给我一起学 jdbc 之 sql 注入
作者:楠羽
- 2022 年 9 月 07 日 福建
本文字数:3495 字
阅读完需:约 11 分钟
📦个人主页:楠羽
🏆简介:一个大二的科班出身的,主要研究 Java 后端开发
⏰座右铭:成功之前我们要做应该做的事情,成功之后才能做我们喜欢的事
💕 过客的你,可以给博主留下一个小小的关注吗?这是给博主最大的支持。以后博主会更新大量的优质的作品!!!!
SQL 注入攻击
1.sql 注入攻击的演示
在登录界面,输入一个错误的用户名或密码,也可以登录成功
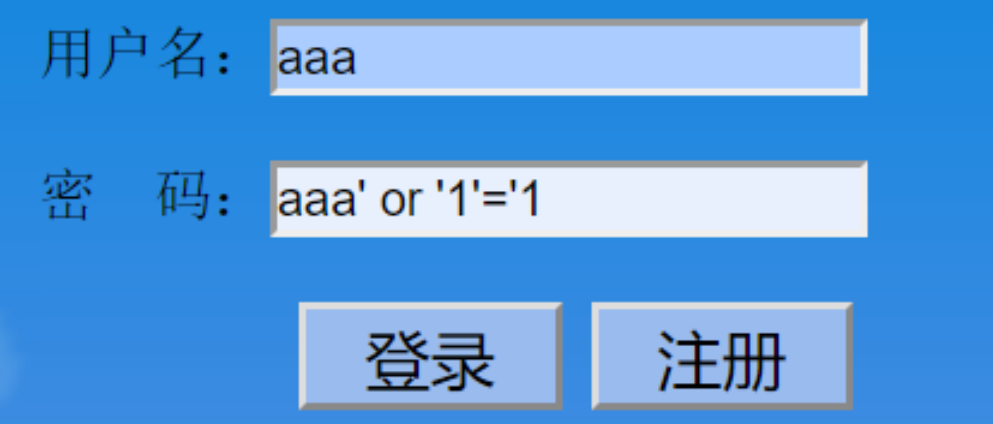
2.sql 注入攻击的原理
按照正常道理来说,我们在密码处输入的所有内容,都应该认为是密码的组成
但是现在 Statement 对象在执行 sql 语句时,将一部分内容当做查询条件来执行了
3.PreparedStatement 的介绍
预编译 sql 语句的执行者对象。在执行 sql 语句之前,将 sql 语句进行提前编译。明确 sql 语句的格式后,就不会改变了。剩余的内容都会认为是参数!参数使用?作为占位符
为参数赋值的方法:setXxx(参数 1,参数 2);
参数 1:?的位置编号(编号从 1 开始)
参数 2:?的实际参数
执行 sql 语句的方法
执行 insert、update、delete 语句:int executeUpdate();
执行 select 语句:ResultSet executeQuery();
4.PreparedStatement 的使用
/*
使用PreparedStatement的登录方法,解决注入攻击
*/
@Override
public User findByLoginNameAndPassword(String loginName, String password) {
//定义必要信息
Connection conn = null;
PreparedStatement pstm = null;
ResultSet rs = null;
User user = null;
try {
//1.获取连接
conn = JDBCUtils.getConnection();
//2.创建操作SQL对象
String sql = "SELECT * FROM user WHERE loginname=? AND password=?";
pstm = conn.prepareStatement(sql);
//3.设置参数
pstm.setString(1,loginName);
pstm.setString(2,password);
System.out.println(sql);
//4.执行sql语句,获取结果集
rs = pstm.executeQuery();
//5.获取结果集
if (rs.next()) {
//6.封装
user = new User();
user.setUid(rs.getString("uid"));
user.setUcode(rs.getString("ucode"));
user.setUsername(rs.getString("username"));
user.setPassword(rs.getString("password"));
user.setGender(rs.getString("gender"));
user.setDutydate(rs.getDate("dutydate"));
user.setBirthday(rs.getDate("birthday"));
user.setLoginname(rs.getString("loginname"));
}
//7.返回
return user;
}catch (Exception e){
throw new RuntimeException(e);
}finally {
JDBCUtils.close(conn,pstm,rs);
}
}
复制代码
5.使用 PreparedStatement 优化 student 表的 CRUD(作业)
public class StudentDaoImpl implements StudentDao {
@Override
public ArrayList<Student> findAll() {
//定义必要信息
Connection conn = null;
PreparedStatement pstm = null;
ResultSet rs = null;
ArrayList<Student> students = null;
try {
//1.获取连接
conn = JDBCUtils.getConnection();
//2.获取操作对象
pstm = conn.prepareStatement("select * from student");
//3.执行sql语句,获取结果集
rs = pstm.executeQuery();
//4.遍历结果集
students = new ArrayList<Student>();
while (rs.next()) {
//5.封装
Student student = new Student();
student.setSid(rs.getInt("sid"));
student.setName(rs.getString("name"));
student.setAge(rs.getInt("age"));
student.setBirthday(rs.getDate("birthday"));
//加入到集合中
students.add(student);
}
//6.返回
return students;
}catch (Exception e){
throw new RuntimeException(e);
}finally {
JDBCUtils.close(conn,pstm,rs);
