《Spring 实战》读书笔记 - 第 2 章 装配 Bean,kafka 调优面试
CD 的一个实现类,使用 @Component 告知 Spring 要为这个类创建 bean。
package com.springinaction;
import org.springframework.stereotype.Component;
@Component
public class SgtPeppers implements CompactDisc {
private String title = "Sgt. Pepper's Lonely Hearts Club Band";
private String artist = "The Beatles";
@Override
public void play() {
System.out.println("Playing " + title + " by " + artist);
}
}
使用基于 Java 的 Spring 配置,使用 @ComponentScan 默认会扫描与配置类相同的包。
package com.springinaction;
import org.springframework.context.annotation.*;
@Configuration
@ComponentScan
public class CDPlayerConfig {
}
如果要是用 XML 来启用组件扫描的话,可以使用以下代码
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:Context = "http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<Context:component-scan base-package="com.springinaction" />
</beans>
使用 JUnit 进行测试,测试 CD 的实现类是否被 Spring 自动创建
package com.springinaction;
import static org.junit.Assert.*;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes=CDPlayerConfig.class)
public class CDPlayerTest {
@Autowired
private CompactDisc sgtPeppers;
@Test
public void cdShouldNotBeNull(){
assertNotNull(sgtPeppers);
}
}
测试成功

此例展示了 Spring 创建 bean 的过程以及测试,其中 SgtPeppers 的 bean 并没有明确设置 ID,但 Spring 会自动指定,默认为 sgtPeppers,首字母为小写。如果想为这个 bean 设置不同的 ID,可以用以下方式:
@Component("lonelyHeartsClub")
public class SgtPeppers implements CompactDisc {
.......
}
到现在,我们没有为 @ComponentScan 设置任何属性。这意味这,按照默认规则,它会以配置类所在的包作为基础包(basepackage)来扫描组件。
如果我们想要将配置类放在单独的包中,使其与其他的应用代码区分开来。那么可用以下方式设置:
@Configuration
@ComponentScan("com.springinaction")
public class CDPlayerConfig { }
或者更加清晰地表明所设置的是基础包
@Configuration
@ComponentScan(basePackages="com.springinaction")
public class CDPlayerConfig { }
也可以设置多个基础包
@Configuration
@ComponentScan(basePackages={"com.springinaction", "video"})
public class CDPlayerConfig { }
除了以上的方式还可以将其指定为包中所包含的类或接口:
@Configuration
@ComponentScan(basePackageClasses={CDPlayer.class, DVDPlayer.class})
public class CDPlayerConfig { }
这些类所在的包将会作为组件扫描的基础包。
系统中大部分类还是存在相互依赖的现象,所以我们就需要了解自动装配。
简单来说,自动装配就是让 Spring 自动满足 bean 依赖的一种方法,在满足依赖的过程中,会在 Spring 应用上下文中寻找匹配某个 bean 需求的其他 bean。为了声明要进行自动装配,我们可以借助 Spring 的 @Autowired 注解。
比如实现 CD 播放器的类,播放器接口是有一个 play 方法
package com.springinaction;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class CDPlayer implements MediaPlayer {
private CompactDisc compactDisc;
@Autowired
public CDPlayer(CompactDisc compactDisc){
this.compactDisc = compactDisc;
}
@Override
public void play() {
compactDisc.play();
}
}
以上使用的是自动装配构造器,还能用在属性 Setter 方法上
@Autowired
public void setCompactDisc(CompactDisc compactDisc){
this.compactDisc = compactDisc;
}
如果没有匹配的 bean,那么在应用上下文创建时,Spring 会抛出一个异常。为了避免异常的出现,你可以将 @Autowired 的 required 属性
设置为 false:
@Autowired(required=false)
public CDPlayer(CompactDisc compactDisc){
