Spring 框架技术总结 (二),java 自学视频免费
Target
:目标对象,代理的目标对象Proxy
:代理,一个类被 AOP 织入增强后,就产生一个结果代理类Joinpoint
:连接点,所谓连接点是指那些被拦截到的点。在 spring 中,这些点指的是方法,就是可以被增强的方法叫做连接点
Pointcut
:所谓切入点是指我们要对哪些Joinpoint
进行拦截的定义对连接点进行配置的点就是切入点
Advice
:通知/增强,所谓通知是指拦截到Joinpoint
之后要做的事情就是通知Aspet
:切面,是指切入点和通知的结合Weaving
:织入,是指把增强应用到目标对象来创建新的代理对象的过程。
[](
)1.5、AOP 开发明确的事项
需要编写的内容
业务核心业务代码(目标类的目标方法)
编写切面类,切面类中有通知(增强功能方法)
在配置文件中,配置织入关系,即将哪些通知与哪些连接点进行结合
AOP 技术实现的内容
Spring 框架监控切入点方法的执行。一旦监控到切入点方法被运行,使用代理机制,动态创建目标对象的代理对象,根据通知类别,在代理对象的对应位置,将通知对应的功能织入,完成完整的代码逻辑运行。
AOP 底层使用哪种代理方式
在 spring 中,框架会根据目标类是否实现了接口来决定采用哪种动态代理的方式。
[](
)1.6、知识要点
aop:面向切面编程
aop 底层实现:基于 JDK 的动态代理和基于 Cglib 的动态代理
aop 的重点概念:
Pointcut :切入点,被增强的方法
Advice:通知/增强,封装增强业务逻辑的方法
Aspect:切面 = 切点 + 通知
Weaving:织入:将切点和通知结合的过程
开发明确事项
谁是切点(切点表达式配置)
谁是通知(切面类中的增强方法)
将切点和通知进行织入配置
[](
)2、基于 XML 的 AOP 开发
===============================================================================
导入 AOP 相关坐标
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.0.5.RELEASE</version>
</dependency>
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.8.4</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>5.0.2.RELEASE</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
创建目标接口和目标类(内部有切点)
public interface TargetInterface{
public void method();
}
public class Target implements TargetInterface {
@Override
public void method(){
System.out.println("save running...");
}
}
创建切面类(内部有增强方法)
public class MyAspect {
// 前置增强方法
public void before(){
System.out.println("前置增强");
}
}
将目标类和切面类的对象创建权交给 spring
<bean id="target" class="com.itheima.aop.Target"></bean>
<bean id="myAspect" class="com.itheima.aop.MyAspect"></bean>
在 applicationContext.xml 中配置织入关系
导入 aop 命名空间
配置切点表达式和前置增强的织入关系
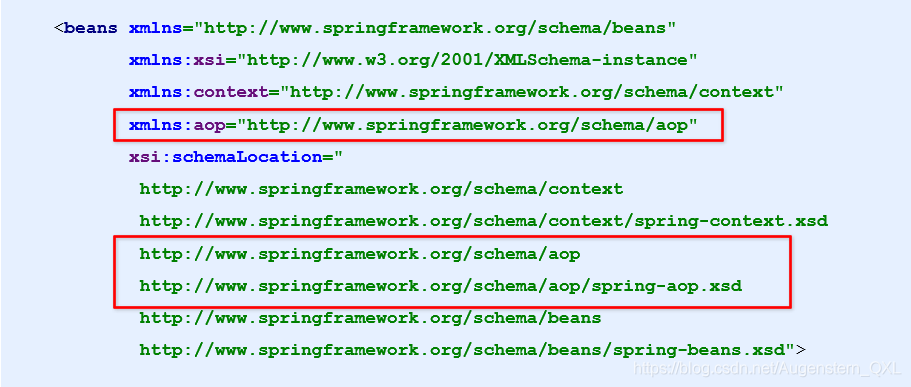
<aop:aspect ref="myAspect">
<aop:before
method="before"
pointcut="execution(public void com.itheima.aop.Target.method())"> </aop:before>
