JAVA StreamAPI
作者:流火
- 2022 年 9 月 07 日 北京
本文字数:2867 字
阅读完需:约 9 分钟
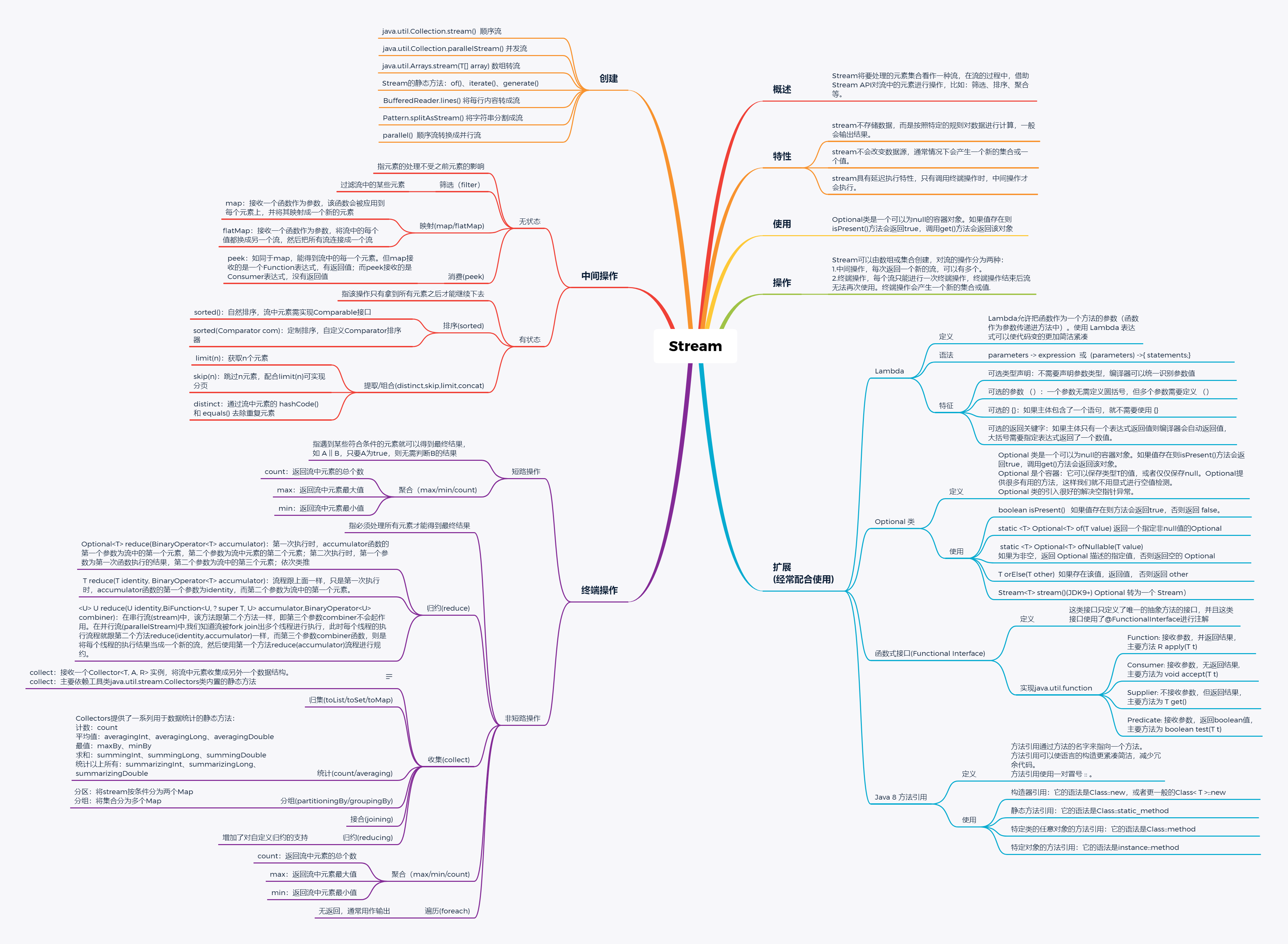
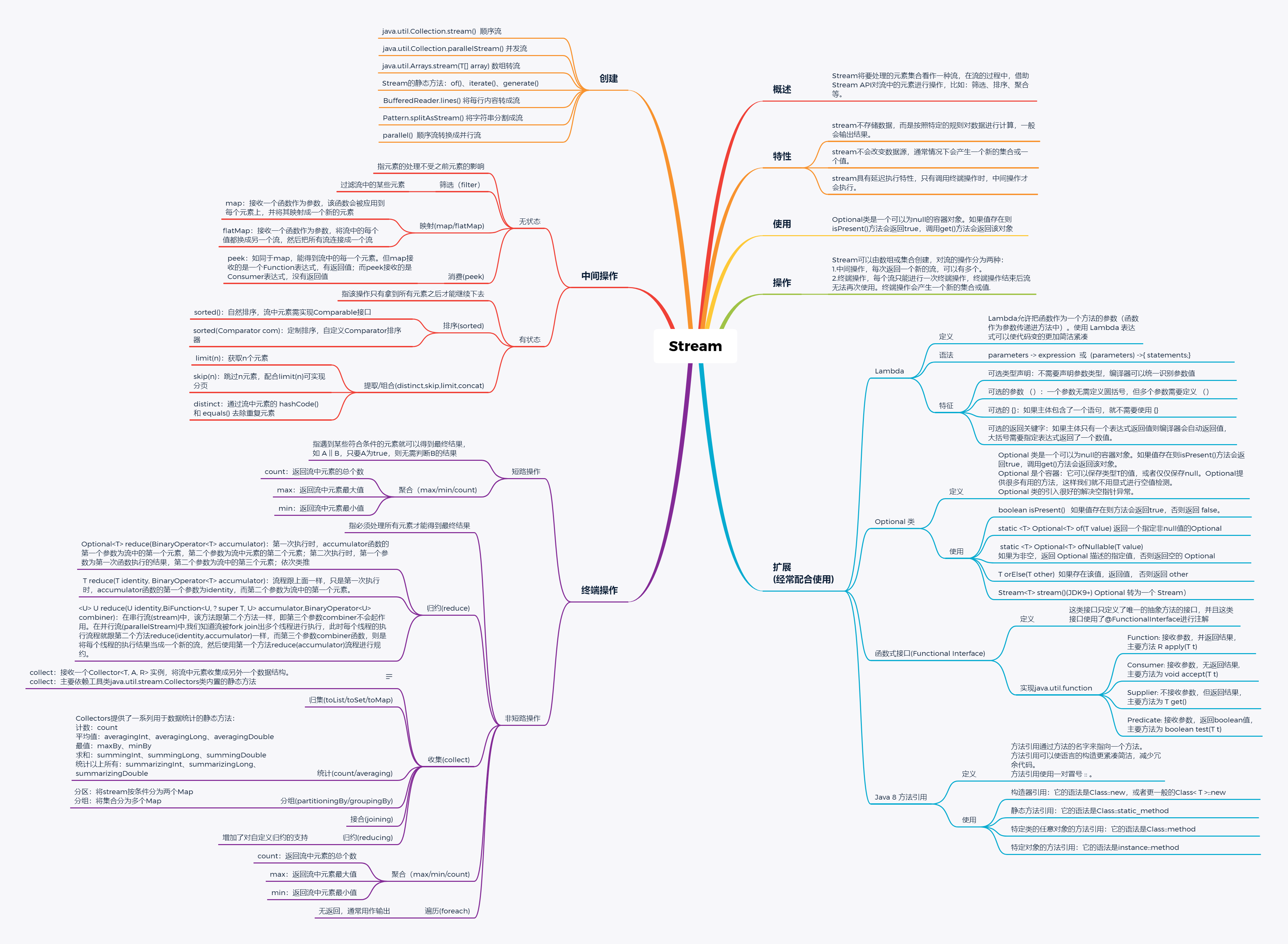
1.概述
Stream API 是 java 8 的新特性,给我们编程提供了极大的遍历。
Stream API 对流的操作分为两种:
中间操作:每次返回一个新的流,可以有多个。
终端操作:每个流只能进行一次终端操作,终端操作结束后流无法再次使用。
终端操作会产生一个新的集合或值
有几个特性:
stream 不存储数据,而是按照特定的规则对数据进行计算,一般会输出结果。
stream 不会改变数据源,通常情况下会产生一个新的集合或一个值。
stream 具有延迟执行特性,只有调用终端操作时,中间操作才会执行
2.实例
2.1 创建
/**
* Collection下的 stream()顺序流 和 parallelStream()并行流 方法
*/
List<String> list = new ArrayList<>();
//获取一个顺序流
Stream<String> stream = list.stream();
//获取一个并行流
Stream<String> parallelStream = list.parallelStream();
/**
* Arrays 中的stream()方法,将数组转成流
*/
Stream<Integer> arrayStream = Arrays.stream(new Integer[10]);
/**
* Stream中的静态方法:of()、iterate()、generate()
*/
Stream<Integer> ofStream = Stream.of(1,2,3,4,5,6);
Stream<Integer> iterateStream = Stream.iterate(0, (x) -> x + 2).limit(6);
Stream<Double> generateStream = Stream.generate(Math::random).limit(2);
/**
* BufferedReader.lines() 方法,将每行内容转成流
*/
BufferedReader reader = new BufferedReader(new FileReader("F:\\test_stream.txt"));
Stream<String> fileStream = reader.lines();
/**
* 使用 Pattern.splitAsStream() 方法,将字符串分隔成流
*/
Pattern pattern = Pattern.compile(",");
Stream<String> stringStream = pattern.splitAsStream("a,b,c,d");
复制代码
2.2 使用
private static List<Student> getList(){
List<Student> list = new ArrayList<Student>();
list.add(new Student("Tom", 17,Student.SubjectEnum.SX, 77));
list.add(new Student("Tom", 17,Student.SubjectEnum.YW, 87));
list.add(new Student("Jack", 18,Student.SubjectEnum.SX, 87));
list.add(new Student("Jack", 18,Student.SubjectEnum.YY, 77));
