Go- 文件读写 -1
发布于: 4 小时前
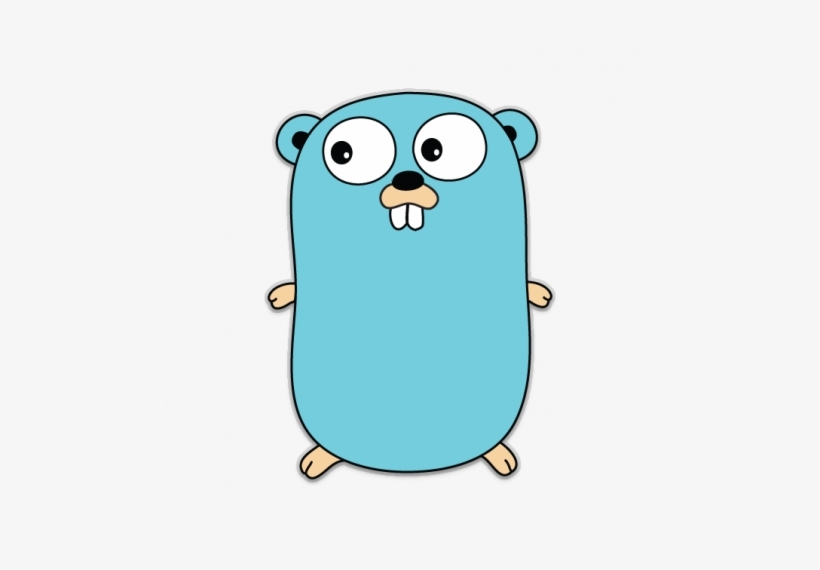
Go 学习笔记,学习内容《Go入门指南》
主要介绍以下内容:
读取键盘和标准输入
读取字符串
利用 bufio 包从标准输入读取数据
利用 bufio 包读文件
读取整个文件
读取压缩文件
写文件
文件拷贝
代码示例可以直接运行
package main
import (
"bufio"
"compress/gzip"
"fmt"
"io"
"io/ioutil"
"os"
"path/filepath"
)
func printDivider(str ...string) {
fmt.Println()
fmt.Println("-----------------------------------------------")
if len(str) > 0 {
fmt.Println(str)
}
}
var (
firstName, lastName, s string
i int
f float32
)
func main() {
/*
读取键盘和标准输入
*/
printDivider("读取键盘和标准输入")
fmt.Println("Please input your full name: ")
//fmt.Scanln(&firstName, &lastName)
//fmt.Printf("Hi %s %s\n", firstName, lastName)
/*
读取字符串
Sscan开头的函数都是从字符串读取
*/
printDivider("读取字符串")
fmt.Sscanf("3.14,100,Hello", "%f,%d,%s", &f, &i, &s)
fmt.Printf("From the string, we read %f, %d, %s\n", f, i, s)
/*
利用bufio包从标准输入读取数据
*/
printDivider("利用bufio包提供的缓冲读取来读取数据")
inputReader := bufio.NewReader(os.Stdin) // 创建一个读取器,并且与标准输入绑定
fmt.Println("Please enter your name: ")
input, err := inputReader.ReadString('\n') // 读取到\n结束,返回的input包含\n
if err != nil {
fmt.Println("There were errors reading, error: ", err)
fmt.Println("exiting program.")
return
}
fmt.Printf("Your name is %s", input)
switch input {
case "Philip\r\n":
fallthrough
case "Chris\r\n":
fallthrough
case "Ivo\r\n":
fmt.Printf("Welcome %s\n", input)
default:
fmt.Printf("You are not welcome here, Goodbye\n")
}
/*
利用bufio包读文件
*/
printDivider("利用bufio包读文件")
inputFile, inputError := os.Open("person.go") // 默认以只读方式打开文件,inputFile类型:*os.File
if inputError != nil {
fmt.Printf("An error occurred on opening the inputfile\n" +
"Does the file exist?\n" +
"Have you got access to it?\n")
return
}
defer inputFile.Close()
inputReader = bufio.NewReader(inputFile) // NewReader函数参数类型:*os.File
for {
inputString, readerError := inputReader.ReadString('\n') // windows和Linux的文件换行符不同,不用关心操作系统类型,直接使用\n就可以
fmt.Printf("The input was: %s", inputString)
if readerError == io.EOF {
break
}
}
/*
读取整个文件
*/
printDivider("读取整个文件")
inFile := "person.go"
outFile := "person_copy.go"
buf, err := ioutil.ReadFile(inFile)
if err != nil { // 若文件不存在,运行错误:File Error: open person-.go: The system cannot find the file specified.
fmt.Fprintf(os.Stderr, "File Error: %s\n", err)
//fmt.Fprintf(os.Stderr, "File Error: %s\n", err.Error())
}
fmt.Printf("%s\n", string(buf))
err = ioutil.WriteFile(outFile, buf, 0644) // err类型是接口类型,调用接口内的函数Error()输出错误信息
if err != nil {
panic(err.Error())
}
filename := filepath.Base("/path/to/golang/")
fmt.Println(filename) // 输出:golang
/* 对于各种格式的文件处理,可以从go package中找到对应的包处理,找不到的时候,自己实现处理 */
/*
读取压缩文件
支持压缩文件类型:gzip、flate、bzip2、lzw、zlib
*/
printDivider("读取压缩文件")
fName := "person.gz"
var r *bufio.Reader
fi, err := os.Open(fName)
if err != nil {
fmt.Fprintf(os.Stderr, "%v, Can't open %s, error: %s\n", os.Args[0], fName, err)
os.Exit(1) // 输出:C:\Users\ADMINI~1\AppData\Local\Temp\go-build2880183904\b001\exe\038_Read.exe, Can't open person.zip, error: open person.zip: The system cannot find the file specified.
}
fz, err := gzip.NewReader(fi)
if err != nil {
r = bufio.NewReader(fi)
} else {
r = bufio.NewReader(fz)
}
for {
line, err := r.ReadString('\n')
if err != nil {
fmt.Println("Done reading file")
break
}
fmt.Println(line)
}
/*
写文件
*/
printDivider("写文件")
outputFile, outputError := os.OpenFile("output.dat", os.O_WRONLY|os.O_CREATE, 0666)
if outputError != nil {
fmt.Printf("An error occured with file opening or creation\n")
return
}
defer outputFile.Close()
outputWrite := bufio.NewWriter(outputFile)
outputString := "Hello world\n"
for i := 0; i < 10; i++ {
outputWrite.WriteString(outputString)
}
outputWrite.Flush()
outputFile.WriteString(" Hello, GO\n")
fmt.Fprintf(outputFile, "Hello, Fprintf\n")
/*
文件拷贝
*/
printDivider("文件拷贝")
srcFile, err := os.Open("person.go")
_, err = io.Copy(outputFile, srcFile) // 追加的方式拷贝
if err != nil {
fmt.Println("io.Copy error: ", err)
}
}
复制代码
划线
评论
复制
发布于: 4 小时前阅读数: 5
版权声明: 本文为 InfoQ 作者【HelloBug】的原创文章。
原文链接:【http://xie.infoq.cn/article/834a385abf723a683c6cc5322】。
本文遵守【CC BY-NC-ND】协议,转载请保留原文出处及本版权声明。
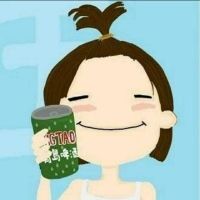
HelloBug
关注
还未添加个人签名 2018.09.20 加入
还未添加个人简介
评论