大厂算法面试之 leetcode 精讲 15. 链表
大厂算法面试之 leetcode 精讲 15.链表
视频讲解(高效学习):点击学习
目录:
链表操作如下图:
时间复杂度:
prepend:
O(1)
append: 如果已知尾节点
O(1)
,否则需要遍历到尾节点,然后加入新节点O(n)
insert: 插入到已知节点的后面
O(1)
,需要先查找后插入O(n)
lookup:
O(n)
Delete:删除已知节点
O(1)
,需要先查找后删除O(n)
206. 反转链表(easy)
方法 1.头插法:
思路:准备一个临时节点,然后遍历链表,准备两个指针 head 和 next,每次循环到一个节点的时候,将
head.next
指向temp.next
,并且将temp.next
指向 head,head 和 next 向后移一位。复杂度分析:时间复杂度:
O(n)
, n 为链表节点数,空间复杂度:O(1)
js:
方法 2.迭代法:
思路: 遍历链表,准备 prev,curr,next 三个指针,在遍历的过程中,让当前指针
curr.next
指向前一个指针 prev,然后不断让 prev,curr,next 向后移动,直到 curr 为 null复杂度分析:时间复杂度:
O(n)
, n 为链表节点数,空间复杂度:O(1)
js:
java:
方法 3.递归:
思路:用递归函数不断传入
head.next
,直到head==null
或者heade.next==null
,到了递归最后一层的时候,让后面一个节点指向前一个节点,然后让前一个节点的 next 置为空,直到到达第一层,就是链表的第一个节点,每一层都返回最后一个节点。复杂度分析:时间复杂度:
O(n)
,n 是链表的长度。空间复杂度:O(n)
, n 是递归的深度,递归占用栈空间,可能会达到 n 层
js:
Java:
92. 反转链表 II(medium)
方法 1
思路:切断 left 到 right 的子链,然后反转,最后在反向连接
复杂度:时间复杂度
O(n)
,空间复杂度O(1)
js:
java:
方法 2
思路:从 left 遍历到 right,在遍历的过程中反转链表
复杂度:时间复杂度
O(n)
,空间复杂度O(1)
js:
java:
24. 两两交换链表中的节点 (medium)
方法 1.递归:
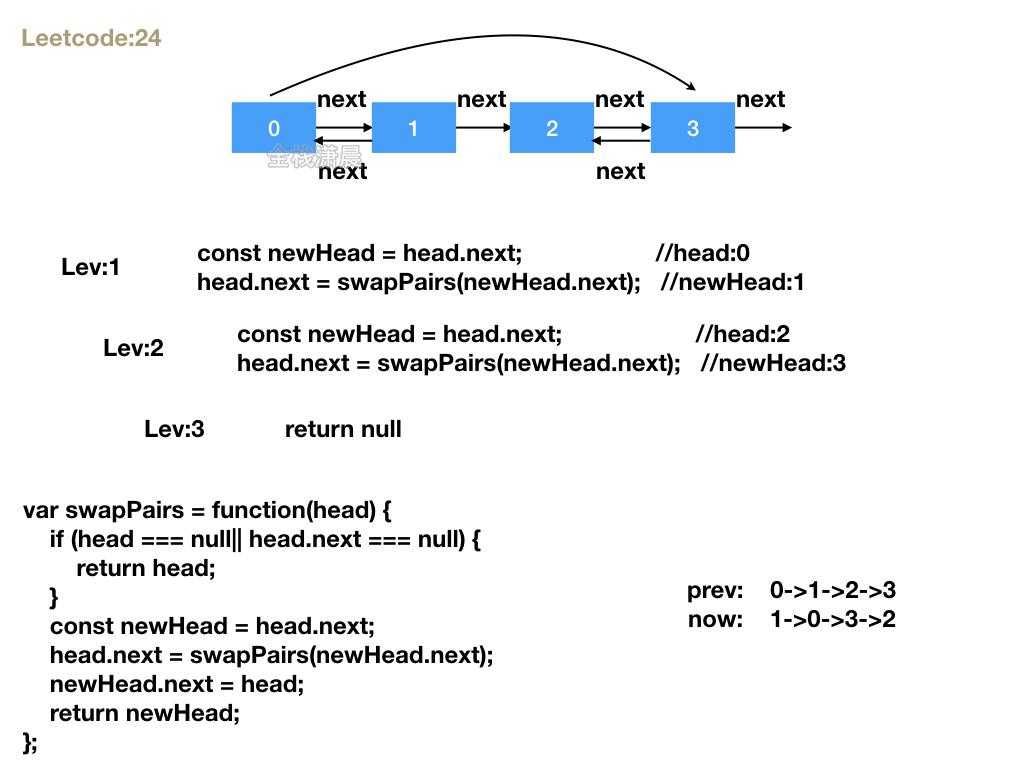
思路:用递归函数不断传入链表的下一个节点,终止条件是
head === null|| head.next === null
,也就是至少存在两个节点进行两两交换,在最后一层的时候开始两两反转,让当前递归层的head.next
指向交换后返回的头节点,然后让反转后的新的头节点指向当前层的 head 的节点,这样就实现了两两交换,最后返回反转后链表的头节点复杂的分析:时间复杂度
O(n)
, n 是链表的节点数量。空间复杂度O(n)
,n
是递归调用的栈空间
js:
Java:
方法 2.循环(虚拟头节点)
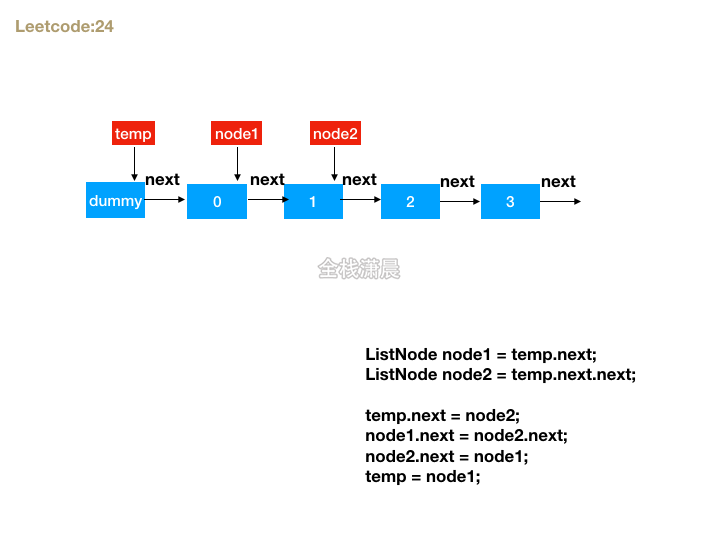
思路:设置虚拟头节点 dummyHead,让
dummyHead.next
指向 head,当temp.next !== null && temp.next.next !== null