list.add(new Student("Lily", 17,Student.SubjectEnum.SX, 97));
list.add(new Student("Lily", 17,Student.SubjectEnum.YW, 77));
list.add(new Student("Owen", 16,Student.SubjectEnum.SX, 87));
list.add(new Student("Anni", 16,Student.SubjectEnum.YW, 97));
list.add(new Student("Alisa", 20,Student.SubjectEnum.YY, 65));
return list;
}
public static void main(String[] args) {
List<Student> list = getList();
//查找是否有 大于17的学生
boolean anyMatch= list.stream()
.anyMatch(s -> (s.getAge() > 17));//转list
System.out.println("找是否有 大于17的学生:"+anyMatch);// true
List<String> nameList=list.stream()
.filter(s -> (s.getAge() > 17)).map(Student::getName).collect(Collectors.toList());//转list
System.out.println("年龄大于17的学生:"+nameList);// [Jack, Jack, Alisa]
Student max = list.stream().max(Comparator.comparingInt(Student::getAge)).get();
System.out.println("年龄最大的学生:" + max.getName());//输出 Alisa;
Student min = list.stream().min(Comparator.comparingInt(Student::getAge)).get();
System.out.println("年龄最小的学生:" + min.getName());//输出 Owen;
long count = list.stream().filter(s -> s.getSubject() == Student.SubjectEnum.SX).count();
System.out.println("学科数学中学生数量 :" + count);//输出 4;
//分数之和
Optional<Integer> sumSalary = list.stream().map(Student::getScore).reduce((s1,s2)->s1+s2);
Optional<Integer> sumSalary1 = list.stream().map(Student::getScore).reduce(Integer::sum);
System.out.println("分数之和 方式1:" + sumSalary.get());//输出 751;
System.out.println("分数之和 方式2:" + sumSalary1.get());//输出 751;
// 求总数
Long count1 = list.stream().collect(Collectors.counting());
// 求平均工资
Double average = list.stream().collect(Collectors.averagingDouble(Student::getScore));
// 求最高工资
