【Java 异常】try-catch,mysql 菜鸟入门系列
}
再看看 String.valueOf
:可以发现打印出 null 其实是经过处理的。
public static String valueOf(Object obj) {
return (obj == null) ? "null" : obj.toString();
}
================================================================================
Java 中有各种各样的异常
所有的异常最终都继承自
java.lang.Throwable
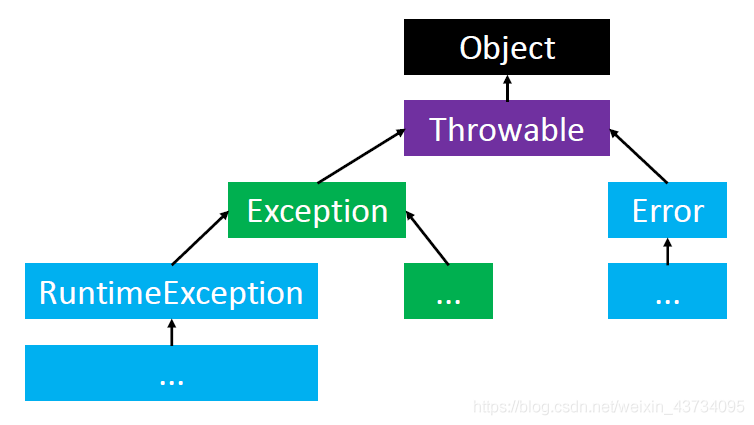
如何防止程序因为抛出异常导致终止运行?
可以通过
try-catch
来捕捉处理异常
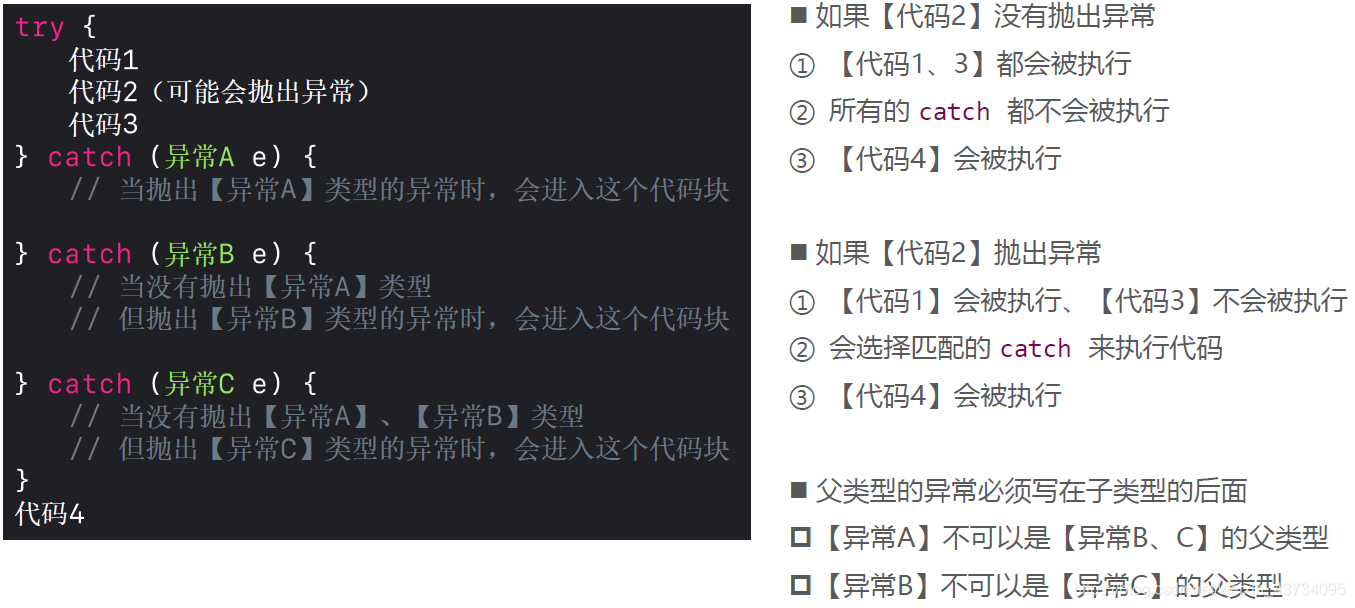
思考:下面代码的打印结果是什么?