</aop:aspect>
</aop:config>
测试
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration("classpath:applicationContext.xml")
public class AopTest {
@Autowired
private TargetInterface target;
@Test
public void test1(){
target.save();
}
}
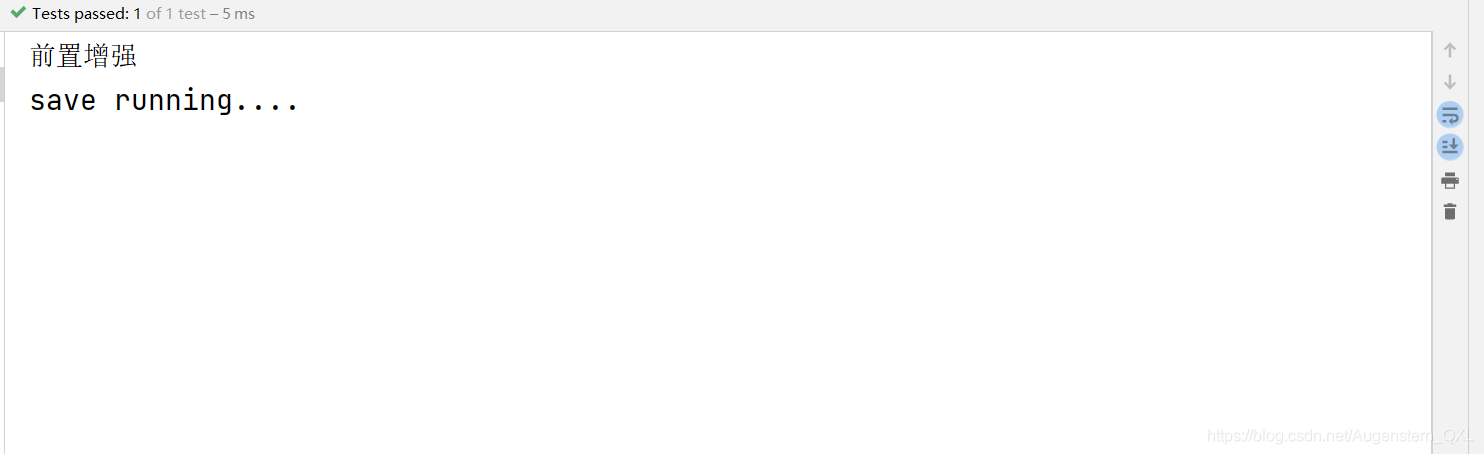
[](
)2.1、AOP 配置
[](
)2.1.1、aop:config
名称:
aop:config
类型:标签
归属:beans 标签
作用:设置 AOP:设置当前类为切面类
格式:
<beans>
aop:config……</aop:config>
aop:config……</aop:config>
</beans>
说明:一个 beans 标签中可以配置多个 aop:config 标签
[](
)2.1.2、aop:aspect
名称:
aop:aspect
类型:标签
归属:aop:config 标签
作用:设置具体的 AOP 通知对应的切入点
格式:
<aop:aspect ref="beanId">……</aop:aspect>
<aop:aspect ref="beanId">……</aop:aspect>
</aop:config>
说明:
一个 aop:config 标签中可以配置多个 aop:aspect 标签
基本属性:
ref :通知所在的 bean 的 id
[](
)2.1.3、aop:pointcut
名称:
aop:pointcut
类型:标签
归属:aop:config 标签、aop:aspect 标签
作用:设置切入点
格式:
<aop:pointcut id="pointcutId" expression="……"/>
<aop:pointcut id="pointcutId" expression="……"/>
</aop:aspect>
</aop:config>
说明:
一个 aop:config 标签中可以配置多个 aop:pointcut 标签,且该标签可以配置在 aop:aspect 标签内
基本属性:
id
:识别切入点的名称expression
:切入点表达式
[](
)2.1、切点表达式
切入点:
切入点描述的是某个方法
切入点表达式是一个快速匹配方法描述的通配格式,类似于正则表达式
表达式语法:
execution([修饰符] 返回值类型 包名.类名.方法名(参数))
execution(public void com.itheima.aop.Target.save())
访问修饰符可以省略
返回值类型、包名、类名、方法名可以使用星号 * 代表任意
包名和类名之间一个点 . 代表当前包下的类,两个点 … 表示当前包及其子包下的类
参数列表可以使用两个点 … 表示任意个数,任意类型的参数列表
*
:单个独立的任意符号,可以独立出现,也可以作为前缀或者后缀的匹配符出现
execution(public * com.itheima..UserService.find(*))
匹配 com.itheima 包下的任意包中的 UserService 类或接口中所有 find 开头的带有一个参数的方法
..
:多个连续的任意符号,可以独立出现,常用于简化包名与参数的书写
execution(public User com..UserService.findById(..))
匹配 com 包下的任意包中的 UserService 类或接口中所有名称为 findById 的方法
例如:
execution(public void com.itheima.aop.Target.method())
execution(void com.itheima.aop.Target.*(..))
execution(* com.itheima.aop..(..))
execution(* com.itheima.aop...(..))
execution(* ...*(..))
