阿里码农肝了 2 晚,整理的 Java 语法总结,网友:考试复习全靠它了
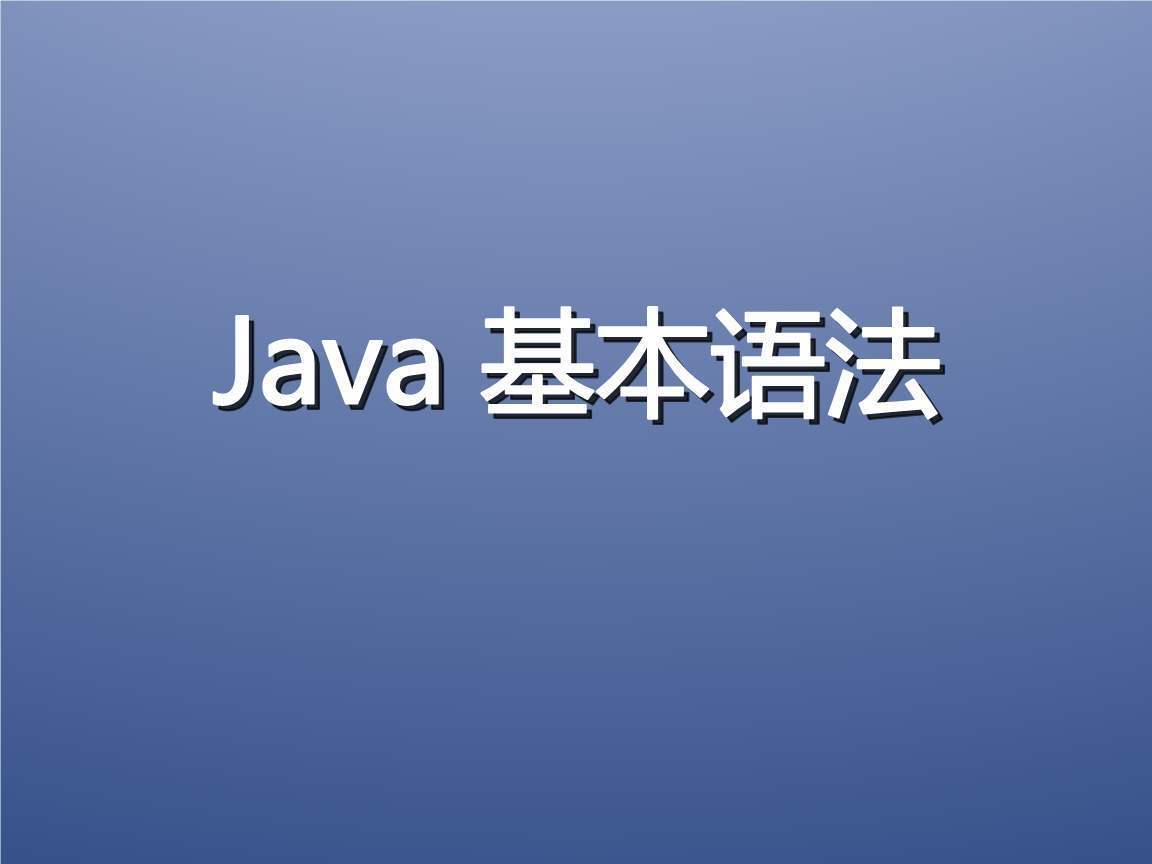
Hello,今天给各位童鞋们分享 java 常见的基本语法,学习 Java 语言,我们就要熟练它的语法,赶紧拿出小本子记下来吧!