public static void main(String[] args) {
System.out.println(1);
try {
System.out.println(2);
Integer i = new Integer("abc");
System.out.println(3);
} catch (NumberFormatException e) {
System.out.println(4);
}
System.out.println(5);
}
1
2
4
5
一个 catch
捕获多种类型的异常:
try {
} catch(异常 A | 异常 B | 异常 C) {
// 当抛出【异常 A】或【异常 B】或【异常 C】类型的异常时,会进入这个代码块
}
从 Java 7 开始,单个 catch 可以捕获多种类型的异常
如果并列的几个异常类型之间存在父子关系,保留父类型即可
这里的变量 e 是隐式 final 的
异常对象的常用方法(getMessage、printStackTrace)
try {
Integer integer = new Integer("abc");
} catch (NumberFormatException e) {
// 异常描述
System.out.println(e.getMessage());
// 异常名称 + 异常
System.out.println(e);
// 打印堆栈信息
e.printStackTrace();
}

try
或 catch
正常执行完毕后,一定会执行 finally
中的代码:
finally
可以和try-catch
搭配使用,也可以只和try
搭配使用经常会在
finally
中编写一些关闭、释放资源 的代码(比如关闭文件)
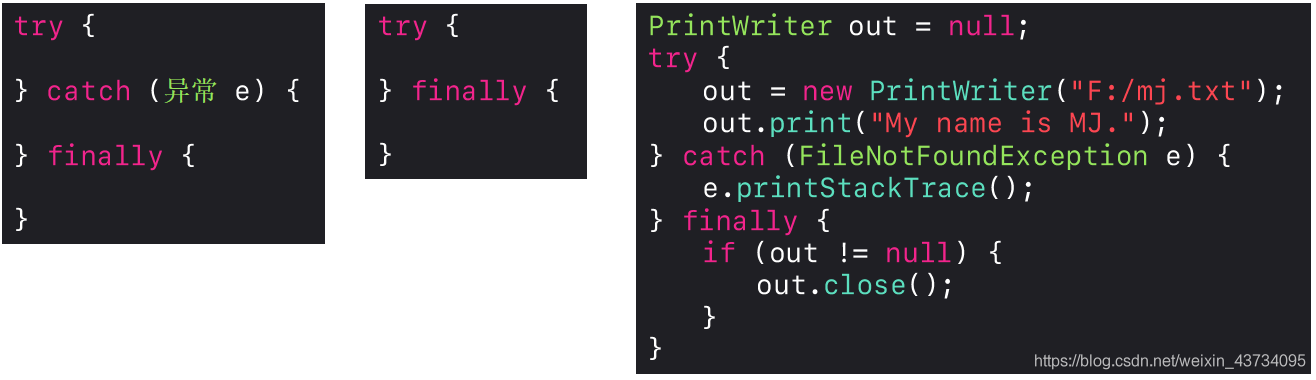
finally
细节:
如果在执行 try
或 catch
时,JVM 退出或者当前线程被中断、杀死
finally
可能不会执行
如果 try
或 catch
中使用了 return
、break
、continue
等提前结束语句
finally
会在return
、break
、continue
之前执行
思考:下面代码的打印结果是什么?
