Spring--JdbcTemplate 基本使用,三年老 Java 经验面经
JdbcTemplate 是 spring 框架中提供的一个对象,是 Spring 对数据库操作在 JDBC 上的封装,使我们进行 JDBC 操作更加简单。
JdbcTemplate 执行 SQL 语句操作时主要提供以下方法:
execute 方法:
可以用于执行任何SQL语句,一般用于执行DDL语句,没有返回值;
update 方法:
用于执行新增、修改、删除等DML语句,返回一个int值,表示影响的行数;
query 方法与 queryXXX 方法:
用于执行查询相关DQL语句;
在使用 JdbcTemplate 之前要先确保自己项目中有添加:org.springframework.spring-jdbc.5.0.2.RELEASE
(JdbcTemplate 位于这个 jar 包中)以及org.springframework.spring-tx.5.0.2.RELEASE
(用于进行事务与异常控制)这两个依赖(jar 包)。
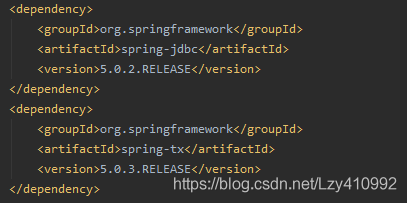
以账户为例,创建数据库表:
use spring;
Drop table if exists account;
create table account(
id int primary key auto_increment,
name varchar(40),
money float
)character set utf8 collate utf8_general_ci;
insert into account (name,money) values('aaa',1000);
insert into account (name,money) values('bbb',1000);
insert into account (name,money) values('ccc',1000);
数据库表:
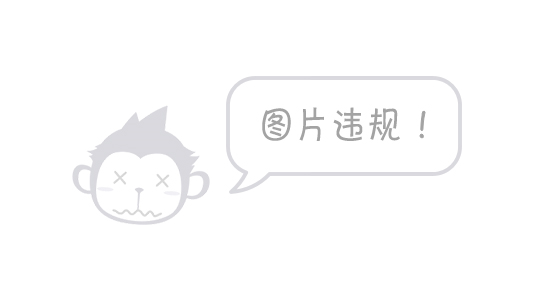
创建 maven 项目并在 pom.xml 中添加相关依赖:
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.0.2.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.0.2.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>5.0.3.RELEASE</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.11</version>
</dependency>
</dependencies>
创建 Account 实体类:
/**
@Author: Ly
@Date: 2020-08-04 23:38
*/
public class Account implements Serializable {
private Integer id;
private String name;
private Float money;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Float getMoney() {
return money;
}
public void setMoney(Float money) {
this.money = money;
}
@Override
public String toString() {
return "Account{" +
"id=" + id +
", name='" + name + ''' +
", money=" + money +
'}';
}
}
基于 spring 内置数据源的 JdbcTemplate 入门案例:
/**
@Author: Ly
@Date: 2020-08-04 23:43
*/
public class JdbcTemplateDemo1 {
public static void main(String[] args) {
//准备数据源:spring 的内置数据源
DriverManagerDataSource ds=new DriverManagerDataSource();
ds.setDriverClassName("com.mysql.cj.jdbc.Driver");
ds.setUrl("jdbc:mysql://localhost:3306/spring?serverTimezone=GMT%2B8&useUnicode=true&characterEncoding=utf-8&useSSL=false");
ds.setUsername("root");
ds.setPassword("123456");
//1.创建对象 JdbcTemplate
//JdbcTemplate jt=new JdbcTemplate(ds);
JdbcTemplate jt=new JdbcTemplate();
//给 jt 设置数据源
jt.setDataSource(ds);
//2.执行操作
jt.execute("insert into account(name,money)values('ccc',1000)");
}
}
配置 bean.xml 文件:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="accountDao" class="com.ly.dao.impl.AccountDaoImpl">
<property name="jdbcTemplate" ref="jdbcTemplate"></property>
</bean>
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="dataSource"></property>
</bean>
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.cj.jdbc.Driver"></property>
<property name="url" value="jdbc:mysql://localhost:3306/spring?serverTimezone=GMT%2B8&useUnicode=true&characterEncoding=utf-8&useSSL=false"></property>
<property name="username" value="root"></property>
<property name="password" value="123456"></property>
</bean>
</beans>
测试代码:
/**
@Author: Ly
@Date: 2020-08-04 23:43
*/
public class JdbcTemplateDemo2 {
public static void main(String[] args) {
//1.获取容器
ApplicationContext ac=new ClassPathXmlApplicationContext("bean.xml");
//2.获取对象
JdbcTemplate jt=ac.getBean("jdbcTemplate",JdbcTemplate.class);
//2.执行操作
jt.execute("insert into account(name,money)values('dsa',1000)");
}
}
//保存操作
jt.update("insert into account(name,money)values(?,?)","eee",2222);
//更新操作
jt.update("update account set name=?,money=? where id=?","test",4567,1);
//删除操作
jt.update("delete from account where id=?",8);
//查询所有操作
List<Account> accounts=jt.query("select * from account where money > ?",new BeanPropertyRowMapper<Account>(Account.class),1000f);
//查询一个操作
List<Account> account=jt.query("select * from account where id = ?",new BeanPropertyRowMapper<Account>(Account.class),1);
//查询所有一行一列(使用聚合函数,但不加 group by 语句)
Integer count=jt.queryForObject("select count(*) from account where money>?",Integer.class,1000f);
在执行查询操作时,我们使用了 BeanPropertyRowMapper,是由 JdbcTemplate 提供的一个返回自定义对象的一个类,我们先看一下 query 方法:public <T> List<T> query(String sql, RowMapper<T> rowMapper)
,BeanPropertyRowMapper 实现了 RowMapper 接口用来对数据进行封装。
自定义 AccountRowMapper 类实现封装账户信息。
List<Account> accounts=jt.query("select * from account where money > ?",new AccountRowMapper(),1000f);
class AccountRowMapper implements RowMapper<Account>{
/**
把结果集中的数据封装到 Account 中,然后由 spring 把每个 Account 加到集合中
@param rs
@param rowNum
@return
@throws SQLException
*/
@Override
评论