JavaScript Array 方法详解
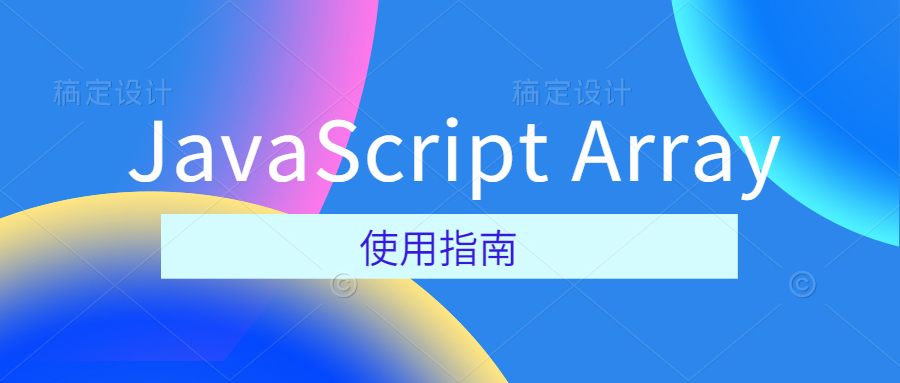
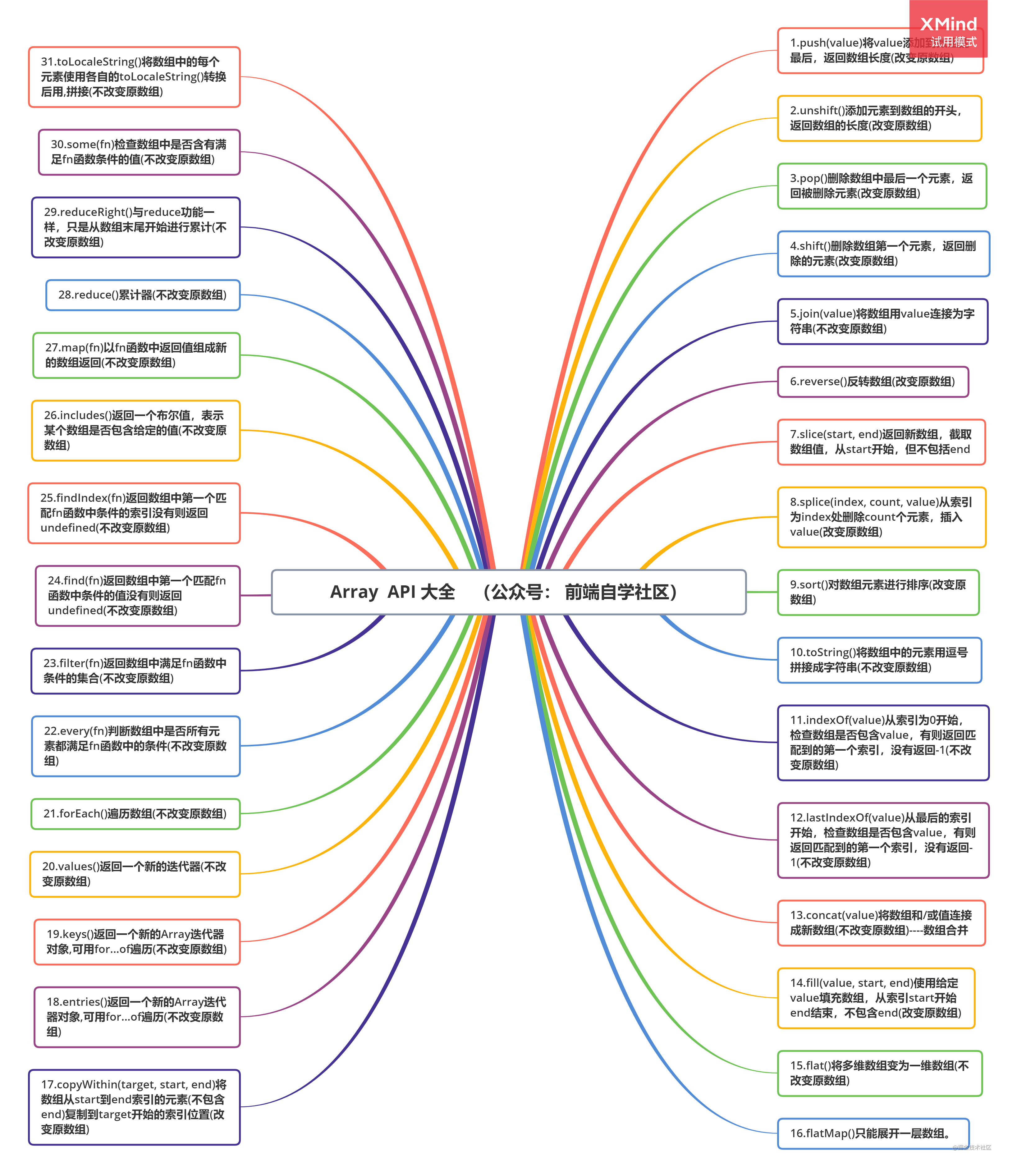
前言
我们在日常开发中,与接口打交道最多了,前端通过访问后端接口,然后将接口数据二次处理渲染到页面当中。二次处理的过程是 考验 Coder
对 Array
是否熟练 以及 在 何种 场景下使用哪种方法处理最优 。小编,在最近开发中就遇到了 Array
问题, 在处理复杂的业务需求时,没想到Array
有类似的方法,然后将方法 组合起来解决当下问题。
文章用下班时间肝了一周才写完,看完点赞、转发 是对我最大的支持。
遍历数组方法
不会改变原数组的遍历方法
forEach()
forEach()
方法按照升序为数组中每一项执行一次给定的函数。
语法
currentValue
: 数组当前项值index
: 数组当前项索引arr
: 数组对象本身thisArg
: 可选参数。当执行回调函数callback
时,用作this
的值。
注意
如果使用 箭头函数表达式来传入函数参数,
thisArg
参数会被忽略,因为箭头函数在词法上绑定了this
值。forEach
不会直接改变调用它的对象,但是那个对象可能会被callback
函数改变。every
不会改变原数组。
reduce()
reduce()
数组元素累计器,返回一个合并的结果值。
语法
accumulator
: 累计器,默认为数组元素第一个值currentValue
: 当前值index
: 当前元素索引 <font style="color:red">可选</font>array
: 数组 <font style="color:red">可选</font>initialValue
: 初始值 <font style="color:red">可选</font>
reduce
有两个参数,一个是回调函数,一个是初始值。
它有两种取值情况:
当提供了
initialValue
初始值时, 那么accumulator
的值为initialValue
,currentValue
的值为数组第一个值
当没有提供
initialValue
初始值时, 那么accumulator
的值 为 数组第一个值,currentValue
为第二个值。
注意
如果数组为空,且没有提供
initialValue
初始值时,会抛出TypeError
.如果数组有一个元素,且没有提供
initialValue
或者 提供了initialValue
