Java 之 Spring 基础与 IOC
<version>5.3.9</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.3.9</version>
</dependency>
Spring 两大特点
控制反转(IOC)
面向切片编程(AOP)支持事务处理
Spring
控制反转:IOC 是一种设计思想,通过描述(XML 或注解)并通过第三方生产或获取对象的方式。之前对象的创建与对象的依赖关系完全在 java 硬编码程序中,控制权在程序;实现 IOC 后,控制权在第三方,实现降藕。
在 Spring 中实现控制反转的是 IoC 容器 ,实现方法是 依赖注入 DI(Dependency Injection,DI)
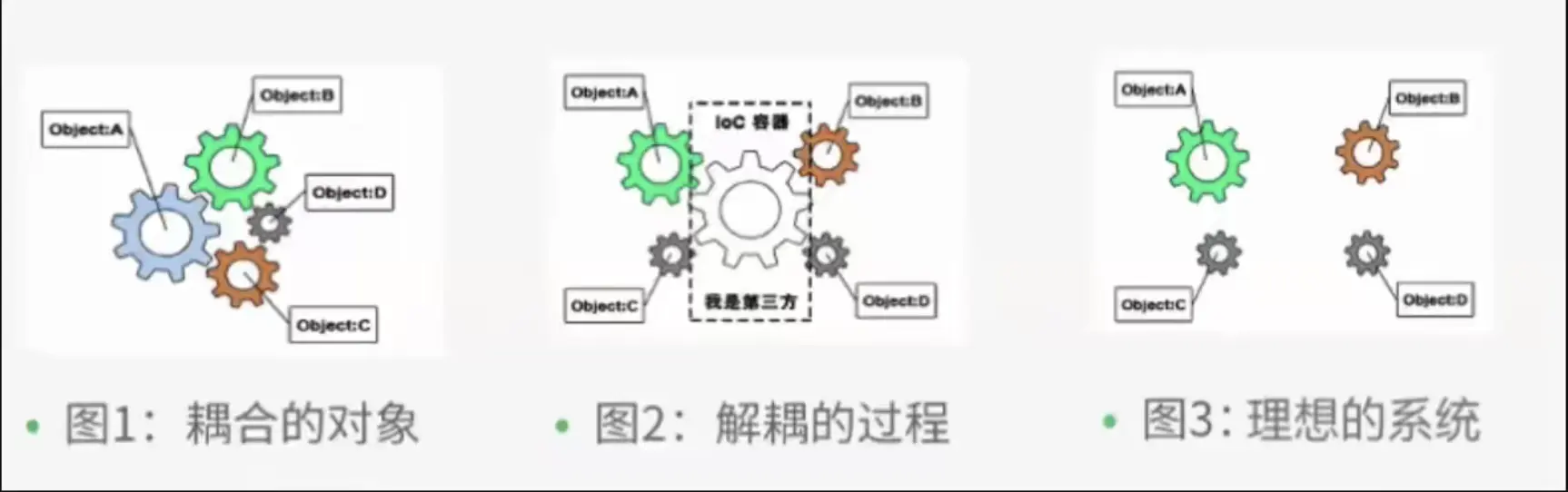
引用狂神的一个例子简单理解下
private UserDao userDao = null;
public UserServiceImpl(){
userDao = new UserDaoImpl();
}
在之前我们使用 JavaWeb 写 service=>dao 的时候,是通过如上的方式去实现的,项目构建大概如下
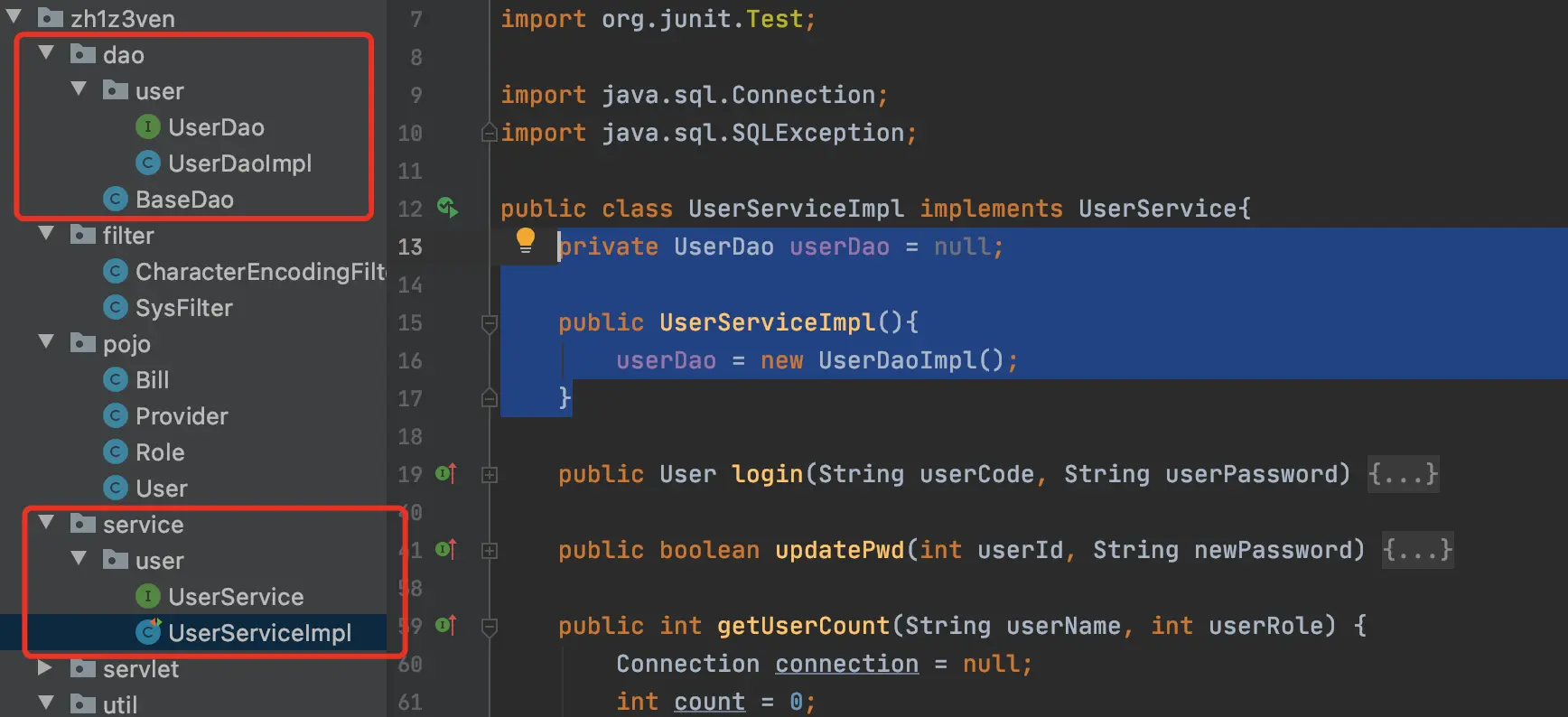
那么如果此时我的 UserDao 接口又了一个新的实现类暂且为 UserDaoImpls ,这个实现类中有新的功能实现,那么就需要到 UserServiceImpl 中再去构造方法加一段如下的代码:
userDao = new UserDaoImpls();
那么这时如果该项目还没发布那到无所谓,如果是已经上线的项目是不可能这样重新去修改代码的,或者如果有 n 个 new,就要修改 n 处。
解决这个问题就是通过一个 set 方法。如下:
public void setUserDao(UserDao userDao) {
this.userDao = userDao;
}
在构造方法中实例化对象的这个操作,改为利用 set 封装并将需要 new 的实现类的名称变为 set 方法的参数,实现用户可控的去 new 一个新的实现类从而添加新的功能展示到页面。
而这个思想就是控制反转(IOC)的原型,将 new 实现类对象的主动权交给了用户而不是程序,从本质上解决了上面的问题,也实现了降藕。
