spring4.1.8 扩展实战之三:广播与监听
- 2022 年 6 月 14 日
本文字数:27258 字
阅读完需:约 89 分钟
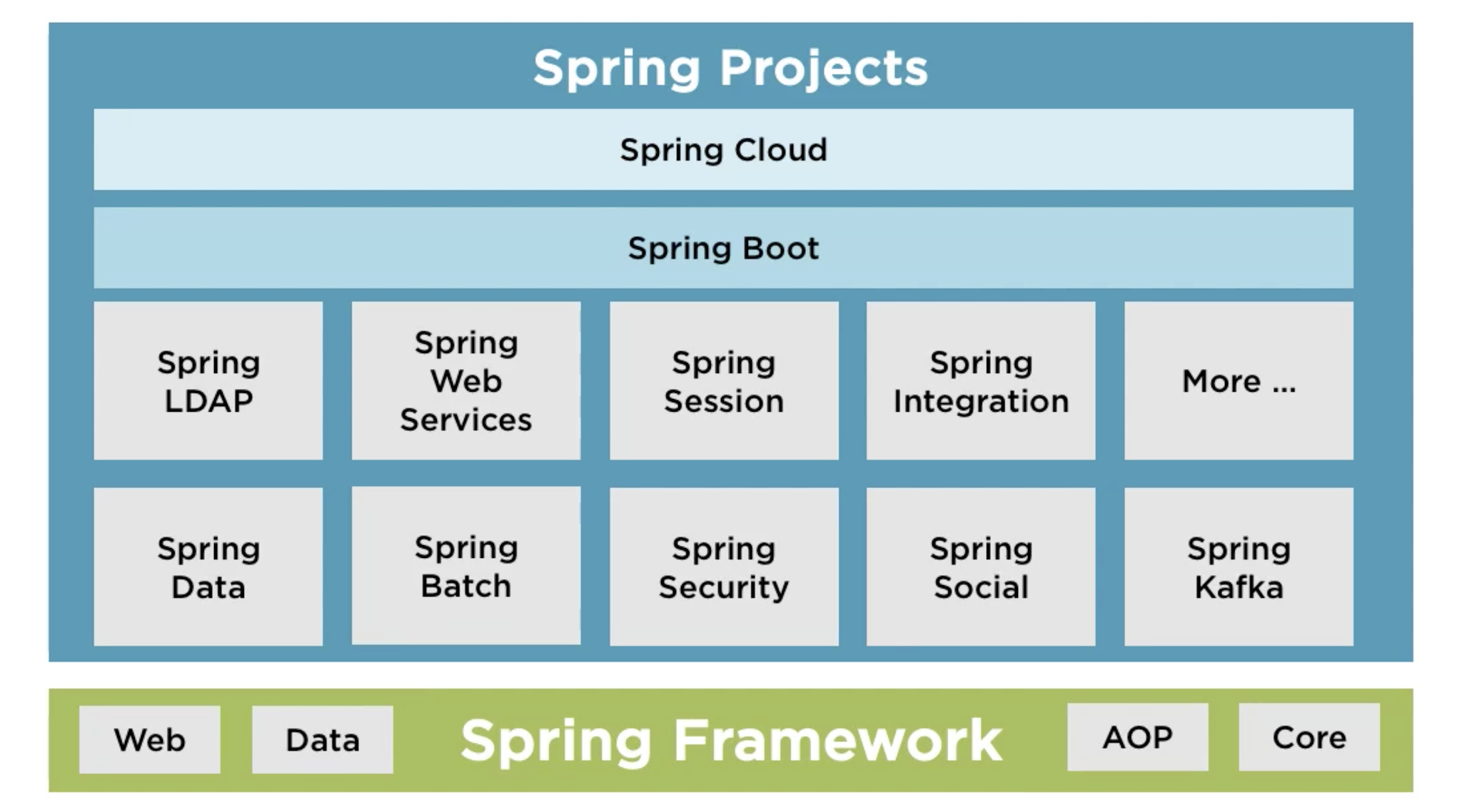
欢迎访问我的 GitHub
这里分类和汇总了欣宸的全部原创(含配套源码):https://github.com/zq2599/blog_demos
提到广播与监听,我们常常会想到 RabbitMQ、Kafka 等消息中间件,这些常用于分布式系统中多个应用之间,有时候应用自身内部也有广播和监听的需求(例如某个核心数据发生变化后,有些业务模块希望立即被感知),这时候 spring 提供的基于 ApplicationContext 的广播与监听就派上用场了,接下来我们从原理到实践,来了解 spring 提供的这套机制吧。
全文概览
总的来说本章内容由以下部分构成:
学习广播服务的核心接口;
spring 的容器内广播和监听的源码查看;
spring 是如何支持自定义广播的;
spring 是如何支持自定义监听的;
开发一个自定义广播;
开发一个自定义监听;
ApplicationEventPublisher:广播服务的核心能力
提到 spring 容器中的广播和监听,就不得不先看看 ApplicationEventPublisher 这个接口,今天的故事都是围绕此君展开:
@FunctionalInterface
public interface ApplicationEventPublisher {
/**
* Notify all <strong>matching</strong> listeners registered with this
* application of an application event. Events may be framework events
* (such as RequestHandledEvent) or application-specific events.
