【Flutter 专题】15 图解 ListView 异步加载数据与 Loading 等待
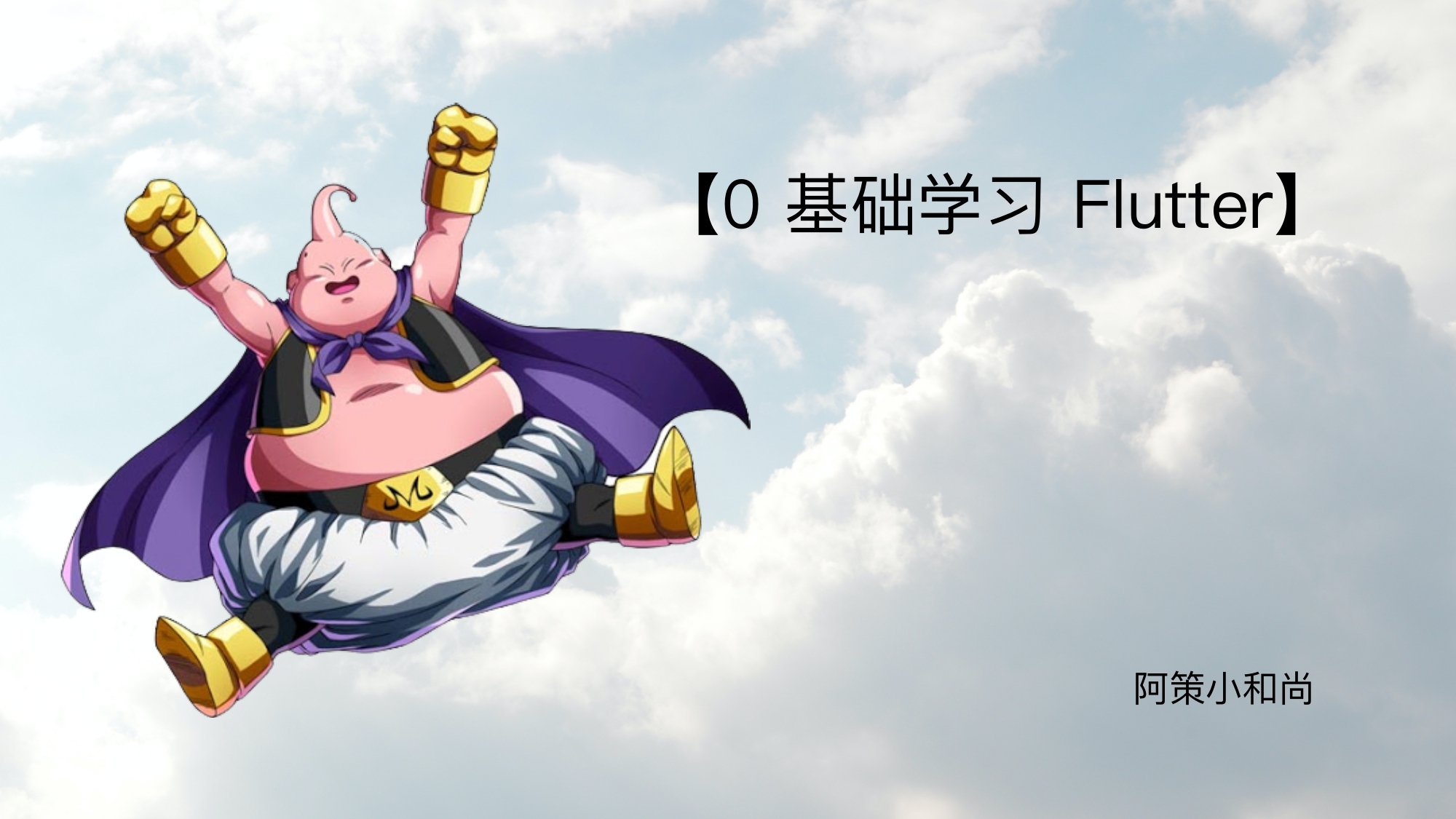
小菜前两天再学 ListView 时,整理了一下在列表中展示多种不同 item 样式,今天继续深入学习异步请求数据并加载新闻列表以及初始进入页面的 loading 等小知识点。暂时还没有学习下拉刷新与上划加载更多。
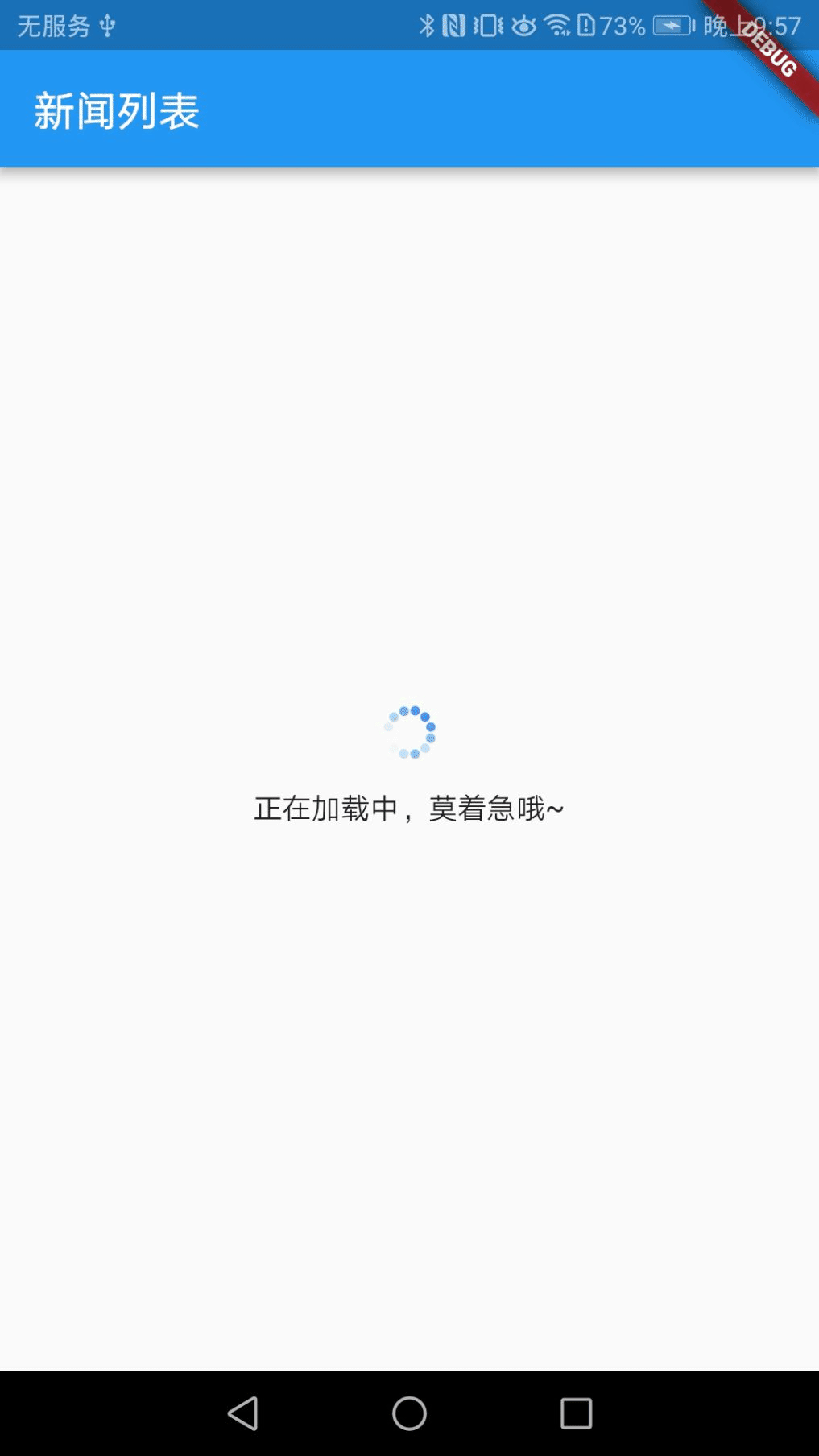
一. 异步请求数据 async + wait
小菜在前一篇关于网络请求小博客中整理过基本的异步使用方法;小菜在学习中发现有两个小地方需要注意一下:
使用 StatefulWidget 时,一定一定不要忘记 setState(() {});
小菜准备在刚进入页面时,开启异步请求数据,可以在 initState() 中进行操作,如下:
@override
void initState() {
getNewsData();
}
二. json 数据解析
请求到数据之后必然得需要 json 解析,首先需要引入 import 'dart:convert' show json; 之后,小菜主要是使用 response.body 中数据进行处理,json.decode(response.body); 将 json 转为标准的 key-value 格式;最让小菜头疼的是实体类转换,实体类的定义一定要全面且字段格式正确,不然解析出问题不容易定位。(请谅解:小菜的测试 url 无法公布)
getNewsData() async {
await http
.get('https://...?sid=xkycs&cid=${cid}&rowNumber=${rowNumber}')
.then((response) {
if (response.statusCode == 200) {
var jsonRes = json.decode(response.body);
newsListBean = NewsListBean(jsonRes);
setState(() {
for (int i = 0; i < newsListBean.list.length; i++) {
