jackson 学习之九:springboot 整合 (配置文件)
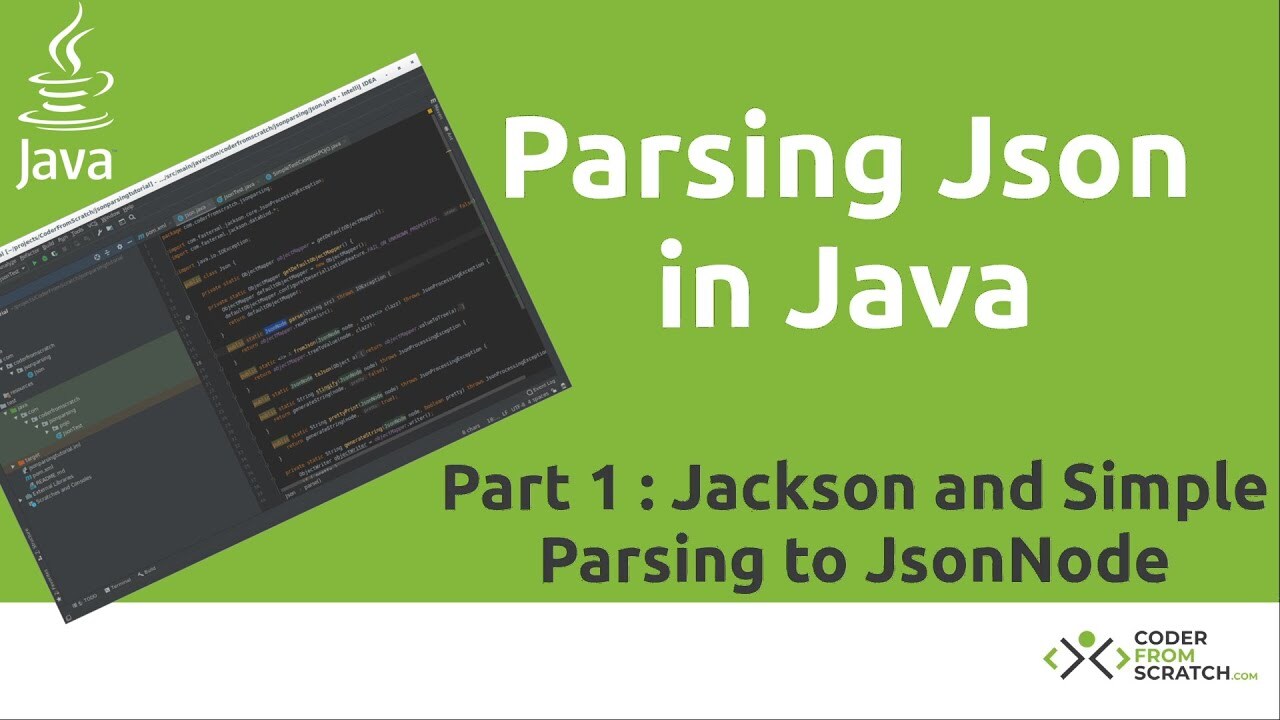
欢迎访问我的 GitHub
这里分类和汇总了欣宸的全部原创(含配套源码):https://github.com/zq2599/blog_demos
关于 springboot 整合 jackson
本文是《jackson 学习》系列的第九篇,学习如何在 springboot 项目中使用 jackson,以 springboot-2.3.3 版本为例,jackson 是 springboot 的默认 json 处理工具,如下图红框所示,jackson 在 maven 配置中被 spring-boot-starter-web 间接依赖,可直接使用:
在 springboot 项目中常用的配置方式有两种:
用 properties 或 yml 配置文件来配置,即本篇的内容;
用配置类来配置,这是下一篇文章的主题;
本篇概览
今天实战内容如下:
开发 springboot 应用,体验 springboot 默认支持 jackson,包括 jackson 注解和 ObjectMapper 实例的注入;
在 application.yml 中添加 jackson 配置,验证是否生效;
源码下载
如果您不想编码,可以在 GitHub 下载所有源码,地址和链接信息如下表所示(https://github.com/zq2599/blog_demos):
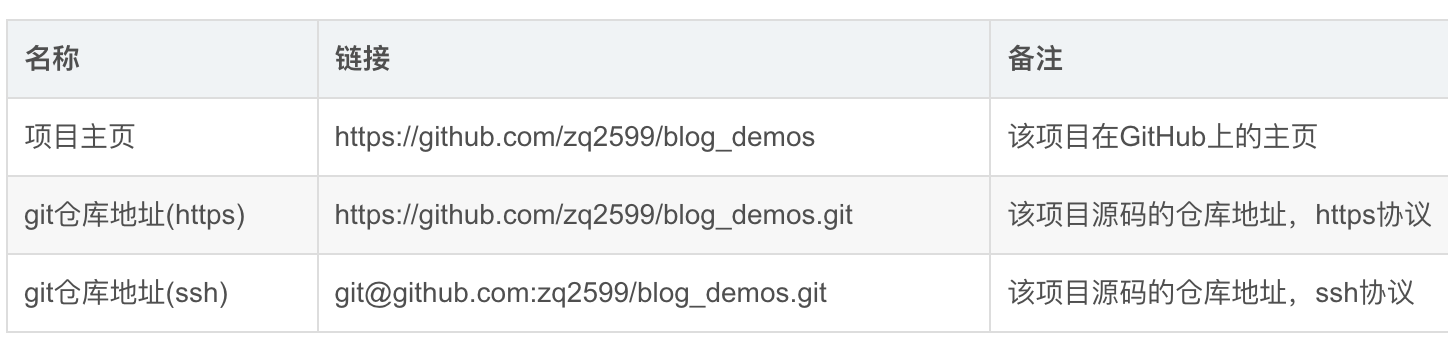
这个 git 项目中有多个文件夹,本章的应用在 jacksondemo 文件夹下,如下图红框所示:
jacksondemo 是父子结构的工程,本篇的代码在 springbootproperties 子工程中,如下图:
开始实战
由于同属于《jackson 学习》系列文章,因此本篇的 springboot 工程作为 jacksondemo 的子工程存在,pom.xml 如下,需要注意的是 parent 不能使用 spring-boot-starter-parent,而是通过 dependencyManagement 节点来引入 springboot 依赖:
启动类很平常:
由于用到了 swagger,因此要添加 swagger 配置:
序列化和反序列化用到的 Bean 类,可见使用了 JsonProperty 属性来设置序列化和反序列化时的 json 属性名,field0 字段刻意没有 get 方法,是为了验证 JsonProperty 的序列化能力:
