Android ExpandableListView 折叠菜单的三层嵌套实现
先说下,因为是三级嵌套,所以需要四个布局文件,Activity 页面本身需要一个布局文件,然后就是三级嵌套的三个布局文件。
Activity 布局文件
<?xml version="1.0" encoding="utf-8"?><LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"android:orientation="vertical" android:layout_width="match_parent"android:layout_height="match_parent"><ExpandableListViewandroid:id="@+id/expand_view"android:layout_width="match_parent"android:layout_height="match_parent"android:cacheColorHint="#00000000"android:childIndicator="@color/white"android:divider="@null"android:fadeScrollbars="false"android:groupIndicator="@null"android:listSelector="#00000000"android:scrollbars="none" /></LinearLayout>
我们可以通过 ExpandableListView 的默认属性来控制部分样式,这里贴上菜鸟教程的属性图片
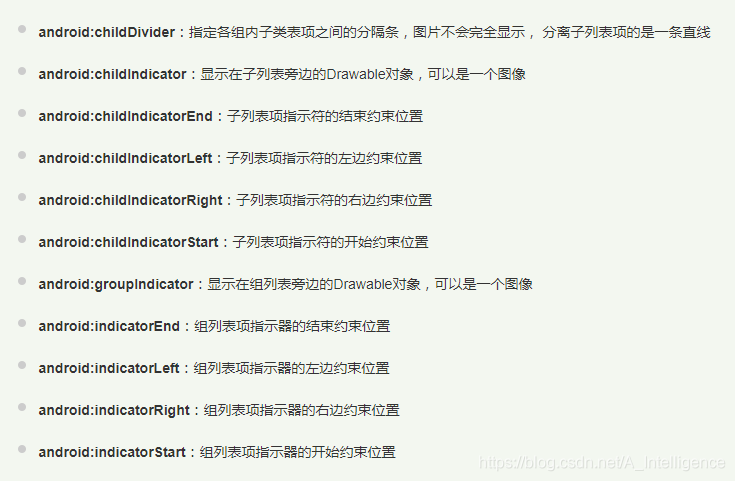
一级菜单布局文件
<?xml version="1.0" encoding="utf-8"?><androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"android:layout_width="match_parent"android:layout_height="44dp"xmlns:app="http://schemas.android.com/apk/res-auto"android:background="@drawable/chapter_gradient_group">
<TextViewandroid:id="@+id/adapter_title"android:layout_width="0dp"android:layout_height="match_parent"app:layout_constraintStart_toStartOf="parent"app:layout_constraintTop_toTopOf="parent"app:layout_constraintBottom_toBottomOf="parent"app:layout_constraintEnd_toEndOf="parent"android:layout_marginHorizontal="10dp"android:paddingStart="20dp"android:singleLine="true"android:ellipsize="end"android:text="@string/groupName"android:textColor="@color/white"android:textSize="16sp"android:gravity="start|center_vertical" />
</androidx.constraintlayout.widget.ConstraintLayout>
二级菜单布局文件
<?xml version="1.0" encoding="utf-8"?><androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"xmlns:app="http://schemas.android.com/apk/res-auto"android:layout_width="match_parent"android:layout_height="match_parent"android:background="@drawable/chapter_gradient_child">
<TextViewandroid:id="@+id/adapter_child_title"android:layout_width="match_parent"android:layout_height="40dp"android:ellipsize="end"android:gravity="start|center_vertical"android:paddingStart="30dp"android:paddingEnd="10dp"android:singleLine="true"android:text="@string/childName"android:textColor="@color/white"app:layout_constraintEnd_toEndOf="parent"app:layout_constraintStart_toStartOf="parent"app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
三级菜单布局文件
<?xml version="1.0" encoding="utf-8"?><androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"xmlns:app="http://schemas.android.com/apk/res-auto"android:layout_width="match_parent"android:layout_height="match_parent"android:background="@drawable/chapter_gradient_grandson">
<TextViewandroid:id="@+id/adapter_grandson_title"android:layout_width="match_parent"android:layout_height="40dp"android:ellipsize="end"android:gravity="start|center_vertical"android:paddingStart="40dp"android:paddingEnd="10dp"android:singleLine="true"android:text="@string/grandsonName"app:layout_constraintEnd_toEndOf="parent"app:layout_constraintStart_toStartOf="parent"app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Adapter
