SpringBoot+WebSocket 实时监控异常
项目结构是这样子的,后面的代码关键注释都有,就不重复描述了
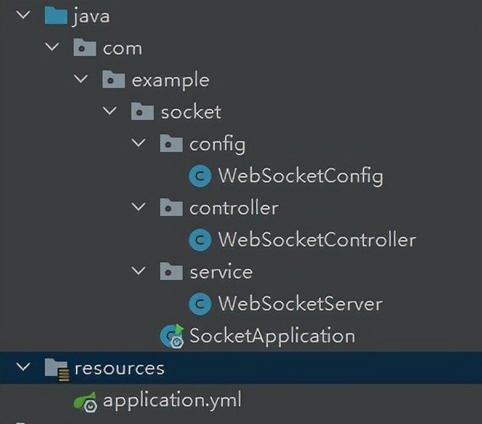
1、新建 SpringBoot 工程,选择 web 和 WebSocket 依赖
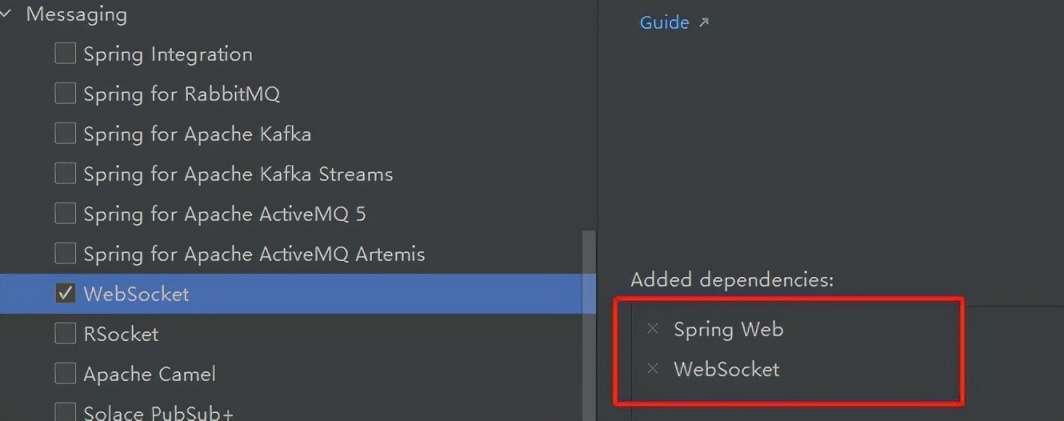
2、配置 application.yml
1 <!DOCTYPE html>
2 <html>
3 <head>
4 <meta charset="utf-8" />
5 <title>实时监控</title>
6 </head>
7 <style>
8 .item {
9 display: flex;
10 border-bottom: 1px solid #000000;
11 justify-content: space-between;
12 width: 30%;
13 line-height: 50px;
14 height: 50px;
15 }
16
17 .item span:nth-child(2){
18 margin-right: 10px;
19 margin-top: 15px;
20 width: 20px;
21 height: 20px;
22 border-radius: 50%;
23 background: #55ff00;
24 }
25 .nowI{
26 background: #ff0000 !important;
27 }
28 </style>
29 <body>
30 <div id="app">
31 <div v-for="item in list" class="item">
32 <span>{{item.id}}.{{item.name}}</span>
33 <span :class='item.state==-1?"nowI":""'></span>
34 </div>
35 </div>
36 </body>
37 <script src="./js/vue.min.js"></script>
38 <script type="text/javascript">
39 var vm = new Vue({
40 el: "#app",
41 data: {
42 list: [{
43 id: 1,
44 name: '张三',
45 state: 1
46 },
47 {
48 id: 2,
49 name: '李四',
50 state: 1
51 },
52 {
53 id: 3,
54 name: '王五',
55 state: 1
56 },
57 {
58 id: 4,
59 name: '韩梅梅',
60 state: 1
61 },
62 {
63 id: 5,
64 name: '李磊',
65 state: 1
66 },
67 ]
68 }
69 })
70
71 var webSocket = null;
72 if ('WebSocket' in window) {
73 //创建 WebSocket 对象
74 webSocket = new WebSocket("ws://localhost:18801/webSocket/" + getUUID());
75
76 //连接成功
77 webSocket.onopen = function() {
78 console.log("已连接");
79 webSocket.send("消息发送测试")
80 }
81 //接收到消息
82 webSocket.onmessage = function(msg) {
83 //处理消息
84 var server Java 开源项目【ali1024.coding.net/public/P7/Java/git】 Msg = msg.data;
85 var t_id = parseInt(serverMsg) //服务端发过来的消息,ID,string 需转化为 int 类型才能比较
86 for (var i = 0; i < vm.list.length; i++) {
87 var item = vm.list[i];
88 if(item.id == t_id){
89 item.state = -1;
90 vm.list.splice(i,1,item)
91 break;
92 }
93 }
94 };
95
96 //关闭事件
97 webSocket.onclose = function() {
98 console.log("websocket 已关闭");
99 };
100 //发生了错误事件
101 webSocket.onerror = function() {
102 console.log("websocket 发生了错误");
103 }
104 } else {
105 alert("很遗憾,您的浏览器不支持 WebSocket!")