* @param event the event to publish
* @see org.springframework.web.context.support.RequestHandledEvent
*/
default void publishEvent(ApplicationEvent event) {
publishEvent((Object) event);
}
/**
* Notify all <strong>matching</strong> listeners registered with this
* application of an event.
* <p>If the specified {@code event} is not an {@link ApplicationEvent},
* it is wrapped in a {@link PayloadApplicationEvent}.
* @param event the event to publish
* @since 4.2
* @see PayloadApplicationEvent
*/
void publishEvent(Object event);
}
从上述代码可以看出,发送消息实际上就是在调用 ApplicationEventPublisher 接口实现类的 publishEvent 方法,接下来看看类图,了解在 spring 容器的设计中 ApplicationEventPublisher 的定位:
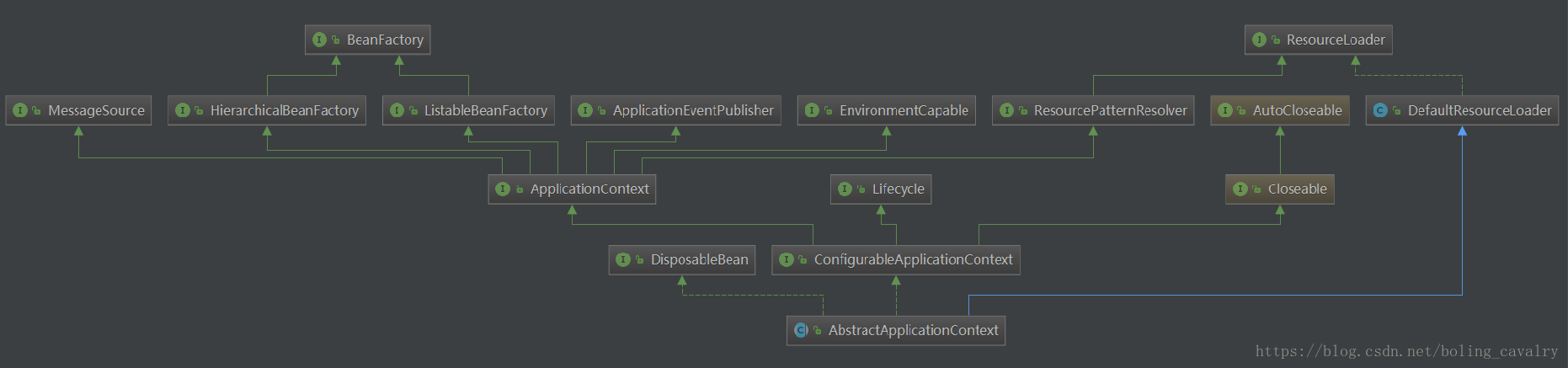
从上图可见,ApplicationContext 继承了 ApplicationEventPublisher,那么 ApplicationContext 的实现类自然也就具备了发送广播的能力,按照这个思路去看一下关键的抽象类 AbstractApplicationContext,果然找到了方法实现:
@Override
public void publishEvent(ApplicationEvent event) {
publishEvent(event, null);
}
如何广播一条消息
来看一个具体的广播的例子吧,spring 容器初始化结束的时候,会执行 AbstractApplicationContext 类的 finishRefresh 方法,里面就会广播一条代表初始化结束的消息,代码如下:
protected void finishRefresh() {
// Clear context-level resource caches (such as ASM metadata from scanning).