的时候,也就是 dummyHead 后面存在至少两个节点,才开始两两交换节点。交换之前准备三个指针 temp 指向 dummyHead,node1 是 dummyHead 后面的第一个节点,node2 是 dummyHead 后的第二个节点,交换的时候让temp.next
指向 node2,node1.next
指向node2.next
,node2.next
指向 node1,每次循环迭代让这三个节点后移一个节点,最后返回dummyHead.next
,核心步骤是
复杂的分析:时间复杂度
O(n)
, n 是链表的节点数量。空间复杂度O(1)
,
Js:
Java:
146. LRU 缓存机制 (medium)
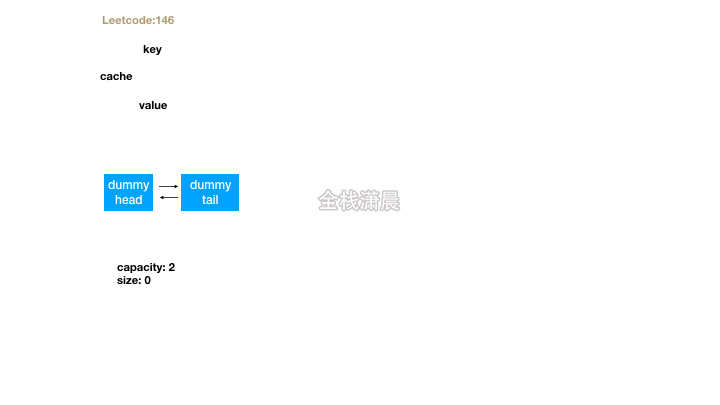
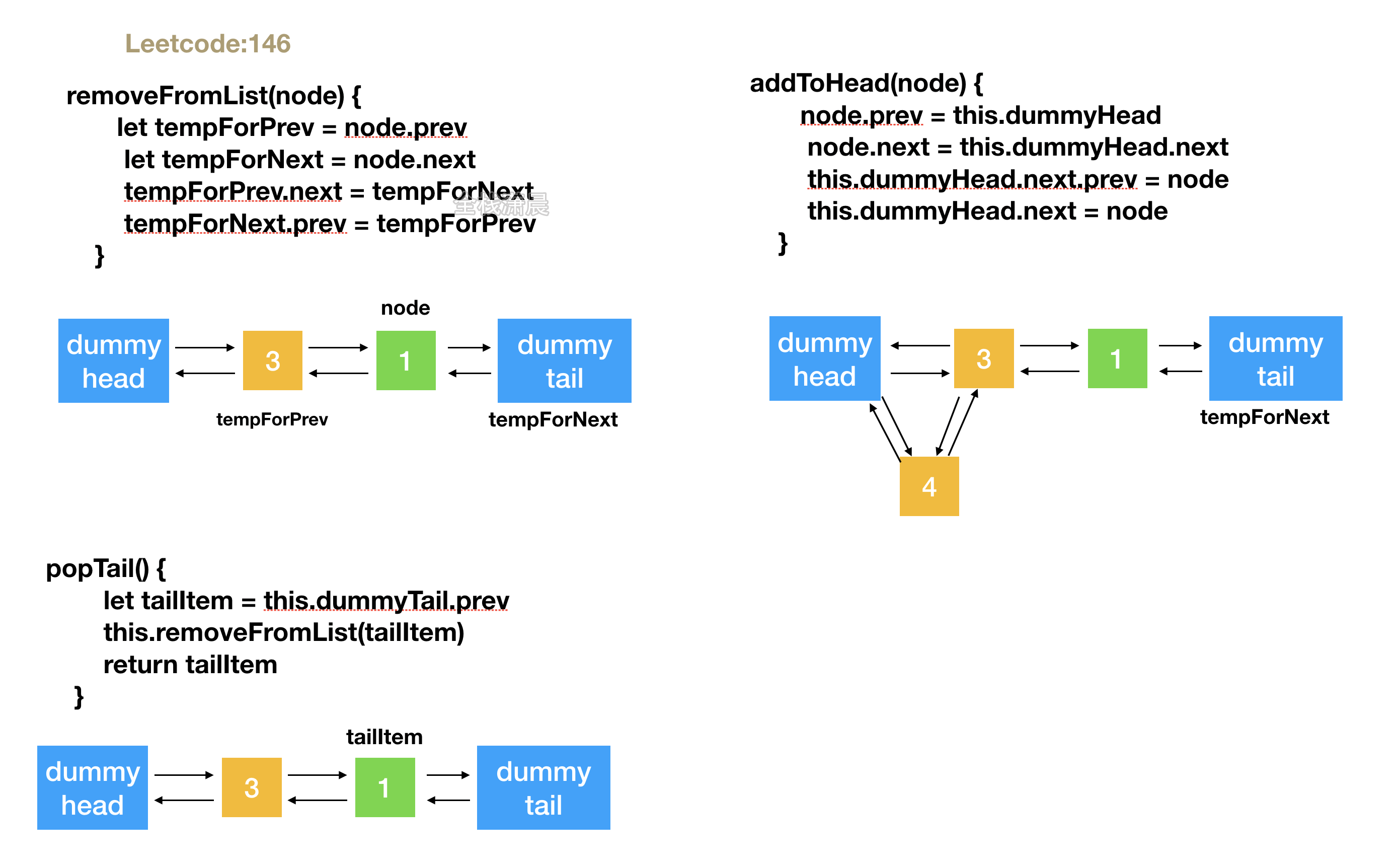
思路:准备一个哈希表和双向链表存储键值对,哈希表 O(1)就能查找到键值对,双向链表方便从链表头部新增节点,也可以从队尾删除节点
get 的时候,查找哈希表中有没有该键值对,不存在就返回-1,存在就返回该节点的值,并且将该节点移动到链表的头部
put 的时候,查找哈希表中有没有该键值对,如果存在就更新该节点,并且移动到链表的头部,不存在就创建一个节点,加入到哈希表和链表的头部,并且让节点数
count+1
,如果超出容量,就从队尾删除一个节点复杂度:put、get 时间复杂度都是
O(1)
,空间复杂度O(c)
,c 是 LRU 的容量
js:
Java:
237. 删除链表中的节点(easy)
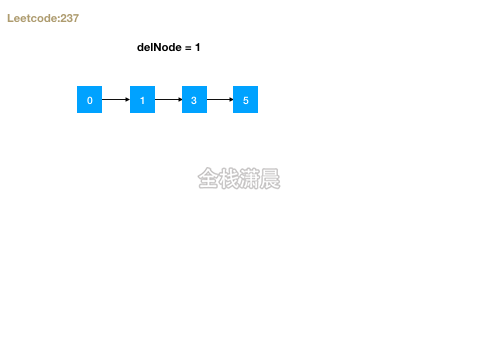
思路:将要删除节点的下一个节点的值覆盖自己的值,然后让当前节点指向下一个节点的 next
