Java 并发包源码学习系列:LBD 双端阻塞队列源码解析,linux 内核架构与底层原理
*/
transient Node<E> first;
/**
指向最后一个节点
Invariant: (first == null && last == null) ||
*/
transient Node<E> last;
/** 节点数量 */
private transient int count;
/** 队列容量 */
private final int capacity;
/** 保证同步 */
final ReentrantLock lock = new ReentrantLock();
/** take 操作发生的条件 */
private final Condition notEmpty = lock.newCondition();
/** put 操作发生的条件 */
private final Condition notFull = lock.newCondition();
}
linkFirst
=============
尝试将节点加入到 first 之前,更新 first,如果插入之后超出容量,返回 false。
private boolean linkFirst(Node<E> node) {
// assert lock.isHeldByCurrentThread();
if (count >= capacity)
return false;
Node<E> f = first;
node.next = f;
first = node;
if (last == null)
last = node;
else
f.prev = node;
++count;
notEmpty.signal();
return true;
}
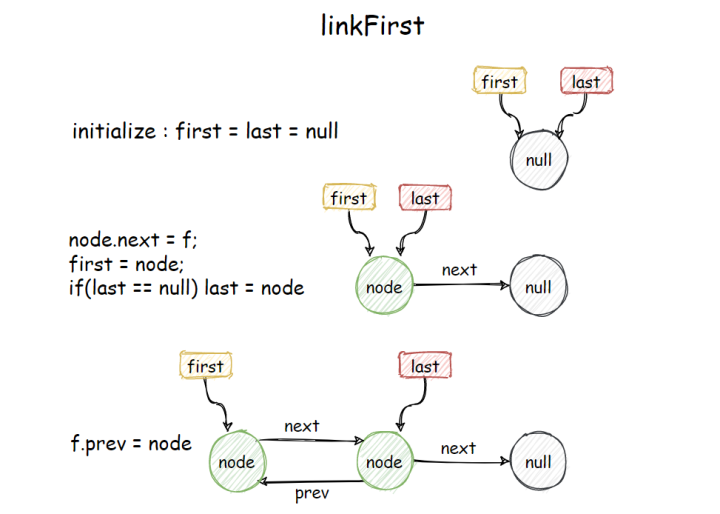
linkLast
============
在 last 节点后加入节点 node,更新 last。如果插入之后超出容量,返回 false。
private boolean linkLast(Node<E> node) {
// assert lock.isHeldByCurrentThread();
if (count >= capacity)
return false;
Node<E> l = last;
node.prev = l;
last = node;
if (first == null)
first = node;
else
l.next = node;
++count;
notEmpty.signal();// 满足 notEmpty 条件
return true;
}
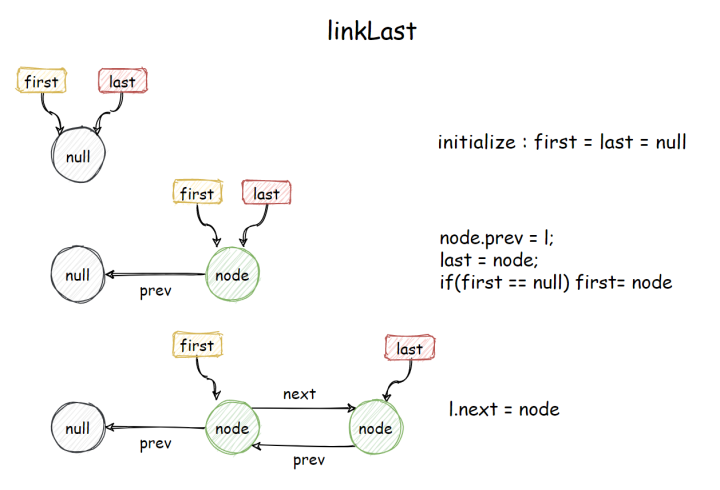
unlinkFirst
===============
移除 first 节点,并返回其 item 值,如果队列为空,则返回 full。
private E unlinkFirst() {
// assert lock.isHeldByCurrentThread();
Node<E> f = first;
if (f == null)
return null;
Node<E> n = f.next;
E item = f.item;
f.item = null;
f.next = f; // help GC
first = n;
if (n == null)
last = null;
else
n.prev = null;
--count;
notFull.signal();// 满足 notFull 条件
return item;
}
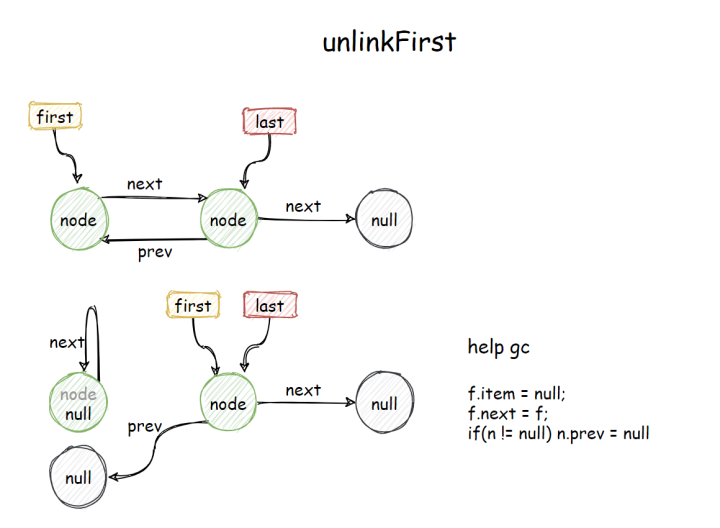
unlinkLast
==============
移除 last 节点,并返回其 item 值,如果队列为空,则返回 full。
private E unlinkLast() {
// assert lock.isHeldByCurrentThread();
Node<E> l = last;
if (l == null)
retur
n null;
Node<E> p = l.prev;
E item = l.item;
l.item = null;
l.prev = l; // help GC
last = p;
if (p == null)
first = null;
else
p.next = null;
--count;
notFull.signal(); // 满足 notFull 条件
return item;
}
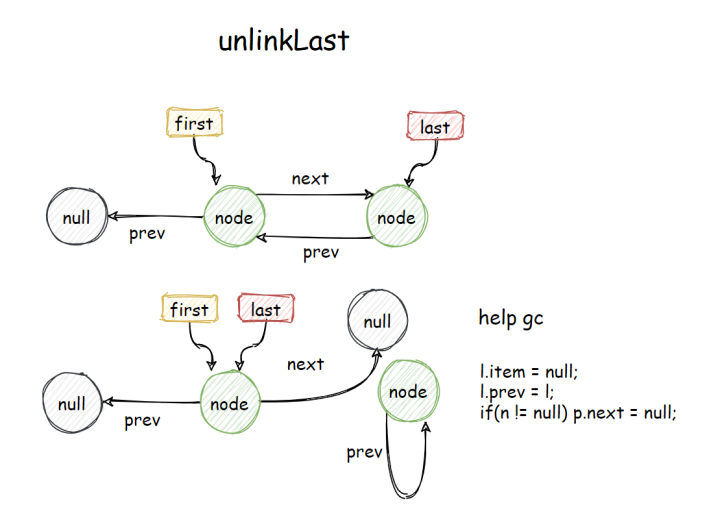
unlink
==========
移除任意一个节点,注意这里并没有操作 x 本身的连接,因为它可能仍被 iterator 使用着。
void unlink(Node<E> x) {
// assert lock.isHeldByCurrentThread();
Node<E> p = x.prev;
Node<E> n = x.next;
// 移除的是 first
if (p == null) {
unlinkFirst();
// 移除的是 last
评论