clearResourceCaches();
// Initialize lifecycle processor for this context.
initLifecycleProcessor();
// Propagate refresh to lifecycle processor first.
getLifecycleProcessor().onRefresh();
// 广播一条消息,类型ContextRefreshedEvent代表spring容器初始化结束
publishEvent(new ContextRefreshedEvent(this));
// Participate in LiveBeansView MBean, if active.
LiveBeansView.registerApplicationContext(this);
}
继续深入 publichEvent 方法内部,看到的代码如下图所示:
上图中的绿色部分的判断不成立,初始化过程中的 registerListeners 方法就会把成员变量 earlyApplicationEvents 设置为空,因此广播消息执行的是 getApplicationEventMulticaster().multicastEvent(applicationEvent, eventType),在 ApplicationContext 的实现类中,真正的广播操作交给了成员变量 applicationEventMulticaster 来完成,没有特殊配置的话,applicationEventMulticaster 是 SimpleApplicationEventMulticaster 类型的实例(详情请看 initApplicationEventMulticaster 方法);
打开的源码,如下所示,是否有种豁然开朗的感觉?广播的实现是如此简单明了,找到已注册的 ApplicationListener,逐个调用 invokeListener 方法,将 ApplicationListener 和事件作为入参传进去就完成了广播;
@Override
public void multicastEvent(final ApplicationEvent event, @Nullable ResolvableType eventType) {
ResolvableType type = (eventType != null ? eventType : resolveDefaultEventType(event));
for (final ApplicationListener<?> listener : getApplicationListeners(event, type)) {
Executor executor = getTaskExecutor();
if (executor != null) {
executor.execute(() -> invokeListener(listener, event));
}
else {
invokeListener(listener, event);
}
}
}
看到这里,invokeListener 方法所做的事情已经很容易猜到了,ApplicationListener 是代表监听的接口,只要调用这个接口的方法并且将 event 作为入参传进去,那么每个监听器就可以按需要自己来处理这条广播消息了,看了代码发现我们的猜测是正确的:
private void doInvokeListener(ApplicationListener listener, ApplicationEvent event) {
try {
//这里就是监听器收到广播时做的事情
listener.onApplicationEvent(event);
}
catch (ClassCastException ex) {
String msg = ex.getMessage();
if (msg == null || matchesClassCastMessage(msg, event.getClass().getName())) {
// Possibly a lambda-defined listener which we could not resolve the generic event type for
// -> let's suppress the exception and just log a debug message.
Log logger = LogFactory.getLog(getClass());
if (logger.isDebugEnabled()) {
logger.debug("Non-matching event type for listener: " + listener, ex);
}
}
else {
throw ex;
}
}
}
另外还有个细节需要补充,这是在后面做自定义广播的时候考虑到的:**如果多线程同时发广播,会不会有线程同步的问题?**发送广播的源码一路看下来,发现操作全部在发送的线程中执行的,对各种成员变量的操作也没有出现线程同步问题,唯一有可能出现问题的地方在获取 ApplicationListener 的时候,这里用到了缓存,有缓存更新的逻辑,而 spring 已经做好了锁来确保线程同步(双重判断也做了),来看看源码,注意中文注释:
protected Collection<ApplicationListener<?>> getApplicationListeners(
ApplicationEvent event, ResolvableType eventType) {
Object source = event.getSource();
Class<?> sourceType = (source != null ? source.getClass() : null);
ListenerCacheKey cacheKey = new ListenerCacheKey(eventType, sourceType);
//retrieverCache是个ConcurrentHashMap
ListenerRetriever retriever = this.retrieverCache.get(cacheKey);
if (retriever != null) {
return retriever.getApplicationListeners();
}
//如果没有从缓存中取到,就要获取了数据再返回
if (this.beanClassLoader == null ||
(ClassUtils.isCacheSafe(event.getClass(), this.beanClassLoader) &&
(sourceType == null || ClassUtils.isCacheSafe(sourceType, this.beanClassLoader)))) {
// 这里有锁
synchronized (this.retrievalMutex) {
retriever = this.retrieverCache.get(cacheKey);
//抢到锁之后再做一次判断,因为有可能在前面BLOCK的时候,另一个抢到锁的线程已经设置好了缓存
if (retriever != null) {
return retriever.getApplicationListeners();
}
retriever = new ListenerRetriever(true);
Collection<ApplicationListener<?>> listeners =
