SpringBoot 实现大文件视频转码(转码基于 FFMPEG 实现)
文件转码
@param filePath
@return com.openailab.oascloud.common.model.ResponseResult
@author zxzhang
@date 2019/12/10
*/
public int transcode(String filePath) {
//1、获取文件名和后缀
String ext = getExt(filePath);
String fileDir = getFileDir(filePath);
String fileName = getFileName(filePath);
//2、删除同名文件
File originFile = new File(fileDir + fileName + BootstrapConst.SPOT + ext);
if (originFile.exists()){
originFile.delete();
}
//3、视频转码
LinkedList<String> ffmpegCmds = new LinkedList<>();
ffmpegCmds.add("ffmpeg");
ffmpegCmds.add("-i");
ffmpegCmds.add(bootstrapConfig.getFileRoot() + filePath);
ffmpegCmds.add("-c:v");
ffmpegCmds.add("libx264");
ffmpegCmds.add("-strict");
ffmpegCmds.add("-2");
ffmpegCmds.add(bootstrapConfig.getFileRoot() + fileDir + BootstrapConst.PATH_SEPARATOR + fileName + ".mp4");
ProcessBuilder builder = new ProcessBuilder();
builder.command(ffmpegCmds);
Process ffmpeg = null;
try {
ffmpeg = builder.start();
} catch (IOException e) {
e.printStackTrace();
}
String cmdStr = Arrays.toString(ffmpegCmds.toArray()).replace(",", "");
LOG.info("---开始执行 FFmpeg 指令:--- 执行线程名:" + builder.toString());
LOG.info("---已执行的 FFmepg 命令:---" + cmdStr);
// 取出输出流和错误流的信息
// 注意:必须要取出 ffmpeg 在执行命令过程中产生的输出信息,如果不取的话当输出流信息填满 jvm 存储输出留信息的缓冲区时,线程就回阻塞住
PrintStream errorStream = new PrintStream(ffmpeg.getErrorStream());
PrintStream inputStream = new PrintStream(ffmpeg.getInputStream());
errorStream.start();
inputStream.start();
// 等待 ffmpeg 命令执行完
int exit = 0;
try {
exit = ffmpeg.waitFor();
} catch (InterruptedException e) {
e.printStackTrace();
}
LOG.info("---执行结果:---" + (exit == 0 ? "【成功】" : "【失败】"));
if (exit == 0) {
originFile = new File(filePath);
if(originFile.exists()){
originFile.delete();
}
}
return exit;
}
/**
获取文件后缀
@param fileName
@return java.lang.String
@author zxzhang
@date 2019/12/10
*/
public String getExt(String fileName) {
return fileName.substring(fileName.lastIndexOf(".") + 1);
}
/**
获取文件所在目录
@param filePath
@return java.lang.String
@author zxzhang
@date 2019/12/10
*/
public String getFileDir(String filePath) {
return filePath.substring(0, filePath.lastIndexOf(BootstrapConst.PATH_SEPARATOR));
}
/**
获取文件名
@param filePath
@return java.lang.String
@author zxzhang
@date 2019/12/10
*/
public String getFileName(String filePath) {
return filePath.substring(filePath.lastIndexOf(BootstrapConst.PATH_SEPARATOR) + 1, filePath.lastIndexOf("."));
}
}
class PrintStream extends Thread {
java.io.InputStream __is = null;
public PrintStream(java.io.InputStream is) {
__is = is;
}
@Override
public void run() {
try {
while (this != null) {
int _ch = __is.read();
if (_ch != -1) {
System.out.print((char) _ch);
} else {
break;
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
2、BootstrapConst 类?
package com.openailab.oascloud.file.common.consts;
/**
@Classname: com.openailab.oascloud.security.common.consts.BootstrapConst
@Description: 简单常量定义
@Author: zxzhang
@Date: 2019/10/8
*/
public class BootstrapConst {
/**
文件缓冲区大小
*/
public static final Integer INPUT_BUFFER_SIZE = 8192;
/**
文件分隔符
*/
public static final char PATH_SEPARATOR = '/';
/**
文件点
*/
public static final char SPOT = '.';
/**
常量一
*/
public static final Integer ONE = 1;
/**
成功
*/
public static final Integer SUCCESS = 1;
/**
失败
*/
public static final Integer FAIL = 0;
/**
true
*/
public static final Boolean TRUE = true;
/**
false
*/
public static final Boolean FALSE = false;
}
三、FFMPeg DockerFile 实现
首先我们构建具有 JDK1.8 和 FFMPeg 环境的镜像
FROM jrottenberg/ffmpeg:centos
MAINTAINER oas.cloud
ADD jdk-8u231-linux-x64.tar.gz /usr/local/
ENV JAVA_HOME /usr/local/jdk1.8.0_231
ENV CLASSPATH JAVA_HOME/lib/tools.jar
ENV PATH JAVA_HOME/bin
WORKDIR /usr/local/oas/
然后将上述文件构建出的镜像的 tag 打为我们自己的镜像仓库地址,改为 10.12.1.202:8088/oascloud/ffmpeg:v1.0,我们的应用程序就可以以上述镜像文件(10.12.1.202:8088/oascloud/ffmpeg:v1.0)为基础构建出新的应用程序镜像,应用程序的 DockerFile 如下:
FROM 10.12.1.202:8088/oascloud/ffmpeg:v1.0
MAINTAINER oas.cloud
ENV LC_ALL=zh_CN.utf8
ENV LANG=zh_CN.utf8
ENV LANGUAGE=zh_CN.utf8
RUN localedef -c -f UTF-8 -i zh_CN zh_CN.utf8
ARG JAR_FILE
COPY ${JAR_FILE} /usr/local/oas/
WORKDIR /usr/local/oas/
代码结构如下:
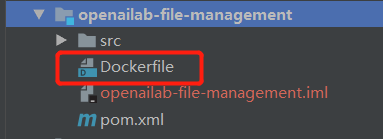
附上 Kubernetes 编排文件,deploy.yaml 内容如下:
apiVersion: apps/v1
kind: Deployment
metadata:
name: openailab-data-file-management
namespace: oas-tengine2dev
labels:
name: openailab-data-file-management
spec:
replicas: 1
selector:
评论