C 语言中如何输出汉字 ; 如何用 C 语言汉字编码输出汉字 (超全版)
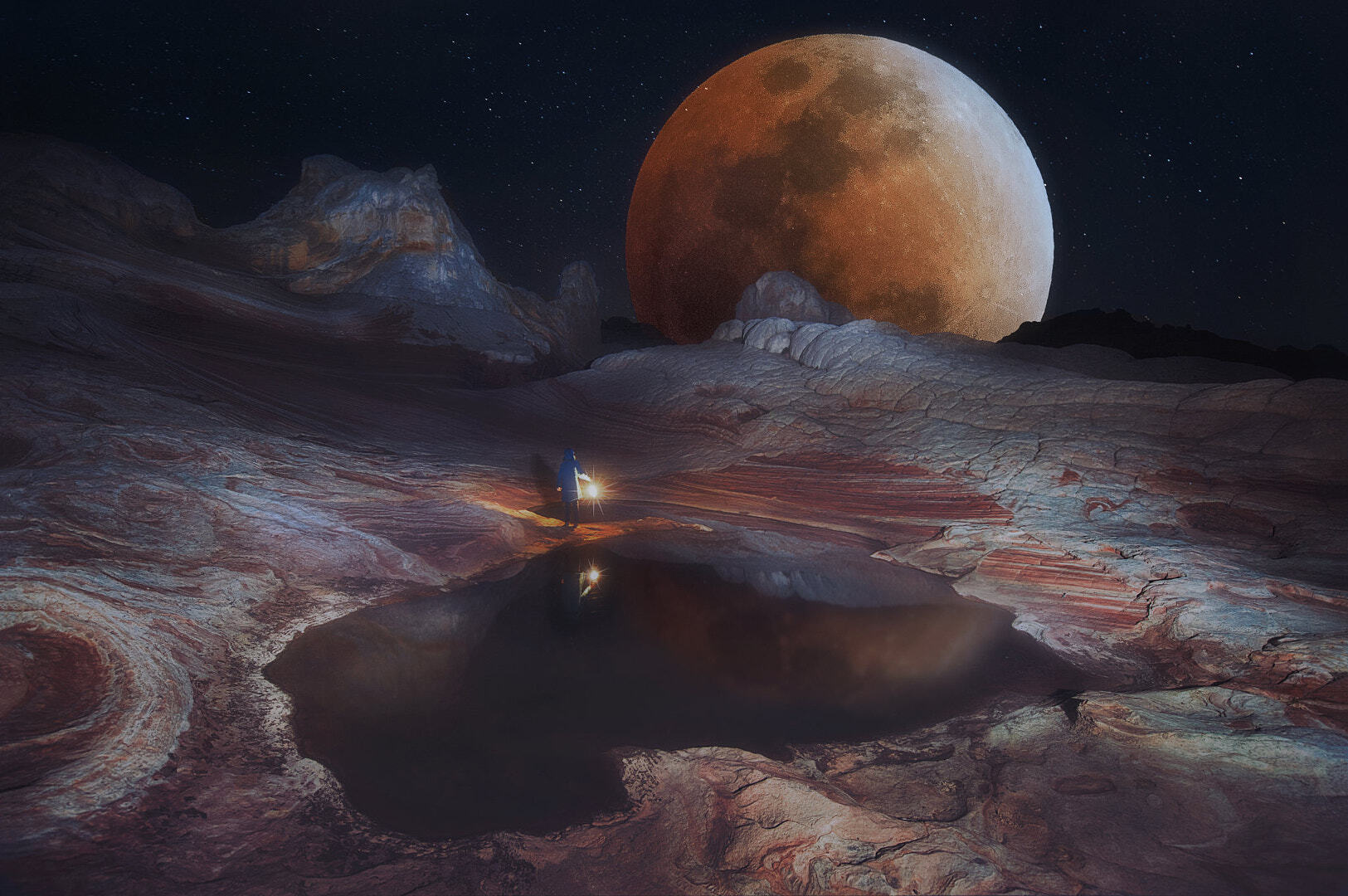
[TOC]
## 前景提要
想用 char 类型存储中文,然后打印出来
方式一:
使用 char [] 数组的方式打印,然后,因为一个汉子两个字节,所以,打印时候,需要两个 %c
实例
复制代码
存在问题: for 循环的次数必须写死跟元素的个数一样,不能随便修改,否则,打印的时候,结果就会有乱码的情况.
图一
解决方法:优化
复制代码
** 完整**
复制代码
方式二:
指针方式改写
1. 数组方式打印
实例
复制代码
2. 指针方式打印
实例
复制代码
<font color =red> 注意</font>
不能写成如下形式,因为字符是一个字节,而汉子是两个,写成如下是无法输出的.
复制代码
3. 优化为 while 方式
实例
复制代码
方式三:
结构体数组改写
1. 使用结构体内数组方式
实例
复制代码
2. 使用结构体内数组指针方式
(1) 基础写法
实例
复制代码
(2) 升级写法,指针的优化,去除一个 for 循环
复制代码
实例
复制代码
(3) 修改打印为指针打印
实例
复制代码
(4) 优化打印结果中的乱码
for 循环次数变化的时候,会出现乱码
复制代码
实例
复制代码
(5) 优化循环,减少循环次数,同时,可以很好的解决上一个 for 存在的乱码问题.
实例
复制代码
## 总结
打印汉字的方法确实比简单的输出'a','b''c'复杂了很多,想到很多情况
结构体指针这里确实有很多问题,不断的在改进
汉字的乱码过滤方式确实想了很久,跟之前的过滤方式不同,之前的方式请看<a href="https://www.cnblogs.com/liuyangfirst/p/15965722.html">c 语言怎么避免打印空数据?</a>
本博主遇到的这个问题能帮助到你,喜欢的话,请关注,点赞,收藏.
版权声明: 本文为 InfoQ 作者【北极的大企鹅】的原创文章。
原文链接:【http://xie.infoq.cn/article/b6ef86bae6970d179a4377ef9】。未经作者许可,禁止转载。
评论