print("==77==${newsListBean.list[i].title}");
dataItems.add(newsListBean.list[i]);
}
});
}
});
}
小菜单独为实体类区分为一个新的 .dart 文件,需要注意的是,若实体类中有列表,一定要注意判空,如下:
class NewsListBean {
List<ListBean> list;
int rowNumber;
bool success;
String msg;
NewsListBean(jsonRes) {
rowNumber = jsonRes['rowNumber'];
success = jsonRes['success'];
msg = jsonRes['msg'];
list = [];
if (jsonRes['list'] != null) {
for (var dataItem in jsonRes['list']) {
list.add(new ListBean(dataItem));
}
}
}
}
class ListBean {
int fileID;
String title;
int version;
String abstractX;
String publishTime;
String realPublishTime;
int articleType;
String pic3;
String pic2;
String pic1;
int bigPic;
String tag;
String contentUrl;
String videoImgUrl;
ListBean(jsonRes) {
fileID = jsonRes['fileID'];
title = jsonRes['title'];
version = jsonRes['version'];
abstractX = jsonRes['abstract'];
publishTime = jsonRes['publishTime'];
realPublishTime = jsonRes['realPublishTime'];
articleType = jsonRes['articleType'];
pic3 = jsonRes['pic3'];
pic2 = jsonRes['pic2'];
pic1 = jsonRes['pic1'];
bigPic = jsonRes['bigPic'];
tag = jsonRes['tag'];
contentUrl = jsonRes['contentUrl'];
videoImgUrl = jsonRes['videoImgUrl'];
}
}
三. 列表加载数据
小菜每次写 item 时都会想到 Flutter 中一切都是 Widget 的重要性,小菜建议很多公共的或重复的 Widget 完全可以提取成统一的 Widget,即方便管理也会大幅度减少代码量。
Widget buildListData(BuildContext context, ListBean listBean) {
Widget itemWidget;
if (listBean != null) {
switch (listBean.articleType) {
case 1:
itemWidget = new Card(
child: new Container(
child: new Column(
children: <Widget>[
new Row(
children: <Widget>[
new Expanded(
child: new Container(
padding: const EdgeInsets.fromLTRB(3.0, 6.0, 3.0, 0.0),
child: new Image.network( listBean.pic1, fit: BoxFit.cover, ),
height: 100.0,
),
flex: 1,
),
new Expanded(
child: new Container(
padding: const EdgeInsets.fromLTRB(3.0, 6.0, 3.0, 0.0),
child: new Image.network( listBean.pic2, fit: BoxFit.cover, ),
height: 100.0,
),
flex: 1,
),
new Expanded(
child: new Container(
padding: const EdgeInsets.fromLTRB(3.0, 6.0, 3.0, 0.0),
