手把手教你应用三种工厂模式在 SpringIOC 中创建对象实例【案例详解】
}
二、通过静态工厂方法创建 Bean 实例
======================
在 Spring 中调用静态工厂方法创建 bean 是将对象创建的过程封装到静态方法中。当客户端需要对象时,只需要简单地调用静态方法,而不用关心创建对象的细节。
在 IOC 容器中,通过静态工厂方法声明创建 bean 的步骤是:
首先在 bean 的 class 属性里指定静态工厂类的全类名,
同时在 factory-method 属性里指定工厂方法的名称。
最后使用<constrctor-arg>元素为该方法传递方法参数。
接下来我们通过具体的代码来实现:
写一个静态工厂的实现类 AirplaneStaticFactory,其中实现造飞机的静态方法 get
AirPlane(String jzName),传入一个参数代表机长姓名。并将创建的飞机对象返回。
package com.spring.factory;
import com.spring.beans.AirPlane;
/**
飞机静态工厂
*/
public class AirplaneStaticFactory {
/**
private String fdj;//飞机发动机
private String yc;//机翼长度
private String jzName;//机长名
private String fjzName;//副机长名
private Integer personNum;//乘客数
*/
public static AirPlane getAirPlane(String jzName) {
AirPlane airPlane = new AirPlane();
airPlane.setFdj("太行");
airPlane.setYc("190.2");
airPlane.setJzName(jzName);
airPlane.setFjzName("小刚");
airPlane.setPersonNum(300);
return airPlane;
}
}
以上准备工作完成之后,就是通过静态工厂方法声明创建 bean 的实现了,按照上面的步骤在 ioc 容器中进行实现,
<!-- 通过静态工厂方法创建 airplane 实例
factory-method 指定工厂方法名 -->
<bean id="airplane01" class="com.spring.factory.AirplaneStaticFactory" factory-method="getAirPlane">
<constructor-arg name="jzName" value="静态机长 01"></constructor-arg>
</bean>
之后通过 ioc 容器的 getBean()方法就可以获取到创建的 bean 对象。测试如下:
//***利用工厂方法来创建 bean
ApplicationContext iocContext4 = new ClassPathXmlApplicationContext("ioc4.xml");
/**
利用工厂方法创建实例
*/
@Test
public void test10() {
// 利用静态工厂创建实例
AirPlane airPlane01 = (AirPlane)iocContext4.getBean("airplane01");
System.out.println(airPlane01);
// 利用实例工厂创建实例
AirPlane airPlane02 = (AirPlane)iocContext4.getBean("airplane02");
System.out.println(airPlane02);
// 利用自定义工厂创建实例
AirPlane airPlane03 = (AirPlane)iocContext4.getBean("myFactoryBean");
System.out.println(airPlane03);
}
测试结果:

三、通过实例工厂方法创建 Bean 实例
======================
实例工厂方法是将对象的创建过程封装到另外一个对象实例的方法里。当客户端需要请求对象时,只需要简单的调用该实例方法而不需要关心对象的创建细节。
实例工厂的实现步骤是
配置工厂类实例的 bean
在 factory-method 属性里指定该工厂方法的名称
使用 construtor-arg 元素为工厂方法传递方法参数
首先我们先写一个实现实例工厂的类 AirplaneInstanceFactory,在其中实现创建 bean 对象的实例方法 getAirPlane(String jzName),
package com.spring.factory;
import com.spring.beans.AirPlane;
/**
飞机实例工厂
*/
public class AirplaneInstanceFactory {
public AirPlane getAirPlane(String jzName) {
AirPlane airPlane = new AirPlane();
airPlane.setFdj("太行");
airPlane.setYc("190.2");
airPlane.setJzName(jzName);
airPlane.setFjzName("小刚");
