Java 高级特性之 IO 流 (1),三面蚂蚁金服(交叉面)定级阿里 P6
fw.write(cbuf,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
//4.关闭流资源。两种方式都可以
//方式一:
// try {
// if(fw != null)
// fw.close();
// } catch (IOException e) {
// e.printStackTrace();
// }finally{
// try {
// if(fr != null)
// fr.close();
// } catch (IOException e) {
// e.printStackTrace();
// }
// }
//方式二:
try {
if(fw != null)
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
try {
if(fr != null)
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
3.2 FileInputStream 类、FileOutputStream 类
import org.junit.Test;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class FileInputOutputStream {
/*需求:将 test/resources 下的 test.png 使用字节流的方式复制到该文件夹下,重命名为 dest.png
分析:
1)将将一些固定的代码抽离出来,将变化的东西放在方法的形参内,将不变的东西放进方法体。
2)不能用字节流来处理文本数据,因为对于英文来说,每一个字符占有一个字节,所以不会有影响。
但是一个中文字符在 UTF-8 编码下是占用三个字符的,所以会出现乱码。
*/
@Test
public void testCopyFile(){
long start = System.currentTimeMillis();
String srcPath = "src/test/resources/test.png";
String destPath = "src/test/resources/dest.png";
copyFile(srcPath,destPath);
//用于测试所用时间
long end = System.currentTimeMillis();
System.out.println("复制操作花费的时间为:" + (end - start));//618
}
public void copyFile(String srcPath,String destPath){
FileInputStream fis = null;
FileOutputStream fos = null;
try {
//
File srcFile = new File(srcPath);
File destFile = new File(destPath);
//
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile);
//复制的过程
byte[] buffer = new byte[1024];
int len;
while((len = fis.read(buffer)) != -1){
fos.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fos != null){
//
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fis != null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
========================================================================
它的作用提高流的读取、写入的速度,之所以可以提高读写速度的原因是因为其内部提供了一个缓冲区。
真正开发的时候是不太会用到节点流的。
当读取数据时,数据按块读入缓冲区,其后的读操作则直接访问缓冲区;
当使用 BufferedInputStream 读取字节文件时, BufferedInputStream 会一次性从文件中读取 8192 个 (8Kb)字节存放在缓冲区中,直到缓冲区装满 了才会重新从文件中读取下一个 8192 个字节数组;
向流中写入字节时,不会直接写到文件,而是先写到缓冲区中,直到缓冲区写满,然后 BufferedOutputStream 会把缓冲区中的数据一次性写到文件 里 。不过也可以通过使用方法 flush() 可以强制手动将缓冲区的内容全部写入输出流;
关闭流的顺序和打开流的顺序是相反的。同时只要关闭最外层处理流就会相应关闭内层节点流。
如果是带缓冲区的流对象的 close() 方法,不但会关闭流, 还会在关闭 流之前刷新缓冲区,关闭后不能再写出。
因为缓冲流下对应的字节流和字符流的操作相同,所以下面只介绍针对字节流的缓冲流。
import org.junit.Test;
import java.io.*;
/**
处理流之一:缓冲流的使用
*/
public class BufferedTest {
@Test
public void testCopyFileWithBuffered(){
long start = System.currentTimeMillis();
String srcPath = "src/test/resources/test.png";
String destPath = "src/test/resources/dest.png";
copyFileWithBuffered(srcPath,destPath);
long end = System.currentTimeMillis();
System.out.println("复制操作花费的时间为:" + (end - start));//618 - 176
}
//实现文件复制的方法
public void copyFileWithBuffered(String srcPath,String destPath){
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
//1.造文件
File srcFile = new File(srcPath);
File destFile = new File(destPath);
//2.造流
//2.1 造节点流
FileInputStream fis = new FileInputStream((srcFile));
FileOutputStream fos = new FileOutputStream(destFile);
//2.2 造缓冲流
bis = new BufferedInputStream(fis);
bos = new BufferedOutputStream(fos);
//3.复制的细节:读取、写入
byte[] buffer = new byte[1024];
int len;
while((len = bis.read(buffer)) != -1){
bos.write(buffer,0,len);
// buf.flush();//就是刷新缓冲区,这样可以提高写入的速度,但是在 write 中实际上已经集成了 flush,所以这里可以不写
}
} catch (IOException e) {
e.printStackTrace();
} finally {
//4.资源关闭
//要求:先关闭外层的流,再关闭内层的流
if(bos != null){
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(bis != null){
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
//说明:关闭外层流的同时,内层流也会自动的进行关闭。关于内层流的关闭,我们可以省略.