Optional<Integer> max1 = list.stream().map(Student::getScore).collect(Collectors.maxBy(Integer::compare));
// 求工资之和
Integer sum = list.stream().collect(Collectors.summingInt(Student::getScore));
// 一次性统计所有信息
DoubleSummaryStatistics collect = list.stream().collect(Collectors.summarizingDouble(Student::getScore));
System.out.println("分数记录数:" + count);//4
System.out.println("平均每人每科分数:" + average);//83.44444444444444
System.out.println("分数总和:" + sum);//751
System.out.println("分数所有统计:" + collect);//DoubleSummaryStatistics{count=9, sum=751.000000, min=65.000000, average=83.444444, max=97.000000}
// 将员工按薪资是否高于8000分组
Map<Boolean, List<Student>> part = list.stream().collect(Collectors.partitioningBy(x -> x.getScore() > 80));
System.out.println("分数大于80是分组情况:" + part);
// 将员工先按性别分组,再按地区分组
Map<Student.SubjectEnum, Map<Integer, List<Student>>> group2 = list.stream().collect(Collectors.groupingBy(Student::getSubject, Collectors.groupingBy(Student::getAge)));
System.out.println("按学科、年龄:" + group2);
// 按工资倒序排序
List<String> newList2 = list.stream().sorted(Comparator.comparing(Student::getScore).reversed())
.map(Student::getName).collect(Collectors.toList());
// 先按工资再按年龄升序排序
List<String> newList3 = list.stream()
.sorted(Comparator.comparing(Student::getScore).thenComparing(Student::getAge)).map(Student::getName)
.collect(Collectors.toList());
System.out.println("分数倒序:" + newList2);
System.out.println("先按分数再按年龄升序排序:" + newList3);
}
复制代码
以上实例,简单参考,有具体问题,具体处理。可以百度分析
参考:
Java 8 Stream:20 个实例,玩转集合的筛选、归约、分组、聚合 (qq.com)
划线
评论
复制
发布于: 刚刚阅读数: 5
版权声明: 本文为 InfoQ 作者【流火】的原创文章。
原文链接:【http://xie.infoq.cn/article/9832f20048d04ec9cc7d58fde】。未经作者许可,禁止转载。
流火
关注
还未添加个人签名 2018.01.31 加入
还未添加个人简介
评论