测试用的 Controller 代码如下,很简单只有两个接口,serialization 返回序列化结果,deserialization 接受客户端请求参数,反序列化成实例,通过 toString()来检查反序列化的结果,另外,还通过 Autowired 注解从 spring 容器中将 ObjectMapper 实例直接拿来用:
验证(不用配置文件)
先来看看没有配置文件时,默认的 jackson 配置的表现,直接在 IDEA 上运行 SpringbootpropertiesApplication;
浏览器访问 http://localhost:8080/swagger-ui.html ,如下图红框 1,json_field0 和 json_field1 都是 JsonProperty 注释,出现在了 swagger 的 model 中,这证明 jackson 注解已经生效:
点击上图的红框 2,看看 springboot 引用返回的序列化结果,如下图:
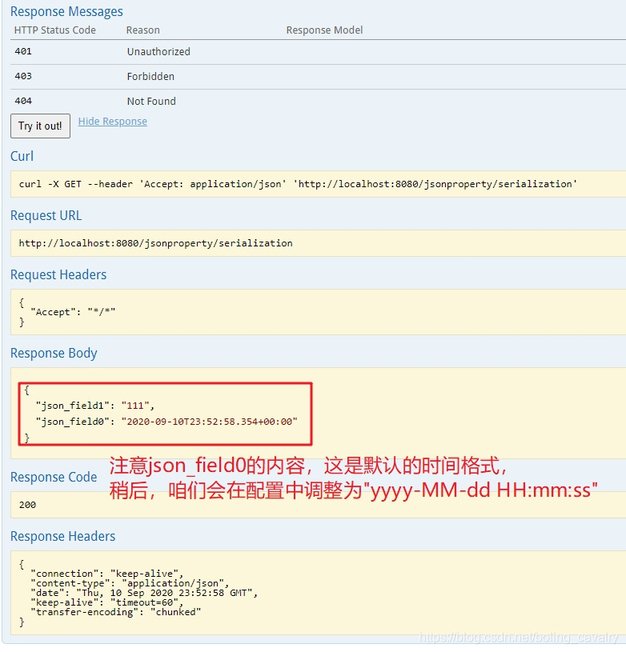
另外,上述红框中的 json 格式,每个属性单独一行,像是做了格式化调整的,这是 springboot 做的?还是 swagger 展示的时候做的?用浏览器访问 http://localhost:8080/jsonproperty/serialization ,结果如下,可见 springboot 返回的是未经过格式化的 json:
接下来咱们添加 jackson 相关的配置信息并验证是否生效;
添加配置文件并验证
在 resources 目录新增 application.yml 文件,内容如下:
将鼠标放置下图红框位置,再按住 Ctlr 键,IDEA 会弹出一个浮层,提示该配置对应的 jackson 代码,如下图:
在上图中,按住 Ctlr 键,用鼠标点击红框位置即可打开此配置对应的 jackson 源码,如下图:
重新运行 springboot 应用,用浏览器访问:http://localhost:8080/jsonproperty/serialization ,结果如下图,可见 json_field0 的格式变成了 yyyy-MM-dd HH:mm:ss,而且 json 输出也做了格式化,证明 application.yml 中的配置已经生效:
再来试试反序列化,打开 swagger 页面,操作和响应如下图所示,注意红框 1 里面请求参数的格式:
- 至此,在 springboot 中通过 yml 配置 jackson 的操作实战就完成了,接下来的章节,咱们在配置类中用代码来完成 yml 的配置;
欢迎关注 InfoQ:程序员欣宸
版权声明: 本文为 InfoQ 作者【程序员欣宸】的原创文章。
原文链接:【http://xie.infoq.cn/article/cf2083c2b6802b372e1ecb9b2】。文章转载请联系作者。
评论