this.compactDisc = compactDisc;
}
如果有多个 bean 都能满足依赖关系的话,Spring 将会抛出一个异常。
另:@Autowired 可以用 @Inject 代替
现在,我们已经在 CDPlayer 的构造器中添加了 @Autowired 注解,Spring 将把一个可分配给 CompactDisc 类型的 bean 自动注入进来,为了验证,我们进行测试
package com.springinaction;
import static org.junit.Assert.*;
import org.junit.Test;
import org.junit.Rule;
import org.junit.contrib.java.lang.system.SystemOutRule;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes=CDPlayerConfig.class)
public class CDPlayerTest {
@Rule
public final SystemOutRule log = new SystemOutRule().enableLog();
@Autowired
private CompactDisc sgtPeppers;
@Autowired
private CDPlayer cdPlayer;
@Test
public void cdShouldNotBeNull(){
assertNotNull(sgtPeppers);
}
@Test
public void play(){
cdPlayer.play();
assertEquals(
"Playing Sgt. Pepper's Lonely Hearts Club Band " +
"by The Beatles\n",
log.getLog()
);
}
}
测试成功

当自动化的方案行不通的时候,就必须采用显示装配的方式。显示装配有两种可选方案:JavaConfig 和 XML。其中 JavaConfig 是更好的方案,因为它更为强大、类型安全并且对重构友好。
继续上面的示例代码,首先创建配置类
package com.springinaction;
import org.springframework.context.annotation.*;
@Configuration
public class CDPlayerConfig {
}
@Configuration 注解表明这个类是一个配置类,该类应该包含在 Spring 应用上下文中如何创建 bean 的细节。
我们移除之前的 @ComponentScan,使用显示配置
@Bean
public CompactDisc sgtPeppers(){
return new SgtPeppers();
}
@Bean 注解会告诉 Spring 这个方法将会返回一个对象,该对象要注册为 Spring 应用上下文中的 bean。方法体中包含了最终产生 bean 实例的逻辑。
默认情况下,bean 的 ID 与带有 @Bean 注解的方法名一样。在本例中,bean 的名字将会是 sgtPeppers。也可以重命名
@Bean(name="lonelyHeartsClubBand")
public CompactDisc sgtPeppers(){
return new SgtPeppers();
}
下面我们将 CD 注入到 CDPlayer 中
@Bean
public CDPlayer cdPlayer(CompactDisc compactDisc){
return new CDPlayer(compactDisc);
}
需要注意的有两点:
构造器中不能用 new 创建的对象(这个对象的类是已经在 Spring 中被声明的),因为 Spring 会拦截所有对已声明对象的调用,并确保直接返回该方法所创建的 bean。这是由于 Spring 所创建的 bean 都是单例的。
compactDisc 会在 Spring 中寻找已经实现 CompactDisc 的 bean。
先来看最简单的 SpringXML 配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context">
</beans>
借助 Spring Tool Suite 创建 XML 配置文件创建和管理 Spring XML 配置文件的一种简便方式是使用 Spring Tool Suite(https://spring.io/tools/sts)。
我们来简单声明一个 bean
<bean id="compactDisc" class="com.springinaction.SgtPeppers" />
下面我们来看一些特征
不再需要直接负责创建 SgtPeppers 的实例,而在基于 JavaConfig 的配置中需要。当 Spring 发现这个元素时,它将会调用 SgtPeppers 的默认构造器来创建 bean。
这个 bean 中,我们将 bean 的类型以字符串的形式设置了 class 属性中。没有类型检查。
XML 声明 DI 时,会有很多种可选的配置方案和风格。具体到构造器注入,有两种基本的配置方案可供选择:
<constructor-arg>
元素使用 Spring3.0 所引入的 c-命名空间
先来看一下他们各组如何注入 bean 引用
构造器注入 bean 引用
<bean id="cdPlay" class="com.springinaction.CDPlayer" >
<constructor-arg ref="compactDisc"/>
</bean>
当 Spring 遇到这个<bean>
元素时,它会创建一个 CDPlayer 实例。<constructor-arg>
元素会告知 Spring 要将一个 ID 为 compactDisc 的 bean 引用传递到 CDPlayer 的构造器中。
作为替代方案,也可以使用 Spring 的 c-命名空间。以下是 XML 的顶部声明其模式。
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:c="http://www.springframework.org/schema/c"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
....