for (int i = 1; i <= 3; i++) {
try{
System.out.println(i + "_try_1");
if (i == 2) continue;
System.out.println(i + "_try_2");
} finally {
System.out.println(i + "_finally");
}
}
1_try_1
1_try_2
1_finally
2_try_1
2_finally
3_try_1
3_try_2
3_finally
for (int i = 1; i <= 3; i++) {
try{
System.out.println(i + "_try_1");
if (i == 2) break;
System.out.println(i + "_try_2");
} finally {
System.out.println(i + "_finally");
}
}
1_try_1
1_try_2
1_finally
2_try_1
2_finally
public static void main(String[] args) {
System.out.println(get());
}
static int get() {
try {
new Integer("abc");
System.out.println(1);
return 2;
} catch (Exception e) {
System.out.println(3);
return 4;
} finally {
System.out.println(5);
}
}
3
5
4
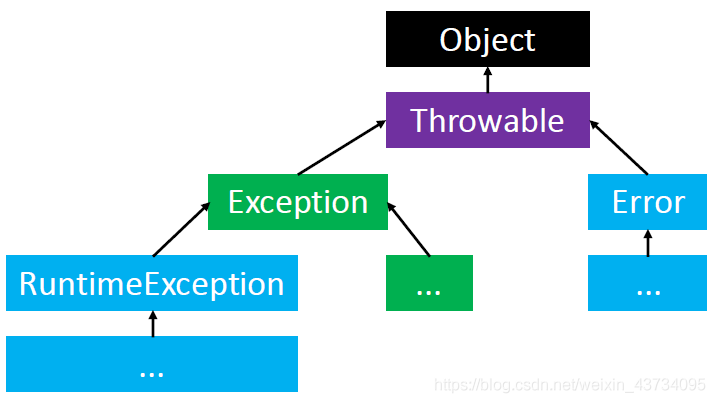
检查型异常(Checked Exception)
这类异常一般难以避免,编译器会进行检查
如果开发者没有处理这类异常,编译器将会报错
哪些异常是检查型异常?
除 Error
、RuntimeException
以外的异常
非检查型异常(Unchecked Exception)
这类异常一般可以避免,编译器不会进行检查
如果开发者没有处理这类异常,编译器将不会报错
哪些异常是非检查型异常?
Error
、RuntimeException
常见的检查型异常:
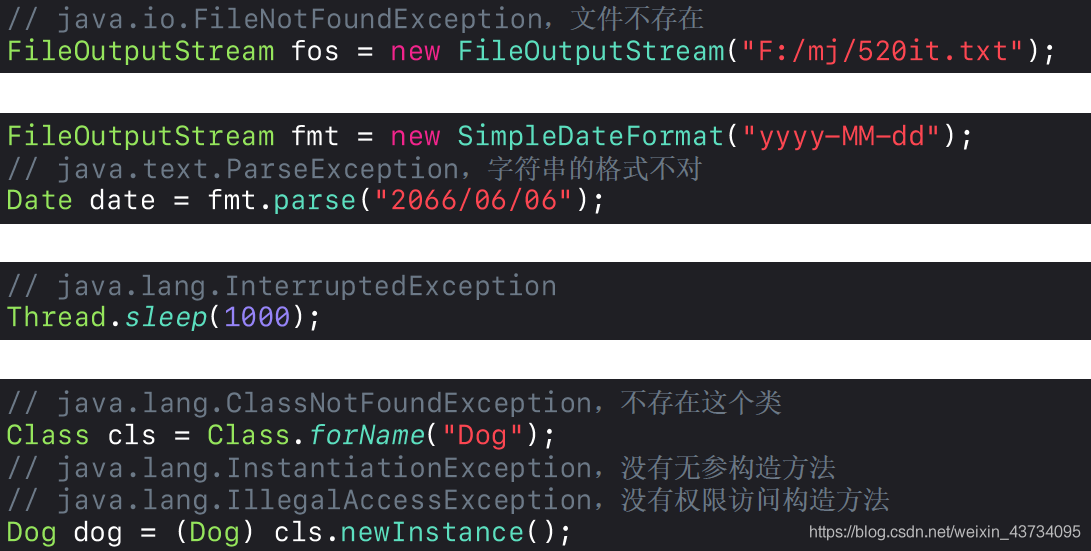
常见的非检查型异常 – Error
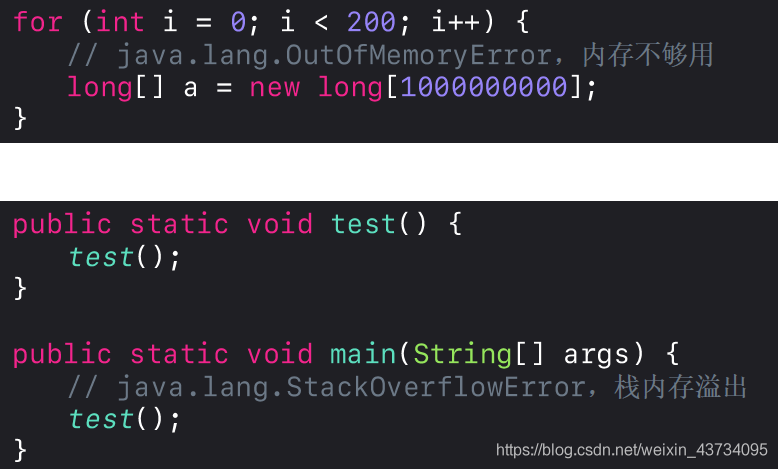
常见的非检查型异常 – RuntimeException
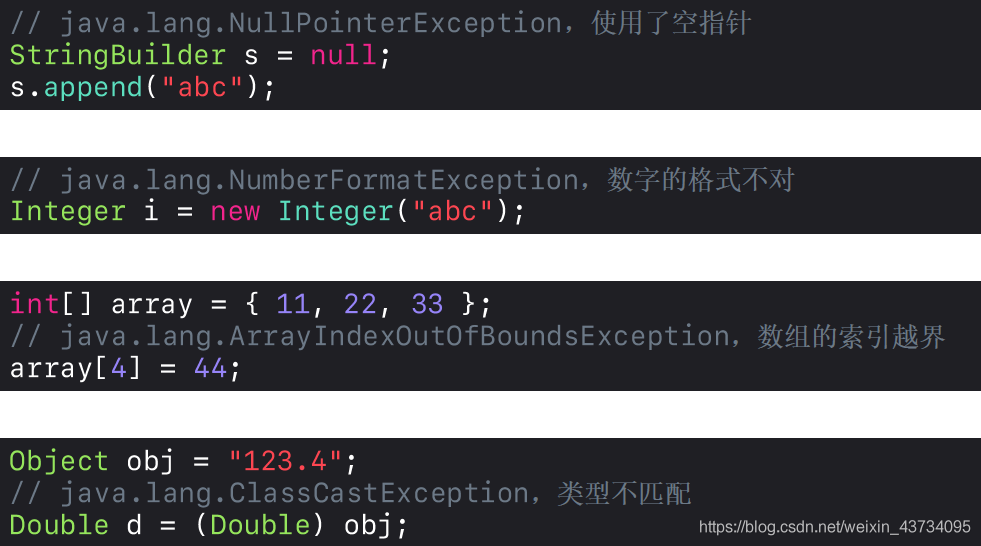
throws
的作用:将异常抛给上层方法
void test() throws FileNotFoundException, ClassNotFoundException {
PrintWriter out = new PrintWriter("F:/mj/520it.txt");
Class cls = Class.forName("Dog");
}
如果 throws
后面的异常类型存在父子关系,保留父类型即可
void test() throws Exception {
PrintWriter out = new PrintWriter("F:/mj/520it.txt");
Class cls = Class.forName("Dog");
}
void test() throws Throwable {
PrintWriter out = new PrintWriter("F:/mj/520it.txt");
Class cls = Class.forName("Dog");
}
可以一部分异常使用 try-catch
处理,另一部分异常使用 throws