child: new Image.network( listBean.pic3, fit: BoxFit.cover, ),
height: 100.0,
),
flex: 1,
),
],
),
new Padding( padding: new EdgeInsets.fromLTRB(8.0, 6.0, 0.0, 6.0), child: botRow(listBean), ),
],
),
),
);
break;
case 2:
itemWidget = new Card(
child: new Column(
children: <Widget>[
new Row(
children: <Widget>[
new Expanded(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
new Padding( padding: new EdgeInsets.fromLTRB(8.0, 6.0, 0.0, 3.0), child: new Text(listBean.title), ),
new Padding( padding: new EdgeInsets.fromLTRB(8.0, 6.0, 0.0, 3.0), child: new Text( listBean.abstractX, style: new TextStyle(fontSize: 14.0), ), ),
new Padding( padding: new EdgeInsets.fromLTRB(8.0, 6.0, 0.0, 3.0), child: botRow(listBean), ),
],
),
flex: 2,
),
new Expanded(
child: new Container(
padding: const EdgeInsets.fromLTRB(3.0, 6.0, 3.0, 6.0),
child: new Image.network( listBean.pic1, fit: BoxFit.cover, ),
height: 100.0,
),
flex: 1,
),
],
),
],
),
);
break;
default:
Widget absWi;
if (listBean.abstractX == null || listBean.abstractX.length == 0) {
absWi = new Container( width: 0.0, height: 0.0, );
} else {
absWi = new Padding( padding: new EdgeInsets.fromLTRB(0.0, 8.0, 0.0, 0.0),child: new Text( listBean.abstractX, style: new TextStyle(fontSize: 14.0), ), );
}
itemWidget = new Card(
child: new Padding(
padding: new EdgeInsets.all(10.0),
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
new Text( listBean.title, style: new TextStyle(fontSize: 17.0), ),
absWi,
new Padding( padding: new EdgeInsets.fromLTRB(0.0, 8.0, 0.0, 0.0), child: botRow(listBean), ),
],
),
),
);
break;
}
return itemWidget;
}
}
// 底部时间和音视频显隐性
Widget botRow(ListBean listBean) {
Widget videoWi;
if (listBean.videoImgUrl == null || listBean.videoImgUrl.length == 0) {
videoWi = new Container( width: 0.0, height: 0.0, );
} else {
videoWi = new Padding(
padding: new EdgeInsets.fromLTRB(0.0, 0.0, 6.0, 0.0),
child: new Row(
children: <Widget>[
new Padding(
