Python OpenCV 对象检测,图像处理取经之旅第 37 篇
Python OpenCV 365 天学习计划,与橡皮擦一起进入图像领域吧。本篇博客是这个系列的第 37 篇。
基础知识铺垫
这篇文章需要配合上一篇一起观看,当然为了更好的学习效果,咱在一起复习一遍。
上篇博客重点学习了两个函数的用法,第一个就是 findContours
函数,用来检测轮廓,该函数的原型如下:
简单写一段代码测试一下:
输出结果如下,注意内容:
cv.findContours
边缘检测函数,返回只第一个是一个列表,其中每一项都是 numpy
中的数组,表示的就是边界值。列表有 1 个,那得到的就只有一个边界。接下来绘制出边缘,这里需要调整的内容有些多,具体代码如下。
其中涉及的输出内容如下:
通过 len(contours)
得到的数字是几,就表示几个边界。
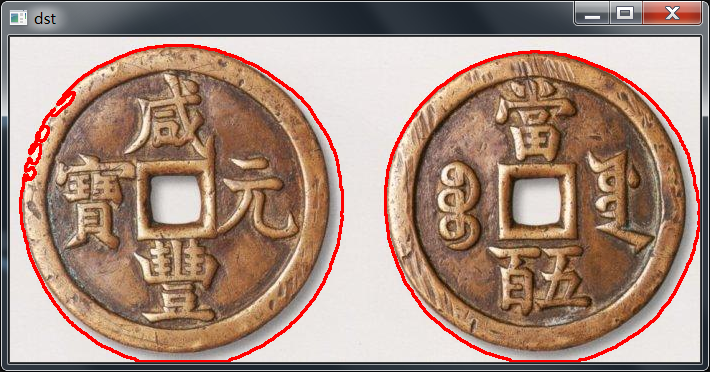
下面绘制轮廓的代码,你可以进行一下尝试。
contourIdx
是找到的轮廓索引,不可以超过轮廓总数,否则会出现如下 BUG。
继续修改,将最后一个参数修改为 -1
,得到的结果如下。
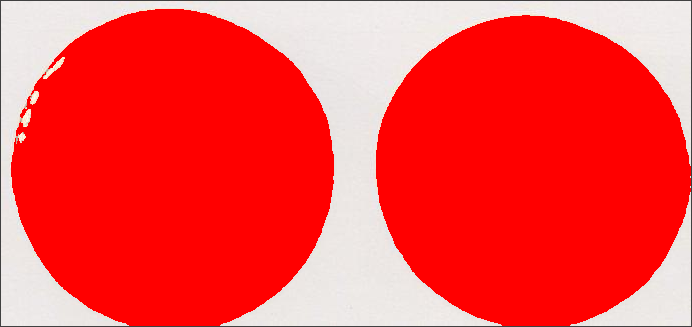
将阈值分割修改为边缘检测
上文我们是通过 cv2.threshold
函数实现的最终效果,接下来在通过 Canny
边缘检测进行一下上述操作。