,数组为空,那么唯一值被返回不会执行callback
回调函数。
求和
计算对象中的值
要累加对象数组中包含的值,必须提供初始值,以便各个item正确通过你的函数。
二维数组转一位数组
find()
find()
返回满足特定条件的元素对象或者元素值, 不满足返回 undefined
语法
element
: 当前元素index
: 当前元素索引 <font style="color:red">可选</font>array
: 数组本身 <font style="color:red">可选</font>thisArg
: 执行回调时用作this
的对象。 <font style="color:red">可选</font>
findIndex()
findIndex()
返回数组中符合条件的第一个元素的索引,没有,则返回 -1
。
语法
element
: 当前元素index
: 当前元素索引 <font style="color:red">可选</font>array
: 数组本身 <font style="color:red">可选</font>thisArg
: 执行回调时用作this
的对象。 <font style="color:red">可选</font>
key()
key()
返回一个新的 Array Iterator 对象,该对象包含数组中每个索引的键。
语法
注意
如果数组中有空原元素,在获取 key 时, 也会加入遍历的队列中。
values()
values()
方法返回一个新的 Array Iterator 对象,该对象包含数组每个索引的值。
语法
返回 布尔值
every()
every
用来判断数组内所有元素是否符合某个条件,返回 布尔值
语法
currentValue
: 数组当前项值 <font style="color:red">必须</font>index
: 数组当前项索引 <font style="color:red">可选</font>arr
: 数组对象本身<font style="color:red">可选</font>thisArg
: 可选参数。当执行回调函数callback
时,用作this
的值。<font style="color:red">可选</font>
注意
当所有的元素都符合条件才会返回
true
every
不会改变原数组。若传入一个空数组,无论如何都会返回
true
。
some()
some()
用来判断数组元素是否符合某个条件,只要有一个元素符合,那么返回 true
.
