*/
static final int hash(Object key) {
int h;
return (key == null) ? 0 : (h = key.hashCode()) ^ (h >>> 16);
复制代码
}
复制代码
key 的哈希值通过它自身**hashCode**的高十六位与低十六位进行亦或得到。这么做得原因是因为,由于哈希表的大小固定为 2 的幂次方,那么某个 key 的 hashCode 值大于 table.length,其高位就不会参与到 hash 的计算(对于某个 key 其所在的桶的位置的计算为 `hash & (table.length - 1)`)。因此通过`hashCode()`的高16位异或低16位实现的:`(h = key.hashCode()) ^ (h >>> 16)`,主要是从速度、功效、质量来考虑的,保证了高位 Bits 也能参与到 Hash 的计算。
### tableSizeFor函数
复制代码
/**
*/
static final int tableSizeFor(int cap) {
int n = cap - 1;
n |= n >>> 1;
n |= n >>> 2;
n |= n >>> 4;
n |= n >>> 8;
n |= n >>> 16;
return (n < 0) ? 1 : (n >= MAXIMUM_CAPACITY) ? MAXIMUM_CAPACITY : n + 1;
复制代码
}
复制代码
根据注释可以知道,这个函数返回大于或等于**cap**的最小二的整数次幂的值。比如对于3,返回4;对于10,返回16。详解如下: 假设对于**n**(32位数)其二进制为 01xx...xx, n >>> 1,进行无符号右移一位, 001xx..xx,位或得 011xx..xx n >>> 2,进行无符号右移两位, 00011xx..xx,位或得 01111xx..xx 依此类推,无符号右移四位再进行位或将得到8个1,无符号右移八位再进行位或将得到16个1,无符号右移十六位再进行位或将得到32个1。根据这个我们可以知道进行这么多次无符号右移及位或操作,那么可让数**n**的二进制位最高位为1的后面的二进制位全部变成1。此时进行 +1 操作,即可得到最小二的整数次幂的值。(《高效程序的奥秘》第3章——2的幂界方 有对此进行进一步讨论,可自行查看) 回到上面的程序,之所以在开头先进行一次 -1 操作,是为了防止传入的**cap**本身就是二的幂次方,此时得到的就是下一个二的幂次方了,比如传入4,那么在不进行 -1 的情况下,将得到8。
### 构造函数
复制代码
/**
*/
public HashMap(int initialCapacity, float loadFactor) {
if (initialCapacity < 0)
throw new IllegalArgumentException("Illegal initial capacity: " +
initialCapacity);
if (initialCapacity > MAXIMUM_CAPACITY)
initialCapacity = MAXIMUM_CAPACITY;
if (loadFactor <= 0 || Float.isNaN(loadFactor))
throw new IllegalArgumentException("Illegal load factor: " +
loadFactor);
this.loadFactor = loadFactor;
//返回2的幂次方
this.threshold = tableSizeFor(initialCapacity);
复制代码
}
复制代码
对于上面的构造器,我们需要注意的是`this.threshold = tableSizeFor(initialCapacity);`这边的 threshold 为 2的幂次方,而不是`capacity * load factor`,当然此处并非是错误,因为此时 table 并没有真正的被初始化,初始化动作被延迟到了`putVal()`当中,所以 threshold 会被重新计算。
复制代码
/**
*/
public HashMap(int initialCapacity) {
this(initialCapacity, DEFAULT_LOAD_FACTOR);
复制代码
}
/**
*/
public HashMap() {
this.loadFactor = DEFAULT_LOAD_FACTOR; // all other fields defaulted
复制代码
}
/**
Constructs a new <tt>HashMap</tt> with the same mappings as the
specified <tt>Map</tt>. The <tt>HashMap</tt> is created with
default load factor (0.75) and an initial capacity sufficient to
hold the mappings in the specified <tt>Map</tt>.