</beans>
在 c-命名空间和模式声明之后,我们就可以使用它来声明构造器参数了,如下所示:
<bean id="cdPlay" class="com.springinaction.CDPlayer" c:cd-ref="compactDisc" />
c 的属性的说明如下:
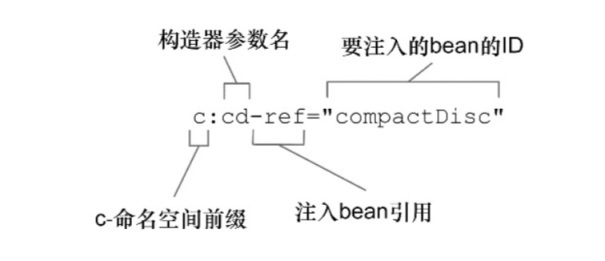
spring 的 c 标签的构造器属性
属性名以“c:”开头,也就是命名空间的前缀。接下来就是要装配的构造器参数名,在此之后是“-ref”,这是一个命名的约定,它会告诉 Spring,正在装配的是一个 bean 的引用,这个 bean 的名字是 compactDisc,而不是字面量“compactDisc”。
还可以将其中引用参数的名称替换成位置信息:
<bean id="cdPlay" class="com.springinaction.CDPlayer" c:_0-ref="compactDisc" />
其中“0”代表参数的索引。
下面我们看一下如何将字面量如何装配到对象中去。
我们先新建一个 CompactDisc 的实现:
package com.springinaction;
import java.util.List;
public class BlankDisc implements CompactDisc {
private String title;
private String artist;
public BlankDisc(String title, String artist){
this.title = title;
this.artist = artist;
}
@Override
public void play() {
System.out.println("Playing " + title + " by " + artist);
}
}
这个实现类中,cd 名称和艺术家是可以从构造器注入的。比 SgtPeppers 的硬编码要灵活。现在我们将已有的 SgtPeppers 替换为这个类:
<bean id="compactDisc" class="com.springinaction.BlankDisc" >
<constructor-arg value="Sgt. Pepper's Lonely Hearts Club Band"/>
<constructor-arg value="The Beatles" />
</bean>
还可以替换为 c 标签写法:
<bean id="compactDisc" class="com.springinaction.BlankDisc" c:title="Sgt. Pepper's Lonely Hearts Club Band" c:artist="The Beatles"/>
也可以用索引:
<bean id="compactDisc" class="com.springinaction.BlankDisc" c:_0="Sgt. Pepper's Lonely Hearts Club Band" c:_1="The Beatles"/>
通常<constructor-arg>
和 c-命名空间的功能是相同的,但一种情况是<constructor-arg>
能实现,但 c-却做不到。
现在我们将 BlankDisc 加入多磁道的属性,并在构造器中能注入。
package com.springinaction;
import java.util.List;
public class BlankDisc implements CompactDisc {
private String title;
private String artist;
private List<String> tracks;
public BlankDisc(String title, String artist, List<String> tracks ){
this.title = title;
this.artist = artist;
this.tracks = tracks;
}
@Override
public void play() {
System.out.println("Playing " + title + " by " + artist);
for (String track : tracks){
System.out.println("-Track: " + track);
}
}
}
最简单的方式是将列表设置为 null
<bean id="compactDisc" class="com.springinaction.BlankDisc" >
<constructor-arg value="Sgt. Pepper's Lonely Hearts Club Band"/>
<constructor-arg value="The Beatles" />
<constructor-arg><null/></constructor-arg>
</bean>
更好的解决方法是提供一个磁道名称的列表。
方案一:
<bean id="compactDisc" class="com.springinaction.BlankDisc" >
<constructor-arg value="Sgt. Pepper's Lonely Hearts Club Band"/>
<constructor-arg value="The Beatles" />
<constructor-arg>
<list>
评论