SpringBoot 下用 Kyro 作为 Redis 序列化工具
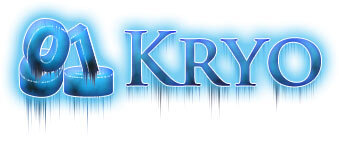
欢迎访问我的 GitHub
这里分类和汇总了欣宸的全部原创(含配套源码):https://github.com/zq2599/blog_demos
本篇概览
有时候我们需要将 Java 对象实例存入 Redis,常用方法有两种:
将对象序列化成字符串后存入 Redis;
将对象序列化成 byte 数组后存入 Redis;
本章实战上述第二种方式,并且序列化工具选择了 Kyro,为了便于开发和验证,将代码写在一个基于 SpringBoot 的 web 工程中;
源码下载
本章实战的源码可以在 github 下载,地址和链接信息如下表所示:
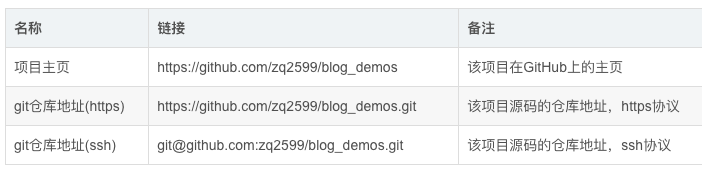
这个 git 项目中有多个文件夹,本章源码在 springboot-redis-kyro-demo 文件夹下,如下图所示:
环境信息
JDK:1.8.0_144;
SpringBoot:1.4.1.RELEASE;
Kyro:4.0.0;
Redis:3.2.11;
开发步骤列表
动手前先将整体步骤梳理一下:
准备 Reids 环境;
创建 SpringBoot 工程,添加依赖;
在 application.properties 中配置 Redis 相关信息;
创建基于 Kyro 的序列化接口实现类;
创建 Redis 配置类;
将存、取、删除等针对对象的基本操作封装成一个服务类;
开发一个 Controller,对应几个 web 接口,用来验证对 Redis 的存、取、删除操作;
启动工程,通过 web 请求操作,并在 Redis 后台检查数据;
步骤已经列清楚了,开始实战吧!
准备 Redis 环境
如何安装 Reids 就不在本章展开了,请自行准备,我这里为了省事是在 Docker 上装的,只需以下一行命令即可(前提是 Docker 可用):
创建 SpringBoot 工程,添加依赖
spring-boot-starter-parent 的版本是 1.4.1.RELEASE;
基于 maven 创建 SpringBoot 工程,需要依赖:spring-boot-starter-web、spring-boot-starter-data-redis;
在 pom.xml 文件中添加 kyro、fastjson 依赖,如下:
在 application.properties 中配置 Redis 相关信息
application.properties 中的配置信息如下,请注意 IP 地址和端口:
创建基于 Kyro 的序列化接口实现类
创建 RedisSerializer 接口的实现类,后续的序列化和反序列化操作都由该类完成:
上述代码有以下三点需要注意:
kryos 是 ThreadLocal 对象,由 ThreadLocal.withInitial 创建,如此一来,每次调用 kryos.get 方法,都能取出当前线程对应的 Kryo 实例,如果没有会调用 new 方法创建;
serialize 方法负责将对象实例序列化成 byte 数组;
deserialize 方法复制将 byte 数组反序列化成对象实例;
创建 Redis 配置类
Redis 配置类如下,通过 setValueSerializer 方法将 KryoRedisSerializer 设置位 value 的序列化器:
将存、取、删除等针对对象的基本操作封装成一个服务类
本次会用到存、取、删除等操作,所以做个简单的封装,实际使用中,您可以自己定制:
以上代码有一处需注意:Redis 连接对象 RedisConnection 使用完毕后,一定要记得释放!
上面用到的 Person 是个简单对象,如下:
启动工程,通过 web 请求操作,并在 Redis 后台检查数据
现在可以启动 SpringBoot 工程进行验证了:
运行 SpringBootApplication;
在浏览器输入 http://localhost:8080/add/1/Jerry/11 即可新增一条记录(注意 localhost:8080 换成您的服务所在地址和端口),如下图:
用 redis-cli 命令登录 redis,执行命令 get person_1,看到内容如下:
在浏览器输入 http://localhost:8080/get/1 即可查询记录,如下图:
在浏览器输入 http://localhost:8080/del/1 即可删除记录,如下图:
在浏览器输入 http://localhost:8080/get/1 查询记录,会提示找不到记录,如下图:
Redis 后台也查不到了:
至此,使用 Kyro 作为 redis 序列化工具的实战已经完成,希望能对您的开发提供一些参考;
欢迎关注 InfoQ:程序员欣宸
版权声明: 本文为 InfoQ 作者【程序员欣宸】的原创文章。
原文链接:【http://xie.infoq.cn/article/de2fe3ae6d44c1de1a8e59d86】。文章转载请联系作者。
评论