模块化开发一:架构搭建 (1)
[八、RxLifecycle](about:blank#%E5%85%
AB%E3%80%81RxLifecycle)
一、Module 与独立 APP 切换
================
1、在 gradle.properties 文件中设置一个参数:isUserModule = false
当设置为 false 时,作为独立 APP 运行,设置为 true 时,作为 library 使用
Project-wide Gradle settings.
IDE (e.g. Android Studio) users:
Gradle settings configured through the IDE will override
any settings specified in this file.
For more details on how to configure your build environment visit
http://www.gradle.org/docs/current/userguide/build_environment.html
Specifies the JVM arguments used for the daemon process.
The setting is particularly useful for tweaking memory settings.
org.gradle.jvmargs=-Xmx1536m
When configured, Gradle will run in incubating parallel mode.
This option should only be used with decoupled projects. More details, visit
http://www.gradle.org/docs/current/userguide/multi_project_builds.html#sec:decoupled_projects
org.gradle.parallel=true
isUserModule = false
2、在 UserCenter 的 build.gradle 中加入以下判断:
if (isUserModule.toBoolean()) {
apply plugin: 'com.android.library'
} else {
apply plugin: 'com.android.application'
}
3、创建两个 manifest 文件,因为两种情况下需要读取的 manifest 文件是不同的
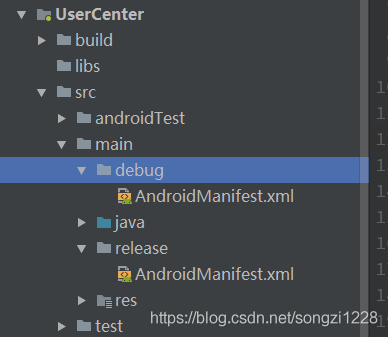
这是 debug 中的:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.gskotlin.user">
<application
android:allowBackup="true"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".ui.activity.RegisterActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
这是 release 中的
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.gskotlin.user">
<application
android:allowBackup="true"
android:label="@string/app_name"
android:supportsRtl="true">
<activity android:name=".ui.activity.RegisterActivity"/>
</application>
</manifest>
4、在 UserCenter 的 build.gradle 中的 buildTypes 同级下面配置 sourceSets,如下:
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
sourceSets {
main {
if (isUserModule.toBoolean()) {
manifest.srcFile 'src/main/release/AndroidManifest.xml'
//release 模式下排除 debug 文件夹中的所有 Java 文件
java{
exclude'debug/**'
}
} else {
manifest.srcFile 'src/main/debug/AndroidManifest.xml'
}
}
}
5、在 App 的 build.gradle 中的依赖中配置
if(isUserModule.toBoolean()){
implementation project(':UserCenter')
}
二、踩坑
====
1、在 kotlin 多模块开发中是不能使用 butterknife 的
================================
因为会报编译错误:Unable to find resource ID
错误原因:Module 资源 ID 与主工程资源 ID 不一致
Github issue:https://github.com/JakeWharton/butterknife/issues/974
解决方案:不使用 butterknife,使用 kotlin-android-extensions
视图绑定,可直接使用 xml 中的 ID 操作该控件
插件级别,无需引入第三方库
无需定义变量,极大地减少代码量
适用于 Activity,Fragment,Adapter 以及自定义 View
配置:apply plugin:'kotlin-android-extensions'
使用:xml 中:
<Button
android:id="@+id/mRegisterBtn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="register"
android:textAllCaps="false"
android:layout_marginTop="30dp"/>
代码中:
package com.gskotlin.user.ui.activity
import android.os.Bundle
import android.support.v7.app.AppCompatActivity
import android.widget.Toast
import com.gskotlin.user.R
import kotlinx.android.synthetic.main.activity_register.*
class RegisterActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_register)
mRegisterBtn.setOnClickListener {
Toast.makeText(this, "haha", Toast.LENGTH_SHORT).show()
}
}
}
三、Anko
======
Github 地址:https://github.com/Kotlin/anko
这是 kotlin 中的一个扩展库
3.1、组成部分:
Anko Commons
Anko Layouts
Anko SQLite
Anko Coroutines
3.1.1? Anko Commons 配置
依赖包:compile "org.jetbrains.anko:anko-commons:$anko_version"
v4 依赖包:compile "org.jetbrains.anko:anko-commons:$anko_version"
3.1.2? Anko Commons 使用
跳转类:startActivity,intent 等
提示类:toast、dialog 等
日志类:verbose()、debug()、info()、warn()、error()等
尺寸类:dip()、sp()、px2dp()、px2sp()、dimen()等
3.1.3? Anko Layouts 配置:
依赖包:compile "org.jetbrains.anko:anko-sdk25:$anko_version"
v7 依赖包:compile "org.jetbrains.anko:anko-appcompat-v7:$anko_version"
事件依赖包:compile "org.jetbrains.anko:anko-sdk25-coroutines:$anko_version"
v7 事件依赖包:compile "org.jetbrains.anko:anko-appcompat-v7-coroutines:$anko_version"
3.1.4? Anko Layouts 使用:
verticlaLayout{
padding = dip(30)
editText{
hint = "Name"
textSize = 24f
}
editText{
hint = "Password"
textSize = 24f
}
button{
textSize = 26f
onClick{toast("Hello")}
评论