@param m the map whose mappings are to be placed in this map
@throws NullPointerException if the specified map is null
*/
public HashMap(Map<? extends K, ? extends V> m) {
this.loadFactor = DEFAULT_LOAD_FACTOR;
putMapEntries(m, false);
复制代码
}
复制代码
/**
返回指定 key 所对应的 value 值,当不存在指定的 key 时,返回 null。
当返回 null 的时候并不表明哈希表中不存在这种关系的映射,有可能对于指定的 key,其对应的值就是 null。
因此可以通过 containsKey 来区分这两种情况。
*/
public V get(Object key) {
Node<K,V> e;
return (e = getNode(hash(key), key)) == null ? null : e.value;
复制代码
}
/**
*/
final Node<K,V> getNode(int hash, Object key) {
Node<K,V>[] tab; Node<K,V> first, e; int n; K k;
if ((tab = table) != null && (n = tab.length) > 0 &&
(first = tab[(n - 1) & hash]) != null) {
if (first.hash == hash && // always check first node
((k = first.key) == key || (key != null && key.equals(k))))
return first;
if ((e = first.next) != null) {
if (first instanceof TreeNode)
return ((TreeNode<K,V>)first).getTreeNode(hash, key);
do {
if (e.hash == hash &&
((k = e.key) == key || (key != null && key.equals(k))))
return e;
} while ((e = e.next) != null);
}
}
return null;
复制代码
}
复制代码
/**
*/
public V put(K key, V value) {
return putVal(hash(key), key, value, false, true);
复制代码
}
/**
@param onlyIfAbsent if true, don't change existing value
1.判断哈希表 table 是否为空,是的话进行扩容操作
2.根据键 key 计算得到的 哈希桶数组索引,如果 table[i] 为空,那么直接新建节点
3.判断 table[i] 的首个元素是否等于 key,如果是的话就更新旧的 value 值
4.判断 table[i] 是否为 TreeNode,是的话即为红黑树,直接在树中进行插入
5.遍历 table[i],遍历过程发现 key 已经存在,更新旧的 value 值,否则进行插入操作,插入后发现链表长度大于 8,则将链表转换为红黑树
*/
final V putVal(int hash, K key, V value, boolean onlyIfAbsent,
boolean evict) {
Node<K,V>[] tab; Node<K,V> p; int n, i;
//哈希表 table 为空,进行扩容操作
if ((tab = table) == null || (n = tab.length) == 0)
n = (tab = resize()).length;
// tab[i] 为空,直接新建节点
if ((p = tab[i = (n - 1) & hash]) == null)
