前端之数据结构(三)集合和字典
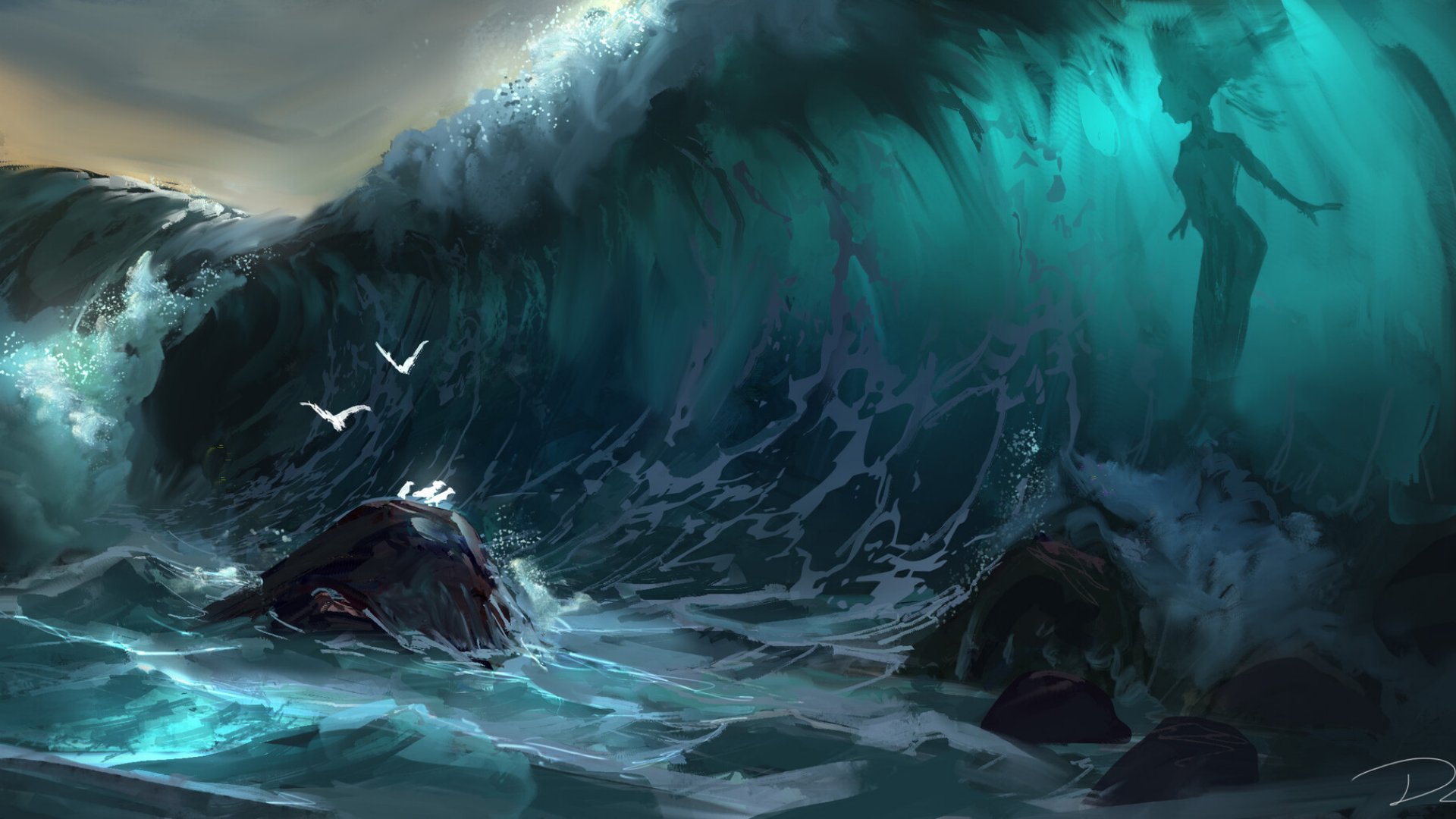
承接上文,继续,今天的集合和字典介绍。
集合
一种无序且唯一的数据结构。
在 ES6 中就有集合,名 Set。
集合的常用操作:去重、判断某元素是否在集合中、求交集等等...
去重
复制代码
判断元素是否在集合中
复制代码
求交集
复制代码
求差集
复制代码
Set 基本操作
使用
Set
对象:new、add、delete、has、size
迭代
Set
:多种迭代方法、Set
与Array
互转、求交集/差集迭代
Set
的key
复制代码
迭代
Set
的value
复制代码
迭代
Set
的key 和 value
复制代码
我们可以发现
Set
的key 和 value
是一样的。
Set 转 Array
复制代码
字典
与集合类似,字典也是一种存储唯一值的数据结构,但它是以
键值对
的形式来存储。在 ES6 中就有集合,名 Map。
字典的常用操作:键值对的增删改查。
Set 的基本操作
复制代码
时间复杂度都是 O(1)。
求交集
复制代码
今天就到这里,明天见!
评论