retrieveApplicationListeners(eventType, sourceType, retriever);
this.retrieverCache.put(cacheKey, retriever);
return listeners;
}
}
else {
// No ListenerRetriever caching -> no synchronization necessary
return retrieveApplicationListeners(eventType, sourceType, null);
}
}
看到这里,我们对 spring 容器内的广播与监听已经有所了解,小结如下:
广播的核心接口是 ApplicationEventPublisher;
我们见过了如何发起一条广播,监听器如何响应广播;
接下来还不能马上动手实战,我们还有两件事情要想弄清楚:
我想发自定义的广播消息,如何具备这个发送能力?
我想接收特定的广播,如何具备这个接收能力?
接下来,继续结合 spring 源码去找上面两个问题的答案;
源码分析(如何具备消息发送能力)
先看消息生产相关的源码,在上一章《spring4.1.8扩展实战之二:Aware接口揭秘》中,我们看到 spring 容器初始化的时候会对实现了 Aware 接口的 bean 做相关的特殊处理,其中就包含 ApplicationEventPublisherAware 这个与广播发送相关的接口,代码如下(ApplicationContextAwareProcessor.java):
private void invokeAwareInterfaces(Object bean) {
if (bean instanceof Aware) {
if (bean instanceof EnvironmentAware) {
((EnvironmentAware) bean).setEnvironment(this.applicationContext.getEnvironment());
}
if (bean instanceof EmbeddedValueResolverAware) {
((EmbeddedValueResolverAware) bean).setEmbeddedValueResolver(this.embeddedValueResolver);
}
if (bean instanceof ResourceLoaderAware) {
((ResourceLoaderAware) bean).setResourceLoader(this.applicationContext);
}
//如果想在应用内发送广播,需要开发一个实现了ApplicationEventPublisherAware接口的bean,就会在此被注入一个ApplicationEventPublisher对象,借助这个对象就能发送广播了
if (bean instanceof ApplicationEventPublisherAware) {
((ApplicationEventPublisherAware) bean).setApplicationEventPublisher(this.applicationContext);
}
if (bean instanceof MessageSourceAware) {
((MessageSourceAware) bean).setMessageSource(this.applicationContext);
}
if (bean instanceof ApplicationContextAware) {
((ApplicationContextAware) bean).setApplicationContext(this.applicationContext);
}
}
}
由以上代码可见,我们可以创建一个 bean,实现了 ApplicationEventPublisherAware 接口,那么该 bean 的 setApplicationEventPublisher 方法就会被调用,通过该方法可以接收到 ApplicationEventPublisher 类型的入参,借助这个 ApplicationEventPublisher 就可以发消息了;
源码分析(如何具备消息接收能力)
在 spring 容器初始化的时候,AbstractApplicationContext 类的 prepareBeanFactory 方法中为所有 bean 准备了一个后置处理器 ApplicationListenerDetector,来看看它的 postProcessAfterInitialization 方法的代码,也就是 bean 在实例化之后要做的事情:
@Override
public Object postProcessAfterInitialization(Object bean, String beanName) {
if (bean instanceof ApplicationListener) {
// potentially not detected as a listener by getBeanNamesForType retrieval
Boolean flag = this.singletonNames.get(beanName);
if (Boolean.TRUE.equals(flag)) {
//注册监听器,其实就是保存在成员变量applicationEventMulticaster的成员变量defaultRetriever的集合applicationListeners中
this.applicationContext.addApplicationListener((ApplicationListener<?>) bean);
}
else if (Boolean.FALSE.equals(flag)) {
if (logger.isWarnEnabled() && !this.applicationContext.containsBean(beanName)) {
// inner bean with other scope - can't reliably process events
logger.warn("Inner bean '" + beanName + "' implements ApplicationListener interface " +
"but is not reachable for event multicasting by its containing ApplicationContext " +
"because it does not have singleton scope. Only top-level listener beans are allowed " +
"to be of non-singleton scope.");
}
this.singletonNames.remove(beanName);
}
}
return bean;
}
如上所示,如果当前 bean 实现了 ApplicationListener 接口,就会调用 this.applicationContext.addApplicationListener 方法将当前 bean 注册到 applicationContext 的监听器集合中,后面有广播就直接找到这些监听器,调用每个监听器的 onApplicationEvent 方法;
现在把广播与监听的关键代码都看过了,可以开始实战了么?稍等,还有最后一个疑问要弄明白:自定义的消息监听器可以指定消息类型,所有的广播消息中,这个监听器只会收到自己指定的消息类型的广播,spring 是如何做到这一点的?
