Go- 字符串、切片和数组的使用
发布于: 2021 年 08 月 26 日
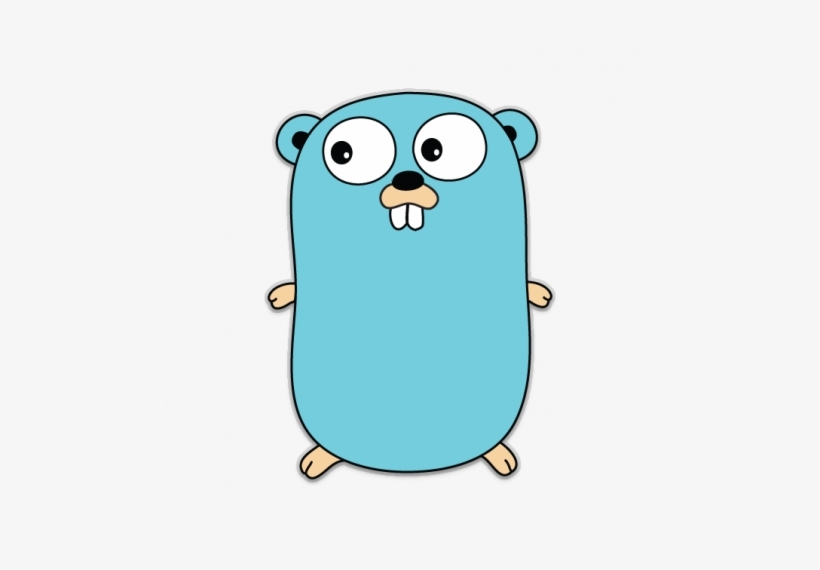
Go 学习笔记,学习内容《Go入门指南》
主要介绍以下内容:
for range 获得字符串元素
由字符串生成字节切片
修改字符串中的字符
字符串对比函数
append 函数常见操作
切片垃圾回收
代码示例可以直接运行
package main
import (
"fmt"
)
func printDivider(str ...string) {
fmt.Println()
fmt.Println("-----------------------------------------------")
if len(str) > 0 {
fmt.Println(str)
}
}
func main() {
/*
for range 获得字符串元素
*/
printDivider("for range 获得字符串元素")
s1 := "\u00ff\u754c" // unicode编码字符,unicode是可变长编码,一个unicode可能占用 2, 3, 4个字节
for ix, ch := range s1 {
fmt.Printf("%d-%c ", ix, ch) // 输出:0-ÿ 2-界
}
fmt.Printf("\n")
/*
由字符串生成字节切片
*/
printDivider("由字符串生成字节切片")
str := "Excellent"
for ix, ch := range str {
fmt.Printf("%d-%c ", ix, ch) // 输出:0-E 1-x 2-c 3-e 4-l 5-l 6-e 7-n 8-t
}
fmt.Printf("\n")
b := []byte(str)
for ix, ch := range b {
fmt.Printf("%d-%c ", ix, ch) // 输出:0-E 1-x 2-c 3-e 4-l 5-l 6-e 7-n 8-t
}
fmt.Printf("\nlen(b) = %d, cap(b) = %d\n", len(b), cap(b)) // 输出:len(b) = 9, cap(b) = 32
/*
修改字符串中的字符
*/
printDivider("修改字符串中的字符")
b[0] = '0'
b[1] = '1'
for ix, ch := range b {
fmt.Printf("%d-%c ", ix, ch) // 输出:0-0 1-1 2-c 3-e 4-l 5-l 6-e 7-n 8-t
}
fmt.Printf("\n")
fmt.Println(str) // 输出:Excellent
s2 := string(b)
fmt.Println(s2) // 输出:01cellent
/*
字符串对比函数
*/
printDivider("字符串对比函数")
res := Compare([]byte("abc"), []byte("abcd"))
fmt.Printf("\"abc\" compare \"abcd\": %d\n", res)
/*
append函数常见操作
*/
printDivider("append函数常见操作")
//s11 := [...]int{1, 2, 3} // 编译错误:first argument to append must be slice; have [3]int
//s11 := []int{1, 2, 3}[:] // OK
s11 := []int{1, 2, 3}
s11 = append(s11, 20, 30) // 追加元素
fmt.Println(s11) // 输出:[1 2 3 20 30]
s11 = append(s11[:3], s11[4:]...) // 删除下标为3的元素
fmt.Println(s11) // 输出:[1 2 3 30]
s11 = append(s11[:1], s11[3:]...) // 删除下标从1到2的元素
fmt.Println(s11) // 输出:[1 30]
s11 = append(s11, make([]int, 5)...) // 扩展5个元素
fmt.Println(s11) // 输出:[1 30 0 0 0 0 0]
/*
切片垃圾回收
切片的底层指向一个数组,数组实际容量大于切片的容量,只有没有切片指向数组的时候,数组才会释放。
如果是一个很大的数组,然后切片只使用很小一部分,应该将使用的数据拷贝到新的结构中,避免内存占用
*/
}
func Compare(a, b []byte) int {
for i := 0; i < len(a) && i < len(b); i++ {
if a[i] < b[i] {
return -1
} else if a[i] > b[i] {
return 1
}
}
/*
if len(a) > len(b) {
return 1
} else if len(a) < len(b) {
return -1
}
*/
switch {
case len(a) > len(b):
return 1
case len(a) < len(b):
return -1
}
return 0
}
复制代码
划线
评论
复制
发布于: 2021 年 08 月 26 日阅读数: 6
版权声明: 本文为 InfoQ 作者【HelloBug】的原创文章。
原文链接:【http://xie.infoq.cn/article/c421e30800eae7e0b2a2c13e2】。
本文遵守【CC BY-NC-ND】协议,转载请保留原文出处及本版权声明。
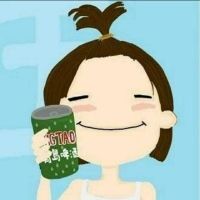
HelloBug
关注
还未添加个人签名 2018.09.20 加入
还未添加个人简介
评论