
</mapper>
创建 MybatisTest 类测试方法:
public class MybatisTest {
private InputStream in;
private SqlSession sqlSession;
private IUserDao userDao;
@Before//再测试方法执行之前执行
评论