tab[i] = newNode(hash, key, value, null);
else {
Node<K,V> e; K k;
//tab[i] 首个元素即为 key,更新旧值
if (p.hash == hash &&
((k = p.key) == key || (key != null && key.equals(k))))
e = p;
//当前节点为 TreeNode,在红黑树中进行插入
else if (p instanceof TreeNode)
e = ((TreeNode<K,V>)p).putTreeVal(this, tab, hash, key, value);
else {
//遍历 tab[i],key 已经存在,更新旧的 value 值,否则进心插入操作,插入后链表长度大于8,将链表转换为红黑树
for (int binCount = 0; ; ++binCount) {
if ((e = p.next) == null) {
p.next = newNode(hash, key, value, null);
//链表长度大于8
if (binCount >= TREEIFY_THRESHOLD - 1) // -1 for 1st
treeifyBin(tab, hash);
break;
}
// key 已经存在
if (e.hash == hash &&
((k = e.key) == key || (key != null && key.equals(k))))
break;
p = e;
}
}
//key 已经存在,更新旧值
if (e != null) { // existing mapping for key
V oldValue = e.value;
if (!onlyIfAbsent || oldValue == null)
e.value = value;
afterNodeAccess(e);
return oldValue;
}
}
//HashMap插入元素表明进行了结构性调整
++modCount;
//实际键值对数量超过 threshold,进行扩容操作
if (++size > threshold)
resize();
afterNodeInsertion(evict);
return null;
复制代码
}
复制代码
/**
*/
final Node<K,V>[] resize() {
Node<K,V>[] oldTab = table;
int oldCap = (oldTab == null) ? 0 : oldTab.length;
int oldThr = threshold;
int newCap, newThr = 0;
if (oldCap > 0) {
//超过最大容量,不再进行扩容
if (oldCap >= MAXIMUM_CAPACITY) {
threshold = Integer.MAX_VALUE;
return oldTab;
}
//容量没有超过最大值,容量变为原来两倍
else if ((newCap = oldCap << 1) < MAXIMUM_CAPACITY &&
oldCap >= DEFAULT_INITIAL_CAPACITY)
//阈值变为原来两倍
newThr = oldThr << 1; // double threshold
}
else if (oldThr > 0) // initial capacity was placed in threshold
//调用了HashMap的带参构造器,初始容量用threshold替换,
//在带参构造器中,threshold的值为 tableSizeFor() 的返回值,也就是2的幂次方,而不是 capacity * load factor
newCap = oldThr;
else { // zero initial threshold signifies using defaults
//初次初始化,容量和阈值使用默认值
newCap = DEFAULT_INITIAL_CAPACITY;