处理:
void test() throws FileNotFoundException {
PrintWriter out = new PrintWriter("F:/mj/520it.txt");
try {
Class cls = Class.forName("Dog");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
thorws
的流程:
如果异常最终抛给了 JVM,那么整个 Java 程序将终止运行。
下面这个例子中,异常从 method1
开始网上抛,到 method2
,再到 method3
,最后抛给 main
,main
又抛给了 JVM;
public static void main(String[] args) throws ClassNotFoundException {
method1(); // 正常来说, 这里必须处理异常了, 否则出异常后 Java 程序会终止运行
}
static void method1() throws ClassNotFoundException {
method2(); // 这里可以处理异常
}
static void method2() throws ClassNotFoundException {
method3(); // 这里可以处理异常
}
static void method3() throws ClassNotFoundException{
Class clazz = Class.forName("Dog"); // 这里可以处理异常
}
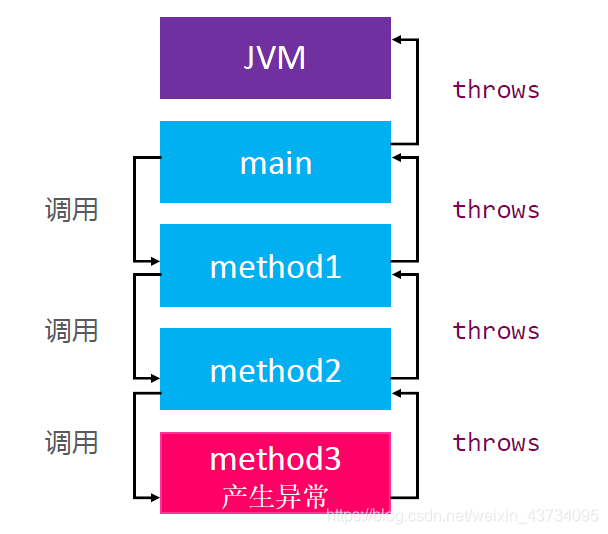
所以如果一直不处理异常,只往上抛最终会导致程序停止运行,一定要在某个地方处理异常。
throws
的细节
当父类的方法没有 throws
异常:子类的重写方法也不能 throws
异常
当父类的方法有 throws
异常,子类的重写方法可以:
不
throws
异常throws
跟父类一样的异常throws
父类异常的子类型
public class Person {
public void test1() {}
public void test2() throws IOException {}
public void test3() throws IOException {}
public void test4() throws IOException {}
public void test5() throws IOException {}
}
public class Student extends Person {
@Override
public void test1() {}
@Override
public void test2() {}
@Override
public void test3() throws IOException {}
@Override
public void test4() throws FileNotFoundException {}
// 只能抛出父类异常的子类, 或者不抛
// public void test5() throws Exception {} // 抛出子类异常的父类异常, 会报错
}
使用 throw
可以抛出一个新建的异常
public class Person {
public Person(String name) throws Exception { // 抛出异常
if (name == null || name.length() == 0) {
// 检查型异常必须处理或者往上抛
throw new Exception("name must not be empty.");
}
}