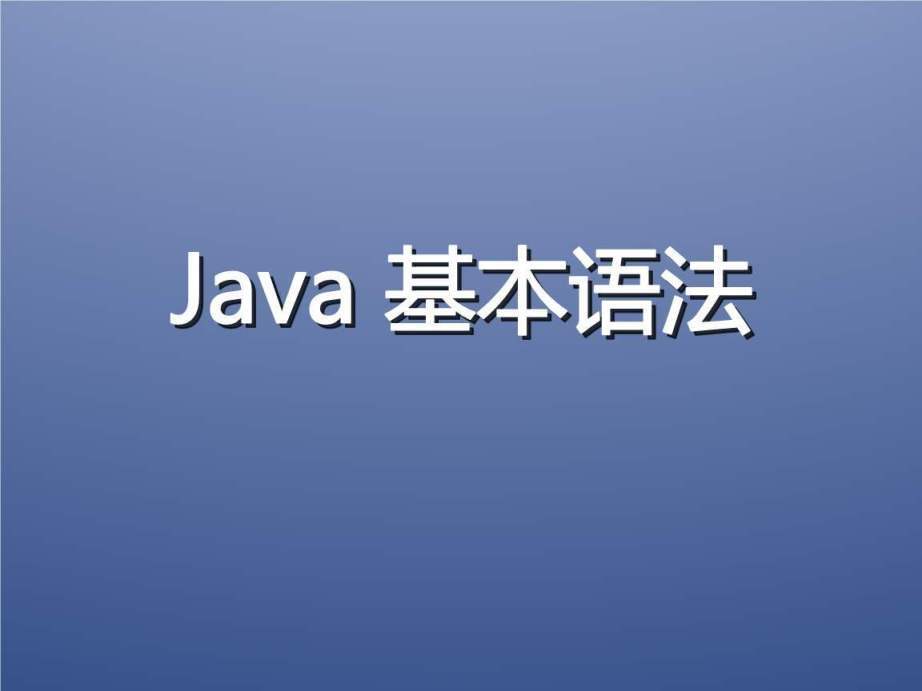
1.注释
单行注释//
多行注释/* */
文档注释/** */
2.标识符
基本
1.关键字
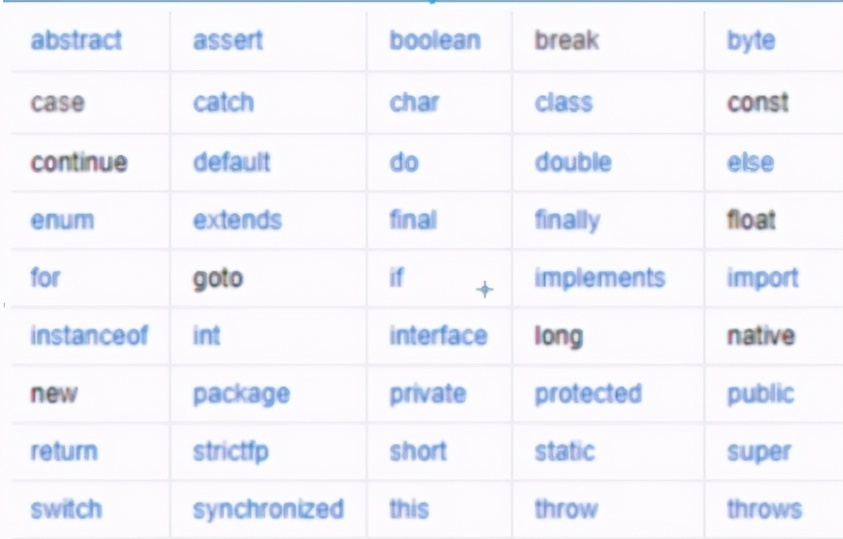
2.所有标识符都以 字母,_ , $ 开头
标识符大小写敏感
3.整数型 byte short int long
浮点型 float double
字符型 char (字符代表的是一个字,两个及多个就不对)
字符串 string (它不是关键字,它是一个类)
布尔值 boolean
long num = 90L;
float num1 = 5.1F;
char name = 'a';
string name1 = "容辞";
boolean flag = true;
4.1bit(1 位)
1Byte(一个字节)
8b = 1B
1024B = 1KB
1024KB = 1M
1024M = 1G
1024G = 1TB
5.转义字符
\t 制表符 \n 换行
关于数据类型面试扩展
1.int
int i = 10;
int i1 = 010;(八进制 0)
int i2 = 0b10;(二进制 0b)
int i3 = 0x10;(十六进制 0x)
2.float/double
float f = 0.1f;
double d = 1.0/10;
System.out.println(f == d); //false
float f1 = 2333333333f;
float f2 = f1+1;
System.out.println(f1 == f2); //true
float 有限 大约 舍入误差
银行业务不能用浮点型表示!可以用类 BigDecimal
结论:少用浮点型进行比较
3.char
char c1 = 'a';
char c2 = '中';
System.out.println(c1);
System.out.println((int)c1); //强制转换类型
System.out.println(c2);
System.out.println((int)c2); //强制转换类型
char c3 = '\u0061'; //unicode
System.out.println(c3);
所有字符型本质上都是数字
这里有个 unicode 编码 u0000—uFFFF
4.boolean
boolean flag = true;
if (flag == true){} //新手
if (flag){} //老手
//less is more!
