Vue 进阶(三):Axios 应用详解
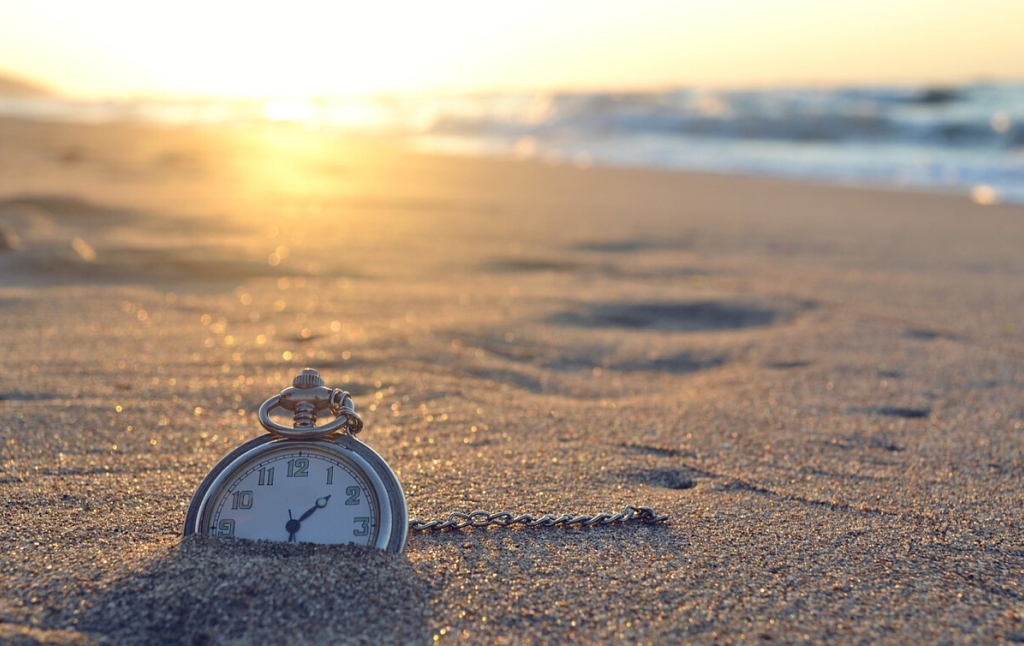
一、前言
Axios
是基于 promise
的 HTTP
库,可以用在浏览器和 node.js
中。
二、功能特性
在浏览器中发送
XMLHttpRequests
请求;在
node.js
中发送http
请求;支持
Promise API
;拦截请求和响应;
转换请求和响应数据;
自动转换
JSON
数据;客户端支持保护安全免受
XSRF
攻击;浏览器支持;
三、安装
使用 bower
:
使用 npm
:
四、配置项与响应结构
4.1 配置项
可用的请求配置项如下,只有 url
是必需的。如果没有指定 method
,默认请求方法是 GET
。
4.2 响应的数据结构
响应的数据包括下面的信息:
当使用 then
或者 catch
时, 会收到下面的响应:
五、示例
5.1 发送 GET 请求
5.2 发送 POST 请求
5.3 发送多个并发请求
处理并发请求方法如下:
可以通过给 axios
传递对应的参数来定制请求:
六、请求方法别名
为方便起见,axios
为所有支持的请求方法都提供了别名。
注意: 当使用别名方法时,
url
、method
和data
属性不需要在config
参数里面指定。
七、默认配置
可以为每一个请求指定默认配置。
7.1 全局 axios 默认配置
7.2 自定义实例默认配置
7.3 配置的优先顺序
Config will be merged with an order of precedence. The order is library defaults found in lib/defaults.js, then defaults property of the instance, and finally config argument for the request. The latter will take precedence over the former. Here's an example.
八、拦截器
8.1 添加拦截器
可以在处理 then
或 catch
之前拦截请求和响应。
8.2 移除一个拦截器
也可以给自定义的 axios
实例添加拦截器:
错误处理
九、Promises
axios
依赖原生 ES6 Promise
实现,如果浏览器环境不支持 ES6 Promises
,需要引入 polyfill
。
9.1 TypeScript
axios
包含一个 TypeScript
定义。
Credits
axios is heavily inspired by the http-like service for use outside of Angular.
版权声明: 本文为 InfoQ 作者【华强】的原创文章。
原文链接:【http://xie.infoq.cn/article/a7f14f28fb2a662cbd7fea48f】。文章转载请联系作者。
评论