Hello.java
package com.zh1z3ven.pojo;
public class Hello {
private String str;
public Hello() {
}
public Hello(String str) {
this.str = str;
}
public String getStr() {
return str;
}
public void setStr(String str) {
this.str = str;
}
@Override
public String toString() {
return "Hello{" +
"str='" + str + ''' +
'}';
}
}
Beans.xml
一个 bean 标签代表一个对象, id 代表在 Spring 中这个类要实例化生成的对象的名字, class 指定这个实体类
设置对象的属性值
引用 Spring 容器中创建的对象
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="hello" class="com.zh1z3ven.pojo.Hello">
<property name="str" value="Spring"/>
</bean>
</beans>
Test.java
在 Spring 中也存在一个上下文,通过 ApplicationContext context = new ClassPathXmlApplicationContext(“beans.xml”); 传入 xml 配置文件名字来获取该 xml 文件的上下文对象。利用 ApplicationContext#getBean() 传入在 xml 中配置的该类的 id 获取该实体类对象。
public class MyTest {
public static void main(String[] args) {
//获取 Spring 上下文对象
ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml");
//从 Spring 中取出对象
Hello hello = (Hello) context.getBean("hello");
System.out.println(hello);
}
}
PS:在配置文件加载的时候,通过 bean 标签注册的对象就已经在 Spring IoC 容器中初始化了。
总体来说就两种方式:
无参构造
默认使用
有参构造
下标赋值
<bean id="hello2" class="com.zh1z3ven.pojo.Hello">
<constructor-arg index="0" value="Spring2"/>
</bean>
类型复制
<bean id="hello3" class="com.zh1z3ven.pojo.Hello">
<constructor-arg type="java.lang.String" value="Spring3"/>
</bean>
属性名赋值
<bean id="hello4" class="com.zh1z3ven.pojo.Hello">
<constructor-arg name="str" value="Spring4"/>
</bean>
import 标签可导入其他 beans.xml 配置文件,而 applicationContext.xml 到时可作为总 bean 的配置文件,而不需要导入多个 xml
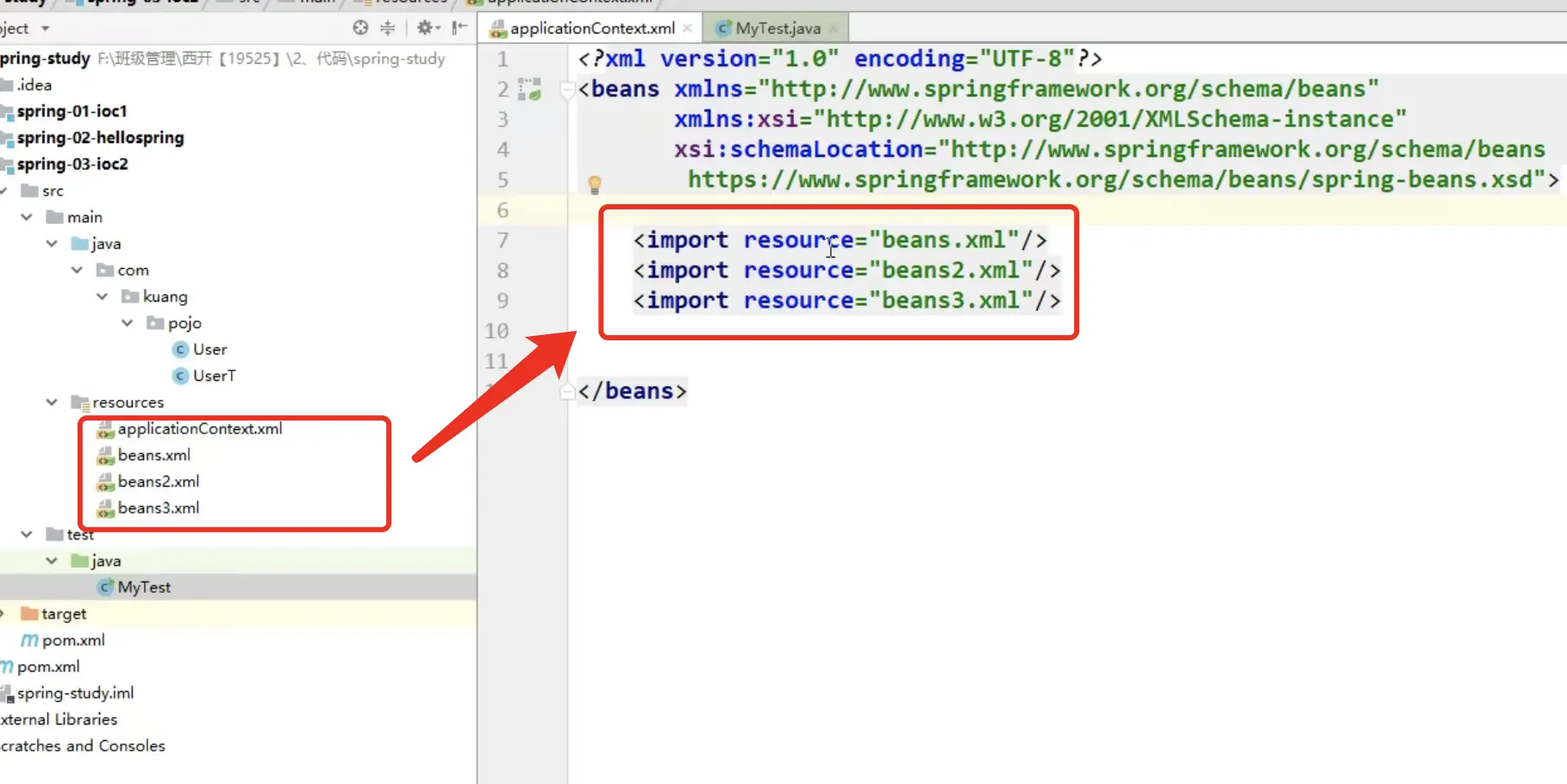
依赖注入(Dependency Injection,DI)
PS:一定需要 pojo 中实现 set 才可以利用 bean 标签注入
1.构造器注入
也就是上面提到的创建对象的方式,分为无参构造和有参构造
无参构造
默认使用
有参构造
下标赋值
<bean id="hello2" class="com.zh1z3ven.pojo.Hello">
<constructor-arg index="0" value="Spring2"/>
</bean>
类型复制
<bean id="hello3" class="com.zh1z3ven.pojo.Hello">
<constructor-arg type="java.lang.String" value="Spring3"/>
</bean>
属性名赋值
<bean id="hello4" class="com.zh1z3ven.pojo.Hello">
<constructor-arg name="str" value="Spring4"/>
</bean>
2.set 注入
依赖:bean 对象的注入依赖于 Spring 容器
