C 语言 _ 文件操作相关练习题
作者:DS小龙哥
- 2022 年 5 月 16 日
本文字数:3928 字
阅读完需:约 13 分钟
当前文章列出了 4 个文件编程相关的练习题。文件拷贝实现、 文件加密、学生管理系统链表模板(未添加文件操作)、学生管理系统模板(通过文件系统保存信息)、等 4 个例子。
1.1 文件拷贝实现
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
/*1. 打开源文件*/
FILE *file_src;
FILE *file_new;
unsigned char buff[1024];
unsigned int cnt;
file_src=fopen("D:/123.pdf","rb");
if(file_src==NULL)
{
printf("源文件打开失败!\n");
return -1;
}
/*2. 创建新文件*/
file_new=fopen("D:/456.pdf","wb");
if(file_new==NULL)
{
printf("新文件创建失败!\n");
return -1;
}
/*3. 拷贝文件*/
while(!feof(file_src))
{
cnt=fread(buff,1,1024,file_src);
fwrite(buff,1,cnt,file_new);
}
/*4. 关闭文件*/
fclose(file_new);
fclose(file_src);
printf("文件拷贝成功!\n");
return 0;
}
复制代码
1.2 文件加密
使用: 异或 ^密码类型: (1) 整型密码 (2) 字符串密码比如: 银行提款机的密码、QQ 密码加密代码:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
/*1. 打开源文件*/
FILE *file_src;
FILE *file_new;
unsigned char buff[1024];
unsigned int cnt;
unsigned int password=123456; //密码数据
unsigned int data; //存放读取的数据
file_src=fopen("D:/123.pdf","rb");
if(file_src==NULL)
{
printf("源文件打开失败!\n");
return -1;
}
/*2. 创建新文件*/
file_new=fopen("D:/456.pdf","wb");
if(file_new==NULL)
{
printf("新文件创建失败!\n");
return -1;
}
/*3. 文件加密*/
while(!feof(file_src))
{
cnt=fread(&data,1,4,file_src);
data=data^password;//文件数据加密
fwrite(&data,1,cnt,file_new);
}
/*4. 关闭文件*/
fclose(file_new);
fclose(file_src);
printf("文件加密成功!\n");
return 0;
}
复制代码
解密代码:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
/*1. 打开源文件*/
FILE *file_src;
FILE *file_new;
unsigned char buff[1024];
unsigned int cnt;
unsigned int password=123456; //密码数据
unsigned int data; //存放读取的数据
file_src=fopen("D:/456.pdf","rb");
if(file_src==NULL)
{
printf("源文件打开失败!\n");
return -1;
}
/*2. 创建新文件*/
file_new=fopen("D:/789.pdf","wb");
if(file_new==NULL)
{
printf("新文件创建失败!\n");
return -1;
}
/*3. 文件加密*/
while(!feof(file_src))
{
cnt=fread(&data,1,4,file_src);
data=data^password;//文件数据加密
fwrite(&data,1,cnt,file_new);
}
/*4. 关闭文件*/
fclose(file_new);
fclose(file_src);
printf("文件解密成功!\n");
return 0;
}
复制代码
1.3 学生管理系统链表模板(未添加文件操作)
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
//存放信息的结构体
struct MyStruct
{
char Name[50]; //存放姓名
int Number; //存放编号
struct MyStruct *next; //存放下一个节点的地址
};
//链表相关的函数接口
struct MyStruct *ListHead=NULL; //链表头
struct MyStruct *CreateListHead(struct MyStruct *head);
void AddrListInfo(struct MyStruct *head,struct MyStruct data);
void DeleteListInfo(struct MyStruct *head,int number);
void PrintListAllInfo(struct MyStruct *head);
int main()
{
struct MyStruct data1={"张三",123};
struct MyStruct data2={"李四",456};
struct MyStruct data3={"小王",789};
ListHead=CreateListHead(ListHead);
//添加信息
AddrListInfo(ListHead,data1);
AddrListInfo(ListHead,data2);
AddrListInfo(ListHead,data3);
//删除节点
DeleteListInfo(ListHead,123);
DeleteListInfo(ListHead,789);
//打印
PrintListAllInfo(ListHead);
return 0;
}
/*
函数功能: 创建链表头
*/
struct MyStruct *CreateListHead(struct MyStruct *head)
{
if(head==NULL)
{
head=malloc(sizeof(struct MyStruct));
head->next=NULL;
}
return head;
}
/*
函数功能: 在链表结尾添加节点
*/
void AddrListInfo(struct MyStruct *head,struct MyStruct data)
{
struct MyStruct *p=head;
struct MyStruct *tmp=NULL;
while(p->next!=NULL)
{
p=p->next;
}
tmp=malloc(sizeof(struct MyStruct));
memcpy(tmp,&data,sizeof(struct MyStruct));
p->next=tmp;
tmp->next=NULL;
}
/*
函数功能: 根据结构体里特有的成员区分进行删除链表节点信息
函数参数: int numbe 编号
*/
void DeleteListInfo(struct MyStruct *head,int number)
{
struct MyStruct *p=head;
struct MyStruct *tmp=NULL;