如何做到只接收指定类型的消息
此问题的答案就在 spring 源码中,但是看源码之前先自己猜测一下,自定义监听器只接收指定类型的消息,以下两种方案都可以实现:
注册监听器的时候,将监听器和消息类型绑定;
广播的时候,按照这条消息的类型去找指定了该类型的监听器,但不可能每条广播都去所有监听器里面找一遍,应该是说广播的时候会触发一次监听器和消息的类型绑定;
带着上述猜测去 spring 源码中寻找答案吧,先看注册监听器的代码,按照之前的分析,注册监听发生在后置处理器 ApplicationListenerDetector 中,看看 this.applicationContext.addApplicationListener 这一行代码的内部逻辑:
@Override
public void addApplicationListener(ApplicationListener<?> listener) {
Assert.notNull(listener, "ApplicationListener must not be null");
if (this.applicationEventMulticaster != null) {
this.applicationEventMulticaster.addApplicationListener(listener);
}
else {
this.applicationListeners.add(listener);
}
}
最终跟踪到 AbstractApplicationEventMulticaster 类的 addApplicationListener 方法:
@Override
public void addApplicationListener(ApplicationListener<?> listener) {
synchronized (this.retrievalMutex) {
// Explicitly remove target for a proxy, if registered already,
// in order to avoid double invocations of the same listener.
Object singletonTarget = AopProxyUtils.getSingletonTarget(listener);
if (singletonTarget instanceof ApplicationListener) {
//如果因为AOP导致创建了监听类的代理,那么就要在注册列表中清除代理类
this.defaultRetriever.applicationListeners.remove(singletonTarget);
}
//把监听器加入集合defaultRetriever.applicationListeners中,这是个LinkedHashSet实例
this.defaultRetriever.applicationListeners.add(listener);
this.retrieverCache.clear();
}
}
从以上代码我们发现了两个关键点:
AOP 是通过代理技术实现的,此时可能通过 CGLIB 生成了监听类的代理类,此类的实例如果也被注册到监听器集合中,那么广播的时候,按照消息类型就会取出两个监听器实例来了,到时候的效果就是一个消息被两个实例消费了,因此这里要先清理掉代理类;
所谓的注册监听器,其实就是把 ApplicationListener 的实现类放入一个 LinkedHashSet 的集合,此处没有任何与消息类型相关的操作,因此,监听器注册的时候并没有将消息类型和监听器绑定;
监听器注册的代码看过了,没有绑定,那么只好去看广播消息的代码了,好在前面才看过广播的代码,印象还算深刻,再次来到 SimpleApplicationEventMulticaster 的 multicastEvent 方法:
@Override
public void multicastEvent(final ApplicationEvent event, @Nullable ResolvableType eventType) {
ResolvableType type = (eventType != null ? eventType : resolveDefaultEventType(event));
//根据消息类型取出对应的所有监听器
for (final ApplicationListener<?> listener : getApplicationListeners(event, type)) {
Executor executor = getTaskExecutor();
if (executor != null) {
executor.execute(() -> invokeListener(listener, event));
}
else {
invokeListener(listener, event);
}
}
}
如上述代码所示,解开我们疑问的关键点就在**getApplicationListeners(event, type)**这段代码了,也就是说在发送消息的时候根据类型去找所有对应的监听器,展开这个方法一探究竟:
protected Collection<ApplicationListener<?>> getApplicationListeners(
ApplicationEvent event, ResolvableType eventType) {
Object source = event.getSource();
Class<?> sourceType = (source != null ? source.getClass() : null);
//缓存的key有两个维度:消息来源+消息类型(关于消息来源可见ApplicationEvent构造方法的入参)
ListenerCacheKey cacheKey = new ListenerCacheKey(eventType, sourceType);
// retrieverCache是ConcurrentHashMap对象,所以是线程安全的,