在使用上述代码,如果想实现填充效果,比较难实现,因为很多路径并不是闭合的。参数调整的不是很理想,见谅。
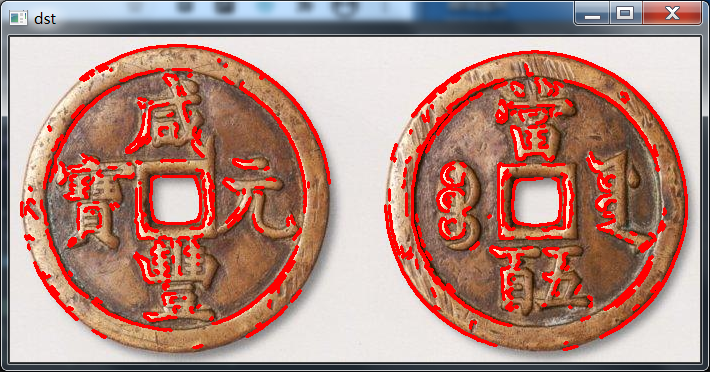
在调整参数的时候,还出现了下述情况,这个地方并未找到合理的解释。
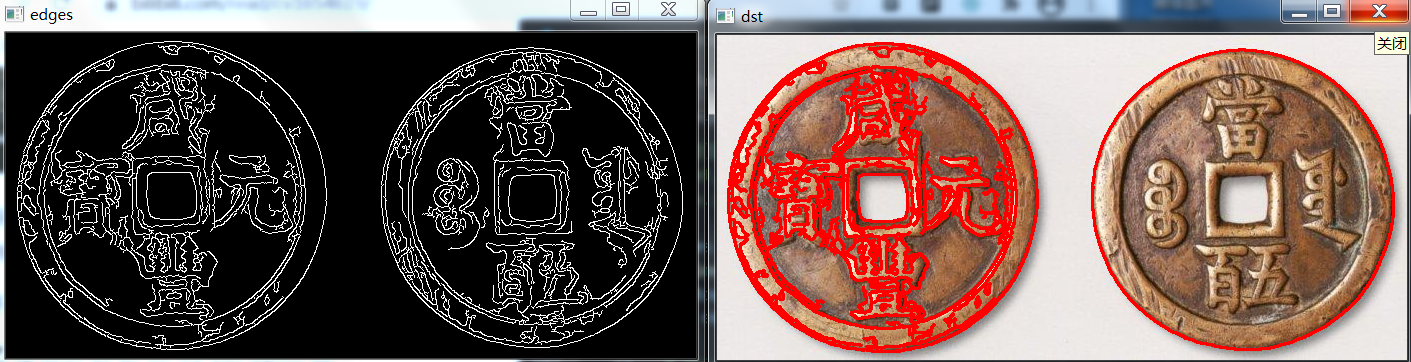
对象测量
获得轮廓之后,可以对轮廓进行一些几何特征的测量,包括 原点距
、中心距
、图像的重心坐标
,这些数学概念留到后续学习,先掌握应用层。
对象测量用到的函数是 cv2.moments
,该函数原型如下:
用途就是输入轮廓,返回一个字典,测试代码如下:
我随便选择一个作为说明,内容如下:
接下来就可以针对上述内容做相应的处理了。例如,求出轮廓的重心。
最常见的一个错误是,出现该错误增加一个非零的分支验证即可。
第二个错误是类型错误,这个我们在学习绘制圆形的时候了解过,具体如下。
修改代码之后的逻辑为:
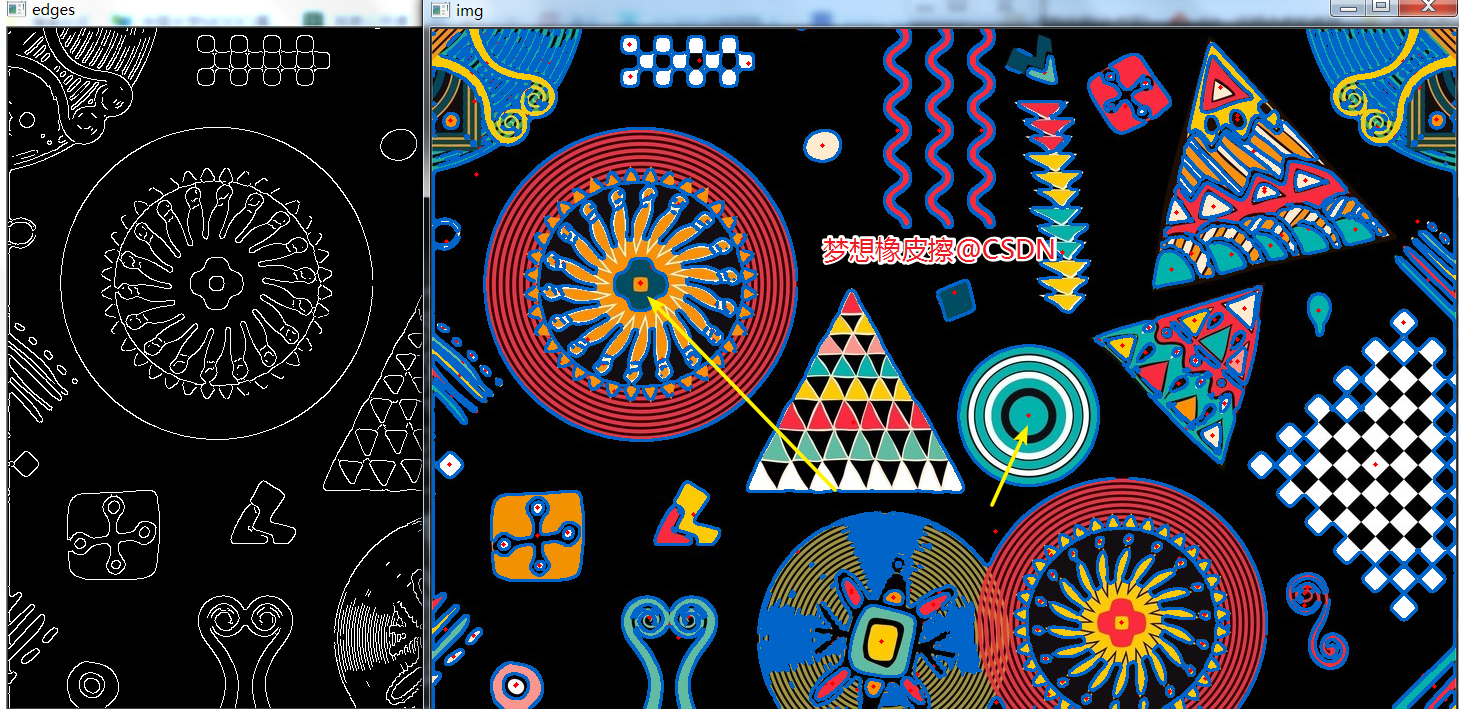
你还可以计算轮廓面积,使用的函数为 cv2.contourArea
。
曲线闭合的时候得的面积是轮廓包围的面积,如果轮廓不闭合或者边界有交叉,获取到的面积不在准确。
版权声明: 本文为 InfoQ 作者【梦想橡皮擦】的原创文章。
原文链接:【http://xie.infoq.cn/article/c61a7c1d9547813a319042879】。文章转载请联系作者。
评论