}
public class Person {
public Person(String name) {
if (name == null || name.length() == 0) {
// 非检查型异常可以不处理
throw new IllegalArgumentException("name must not be empty.");
}
}
}
自定义异常(Exception、RuntimeException)
开发中自定义的异常类型,基本都是以下 2 种做法:
继承自
Exception
使用起来代码会稍微复杂
希望开发者重视这个异常、认真处理这个异常(该异常无法从代码层面去避免,必须引起重视)
继承自
RuntimeException
使用起来代码会更加简洁
不严格要求开发者去处理这个异常(因为规范的代码可以避免该异常)
示例:
自定义两种异常:EmptyNameException
、WrongAgeException
public class EmptyNameException extends RuntimeException {
public EmptyNameException() {
super("name must not be empty");
}
}
public class WrongAgeException extends RuntimeException {
private int age;
public WrongAgeException(int age) {
super("wrong age:" + age + ", age must be > 0");
}
}
在 Person
类中使用自定义的异常:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
if (name == null || name.length() == 0) {
throw new EmptyNameException();
}
if (age <= 0) {
throw new WrongAgeException(age);
}
this.name = name;
this.age = age;
}
}
使用 Person
类有几率抛出异常:
public static void main(String[] args) {
//WrongAgeException: wrong age:-10, age must be > 0
Person person = new Person("Jack", -10);
// 这句代码不会执行
System.out.println(1);
}
将错误处理代码与普通代码区分开
能将错误信息传播到调用堆栈中
能对错误类型进行区分和分组
=============
===========================================================================
具体见:File、字符集、字节流、字符流、缓冲流、数据流、对象流、序列化、try-with-resources语句
从 Java7 开始推出的try-with-resources
语句(可以没有catch
、finally
)
try(资源 1; 资源 2; ...) {
} catch (Exception e) {
} finally {
}
可以在try
后面的小括号中声明一个或多个资源(resource)
实现了
java.lang.AutoCloseable
接口的实例,都可以称之为是资源
不管try
中的语句是正常还是意外结束
最终都会自动按顺序调用每一个资源的
close
方法(close
方法的调用顺序与资源的声明顺序相反)调用完所有资源的
close
方法后,再执行finally
中的语句
评论