复杂度:时间复杂度和空间复杂度都是
O(1)
js:
java;
19. 删除链表的倒数第 N 个结点 (medium)
方法 1:栈
思路:循环链表,将所有的节点入栈,然后在弹出栈 n 次,就是我们需要删除的节点
复杂度:时间复杂度
O(L)
,L 是链表的长度,空间复杂度O(L)
。
方法 2:遍历 2 次
思路:遍历一次链表的到链表的长度 L,在重头遍历到
L-n+1
的位置就是需要删除的节点。复杂度:时间复杂度
O(L)
,L 是链表的长度,空间复杂度O(1)
方法 3:遍历 1 次
思路:新建 dummy 节点指向 head,指针 n1,n2 指向 head,循环 n2 指针到 n 的位置,然后在同时移动 n1,n2,直到结尾,n1,n2 的距离是 n,此时 n1 的位置就是需要删除元素的位置
复杂度:时间复杂度
O(L)
,L 是链表的长度,空间复杂度O(1)
js:
java:
203. 移除链表元素 (easy)
方法 1.递归
思路:递归调用函数 removeElements,传入
head.next
和 val,如果当前元素值是 val,则返回下一个元素,否则直接返回当前元素复杂度:时间复杂度
O(n)
,n 是链表的长度,空间复杂度是O(n)
,递归栈的深度,最大为 n
js:
java:
方法 2.迭代
思路:创建 dummy 节点,将 dummy 节点的 next 指向 head,temp 指向 dummy,当 temp 的 next 不为 null 不断移动 temp 指针,当 temp 的 next 值是要删除的 则删除该节点
复杂度:时间复杂度
O(n)
,n 是链表的长度,空间复杂度是O(1)