// fos.close();
// fis.close();
}
}
}
========================================================================
转换流提供了字节流和字符流之间的转换,很多时候我们使用转换流来处理文件乱码问题,也可实现编码和解码的功能。
编码:字节、字节数组 → 字符数组、字符串
解码:字符数组、字符串 → 字节、字节数组
转换流的编解码应用:
可以将字符按指定编码格式存储
可以对文本数据按指定解码格式来解读
指定编码表的动作由构造器完成
在 Unicode 国际标准码出现之前,所有的字符集都是和具体编码方案绑定在一起的,即字符集=编码方式。这直接将字符和最终字节流绑定死了。例如面向中文的 GBK 编码,就是使用利用两个字节编码所有中文字符,同时,通过判断每一个字节的首部的 0 或 1 来判断该字节是否与后一个字节相连。
Unicode 国际标准码,就是利用两个字节将目前人类使用的所有字符都包括进去,然而数量庞大,已经不能像 GBK 那样空出两个字节的头部。所以,Unicode 只是定义了一个庞大的、全球通用的字符集,**并为每个字符规定了唯一确定的编号,具体存储成什么样的字节流,取
决于字符编码方案**。
推荐的 Unicode 编码是 UTF-8 和 UTF-16 。UTF-8 就是每次 8 个位传输数据,而 UTF 16 就是每次 16 个位。之所以这些最常用,是因为一个字节是 8 位的。
下面做一个示例:
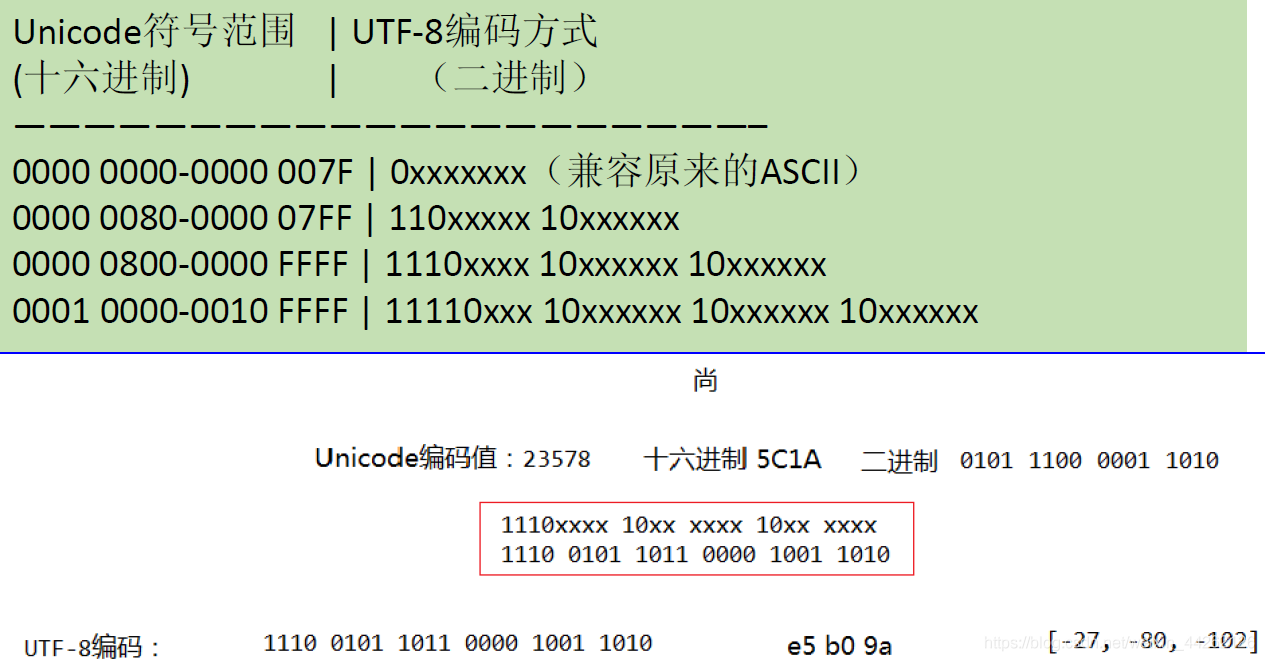
“尚”这个字的 Unicode 编码值是 23578(十进制),对应的二进制为 0101 1100 0001 1010,因为其十六进制的范围是在 0000 0800-0000 FFFF 之间,所以对应的 UTF-8 编码方式是三位的,如上如所示,将对应的二进制放在 UTF-8 的编码方式里面,得到如上所示。
通过该图片也可以发现因为字母的 Ascii 值在 128 以下,而 2 的 7 次方是 128,所以完全可以将全部的字母涵盖进去,所以所以一个 byte 在 UTF-8 下可以对应一个字母。
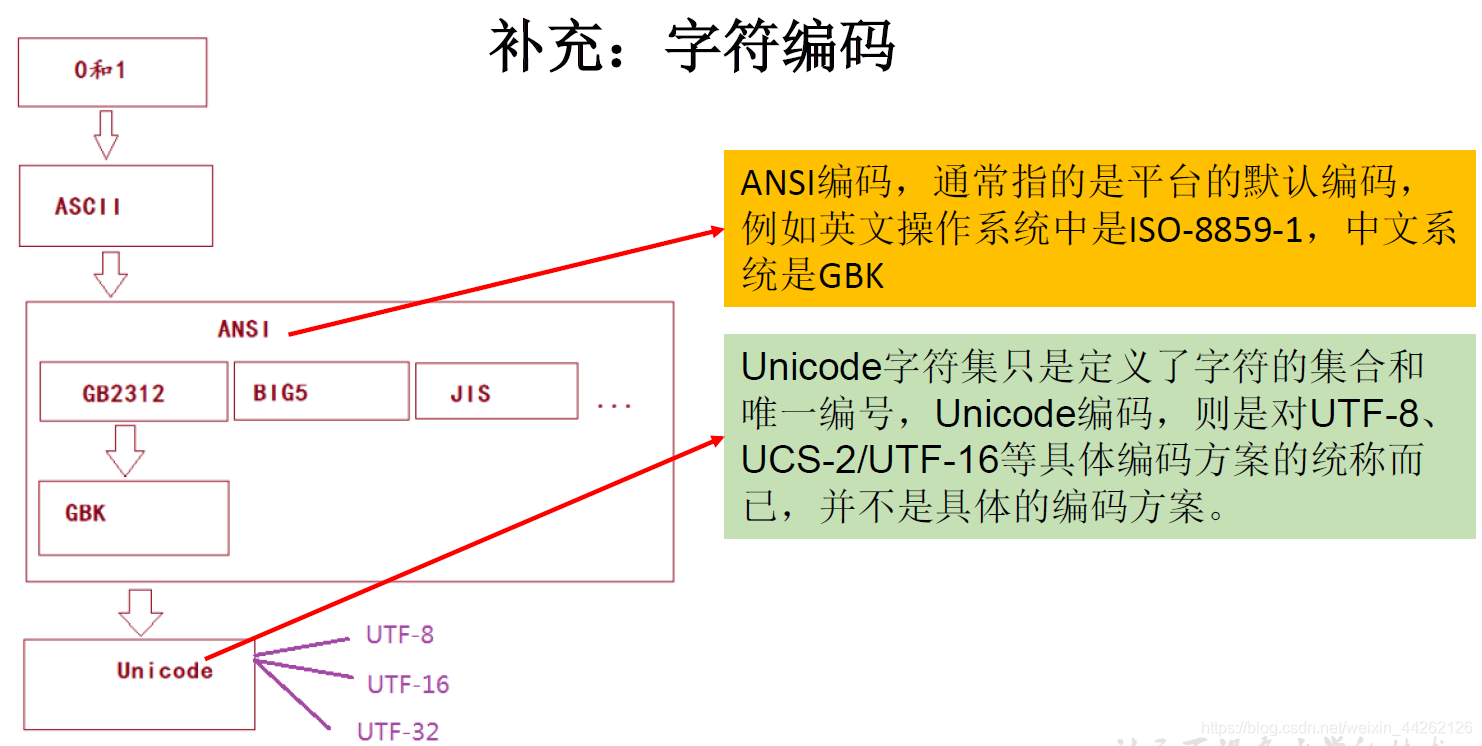
InputStreamReader :将 InputStream 转换为 Reader
OutputStreamWriter :将 Writer 转换为 OutputStream
/*
需求:第一个是转换流
*/
@Test
public void testTransformStream() throws Exception{
//1.造文件、造流
File file1 = new File("src/test/resources/helloworld.txt");
File file2 = new File("src/test/resources/hellowrite.txt");
FileInputStream fis = new FileInputStream(file1);
FileOutputStream fos = new FileOutputStream(file2);
InputStreamReader isr = new InputStreamReader(fis, StandardCharsets.UTF_8);
OutputStreamWriter osw = new OutputStreamWriter(fos,"gbk");
//2.读写过程
char[] cbuf = new char[20];
int len;