语法
currentValue
: 数组当前项值 <font style="color:red">必须</font>index
: 数组当前项索引 <font style="color:red">可选</font>arr
: 数组对象本身<font style="color:red">可选</font>thisArg
: 可选参数。当执行回调函数callback
时,用作this
的值。<font style="color:red">可选</font>
注意
some()
被调用时不会改变数组。如果用一个空数组进行测试,在任何情况下它返回的都是
false
。some()
在遍历时,元素范围已经确定,在遍历过程中添加的元素,不会加入到遍历的序列中。
不改变原有数组,形成新的数组
filter()
filter()
用来遍历原数组,过滤拿到符合条件的数组元素,形成新的数组元素。
语法
currentValue
: 数组当前项值 <font style="color:red">必须</font>index
: 数组当前项索引 <font style="color:red">可选</font>arr
: 数组对象本身<font style="color:red">可选</font>thisArg
: 可选参数。当执行回调函数callback
时,用作this
的值。<font style="color:red">可选</font>
注意
filter
不会改变原数组,它返回过滤后的新数组。filter()
在遍历时,元素范围已经确定,在遍历过程中添加的元素,不会加入到遍历的序列中。
map()
map()
创建一个新的数组,其结果是该数组中的每个元素都调用一个提供的函数后返回的结果。
语法
currentValue
: 数组当前项值 <font style="color:red">必须</font>index
: 数组当前项索引 <font style="color:red">可选</font>arr
: 数组对象本身<font style="color:red">可选</font>thisArg
: 可选参数。当执行回调函数callback
时,用作this
的值。<font style="color:red">可选</font>
注意
map
不修改调用它的原数组本身map()
在遍历时,元素范围已经确定,在遍历过程中添加的元素,不会加入到遍历的序列中。
<hr/>
数组 CRUD
改变原数组方法
reverse()
reverse()
方法将数组中元素的位置颠倒,并返回该数组。数组的第一个元素会变成最后一个,数组的最后一个元素变成第一个。该方法会改变原数组。
sort()
sort()
方法采用 原地算法进行排序并返回数组。默认排序顺序是在将元素转换为字符串,然后比较它们的UTF-16
代码单元值序列
原地算法是一个使用辅助的数据结构对输入进行转换的算法。但是,它允许有少量额外的存储空间来储存辅助变量。当算法运行时,输入通常会被输出覆盖。原地算法仅通过替换或交换元素来更新输入序列。
删除元素
shift()
shift()
方法从数组中删除第一个元素,并返回该元素的值。此方法更改数组的长度。
语法
注意
从数组中删除的元素; 如果数组为空则返回
undefined
pop()
pop()
方法从数组中删除最后一个元素,并返回该元素的值。此方法更改数组的长度。
用法和 shift
类似。
语法
注意
从数组中删除的元素; 如果数组为空则返回
undefined
splice()
splice()
方法通过删除或替换现有元素或者原地添加新的元素来修改数组,并以数组形式返回被修改的内容。此方法会改变原数组。
语法
start
: 开始的索引deleteCount
: 删除的个数 <font style="color:red">可选</font>[item1,item2 .....]
;从开始的索引进行 添加的增加和替换的元素, <font style="color:red">可选</font>
注意
由被删除的元素组成的一个数组。如果只删除了一个元素,则返回只包含一个元素的数组。如果没有删除元素,则返回空数组。
如果只传递了开始的索引位置,则会删除索引后的所有元素对象
从索引为 2 开始, 删除 1 个数组元素对象,添加两个数组元素对象
增加元素
splice()
上面已经有介绍
push()
push()
方法将一个或多个元素添加到数组的末尾,并返回该数组的新长度。
语法
合并数组
unshift()
unshift()
方法将一个或多个元素添加到数组的开头,并返回该数组的新长度。
不改变原数组元素方法
indexOf()
indexOf()
方法返回可以在数组中找到给定元素的第一个索引,如果不存在,则返回 -1。
语法
searchElement
: 要查找的元素fromIndex
: 按指定的索引进行查找出现的指定元素的第一个索引。 <font style="color:red">可选</font>如果索引大于或等于数组的长度,则返回-1
如果提供的索引值为负数,则将其视为距数组末尾的偏移量
如果提供的索引为负数,仍然从前到后搜索数组
如果提供的索引为 0,则将搜索整个数组。
默认值:0(搜索整个数组)。
数组去重
创建一个新的空数组,通过indexOf
来判断空数组是否第一次存在某个元素,
不存在则返回 [ < 0 ] ,
push
到空数组中.
