leetcode 232. Implement Queue using Stacks 用栈实现队列 (简单)
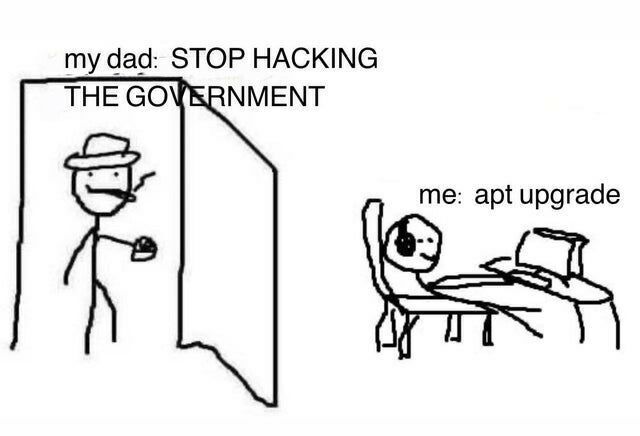
一、题目大意
标签: 栈和队列
https://leetcode.cn/problems/implement-queue-using-stacks
请你仅使用两个栈实现先入先出队列。队列应当支持一般队列支持的所有操作(push、pop、peek、empty):
实现 MyQueue 类:
void push(int x) 将元素 x 推到队列的末尾 int pop() 从队列的开头移除并返回元素 int peek() 返回队列开头的元素 boolean empty() 如果队列为空,返回 true ;否则,返回 false 说明:
你 只能 使用标准的栈操作 —— 也就是只有 push to top, peek/pop from top, size, 和 is empty 操作是合法的。你所使用的语言也许不支持栈。你可以使用 list 或者 deque(双端队列)来模拟一个栈,只要是标准的栈操作即可。
示例 1:
输入:["MyQueue", "push", "push", "peek", "pop", "empty"][[], [1], [2], [], [], []]输出:[null, null, null, 1, 1, false]
解释:
提示:
1 <= x <= 9
最多调用 100 次 push、pop、peek 和 empty
假设所有操作都是有效的 (例如,一个空的队列不会调用 pop 或者 peek 操作)
进阶:你能否实现每个操作均摊时间复杂度为 O(1) 的队列?换句话说,执行 n 个操作的总时间复杂度为 O(n) ,即使其中一个操作可能花费较长时间。
二、解题思路
用两个栈来实现一个队列:因为需要得到先入先出的结果,所以必定要通过一个额外栈翻一次数组。这个翻转过程既可以在插入时完成,也可以在取值时完成。
下面解决在插入时完成翻转过程。
三、解题方法
3.1 Java 实现
四、总结小记
2022/8/7 周末也要刷一题
版权声明: 本文为 InfoQ 作者【okokabcd】的原创文章。
原文链接:【http://xie.infoq.cn/article/d526eaf1662bd0eea1821bcca】。文章转载请联系作者。
评论