}
}
@Override
public Student findById(Integer sid) {
//定义必要信息
Connection conn = null;
PreparedStatement pstm = null;
ResultSet rs = null;
Student student = null;
try {
//1.获取连接
conn = JDBCUtils.getConnection();
//2.获取操作对象
pstm = conn.prepareStatement("select * from student where sid = ? ");
pstm.setInt(1,sid);
//3.执行sql语句,获取结果集
rs = pstm.executeQuery();
//4.遍历结果集
if (rs.next()) {
//5.封装
student = new Student();
student.setSid(rs.getInt("sid"));
student.setName(rs.getString("name"));
student.setAge(rs.getInt("age"));
student.setBirthday(rs.getDate("birthday"));
}
//6.返回
return student;
}catch (Exception e){
throw new RuntimeException(e);
}finally {
JDBCUtils.close(conn,pstm,rs);
}
}
@Override
public int insert(Student student) {
//定义必要信息
Connection conn = null;
PreparedStatement pstm = null;
int result = 0;
try {
//1.获取连接
conn = JDBCUtils.getConnection();
//2.获取操作对象
pstm = conn.prepareStatement("insert into student(sid,name,age,birthday)values(null,?,?,?)");
//3.设置参数
//pstm.setInt(1,null);
pstm.setString(1,student.getName());
pstm.setInt(2,student.getAge());
pstm.setDate(3,new Date(student.getBirthday().getTime()));
//4.执行sql语句
result = pstm.executeUpdate();
}catch (Exception e){
throw new RuntimeException(e);
}finally {
JDBCUtils.close(conn,pstm);
}
return result;
}
@Override
public int update(Student student) {
//定义必要信息
Connection conn = null;
PreparedStatement pstm = null;
int result = 0;
try {
//1.获取连接
conn = JDBCUtils.getConnection();
//2.获取操作对象
pstm = conn.prepareStatement("update student set name=?,age=?,birthday=? where sid=? ");
//3.设置参数
pstm.setString(1,student.getName());
pstm.setInt(2,student.getAge());
pstm.setDate(3,new Date(student.getBirthday().getTime()));
pstm.setInt(4,student.getSid());
//4.执行sql语句
result = pstm.executeUpdate();
}catch (Exception e){
throw new RuntimeException(e);
}finally {
JDBCUtils.close(conn,pstm);
}
return result;
}
@Override
public int delete(Integer sid) {
//定义必要信息
Connection conn = null;
PreparedStatement pstm = null;
int result = 0;
try {
//1.获取连接
conn = JDBCUtils.getConnection();
//2.获取操作对象
pstm = conn.prepareStatement("delete from student where sid=? ");
//3.设置参数
pstm.setInt(1,sid);
//4.执行sql语句
result = pstm.executeUpdate();
}catch (Exception e){
throw new RuntimeException(e);
}finally {
JDBCUtils.close(conn,pstm);
}
return result;
}
}
复制代码
划线
评论
复制
发布于: 刚刚阅读数: 8
版权声明: 本文为 InfoQ 作者【楠羽】的原创文章。
原文链接:【http://xie.infoq.cn/article/a3241e9cb389854fcb06e51da】。文章转载请联系作者。
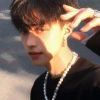
楠羽
关注
还未添加个人签名 2022.08.04 加入
还未添加个人简介
评论