padding: new EdgeInsets.fromLTRB(0.0, 2.0, 6.0, 0.0),
child: new Center( child: new Icon( Icons.queue_music, size: 13.0, color: Colors.blueAccent, ), ),
),
new Text( '音频', style: new TextStyle(fontSize: 13.0), ),
],
),
);
}
return new Padding(
padding: new EdgeInsets.fromLTRB(0.0, 8.0, 0.0, 0.0),
child: new Row(
children: <Widget>[
videoWi,
new Padding(
padding: new EdgeInsets.fromLTRB(0.0, 0.0, 6.0, 0.0),
child: new Icon( Icons.access_time, size: 13.0, color: Colors.blueAccent,),
),
new Text( listBean.publishTime, style: new TextStyle(fontSize: 13.0),),
],
),
);
}
小菜处理成在没有加载出列表数据之前添加一个 loading 提醒,如下:
Widget childWidget() {
Widget childWidget;
if (dataItems != null && dataItems.length != 0) {
childWidget = new Padding(
padding: EdgeInsets.all(6.0),
child: new ListView.builder(
itemCount: dataItems.length,
itemBuilder: (context, item) {
return buildListData(context, dataItems[item]);
},
),
);
} else {
childWidget = new Stack(
children: <Widget>[
new Padding(
padding: new EdgeInsets.fromLTRB(0.0, 0.0, 0.0, 35.0),