while((len = isr.read(cbuf)) != -1){
osw.write(cbuf,0,len);
}
//3.关闭资源
isr.close();
osw.close();
}
========================================================================
System.in 和 System.out 分别代表了系统标准的输入和输出设备,默认的输入设备是键盘,输出设备是显示器,当然可以通过重定向:通过 System 类的 setIn setOut 方法对默认设备进行改变。
System.in 的类型是 InputStream
System.out 的类型是 PrintStream
//输出重定向
public static void setIn(InputStream in)
public static void setOut(PrintStream out)
/*
标准输入流与输出流
需求:从键盘输入字符串,要求将读取到的整行字符串转成大写输出。然后继续进行输入操作,
直至当输入“e”或者“exit”时,退出程序。
分析:
System.in:标准的输入流,默认从键盘输入
System.out:标准的输出流,默认从控制台输出
System 类的 setIn(InputStream is) / setOut(PrintStream ps)方式重新指定输入和输出的流。
*/
class testSystemInOut{
public static void main(String[] args) {
BufferedReader br = null;
try {
InputStreamReader isr = new InputStreamReader(System.in);
br = new BufferedReader(isr);
while (true) {
System.out.println("请输入字符串:");
String data = br.readLine();
if ("e".equalsIgnoreCase(data) || "exit".equalsIgnoreCase(data)) {
System.out.println("程序结束");
break;
}
String upperCase = data.toUpperCase();
System.out.println(upperCase);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (br != null) {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
打印流的目的是实现将基本数据类型的数据格式转化为字符串输出。 打印流常常和标准输入、输出流一起使用。
代码演示:
/*
需求:将循环中的 ASCII 码对应的字符输出保存到文件中。PrintStream 是处理流,FileOutputStream 是节点流。
打印流常常和标准输入输出流的 SetOut 和 SetIn 一起使用。
*/
@Test
public void testPrintStream(){
PrintStream ps = null;
try {
FileOutputStream fos = new FileOutputStream(new File("D:\IO\text.txt"));
// 创建打印输出流,设置为自动刷新模式(写入换行符或字节 '\n' 时都会刷新输出缓冲区)
ps = new PrintStream(fos, true);
