前端开发之 Vue 技术栈编写表单组件
使用 Inertia + Vue 编写单页面组件
初始化 Jetstream + Inertia
开始之前,假设你已经新安装了一个系统 Laravel 8 项目,然后需要通过 Composer 安装 Jetstream 扩展包:
composer require laravel/jetstream
接下来,初始化带有 Inertia 技术栈的 Jetstream:
php artisan jetstream:install inertia
运行如下代码初始化前端依赖:
npm install && npm run dev
编译成功后,运行数据库迁移命令初始化相关数据表:
php artisan migrate
安装 & 初始化完成后,可以在 resources/js 目录下看到新创建的脚手架组件:
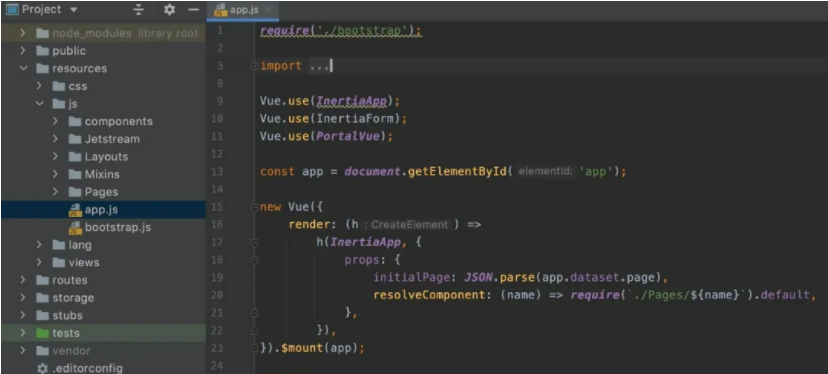
所有的 Inertia 页面组件默认位于 Pages 目录下,Jetstream 目录下存放的则是 Jetstream 自带的组件。
基于 Inertia 编写 Vue 页面组件
接下来,我们来创建一个用于发布文章的页面组件,其中还包含了独立的文章发布表单组件,在 Pages 目录下新建 Post 子目录用于存在前端培训文章相关页面的组件,然后在该目录下新建 PostCreateForm.Vue 作为表单组件,以及 Create.vue 作为页面组件。
友情提示:你可以先阅读一下 Inertia 官方文档熟悉 Inertia 基本语法,以及 Jetstream Inertia 部分文档了解如何在 Laravel 中编写 Vue 组件。
先来编写表单组件 PostCreateForm.vue:
<template><jet-form-section @submitted="storePostInformation"><template #title>发布新文章</template><template #description>这里是发布新文章页面</template>
</template>
<script>import JetLabel from './../../Jetstream/Label'import JetButton from './../../Jetstream/Button'import JetInput from './../../Jetstream/Input'import JetInputError from './../../Jetstream/InputError'import JetFormSection from './../../Jetstream/FormSection'
export default {components: {JetLabel,JetButton,JetInput,JetInputError,JetFormSection},
}</script>
就是一个正常的 Vue 单纯文件组件,只是应用了 Inertia 内置的 API 方法来提交表单,以及渲染表单验证错误信息。
然后在 Create.vue 中引入这个表单组件渲染发布文章页面:
<template><app-layout><template #header><h2 class="font-semibold text-xl text-gray-800 leading-tight">发布文章</h2></template>
</template>
<script>import AppLayout from './../../Layouts/AppLayout'import JetSectionBorder from './../../Jetstream/SectionBorder'import PostCreateForm from "./PostCreateForm";
export default {components: {AppLayout,JetSectionBorder,PostCreateForm},}</script>在这个页面组件中,使用了 Jetstream 提供的 AppLayout 组件进行页面布局。至此,就完成了前端页面的开发。
编写后端路由和控制器代码
最后,我们需要在 Laravel 应用后端注册路由和对应的控制器方法,来完成前端页面组件的渲染以及与后端的接口交互。