106 }
107
108 function getUUID() { //获取唯一的 UUID
109 return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) {
110
111 var r = Math.random() * 16 | 0,
112 v = c == 'x' ? r : (r & 0x3 | 0x8);
113 return v.toString(16);
114 });
115 }
116 </script>
117 </html>
3、WebSocketConfig 配置类
1 @Configuration
2 public class WebSocketConfig {
3
4 /**
5 * 注入一个 ServerEndpointExporter,该 Bean 会自动注册使用 @ServerEndpoint 注解申明的 websocket endpoint
6 */
7 @Bean
8 public ServerEndpointExporter serverEndpointExporter(){
9 return new ServerEndpointExporter();
10 }
11 }
4、WebSocketServer 类,用来进行服务端和客户端之间的交互
1 /**
2 * @author jae
3 * @ServerEndpoint("/webSocket/{uid}") 前端通过此 URI 与后端建立链接
4 */
5
6 @ServerEndpoint("/webSocket/{uid}")
7 @Component
8 public class WebSocketServer {
9
10 private static Logger log = LoggerFactory.getLogger(WebSocketServer.class);
11
12 //静态变量,用来记录当前在线连接数。应该把它设计成线程安全的。
13 private static final AtomicInteger onlineNum = new AtomicInteger(0);
14
15 //concurrent 包的线程安全 Set,用来存放每个客户端对应的 WebSocketServer 对象。
16 private static CopyOnWriteArraySet<Session> sessionPools = new CopyOnWriteArraySet<Session>();
17
18 /**
19 * 有客户端连接成功
20 */
21 @OnOpen
22 public void onOpen(Session session, @PathParam(value = "uid") String uid){
23 sessionPools.add(session);
24 onlineNum.incrementAndGet();
25 log.info(uid + "加入 webSocket!当前人数为" + onlineNum);
26 }
27
28 /**
29 * 连接关闭调用的方法
30 */
31 @OnClose
32 public void onClose(Session session) {
33 sessionPools.remove(session);
34 int cnt = onlineNum.decrementAndGet();
35 log.info("有连接关闭,当前连接数为:{}", cnt);
36 }
37
38 /**
39 * 发送消息
40 */
41 public void sendMessage(Session session, String message) throws IOException {
42 if(session != null){
43 synchronized (session) {
44 session.getBasicRemote().sendText(message);
45 }
46 }
47 }
48
49 /**
50 * 群发消息
51 《一线大厂 Java 面试题解析+后端开发学习笔记+最新架构讲解视频+实战项目源码讲义》开源 */
52 public void broadCastInfo(String message) throws IOException {
53 for (Session session : sessionPools) {
54 if(session.isOpen()){
55 sendMessage(session, message);
56 }
57 }
58 }
59
60 /**
61 * 发生错误
62 */
63 @OnError
64 public void onError(Session session, Throwable throwable){
65 log.error("发生错误");
写在最后
还有一份 JAVA 核心知识点整理(PDF):JVM,JAVA 集合,JAVA 多线程并发,JAVA 基础,Spring 原理,微服务,Netty 与 RPC,网络,日志,Zookeeper,Kafka,RabbitMQ,Hbase,MongoDB,Cassandra,设计模式,负载均衡,数据库,一致性哈希,JAVA 算法,数据结构,加密算法,分布式缓存,Hadoop,Spark,Storm,YARN,机器学习,云计算...
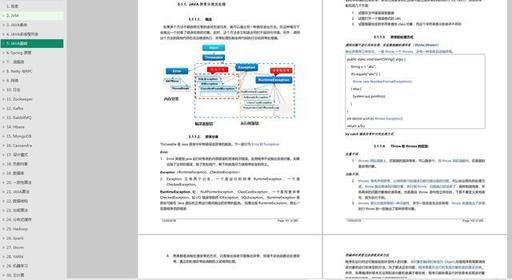
评论