[](
)2.2、通知的类型
通知的配置语法:
<aop:通知类型 method="切面类中方法名" pointcut="切点表达式"></aop:通知类型>
| 名称 | 标签 | 说明 |
| --- | --- | --- |
| 前置通知 | <aop:before>
| 用于配置前置通知。指定增强的方法在切入点方法之前执行 |
| 后置通知 | <aop:after-returning>
| 用于配置后置通知。指定增强的方法在切入点方法之后执行 |
| 环绕通知 | <aop:around>
| 用于配置环绕通知。指定增强的方法在切入点方法之前和之后都执行 |
| 异常抛出通知 | <aop:throwing>
| 用于配置异常抛出通知。指定增强的方法在出现异常时执行 |
| 最终通知 | <aop:after>
| 用于配置最终通知。无论增强方式执行是否有异常都会执行 |
[](
)2.2.1、aop:before
名称:
aop:before
类型:标签
归属:aop:aspect 标签
作用:设置前置通知
格式:
<aop:aspect ref="adviceId">
<aop:before method="methodName" pointcut="……"/>
</aop:aspect>
说明:一个 aop:aspect 标签中可以配置多个 aop:before 标签
基本属性:
method
:在通知类中设置当前通知类别对应的方法pointcut
:设置当前通知对应的切入点表达式,与 pointcut-ref 属性冲突pointcut-ref
:设置当前通知对应的切入点 id,与 pointcut 属性冲突
[](
)2.2.2、aop:after-returning
名称:
aop:after-returning
类型:标签
归属:aop:aspect 标签
作用:设置返回后通知
格式:
<aop:aspect ref="adviceId">
<aop:after-returning me
thod="methodName" pointcut="……"/>
</aop:aspect>
说明:一个 aop:aspect 标签中可以配置多个 aop:after-returning 标签
基本属性:
method
:在通知类中设置当前通知类别对应的方法pointcut
:设置当前通知对应的切入点表达式,与 pointcut-ref 属性冲突pointcut-ref
:设置当前通知对应的切入点 id,与 pointcut 属性冲突
[](
)2.2.2、aop:around
名称:
aop:around
类型:标签
归属:aop:aspect 标签
作用:设置环绕通知
格式:
<aop:aspect ref="adviceId">
<aop:around method="methodName" pointcut="……"/>
</aop:aspect>
说明:一个 aop:aspect 标签中可以配置多个 aop:around 标签
基本属性:
method
:在通知类中设置当前通知类别对应的方法pointcut
:设置当前通知对应的切入点表达式,与 pointcut-ref 属性冲突pointcut-ref
:设置当前通知对应的切入点 id,与 pointcut 属性冲突
[](
)2.2.3、aop:after-throwing
名称:
aop:after-throwing
类型:标签
归属:aop:aspect 标签
作用:设置抛出异常后通知
格式:
<aop:aspect ref="adviceId">
<aop:after-throwing method="methodName" pointcut="……"/>
</aop:aspect>
说明:一个 aop:aspect 标签中可以配置多个 aop:after-throwing 标签
基本属性:
method
:在通知类中设置当前通知类别对应的方法pointcut
:设置当前通知对应的切入点表达式,与 pointcut-ref 属性冲突pointcut-ref
:设置当前通知对应的切入点 id,与 pointcut 属性冲突
[](
)2.2.4、示例
创建切面类(内部有增强方法)
public class MyAspect {
//前置增强
public void before(){
System.out.println("前置增强....");
}
//后置增强
public void afterReturning(){
System.out.println("后置增强....");
}
//环绕增强
//Proceeding JoinPoint:正在执行的连接点 == 切点
public Object around(ProceedingJoinPoint pjp){
System.out.println("环绕前增强....");
Object proceed = pjp.proceed();
System.out.println("环绕后增强....");
return proceed;
}
//异常抛出增强
public void afterThrowing(){
System.out.println("异常抛出增强....");
}
//最终通知增强
public void after(){
System.out.println("最终通知增强....");
}
}
配置切点表达式和增强的织入关系
<aop:aspect ref="myAspect">
<aop:before method="myPointcut" pointcut="execution(* com.itheima.aop..(..))"/>
<aop:around method="around" pointcut="execution(* com.itheima.aop..(..))" />
<aop:after-returning method="afterRetruning" pointcut="(* com itheima.aop..(..))" />
<aop:after-returning method="afterThrowing" pointcut="(* com itheima.aop..(..))" />
<aop:after method="after" pointcut="(* com itheima.aop..(..))" />
</aop:aspect>
</aop:config>
[](
)2.3、切点表达式的抽取
当多个增强的切点表达式相同时,可以将切点表达式进行抽取,在增强中使用 pointcut-ref
属性代替 pointcut
属性来引用抽取后的切点表达式
评论