控制器方法
首先创建一个 PostController 控制器:
php artisan make:controller PostController 分别编写 create 方法用于渲染发布文章页面组件以及 store 方法用于处理表单提交数据的验证和处理:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;use Illuminate\Support\Facades\Validator;use Inertia\Inertia;
class PostController extends Controller{// 渲染 Inertia 页面组件 public function create(Request $request){return Inertia::render('Post/Create');}
{Validator::make($request->all(), ['title' => ['required', 'max:255'],'author' => ['required'],'body' => ['required'],], ['required' => ':attribute 字段不能为空'])->validateWithBag('storePostInformation');
}
store 方法很简单,只是处理了表单提交数据的验证,具体语法可以参考 Laravel 验证文档,create 方法则使用了 Jetstream 提供的语法实现 Inertia 页面组件的渲染,这里我们只需要渲染 Blade 视图模板一样,渲染出基于 Inertia 编写的 Vue 页面组件即可,非常方便,前面我们提到过这些页面组件默认存放在 resources/js/Pages 目录下,所以这里会去该目录下定位 Post/Create.vue 页面组件进行渲染。
如果是修改表单的话,你还可以设置 Inertia::render 函数的第二个参数以 props 传递变量数据到页面组件,我们这里是创建表单,所以就没有传递数据。
注册路由
然后在 routes/web.php 中注册上述控制器方法对应的路由:
use App\Http\Controllers\PostController;
Route::group(['middleware' => config('jetstream.middleware', ['web'])], function () {
Route::group(['middleware' => ['auth', 'verified']], function () {
Route::get('/post/create', [PostController::class, 'create']);
Route::post('/post', [PostController::class, 'store']);
});
});
由于我们在 Vue 页面组件中使用了 AppLayout 这个布局组件,其中涉及了需要认证用户信息的渲染,所以这里需要应用相关的认证中间件。
使用 Vue 组件渲染页面发布文章
如果是在本地开发的话,建议运行 npm run watch 监听前端代码修改并自动编译,这样前端资源文件的调整可以立即生效,我们这里运行这个命令编译前端资源。
编译成功后,桌面右上角会有通知弹出,然后到浏览器访问 /post/create 路由,就可以看到上述 Post/Create.vue 页面组件渲染的文章发布页面了(首次访问的话需要注册登录):
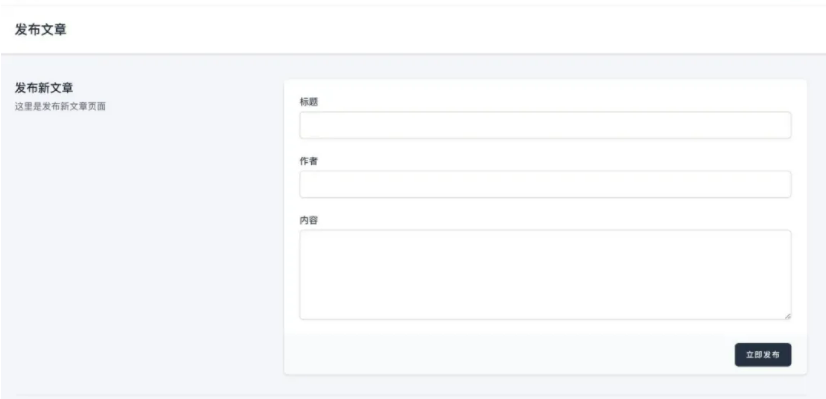
我们尝试填写一些字段,然后提交,会有报错提示:
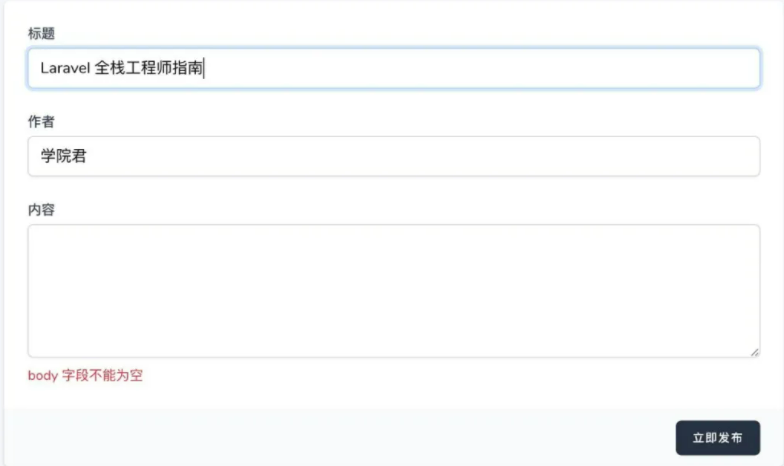
说明页面组件和表单组件都已经可以正常渲染和工作。
小结
好了,以上就是基于 Laravel 8 新引入的 Jetstream 扩展包提供的 Inertia 技术栈编写 Vue 页面组件的简单示例,如果你对基于 Livewire 技术栈编写 Blade 页面组件感兴趣,可以查看同一功能的实现实例。
尽管 Laravel 中提供了这样的对新手更加友好的前端组件开发快速入门解决方案,不过接下来我们还是要完成基于纯 Vue 语法来编写表单组件,你可以对比选择适用自己的技术方案。
转自 web 前端开发
评论