3.类型转换
高低顺序:
char,short,byte—>int—>long—>float—>double
int i = 128;
byte b = (byte)i; //强制转换--->从高到低
System.out.println(i);
System.out.println(b); //输出 b=-128--->内存溢出
int i = 128;
double b = i; //自动转换--->从低到高
System.out.println(i);
System.out.println(b);
System.out.println((int)23.77); //23
System.out.println((int)-45.5789f); //-45
char c = 'a';
int t = c+1;
System.out.println(t); //98
System.out.println((char)t); //b
注意事项:
布尔型不能进行转换
转换的时候可能存在内存溢出或精度问题
int money = 10_0000_0000; //可在数字中加入下划线
System.out.println(i); //1000000000
int year = 20;
int total = money*year;
long total1 = money*(long)year;
System.out.println(total); //溢出
System.out.println((long)total); //溢出
System.out.println(total1); //20000000000
4.变量
变量作用域
1.实例变量:它在类内方法外
实例变量如果不自行初始化,它的默认值为 0 0.0 u0000
布尔值默认 false
除了基本类型外其余默认为 null
2.类变量(static)
3.局部变量:局部变量必须声明和初始化值
public class Helloworld {
String name; //实例变量:从属于对象
int age;
static double salary = 25000; //类变量
public static void main(String[] args) //mian 方法
int a = 1; //局部变量
System.out.println(a);
//变量类型+变量名字 = new Helloworld()
Helloworld Helloworld = new Helloworld(); //实例变量
System.out.println(Helloworld.name);
System.out.println(salary); //类变量
}
}
变量的命名规范
1.变量命名: 见名知意
2.类成员变量: 首字母小写,除了第一个单词后面单词首字母大写(驼峰原则)
3.常量名:全是大写字母或者下划线
4.类名:首字母大写,驼峰原则(新建文件命名)
5.常量
一种特殊的变量,它的值被设定后便不可以改变!
final 常量名 = 常量值
常量名一般用大写字符
public class Helloworld {
static final double PI = 3.14; //常量
public static void main(String[] args) {
System.out.println(PI);
}
}
6.基本运算符
ctrl+D 复制当前行到下一行
当使用除法的时候会出现小数一定要注意数据类型,要把整数强制转化为小数
整数型进行运算的时候,当有 long 参与,结果为 long 型;无 long 参与,结果全为 int。(double 同理)
与或非 &&,||,!
自增自减 ++ ——
int a =10;
int b = a++; //先给 b 赋值,然后 a 在自增
(隐藏了 a=a+1)
System.out.println(a); //a=11
(隐藏了 a=a+1)
int c = ++a; //a 先自增,再给 c 赋值
System.out.println(a); //a=12
System.out.println(b); //b=10
System.out.println(c); //c=12
幂
double pow = Math.pow(2,3); //Math 类
System.out.println(pow); //8.0
逻辑运算符
&& || !
boolean a = true;
boolean b = false;
System.out.println("a&&b:"+(a&&b));
System.out.println("a||b:"+(a||b));
System.out.println("!(a&&b):"+(!(a&&b)));
短路原则:当执行与运算时,如果前面的已经为 flase 那么根本没有执行与运算。
int c = 5;
boolean d = (c<=4)&&(c++<=4);
System.out.println(d); //flase
System.out.println(c); //c=5
位运算
& | ~ (非) ^
int a = 0b1000;
int b = 0b1101;
System.out.println(a&b); //与运算 1000 & 1101 = 1000
System.out.println(a|b); //或运算 1101
System.out.println(a^b); //异或运算 0101 相同就取 0,不同就取 1
<< >>
System.out.println(2<<3); //16 << 代表乘 2
System.out.println(8>>2); //2 >> 代表除 2
三元运算符
a+=b a=a+b
x ? y : z 如果 x==true,则执行 y;否则执行 z
int score = 80;
String type = score<60?"不及格":"及格";
System.out.println(type); //及格
字符串连接符 +
int a = 10;
int b = 20;
System.out.println(""+a+b); //1020
System.out.println(a+b+""); //30
好啦,今天的文章就到这里,希望能帮助到屏幕前迷茫的你们!
评论