lastIndexOf()
lastIndexOf()
查找数组中元素最后一次出现的索引,如未找到返回-1。
如果不存在则返回 -1。从数组的后面向前查找,从 fromIndex
处开始。
语法
searchElement
: 要查找的元素fromIndex
: 按指定的索引进行查找出现的指定元素的第一个索引。 <font style="color:red">可选</font>从指定的索引位置 逆向 查找
默认为数组的长度减 1(
arr.length - 1
),即整个数组都被查找。如果该值大于或等于数组的长度,则整个数组会被查找。
如果为负值,数组仍然会被从后向前查找。
如果该值为负时,其绝对值大于数组长度,则方法返回 -1,即数组不会被查找。
注意
lastIndexOf
使用的是 严格相等 === 比较searchElement
和数组中的元素。
inCludes()
includes()
方法用来判断一个数组是否包含一个指定的值,根据情况,如果包含则返回 true,否则返回 false。
语法
searchElement
: 要查找的元素查找时,区分大小写
fromIndex
: 按指定的索引进行查找出现的指定元素的第一个索引。 <font style="color:red">可选</font>从指定的索引进行查找
如果为负值,则按升序从
array.length + fromIndex
的索引开始搜如果
fromIndex
大于等于数组的长度,则会返回false
,且该数组不会被搜索。默认为 0
concat()
concat()
方法用于合并两个或多个数组。
语法
注意
concat
方法不会改变this
或任何作为参数提供的数组,而是返回一个浅拷贝,它包含与原始数组相结合的相同元素的副本对象引用(而不是实际对象):
concat
将对象引用复制到新数组中。 原始数组和新数组都引用相同的对象。 也就是说,如果引用的对象被修改,则更改对于新数组和原始数组都是可见的。 这包括也是数组的数组参数的元素。数据类型如字符串,数字和布尔(不是
String
,Number
和Boolean
) 对象):concat
将字符串和数字的值复制到新数组中。
toString()
toString()
返回一个字符串,表示指定的数组及其元素。
当一个数组被作为文本值或者进行字符串连接操作时,将会自动调用其 toString
方法。
对于数组对象,toString
方法连接数组并返回一个字符串,其中包含用逗号分隔的每个数组元素。
语法
join()
join()
方法通过连接数组元素用逗号或指定的分隔符字符串分隔,返回一个字符串。
如果数组只有一项,则将在不使用分隔符的情况下返回该项。
语法
separator
: 指定的分割的 字符 <font style="color:red">可选</font>
slice()
slice()
方法返回一个新的数组对象,这一对象是一个由 begin
和 end
决定的原数组的浅拷贝(包括 begin
,不包括end
)。原始数组不会被改变。
语法
begin
: 指定截取的开始索引 <font style="color:red">可选</font>默认从 0 开始
如果
begin
为负数,则以数组末尾开始 的 绝对值开始截取slice(-2)
末尾第 2 个元素如果
begin
超出原数组的索引范围,则会返回空数组。
end
: 指定截取的结束索引 <font style="color:red">可选</font>如果
end
被省略,则slice
会一直提取到原数组末尾。如果
end
大于数组的长度,slice
也会一直提取到原数组末尾。如果
end
为负数, 则它表示在原数组中的倒数第几个元素结束抽取。
参考文献
最后
文章用下班时间肝了一周,写作不易,文中的内容都是 参考 MDN 文档 。如果喜欢的话可以点赞👍👍👍关注,支持一下,希望大家可以看完本文有所收获 !
版权声明: 本文为 InfoQ 作者【HaiJun】的原创文章。
原文链接:【http://xie.infoq.cn/article/8c4aef9bbb994037e8014a61b】。文章转载请联系作者。
评论