【Spring Boot 12】看完这篇,nginxkeepalived 原理
i++;
jedis.set("test" + i, i + "");
}
} finally {// 关闭连接
jedis.close();
}
// 打印 1 秒内对 Redis 的操作次数
System.out.println("redis 每秒操作:" + i + "次");
}
-----------测试结果-----------
redis 每秒操作:10734 次
2、使用 Redis 连接池
跟数据库连接池相同,Java Redis 也同样提供了类?redis.clients.jedis.JedisPool
来管理我们的 Reids 连接池对象,并且我们可以使用?redis.clients.jedis.JedisPoolConfig
来对连接池进行配置,代码如下:
JedisPoolConfig poolConfig = new JedisPoolConfig();
// 最大空闲数
poolConfig.setMaxIdle(50);
// 最大连接数
poolConfig.setMaxTotal(100);
// 最大等待毫秒数
poolConfig.setMaxWaitMillis(20000);
// 使用配置创建连接池
JedisPool pool = new JedisPool(poolConfig, "localhost");
// 从连接池中获取单个连接
Jedis jedis = pool.getResource();
// 如果需要密码
//jedis.auth("password");
Redis 只能支持六种数据结构?(string/hash/list/set/zset/hyperloglog)的操作?,但在 Java 中我们通常以类对象为主,所以在 Redis 存储的数据结构月 java 对象之间进行转换,如自己编写一些工具类?比如一个角色对象的转换,还是比较容易的,但是涉及到许多对象的时候,这其中无论工作量还是工作难度都是很大的,所以总体来说,?就操作对象而言,使用 Redis 还是挺难的,好在 spring 对这些进行了封装和支持。
五、在 spring 中使用 Redis
=================
上面说到了 Redis 无法操作对象的问题,无法在那些基础类型和 Java 对象之间方便的转换,但是在 Spring 中,这些问题都可以通过使用 RedisTemplate 得到解决!
想要达到这样的效果,除了 Jedis 包以外还需要在 Spring 引入 spring-data-redis 包。
1、使用 spring 配置 JedisPoolConfig 对象
大部分的情况下,我们还是会用到连接池的,于是先用 Spring 配置一个 JedisPoolConfig 对象:
<bean id="poolConfig" class="redis.clients.jedis.JedisPoolConfig">
<property name="maxIdle" value="50"/>
<property name="maxTotal" value="100"/>
<property name="maxWaitMillis" value="20000"/>
</bean>
2、为连接池配置工厂模型
好了,我们现在配置好了连接池的相关属性,那么具体使用哪种工厂实现呢?在 Spring Data Redis 中有四种可供我们选择的工厂模型,它们分别是:
JredisConnectionFactory
JedisConnectionFactory
LettuceConnectionFactory
SrpConnectionFactory
我们这里就简单配置成 JedisConnectionFactory:
<bean id="connection
Factory" class="org.springframework.data.redis.connection.jedis.JedisConnectionFactory">
<property name="hostName" value="localhost"/>
<property name="port" value="6379"/>
<property name="poolConfig" ref="poolConfig"/>
</bean>
3、配置 RedisTemplate
普通的连接根本没有办法直接将对象直接存入 Redis 内存中,我们需要替代的方案:将对象序列化(可以简单的理解为继承 Serializable 接口)。我们可以把对象序列化之后存入 Redis 缓存中,然后在取出的时候又通过转换器,将序列化之后的对象反序列化回对象,这样就完成了我们的要求:
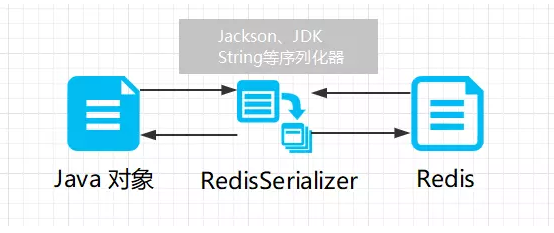
RedisTemplate 可以帮助我们完成这份工作,它会找到对应的序列化器去转换 Redis 的键值:
<bean id="redisTemplate"
class="org.springframework.data.redis.core.RedisTemplate"
p:connection-factory-ref="connectionFactory"/>
4、测试
首先编写好支持我们测试的 POJO 类:
/**
@author: 素小暖
@create: 2020-2-12
*/
public class Student implements Serializable{
private String name;
private int age;
/**
给该类一个服务类用于测试
*/
public void service() {
System.out.println("学生名字为:" + name);
System.out.println("学生年龄为:" + age);
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
然后编写测试类:
@Test
public void test() {
ApplicationContext context =
new ClassPathXmlApplicationContext("applicationContext.xml");
RedisTemplate redisTemplate = context.getBean(RedisTemplate.class);
Student student = new Student();
student.setName("我没有三颗心脏");
student.setAge(21);
redisTemplate.opsForValue().set("student_1", student);
Student student1 = (Student) redisTemplate.opsForValue().get("student_1");
student1.service();
}
运行结果:
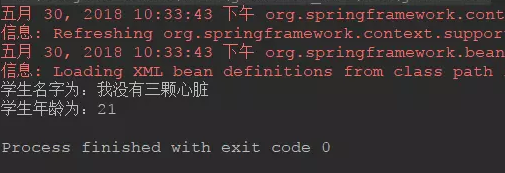
六、springboot 中使用 Redis
=====================
1、在 springboot 中添加 Redis 依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
2、添加配置文件 application.peoperties
REDIS (RedisProperties)
Redis 数据库索引(默认为 0)
spring.redis.database=0
Redis 服务器地址
spring.redis.host=localhost
Redis 服务器连接端口
spring.redis.port=6379
Redis 服务器连接密码(默认为空)
spring.redis.password=
连接池最大连接数(使用负值表示没有限制)
spring.redis.pool.max-active=8
连接池最大阻塞等待时间(使用负值表示没有限制)
spring.redis.pool.max-wait=-1
连接池中的最大空闲连接
spring.redis.pool.max-idle=8
连接池中的最小空闲连接
spring.redis.pool.min-idle=0
连接超时时间(毫秒)
spring.redis.timeout=0
3、测试访问
@RunWith(SpringJUnit4ClassRunner.class)
@SpringBootTest()
public class ApplicationTests {
@Autowired
private StringRedisTemplate stringRedisTemplate;
@Test
public void test() throws Exception {
// 保存字符串
stringRedisTemplate.opsForValue().set("aaa", "111");
Assert.assertEquals("111", stringRedisTemplate.opsForValue().get("aaa"));
评论