【Java8 新特性 3】Supplier 简介,springboot 面试题
import java.util.function.Supplier;
public class TestSupplier {
private int age;
public static void test(){
System.out.println("Java8 新特性,Supplier");
}
TestSupplier(){
System.out.println("构造函数,age,"+age);
}
public static void main(String[] args) {
//创建 Supplier 容器,声明为 TestSupplier 类型,此时并不会调用对象的构造方法,即不会创建对象
Supplier<TestSupplier> sup= TestSupplier::new;
sup.get().test();
System.out.println("--------");
//调用 get()方法,此时会调用对象的构造方法,即获得到真正对象
sup.get();
//每次 get 都会调用构造方法,即获取的对象不同
System.out.printl
n("是否是相同的对象实例"+sup.get()==sup.get().toString());
}
}
3、控制台输出?
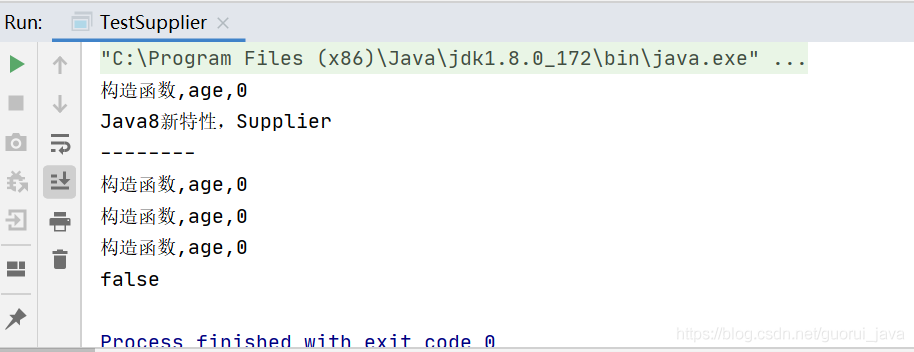
4、通俗易懂
private static int getMax(Supplier<Integer> supplier) {
return supplier.get();
}
private static void test01() {
Integer[] arr = {10,2,5,3,9,4};
int max2 = getMax(() -> {
int max = 0;
for(Integer i : arr) {
if(i > max) {
max = i;
}
}
return max;
});
System.out.println(max2);//10
}
二、Supplier 源码分析
Supplier<T>接口源码中只有一个 get()方法。
每次 get 都会调用构造方法,即获取的对象不同。
/*
Copyright (c) 2012, 2013, Oracle and/or its affiliates. All rights reserved.
ORACLE PROPRIETARY/CONFIDENTIAL. Use is subject to license terms.
*/
package java.util.function;
/**
Represents a supplier of results.
<p>There is no requirement that a new or distinct result be returned each
time the supplier is invoked.
<p>This is a <a href="package-summary.html">functional interface</a>
whose functional method is {@link #get()}.
@param <T> the type of results supplied by this supplier
@since 1.8
*/
@FunctionalInterface
public interface Supplier<T> {
/**
Gets a result.
@return a result
*/
T get();
}
可以看到这份代码中,有一个比较奇特的注解 @FunctionalInterface,这是一个函数式接口的声明。该注解不是必须的,如果一个接口符合"函数式接口"定义,那么加不加该注解都没有影响。加上该注解能够更好地让编译器进行检查。如果编写的不是函数式接口,但是加上了 @FunctionInterface,那么编译器会报错。
三、lambda 表达式与 Supplier 的组合使用
为何不直接使用 new?而使用 Supplier,感觉华而不实,和 new 有啥区别?
1、person 类
package com.guor.effective.chapter2.java8;
public class Person {
Integer id;
String name;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
评论