注入:bean 对象的属性,由容器来注入
3.拓展注入
Student.java
public class Student {
private String name;
private Address address;
private String[] books;
private List<String> hobbys;
private Map<String,String> card;
private Set<String> games;
private String wife;
private String apache;
private Properties info;
public String getApache() {
return apache;
}
public void setApache(String apache) {
this.apache = apache;
}
public String[] getBooks() {
return books;
}
public void setBooks(String[] books) {
this.books = books;
}
public List<String> getHobbys() {
return hobbys;
}
public void setHobbys(List<String> hobbys) {
this.hobbys = hobbys;
}
public Map<String, String> getCard() {
return card;
}
public void setCard(Map<String, String> card) {
this.card = card;
}
public Set<String> getGames() {
return games;
}
public void setGames(Set<String> games) {
this.games = games;
}
public String getWife() {
return wife;
}
public void setWife(String wife) {
this.wife = wife;
}
public Properties getInfo() {
return info;
}
public void setInfo(Properties info) {
this.info = info;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + ''' +
", address=" + address +
", books=" + Arrays.toString(books) +
", hobbys=" + hobbys +
", card=" + card +
", games=" + games +
", wife='" + wife + ''' +
", info=" + info +
'}';
}
}
beans.xml
<bean id="address" class="com.zh1z3ven.pojo.Address">
<property name="address" value="beijing"/>
</bean>
<bean id="student1" class="com.zh1z3ven.pojo.Student">
<property name="name" value="zh1z3ven"/>
<property name="address" ref="address"/>
<property name="books">
<array>
<value>红楼梦</value>
<value>西游记</value>
<value>水浒传</value>
<value>三国演义</value>
</array>
</property>
<property name="hobbys">
<list>
<value>听音乐</value>
<value>看电影</value>
<value>敲代码</value>
<value>写文章</value>
</list>
</property>
<property name="card">
<map>
<entry key="银行卡" value="1"></entry>
<entry key="身份证" value="2"></entry>
<entry key="学生证" value="3"></entry>
<entry key="电话卡" value="4"></entry>
<entry key="校园卡" value="5"></entry>
</map>
</property>