js:
java:
2. 两数相加 (medium)
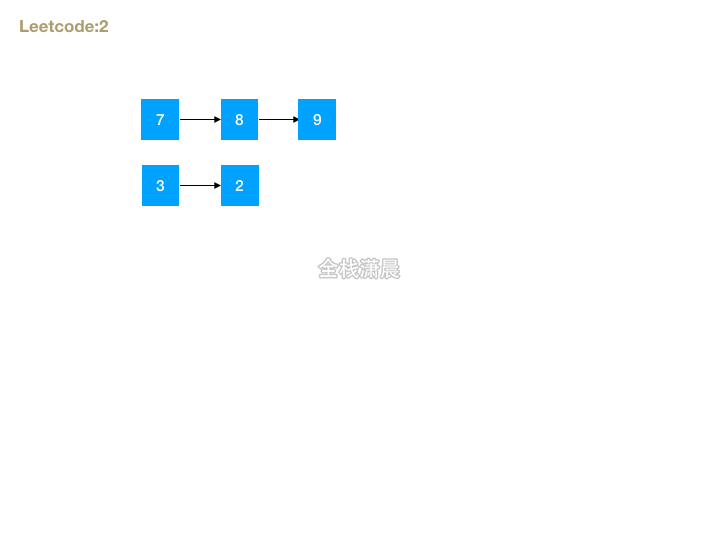
思路:循环两个链表,计算每个节点相加的和在加进位,然后计算进位,处理最后一次的进位。
复杂度:时间复杂度
O(max(m,n))
,循环的次数是链表较长的那个。空间复杂度O(1)
js:
java:
21. 合并两个有序链表 (easy)
方法 1.递归
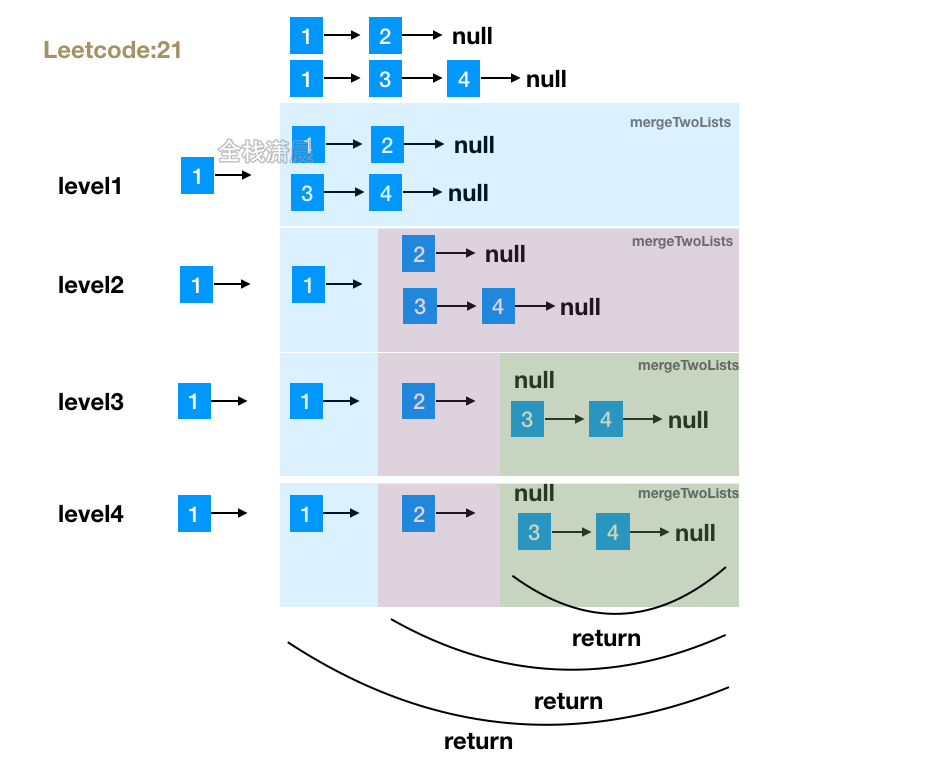
思路:递归合并节点,当前节点谁小,就让这个较小的节点的 next 和另一个链表继续递归合并,直到两个链表有一个的 nxet 不存在了,那就没法分割问题了,只能返回
复杂度:时间复杂度
O(m+n)
,m、n 为两个链表的长度,每次递归排除掉一个节点,总递归次数是m+n
。空间复杂度O(m+n)
,递归栈空间
js:
java:
方法 2.迭代
思路:设立虚拟头节点 prehead,prev 节点初始指向 prehead,循环两个链表,两个链表中小的节点接在 prev 的后面,不断移动 prev,最后返回
prehead.next
复杂度:时间复杂度
O(m+n)
,m、n 为两个链表的长度,循环m+n
次。空间复杂度O(1)
js:
java:
83. 删除排序链表中的重复元素 (easy)
时间复杂度:O(n)
。空间复杂度O(1)
js:
java:
328. 奇偶链表 (medium)
思路:奇偶指针循环链表,奇数指针不断串连奇数节点,偶数指针不断串连偶数节点,最后奇数指针的结尾连接偶数节点的开始
复杂度:时间复杂度
O(n)
,空间复杂度O(1)
js:
java:
评论