newThr = (int)(DEFAULT_LOAD_FACTOR * DEFAULT_INITIAL_CAPACITY);
}
if (newThr == 0) {
//计算新的阈值
float ft = (float)newCap * loadFactor;
newThr = (newCap < MAXIMUM_CAPACITY && ft < (float)MAXIMUM_CAPACITY ?
(int)ft : Integer.MAX_VALUE);
}
threshold = newThr;
@SuppressWarnings({"rawtypes","unchecked"})
//以下为扩容过程的重点
Node<K,V>[] newTab = (Node<K,V>[])new Node[newCap];
table = newTab;
if (oldTab != null) {
for (int j = 0; j < oldCap; ++j) {
Node<K,V> e;
if ((e = oldTab[j]) != null) {
//将原哈希桶置空,以便GC
oldTab[j] = null;
//当前节点不是以链表形式存在,直接计算其应放置的新位置
if (e.next == null)
newTab[e.hash & (newCap - 1)] = e;
//当前节点是TreeNode
else if (e instanceof TreeNode)
((TreeNode<K,V>)e).split(this, newTab, j, oldCap);
else { // preserve order
//节点以链表形式存储
Node<K,V> loHead = null, loTail = null;
Node<K,V> hiHead = null, hiTail = null;
Node<K,V> next;
do {
next = e.next;
//原索引
if ((e.hash & oldCap) == 0) {
if (loTail == null)
loHead = e;
else
loTail.next = e;
loTail = e;
}
//原索引 + oldCap
else {
if (hiTail == null)
hiHead = e;
else
hiTail.next = e;
hiTail = e;
}
} while ((e = next) != null);
if (loTail != null) {
loTail.next = null;
newTab[j] = loHead;
}
if (hiTail != null) {
hiTail.next = null;
newTab[j + oldCap] = hiHead;
}
}
}
}
}
return newTab;
复制代码
}
复制代码
因为哈希表使用2次幂的拓展(指长度拓展为原来的2倍),所以在扩容的时候,元素的位置要么在原位置,要么在原位置再移动2次幂的位置。为什么是这么一个规律呢?我们假设 n 为 table 的长度,图(a)表示扩容前的key1和key2两种key确定索引位置的示例,图(b)表示扩容后key1和key2两种key确定索引位置的示例,其中hash1是key1对应的哈希与高位运算结果。
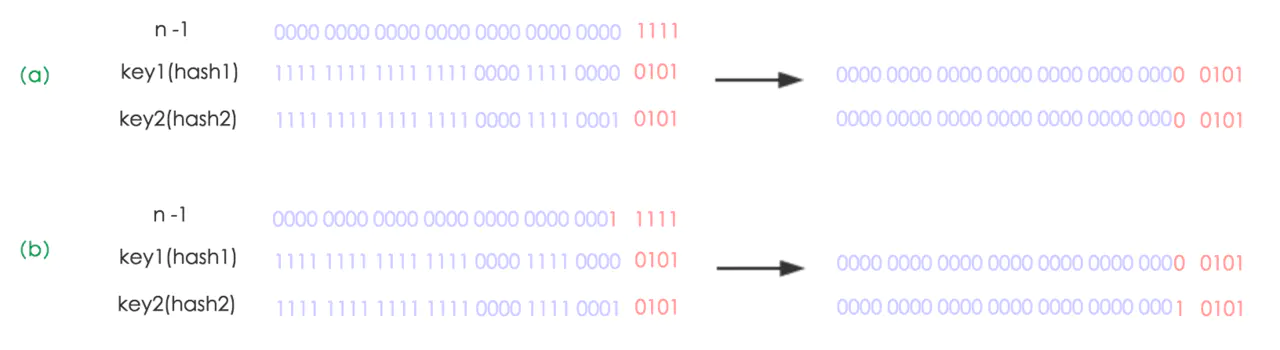
元素在重新计算hash之后,因为n变为2倍,那么n-1的mask范围在高位多1bit(红色),因此新的index就会发生这样的变化:
# 1200页Java架构面试专题及答案
小编整理不易,对这份**1200页Java架构面试专题及答案**感兴趣劳烦帮忙**转发/点赞一下,[然后点击这里即可免费领取!](https://gitee.com/vip204888/java-p7)**
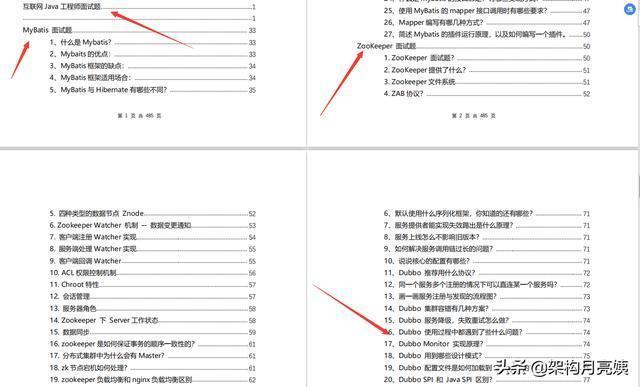
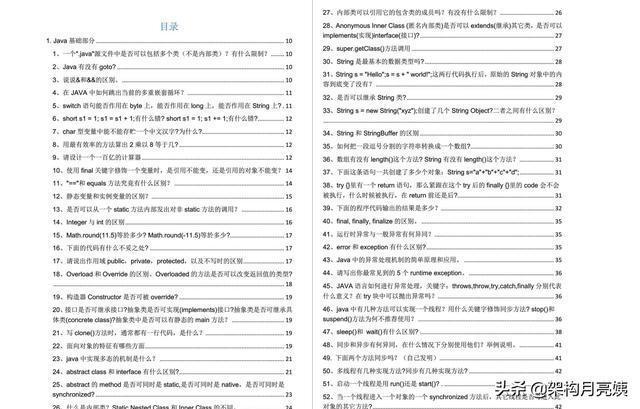
# 百度、字节、美团等大厂常见面试题
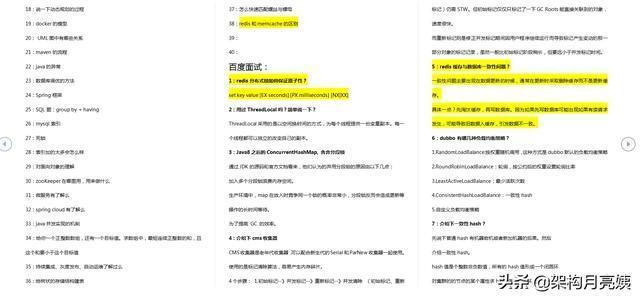
复制代码
评论