<property name="games">
<set>
<value>LOL</value>
<value>CF</value>
<value>qq</value>
</set>
</property>
<property name="wife">
<null></null>
</property>
<property name="apache" value=""/>
<property name="info">
<props>
<prop key="id">10</prop>
<prop key="city">北京</prop>
</props>
</property>
</bean>
1.p 命名空间注入,可以直接注入属性值,类似于 bean 标签中 property-name-value
Beans.xml 头部需导入
xmlns:p="http://www.springframework.org/schema/p"
<bean id="user1" class="com.zh1z3ven.pojo.User" p:name="zh1z3ven1" p:age="18"/>
2.c 命名空间注入,通过构造器注入,类似于 construct-args(需要实现有参构造)
xmlns:c="http://www.springframework.org/schema/c"
<bean id="user2" class="com.zh1z3ven.pojo.User" c:name="zh1z3ven2" p:age="19"/>
@Test
public void test(){
ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml");
User user1 = context.getBean("user1", User.class);
System.out.println(user1.getName());
System.out.println(user1.getAge());
User user2 = context.getBean("user2", User.class);
System.out.println(user2.getName());
System.out.println(user2.getAge());
}
bean 的作用域
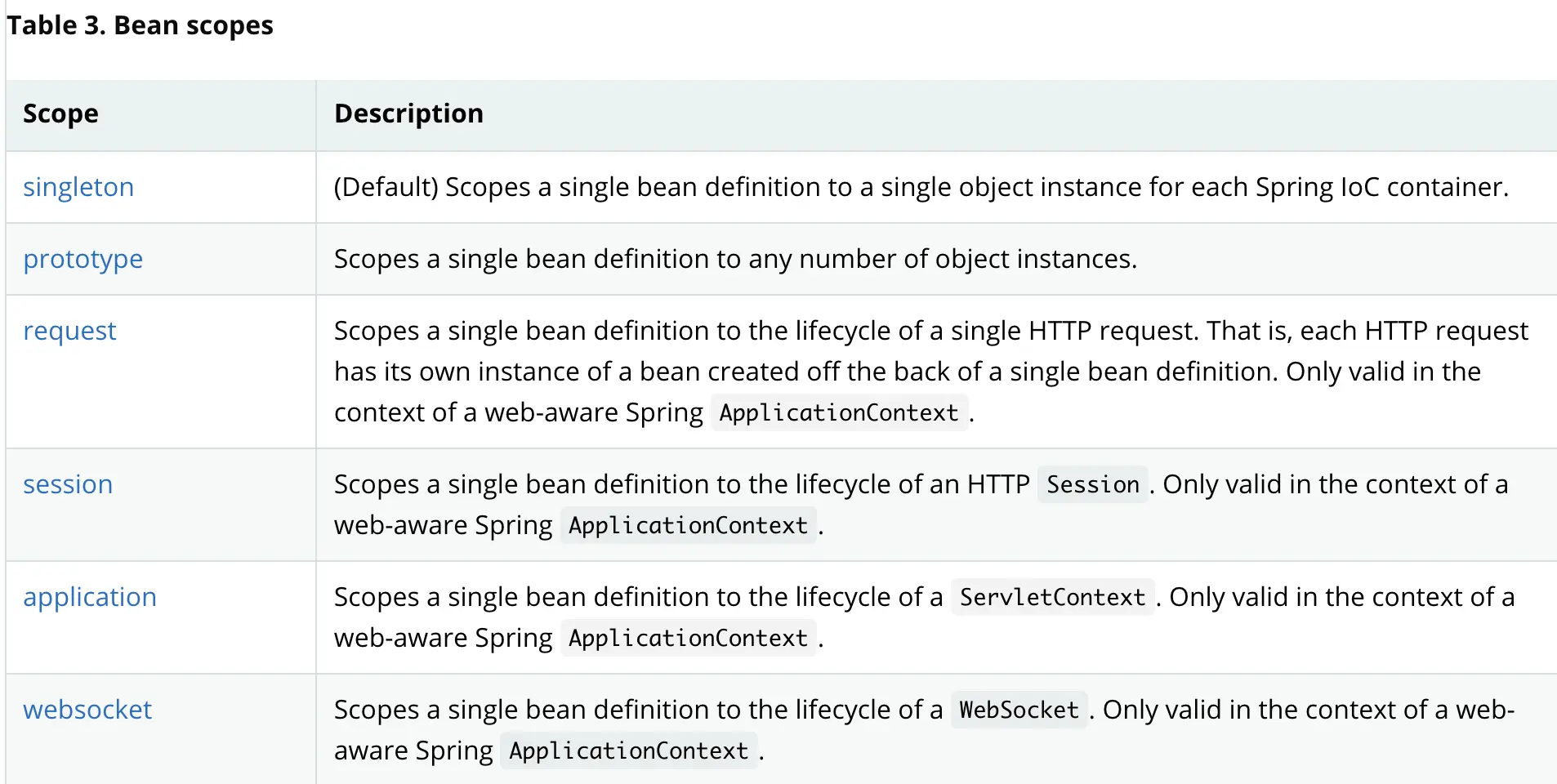
默认 bean 为 scope = singleton 单例模式运行的
显示定义:<bean id="user1" class="com.zh1z3ven.pojo.User" p:name="zh1z3ven1" p:age="18" scope="singleton"/>
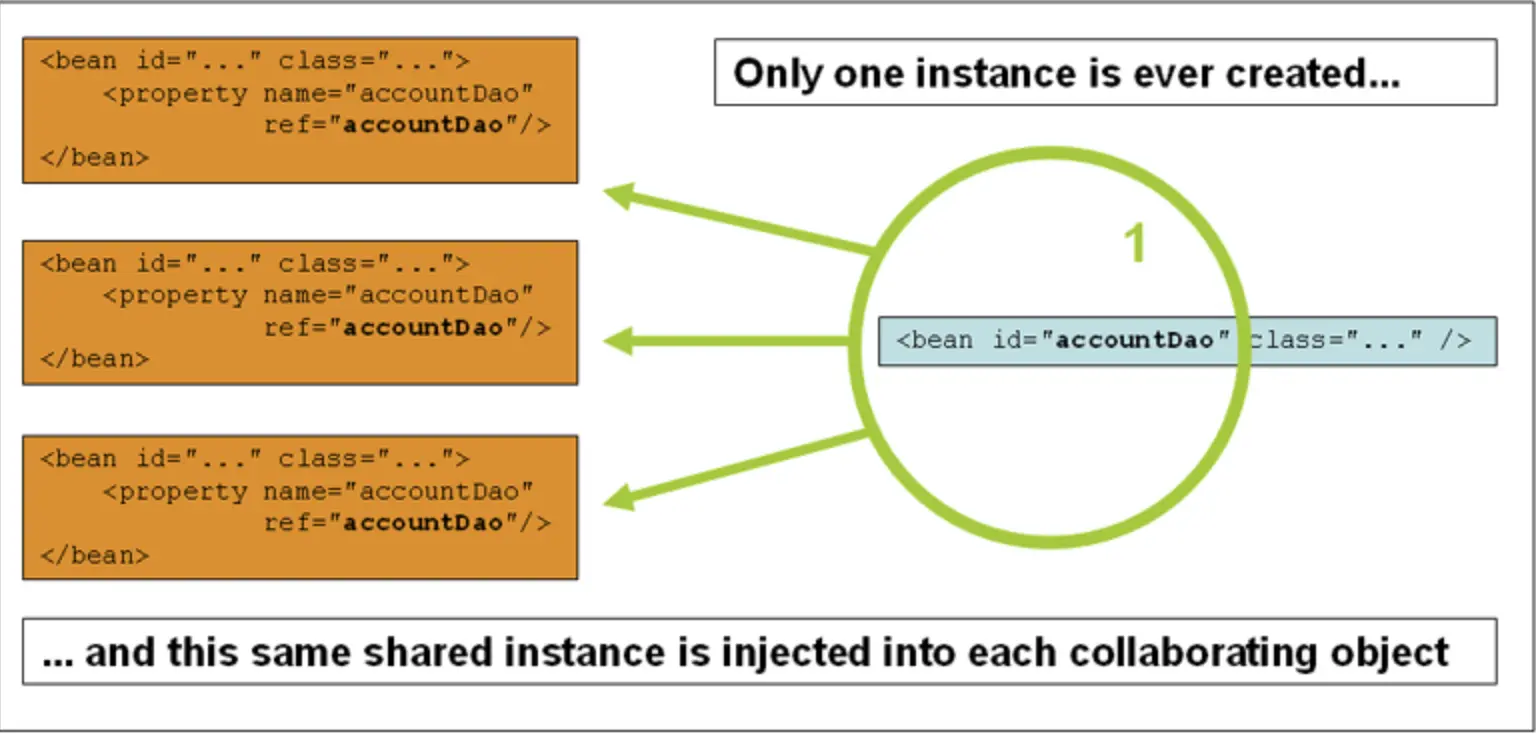
单例模式,共享一个对象,比如如下例子,getBean 指向的是同一个 bean,那么在 Spring IoC 容器中仅仅会生成一个"user2"对象保存在内存中,当调用 ApplicationContext.getBean(“user2”)时返回该对象
<bean id="user2" class="com.zh1z3ven.pojo.User" c:name="zh1z3ven2" c:age="19"/>
@Test
public void test(){
ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml");
User user1 = context.getBean("user2", User.class);
User user2 = context.getBean("user2", User.class);
System.out.println(user1==user2);
}
原型模式 prototype 与 singleton 不同,每次上下文去 getBean()时都会在 Spring IoC 容器内创建一次该对象
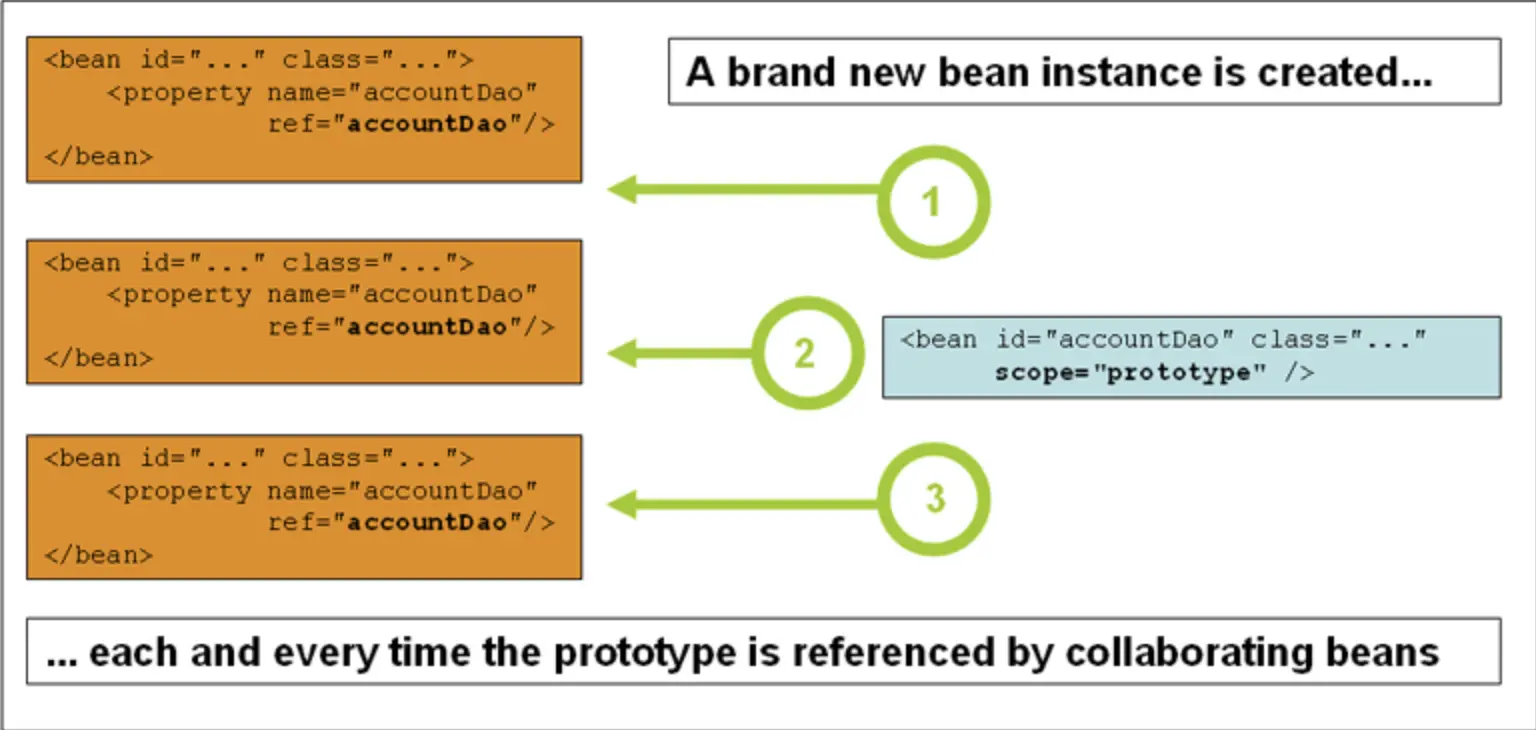
还是拿上面的测试代码,可以发现已经不是同一个对象了,有点类似于多态
public voi
d test2(){
ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml");
User user1 = context.getBean("user2", User.class);
User user2 = context.getBean("user2", User.class);
System.out.println(user1==user2);
}
而其余的生命周期在 Web 中才会遇到。
评论