1
如何让网络音频或本地音频文件发布到频道中
发布于: 2021 年 05 月 14 日
前言
大家好,今天带给大家一个基于 anyRTC Web SDK 实现播放网络音频或本地音频文件的功能。
前提条件
在开始写代码之前还需要做一些准备工作,如果你之前没有使用过 anyRTC Web SDK 这里还需要花几分钟时间准备一下, 详见:开发前期准备
操作流程
配置
const config = {
appid: appid, // 这里填写前面 "前提条件" 里面获取到的appid
channel: '505050', // 频道号
uid: 'web' + Math.floor(Math.random() * 2021428) // 随机生成的用户id
};
var client = null; // 本地客户端对象
复制代码
获取 DOM 节点
const urlButton = document.getElementsByTagName('span')[0];
const fileButton = document.getElementsByTagName('span')[1];
const urlInput = document.getElementsByTagName('input')[0];
const fileInput = document.getElementsByTagName('input')[1];
const join = document.getElementsByTagName('p')[0];
复制代码
绑定点击事件
// 网络音频
urlButton.onclick = () => {
// http://music.163.com/song/media/outer/url?id=1811921555.mp3
// 为了节省大家的时间,笔者这里给大家准备了一个网络音频资源
// 大家可以把上面的链接放到浏览器地址栏访问一下,然后浏览器地址栏的地址会发生变化
// 然后把改变后的浏览器地址栏地址复制到input框里面
const source = urlInput.value;
if (source) {
publishAndPlay(source);
} else {
alert('请输入网络mp3链接');
}
};
// 本地音频文件
fileButton.onclick = () => {
const source = fileInput.files[0];
if (source) {
publishAndPlay(source);
} else {
alert('请选择音频文件');
}
};
// 加入房间的逻辑本来是可以初始化的时候就自动做的
// 但是由于谷歌浏览器不允许自动播放音频(必须要用户和页面发生交互才能播放), 所以才加上点击加入房间的步骤;
// 这是为了远端用户能自动播放
join.onclick = () => {
const { appid, channel, uid } = config;
// 加入频道
client.join(appid, channel, null, uid).then(() => {
// 一些样式操作
urlButton.parentNode.style.display = 'flex';
fileButton.parentNode.style.display = 'flex';
join.style.display = 'none';
}, () => {
alert('加入房间失败');
});
};
复制代码
方法函数
const publishAndPlay = async (source) => {
const bufferAudioOptions = {source};
// 通过音频文件或者 AudioBuffer 对象创建一个音频轨道。
const track = await ArRTC.createBufferSourceAudioTrack(bufferAudioOptions);
// 本地播放音频 (必须)
track.play();
// 启动进程音频缓冲 (必须)
track.startProcessAudioBuffer();
// 发布到频道中 (到这里远端用户(确保已加入频道)和本地都能听见声音了)
client.publish(track);
};
复制代码
项目初始化
const init = () => {
client = ArRTC.createClient({ mode: "rtc", codec: "h264" });
// 监听用户发布音视频流
client.on("user-published", async (user, mediaType) => {
await client.subscribe(user, mediaType);
if (mediaType === 'audio') {
user.audioTrack.play();
}
});
};
init();
复制代码
完整代码如下
至此播放网络音频或本地音频文件的功能就完成了,感兴趣的小伙伴可以复制下方代码查看运行效果,如果你喜欢这篇文章或者想持续关注 anyRTC 的最新技术动向,可以关注我哦;
HTML 部分
<div class='main'>
<p class='join'>加入房间</p>
<div class='url-box'>
<input type="text" placeholder="输入网络mp3链接">
<span class='button'>采集音频</span>
</div>
<div class='file-box'>
<input type="file">
<span class='button'>采集音频</span>
</div>
</div>
<script src="/<YOUR_PATH>/to/ArRTC@latest.js"></script>
<script src="index.js"></script>
复制代码
CSS 部分
.main {
display: flex;
justify-content: space-between;
width: 500px;
margin: 50px auto;
}
.main .join {
position: fixed;
top: 50%;
left: 50%;
width: 100px;
line-height: 34px;
border-radius: 4px;
text-align: center;
color: #fff;
font-size: 12px;
background-color: #409EFF;
cursor: pointer;
transform: translate(-50px, -17px);
transition: .3s;
}
.main .join:hover {
background-color: #66B1FF;
}
.main .url-box, .file-box {
display: none;
height: 80px;
justify-content: space-between;
flex-direction: column;
margin-bottom: 40px;
}
.main .button {
display: inline-block;
width: 80px;
line-height: 26px;
border-radius: 2px;
text-align: center;
color: #fff;
font-size: 12px;
background-color: #409EFF;
cursor: pointer;
}
复制代码
Javascript 部分
const urlButton = document.getElementsByTagName('span')[0];
const fileButton = document.getElementsByTagName('span')[1];
const urlInput = document.getElementsByTagName('input')[0];
const fileInput = document.getElementsByTagName('input')[1];
const join = document.getElementsByTagName('p')[0];
const config = {
appid: '',
channel: '505050',
uid: 'web' + Math.floor(Math.random() * 2021428)
};
var client = null;
urlButton.onclick = () => {
const source = urlInput.value;
if (source) {
publishAndPlay(source);
} else {
alert('请输入网络mp3链接');
}
};
fileButton.onclick = () => {
const source = fileInput.files[0];
if (source) {
publishAndPlay(source);
} else {
alert('请选择音频文件');
}
};
join.onclick = () => {
const { appid, channel, uid } = config;
client.join(appid, channel, null, uid).then(() => {
urlButton.parentNode.style.display = 'flex';
fileButton.parentNode.style.display = 'flex';
join.style.display = 'none';
}, () => {
alert('加入房间失败');
});
};
const publishAndPlay = async (source) => {
const bufferAudioOptions = {source};
const track = await ArRTC.createBufferSourceAudioTrack(bufferAudioOptions);
track.play();
track.startProcessAudioBuffer();
client.publish(track);
};
const init = () => {
client = ArRTC.createClient({ mode: "rtc", codec: "h264" });
client.on("user-published", async (user, mediaType) => {
await client.subscribe(user, mediaType);
if (mediaType === 'audio') {
user.audioTrack.play();
}
});
};
init();
复制代码
划线
评论
复制
发布于: 2021 年 05 月 14 日阅读数: 16
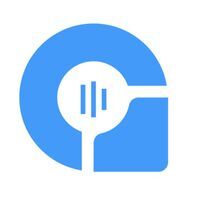
anyRTC开发者
关注
实时交互,万物互联! 2020.08.10 加入
实时交互,万物互联,全球实时互动云服务商领跑者!
评论