上面说过 ExpandableListView 继承自 ListView,所以我们需要 Adapter,三级嵌套,我们需要两个 Adapter。
这里有必要说一下,为什么是两个 Adapter,ExpandableListView 的 Adapter 继承自 BaseExpandableListAdapter。需要重写 getGroupView 和 getChildView。这两个方法中的 view 分别 inflate 父级菜单的布局和子级菜单的布局文件。
所以我们上面的三个级别的菜单布局文件通过两个 Adapter 来连接。分别是一级菜单的 Adapter 和三级菜单的 Adapter。
下面给出这两个 Adapter 的详细说明,需要注意的地方已经进行备注,请仔细看备注
一级菜单 Adapter
最值得注意的是该 Adapter 的 getChildView 方法和 getChildrenCount。因为有些数据不包含三级菜单,有些包含了三级菜单。另外,这个地方需要对下级嵌套的 ExpandableListView 进行处理。
/**
三级折叠菜单的一级 Adapter
@author StarryRivers*/public class ChapterExpandableAdapter extends BaseExpandableListAdapter {
...
@Overridepublic int getGroupCount() {// 父菜单长度 return fatherChapterList.size();}
@Overridepublic int getChildrenCount(int groupPosition) {// 子菜单长度,嵌套所以返回只能 1return 1;}
@Overridepublic View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {GroupViewHolder groupHolder;// 尽可能重用旧 view 处理 if (convertView == null) {convertView = LayoutInflater.from(parent.getContext()).inflate(R.layout.adapter_expandable_group_view, parent
, false);groupHolder = new GroupViewHolder();groupHolder.groupTitle = convertView.findViewById(R.id.adapter_title);convertView.setTag(groupHolder);} else {groupHolder = (GroupViewHolder) convertView.getTag();}// 设置 titlegroupHolder.groupTitle.setText(fatherChapterList.get(groupPosition).getName());return convertView;}
@Overridepublic View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {if (convertView == null) {convertView = new CustomExpandableListView(context);}CustomExpandableListView expandableListView = (CustomExpandableListView) convertView;// 加载子级 AdapterChapterExpandableLowAdapter lowAdapter = new ChapterExpandableLowAdapter(context);lowAdapter.setTotalList(fatherChapterList.get(groupPosition).getSec());expandableListView.setAdapter(lowAdapter);
if (fatherChapterList.get(groupPosition).getSec().get(childPosition).getThird().size() == 0) {expandableListView.setGroupIndicator(null);}// 本身的父级,相当于三级目录的子级监听 expandableListView.setOnGroupClickListener((parent12, v, groupPosition12, id) -> {// 如果第三层 size 为 0,意味着没有三级菜单 if (fatherChapterList != null && fatherChapterList.size() > 0 && fatherChapterList.get(groupPosition).getSec().get(groupPosition12).getThird().size() == 0) {// TODO 业务处理}// 存在第三级数据,事件分发机制继续想下传递 return false;});expandableListView.setOnChildClickListener((parent1, v, groupPosition1, childPosition1, id) -> {// 三级菜单的业务处理 return true;});return expandableListView;}
/**
子列表是否可选,如果为 false,则子项不能触发点击事件,默认为 false
@param groupPosition groupPosition
@param childPosition childPosition
@return result*/@Overridepublic boolean isChildSelectable(int groupPosition, int childPosition) {return true;}
/**
父级菜单的 ViewHolder*/static class GroupViewHolder {TextView groupTitle;}
评论