airPlane.setPersonNum(300);
return airPlane;
}
}
之后在 IOC 容器中配置实现工厂类的 bean,指明是将哪一个实例工厂类实例化的。
<bean id="airplaneInstanceFacatory" class="com.spring.factory.AirplaneInstanceFactory"></bean>
现在就是将 bean 对象进行实例化的过程了,在 IOC 容器中,我们创建一个 bean 实例,调用实例工厂中的工厂方法,来对 bean 进行实例化,在这里我们需要使用 factory-method 属性里指定该工厂方法的名称,同时使用 construtor-arg 元素为工厂方法传递方法参数。具体实现是这样的:
<bean id="airplane02" class="com.spring.beans.AirPlane"
factory-bean="airplaneInstanceFacatory" factory-method="getAirPlane"
<constructor-arg name="jzName" value="实例机长 01"></constructor-arg>
</bean>
现在通过实例工厂创建 bean 对象的过程就结束了,通过获取到 ioc 容器中的 bean 对象进行测试:
//***利用工厂方法来创建 bean
ApplicationContext iocContext4 = new ClassPathXmlApplicationContext("ioc4.xml");
/**
利用工厂方法创建实例
*/
@Test
public void test10() {
// 利用静态工厂创建实例
AirPlane airPlane01 = (AirPlane)iocContext4.getBean("airplane01");
System.out.println(airPlane01);
// 利用实例工厂创建实例
AirPlane airPlane02 = (AirPlane)iocContext4.getBean("airplane02");
System.out.println(airPlane02);
// 利用自定义工厂创建实例
AirPlane airPlane03 = (AirPlane)iocContext4.getBean("myFactoryBean");
System.out.println(airPlane03);
}
测试结果:
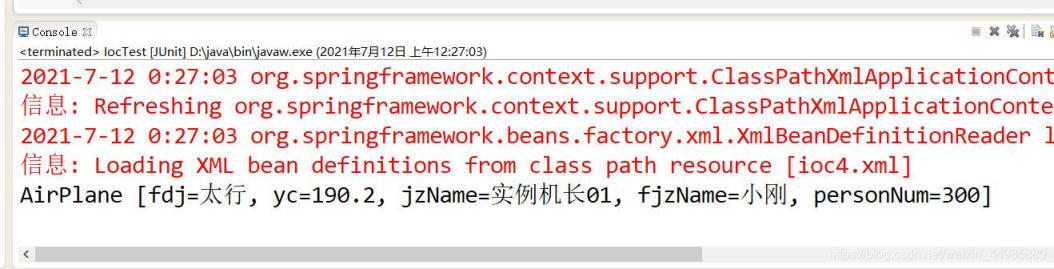
四、通过自定义的 factoryBean 来创建 bean 对象
================================
通过自定义的 factoryBean 来创建 bean 对象时,我们需要在工厂类上实现 factoryBean 接口,
Spring 中有两种类型的 bean,一种是普通 bean,另一种是工厂 bean,即 FactoryBean。
工厂 bean 跟普通 bean 不同,其返回的对象不是指定类的一个实例,其返回的是该工厂 bean 的 getObject 方法所返回的对象。
工厂 bean 必须实现 org.springframework.beans.factory.FactoryBean 接口,使用 factoryBean 接口的一个好处就是只要是实现这个接口的类,spring 都会认为是工厂。
该接口规定了三个方法:
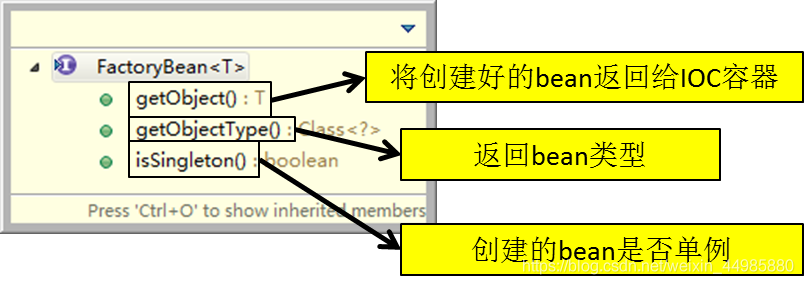
我们先来创建一个自定义的、容器能够认识的 factoryBean 类,并且重写其中的三个方法。
package com.spring.factory;
import org.springframework.beans.factory.FactoryBean;
import com.spring.beans.AirPlane;
import com.spring.beans.Book;
/**
创建一个实例工厂的接口
创建一个容器能够认识的 factoryBean 类,
只要是这个接口实现的类,spring 都会认为是工厂
*/
public class MyFactoryBeanImplements implements FactoryBean<AirPlane>{
/**
工厂方法,返回创建的对象
*/
@Override
public AirPlane getObject() throws Exception {
// TODO Auto-generated method stub
AirPlane airPlane = new AirPlane();
airPlane.setFdj("太行-2");
airPlane.setYc("290.2");
airPlane.setJzName("自定义机长 01");
airPlane.setFjzName("小刚");
airPlane.setPersonNum(300);
return airPlane;
}
/**
返回对象的类型
*/
@Override
public Class<?> getObjectType() {
// TODO Auto-generated method stub
return AirPlane.class;
}
/**
isSingleton 是单例吗?
false 不是单例
true 是单例
评论