child: new Center(
child: SpinKitFadingCircle(
color: Colors.blueAccent,
size: 30.0,
),
),
),
new Padding(
padding: new EdgeInsets.fromLTRB(0.0, 35.0, 0.0, 0.0),
child: new Center(
child: new Text('正在加载中,莫着急哦~'),
),
),
],
);
}
return childWidget;
}
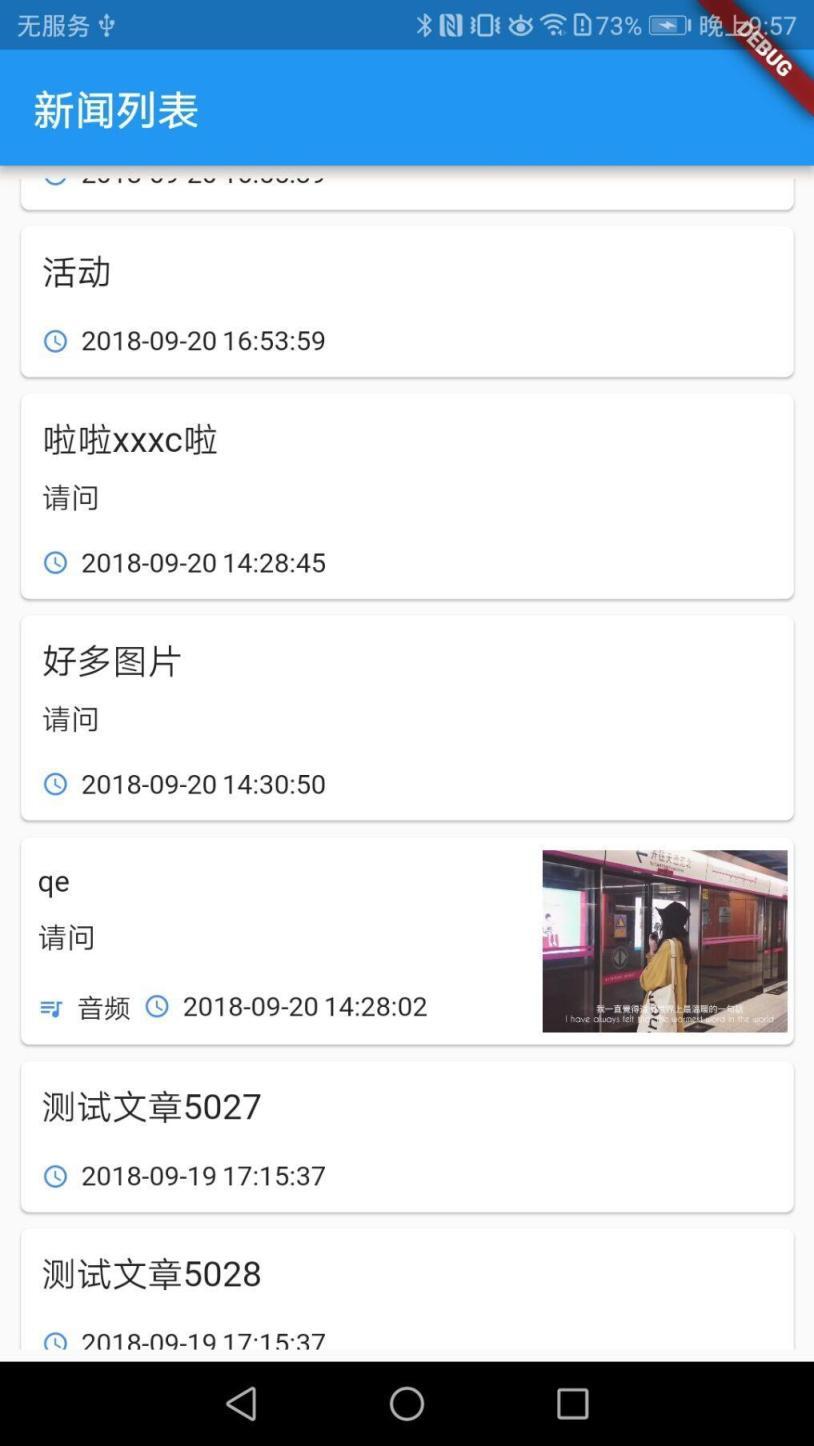
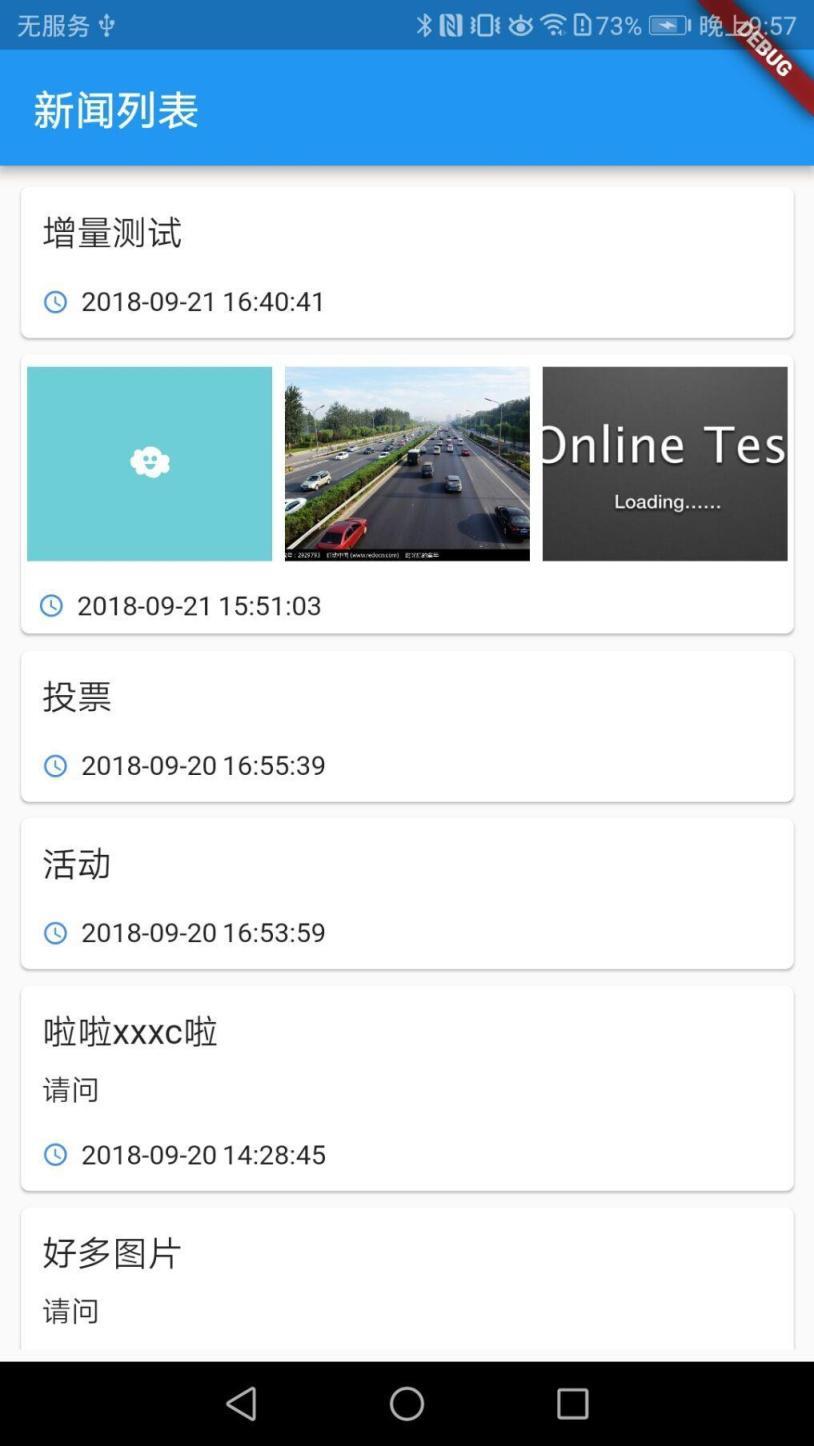
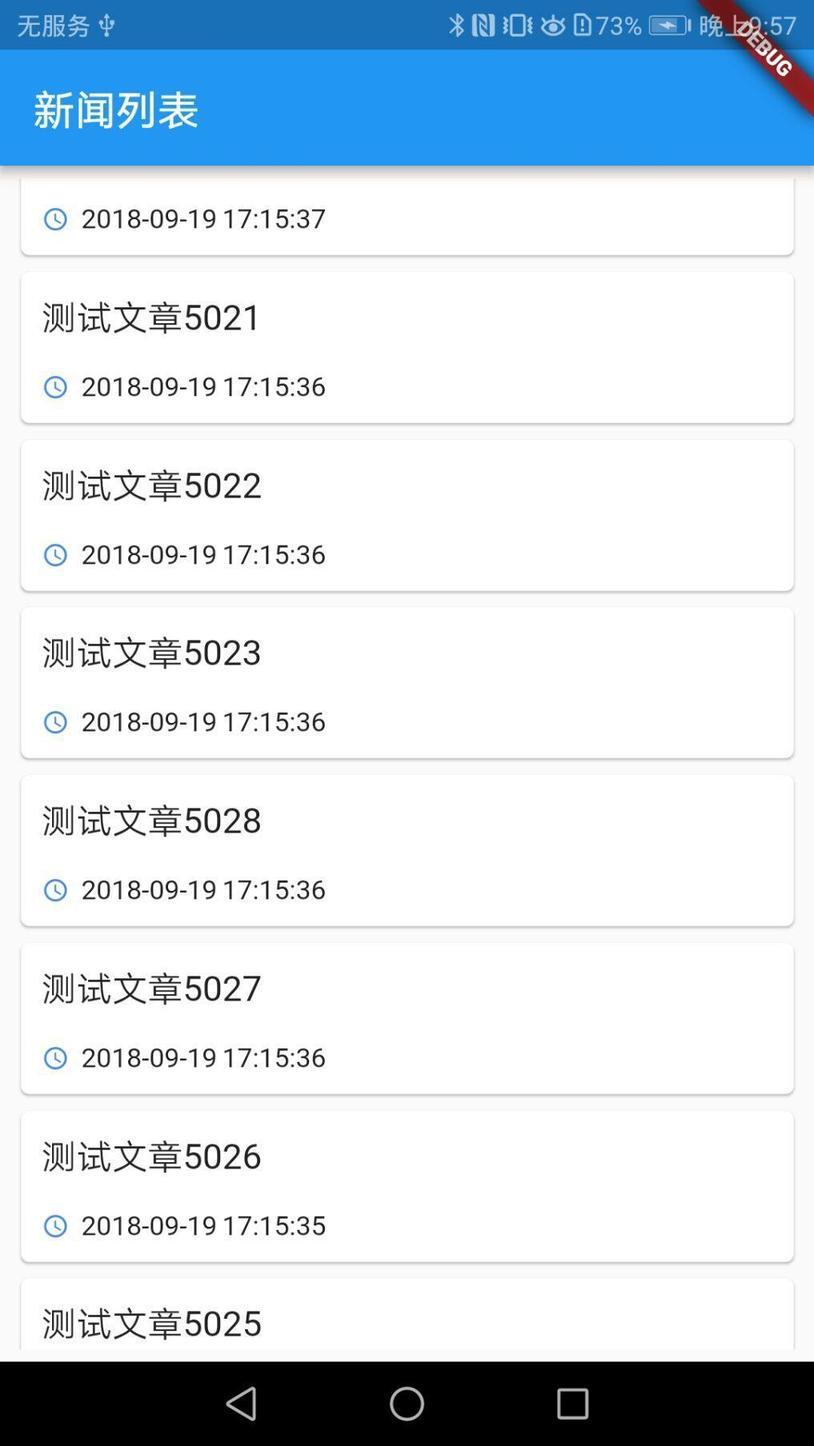
四. loading 提醒
小菜在加载数据之后发现,网络状况不佳或数据量大时都应有 loading 提醒,尽量给用户一个良好的体验。 小菜偷了个懒,借用一个三方库 flutter_spinkit,这个 loading 库集成简单而且效果多样,基本包含日常中常见的样式。
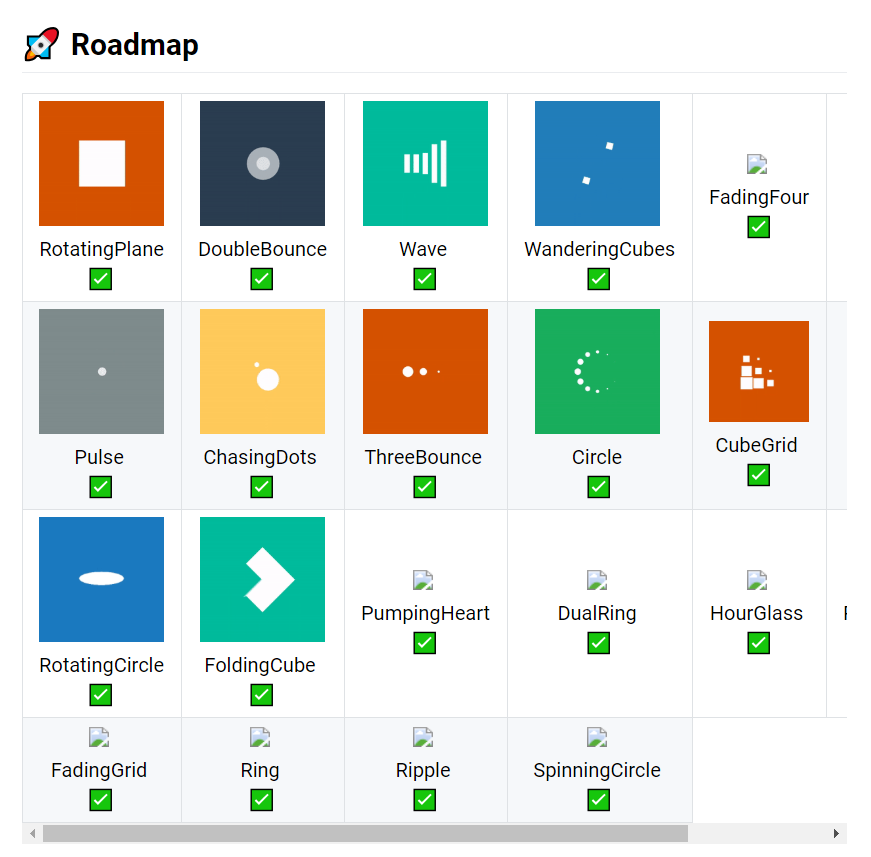
####集成步骤:
pubspec.yaml 中添加 flutter_spinkit: "^2.1.0";
在相应的 .dart 文件中添加引用 import 'package:flutter_spinkit/flutter_spinkit.dart';
添加需要展示的样式:SpinKit + Wave() 方式,同时与官网的使用有点区别,官网中用 width 和 height 来设置宽高,但是小菜在测试过程中,源码中提供了 size 方法,一个属性即可。
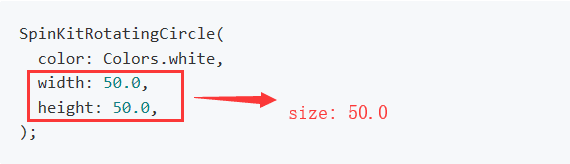
new Column(
children: <Widget>[
new SpinKitRotatingPlain(color: Colors.blueAccent, size: 30.0,),
new SpinKitRotatingCircle(color: Colors.blueAccent, size: 30.0,),
