前端 JavaScript 实现一个简易计算器
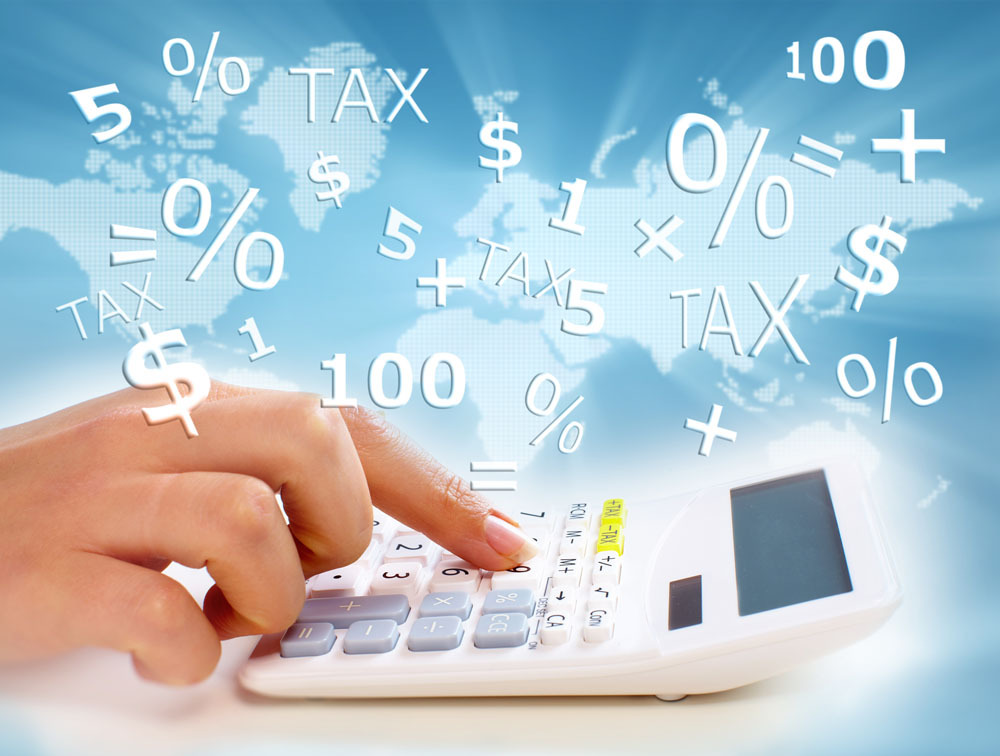
前端使用 JavaScript 实现一个简易计算器,没有难度,但是里面有些小知识还是需要注意的,算是一次基础知识回顾吧。
题目
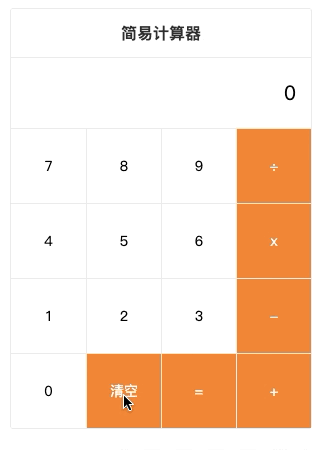
实现一个简易版的计算器,需求如下:
1、除法操作时,如果被除数为 0,则结果为 02、结果如果为小数,最多保留小数点后两位,如 2 / 3 = 0.67(显示 0.67), 1 / 2 = 0.5(显示 0.5)3、请阅读并根据提示补充完成函数 initEvent、result 和 calculate4、请不要手动修改 html 和 css5、不要使用第三方插件
实现
HTML 文件
CSS 文件
JavaScript 文件
分析
主要分析一下本题中 JavaScript 部分涉及的知识点。
事件委托
我们没有给数字和符号元素分别添加点击事件,而是通过给它们的父元素 .cal-keyboard
添加点击事件,再通过事件冒泡获得它父元素绑定的事件响应,使用事件委托有以下优点:
减少内存消耗
可以方便地动态添加或者删除元素
管理的函数减少
减少操作 DOM 节点的次数,提高性能
事件委托的步骤:
给父元素绑定响应事件
监听子元素的冒泡事件
获取触发事件的目标元素
保留小数位
大家的第一反应可能是使用 toFixed() 方法,但是这个方法在小数位不足的情况下会在后面补 0,比如:
可以看到,这个是不符合要求的。
还有一个问题需要注意:小数的相加结果可能并不符合预期,比如:
这里我们建议使用 Math.floor()
方法来处理小数位,先给结果乘以 100,再通过 Math.floor()
取得整数部分,然后除以 100,得到符合要求的结果,比如:
~
~ 本文完
~
学习有趣的知识,结识有趣的朋友,塑造有趣的灵魂!
大家好,我是〖编程三昧〗的作者 隐逸王,我的公众号是『编程三昧』,欢迎关注,希望大家多多指教!
你来,怀揣期望,我有墨香相迎! 你归,无论得失,唯以余韵相赠!
知识与技能并重,内力和外功兼修,理论和实践两手都要抓、两手都要硬!
版权声明: 本文为 InfoQ 作者【编程三昧】的原创文章。
原文链接:【http://xie.infoq.cn/article/9e014c3f9012a9fb0ae6a1d09】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
评论