if (ps != null) {// 把标准输出流(控制台输出)改成文件
//setOut()方法用于重新指定一个打印流
System.setOut(ps);
}
for (int i = 0; i <= 255; i++) { // 输出 ASCII(i)码对应的字符
System.out.print((char) i);
if (i % 50 == 0) { // 每 50 个数据一行
System.out.println(); // 换行
}
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} finally {
if (ps != null) {
ps.close();
}
}
}
DataInputStream 和 DataOutputStream。为了方便地操作 Java 语言的基本数据类型和 String 的数据,可以使用数据流。
/*
需求:将内存中的字符串、基本数据类型的变量写出到文件中,然后将文件中存储的基本数据类型变量和字符串读取到内存中,保存在变量中。
注意点:读取不同类型的数据的顺序要与当初写入文件时,保存的数据的顺序一致!
*/
@Test
public void testDataStream() throws IOException{
//1.
DataOutputStream dos = new DataOutputStream(new FileOutputStream("src/test/resources/datastream.txt"));
//2.保存的文件不能直接双击打开.txt 文件来读取,而是使用 DataInputStream 来读,不然是会乱码的。
dos.writeUTF("刘建辰");
dos.flush();//刷新操作,将内存中的数据写入文件
dos.writeInt(23);
dos.flush();
dos.writeBoolean(true);
dos.flush();
//3.
dos.close();
//1.
DataInputStream dis = new DataInputStream(new FileInputStream("src/test/resources/datastream.txt"));
//2.
String name = dis.readUTF();
int age = dis.readInt();
boolean isMale = dis.readBoolean();
System.out.println("name = " + name);
System.out.println("age = " + age);
System.out.println("isMale = " + isMale);
//3.关闭流
dis.close();
}
========================================================================
ObjectInputStream 和 OjbectOutputSteam 对象流的作用是存储和读取基本数据类型数据或对象。它的强大之处就是可以把 Java 中的对象写入到数据源中,也能把对象从数据源中还原回来。
序列化: 用 ObjectOutputStream 类保存基本类型数据或对象的机制
反序列化: 用 ObjectInputStream 类读取基本类型数据或对象的机制
注意:ObjectOutputStream 和 ObjectInputStream 不能序列化 static 和 transient 修饰的成员变量。
对象的序列化机制:
评论