while(p->next!=NULL)
{
tmp=p; //保存上一个节点的信息
p=p->next;
if(p->Number==number)
{
tmp->next=tmp->next->next;
free(p);
p=head; //链表头归位
}
}
}
/*
函数功能: 打印所有节点信息
函数参数: int numbe 编号
*/
void PrintListAllInfo(struct MyStruct *head)
{
struct MyStruct *p=head;
int cnt=0;
printf("\n链表全部信息如下:\n");
while(p->next!=NULL)
{
p=p->next;
cnt++;
printf("第%d个节点信息: %s,%d\n",cnt,p->Name,p->Number);
}
}
复制代码
1.4 学生管理系统模板(通过文件系统保存信息)
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
//存放信息的结构体
struct MyStruct
{
char Name[50]; //存放姓名
int Number; //存放编号
struct MyStruct *next; //存放下一个节点的地址
};
//链表相关的函数接口
struct MyStruct *ListHead=NULL; //链表头
struct MyStruct *CreateListHead(struct MyStruct *head);
void AddrListInfo(struct MyStruct *head,struct MyStruct data);
void DeleteListInfo(struct MyStruct *head,int number);
void PrintListAllInfo(struct MyStruct *head);
//文件操作相关函数
void SaveListAllInfo(struct MyStruct *head,char *path);
void GetListAllInfo(struct MyStruct *head,char *path);
#if 0
int main()
{
struct MyStruct data1={"张三",123};
struct MyStruct data2={"李四",456};
struct MyStruct data3={"小王",789};
ListHead=CreateListHead(ListHead);
AddrListInfo(ListHead,data1);
AddrListInfo(ListHead,data2);
AddrListInfo(ListHead,data3);
//保存节点信息
SaveListAllInfo(ListHead,"D:/list.ini");
//打印
PrintListAllInfo(ListHead);
return 0;
}
#endif
#if 1
int main()
{
ListHead=CreateListHead(ListHead);
//获取节点信息
GetListAllInfo(ListHead,"D:/list.ini");
//打印
PrintListAllInfo(ListHead);
return 0;
}
#endif
/*
函数功能: 创建链表头
*/
struct MyStruct *CreateListHead(struct MyStruct *head)
{
if(head==NULL)
{
head=malloc(sizeof(struct MyStruct));
head->next=NULL;
}
return head;
}
/*
函数功能: 在链表结尾添加节点
*/
void AddrListInfo(struct MyStruct *head,struct MyStruct data)
{
struct MyStruct *p=head;
struct MyStruct *tmp=NULL;
while(p->next!=NULL)
{
p=p->next;
}
tmp=malloc(sizeof(struct MyStruct));
memcpy(tmp,&data,sizeof(struct MyStruct));
p->next=tmp;
tmp->next=NULL;
}
/*
函数功能: 根据结构体里特有的成员区分进行删除链表节点信息
函数参数: int numbe 编号
*/
void DeleteListInfo(struct MyStruct *head,int number)
{
struct MyStruct *p=head;
struct MyStruct *tmp=NULL;
while(p->next!=NULL)
{
tmp=p; //保存上一个节点的信息
p=p->next;
if(p->Number==number)
{
tmp->next=tmp->next->next;
free(p);
p=head; //链表头归位
}
}
}
/*
函数功能: 打印所有节点信息
函数参数: int numbe 编号
*/
void PrintListAllInfo(struct MyStruct *head)
{
struct MyStruct *p=head;
int cnt=0;
printf("\n链表全部信息如下:\n");
while(p->next!=NULL)
{
p=p->next;
cnt++;
printf("第%d个节点信息: %s,%d\n",cnt,p->Name,p->Number);
}
}
/*
函数功能: 保存链表节点信息
*/
void SaveListAllInfo(struct MyStruct *head,char *path)
{
struct MyStruct *p=head;
FILE *file;
file=fopen(path,"a+b");
if(file==NULL)
{
printf("保存信息的文件打开失败!\n");
return ;
}
while(p->next!=NULL)
{
p=p->next;
fwrite(p,1,sizeof(struct MyStruct),file);
}
fclose(file);
}
/*
函数功能: 从文件里获取链表节点信息
*/
void GetListAllInfo(struct MyStruct *head,char *path)
{
struct MyStruct *p=head;
FILE *file;
struct MyStruct data;
file=fopen(path,"rb");
if(file==NULL)
{
printf("保存信息的文件打开失败!\n");
return;
}
//循环读取文件里的数据
while(!feof(file))
{
fread(&data,1,sizeof(struct MyStruct),file); //读取链表节点数据
AddrListInfo(head,data); //添加链表节点
}
fclose(file);
}
复制代码
划线
评论
复制
发布于: 刚刚阅读数: 2
版权声明: 本文为 InfoQ 作者【DS小龙哥】的原创文章。
原文链接:【http://xie.infoq.cn/article/d190b2c3f7f790ef7798d15ce】。文章转载请联系作者。
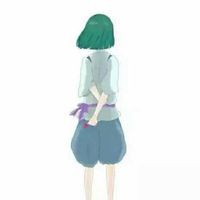
DS小龙哥
关注
之所以觉得累,是因为说的比做的多。 2022.01.06 加入
熟悉C/C++、51单片机、STM32、Linux应用开发、Linux驱动开发、音视频开发、QT开发. 目前已经完成的项目涉及音视频、物联网、智能家居、工业控制领域
评论