new SpinKitDoubleBounce(color: Colors.blueAccent, size: 30.0,),
new SpinKitRing(color: Colors.blueAccent, size: 30.0,),
new SpinKitWave(color: Colors.blueAccent, size: 30.0,),
new SpinKitWanderingCubes(color: Colors.blueAccent, size: 30.0,),
new SpinKitFadingCube(color: Colors.blueAccent, size: 30.0,),
new SpinKitFadingFour(color: Colors.blueAccent, size: 30.0,),
new SpinKitPulse(color: Colors.blueAccent, size: 30.0,),
new SpinKitChasingDots(color: Colors.blueAccent, size: 30.0,),
new SpinKitHourGlass(color: Colors.blueAccent, size: 30.0,),
new SpinKitSpinningCircle(color: Colors.blueAccent, size: 30.0,),
],
)
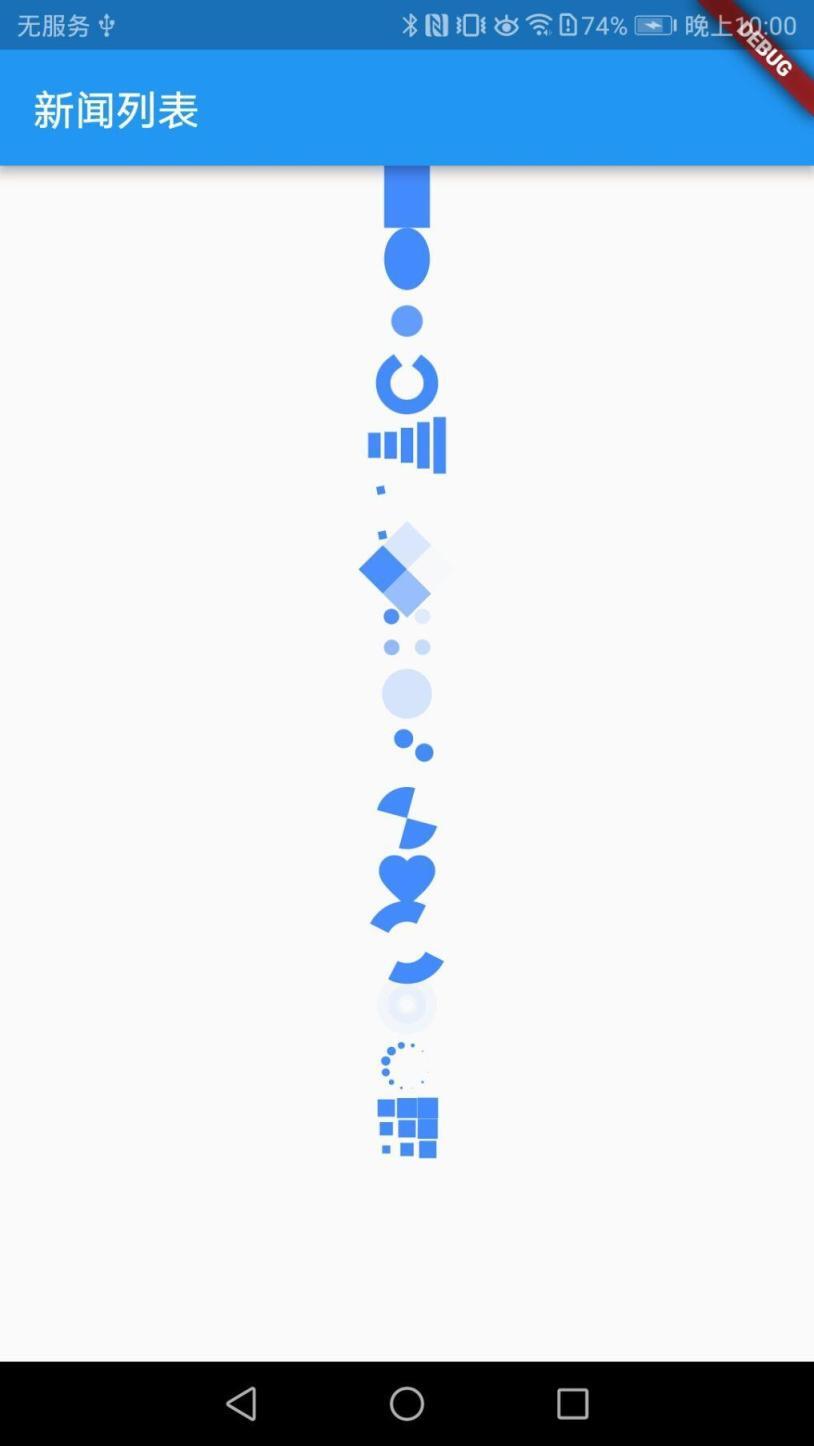
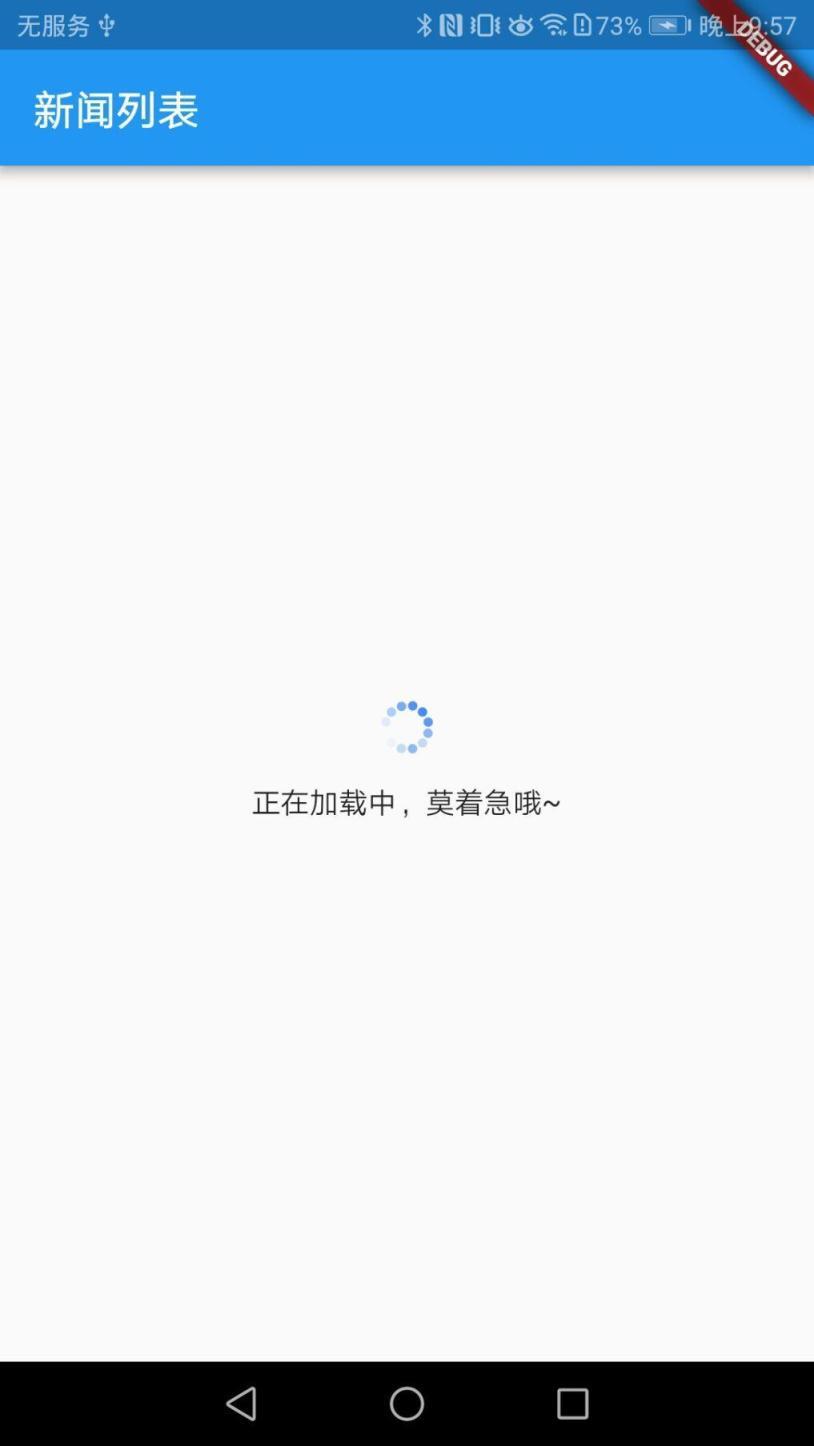
小菜刚接触 Flutter 时间不长,还有很多不清楚和不理解的地方,如果又不对的地方还希望多多指出。
来源:阿策小和尚
版权声明: 本文为 InfoQ 作者【阿策小和尚】的原创文章。
原文链接:【http://xie.infoq.cn/article/bca64eae51ce3a3e468382705】。文章转载请联系作者。
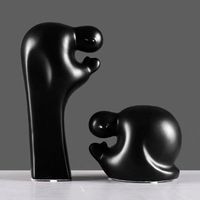
阿策小和尚
还未添加个人签名 2021.05.13 加入
Android / Flutter 小菜鸟~
评论