// ListenerRetriever中有个监听器的集合,并有些简单的逻辑封装,调用它的getApplicationListeners方法返回的监听类集合是排好序的(order注解排序)
ListenerRetriever retriever = this.retrieverCache.get(cacheKey);
if (retriever != null) {
//如果retrieverCache中找到对应的监听器集合,就立即返回了
return retriever.getApplicationListeners();
}
if (this.beanClassLoader == null ||
(ClassUtils.isCacheSafe(event.getClass(), this.beanClassLoader) &&
(sourceType == null || ClassUtils.isCacheSafe(sourceType, this.beanClassLoader)))) {
//如果retrieverCache中没有数据,就在此查出数据并放入缓存,
//先加锁
synchronized (this.retrievalMutex) {
//双重判断的第二重,避免自己在BLOCK的时候其他线程已经将数据放入缓存了
retriever = this.retrieverCache.get(cacheKey);
if (retriever != null) {
return retriever.getApplicationListeners();
}
//新建一个ListenerRetriever对象
retriever = new ListenerRetriever(true);
//retrieveApplicationListeners方法复制找出某个消息类型加来源类型对应的所有监听器
Collection<ApplicationListener<?>> listeners =
retrieveApplicationListeners(eventType, sourceType, retriever);
//存入retrieverCache
this.retrieverCache.put(cacheKey, retriever);
//返回结果
return listeners;
}
}
else {
// No ListenerRetriever caching -> no synchronization necessary
return retrieveApplicationListeners(eventType, sourceType, null);
}
}
上述代码解开了所有疑惑:**在广播消息的时刻,如果某个类型的消息在缓存中找不到对应的监听器集合,就调用 retrieveApplicationListeners 方法去找出符合条件的所有监听器,然后放入这个集合;**这和之前的猜测匹配度挺高的...
retrieveApplicationListeners 方法在面对各种监听器的时候处理逻辑过于复杂,就不在这里展开了;
至此,我们对广播和监听的原理已经有了较全面的认识,可以动手实战了;
开发一个自定义广播
现在基于 maven 创建一个 SpringBoot 的 web 工程 customizeapplicationevent,在工程中自定义一个发送广播的 bean,在收到一个 web 请求时,利用该 bean 发出广播;
创建工程 customizeapplicationevent,pom.xml 如下:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.bolingcavalry</groupId>
<artifactId>customizeapplicationevent</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>customizeapplicationevent</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.4.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
自定义一个消息类型 CustomizeEvent:
package com.bolingcavalry.customizeapplicationevent.event;
import org.springframework.context.ApplicationContext;
import org.springframework.context.event.ApplicationContextEvent;
/**
* @Description : 自定义的消息类型
* @Author : zq2599@gmail.com
* @Date : 2018-08-16 06:09
*/
public class CustomizeEvent extends ApplicationContextEvent {
public CustomizeEvent(ApplicationContext source) {
super(source);
}
}
自定义广播发送者 CustomizePublisher,除了用到 ApplicationEventPublisher,还要用到 ApplicationContext(构造 CustomizeEvent 对象的时候要用),所以要实现两个 Aware 接口:
package com.bolingcavalry.customizeapplicationevent.publish;
import com.bolingcavalry.customizeapplicationevent.event.CustomizeEvent;
import com.bolingcavalry.customizeapplicationevent.util.Utils;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.context.ApplicationEventPublisherAware;
import org.springframework.stereotype.Service;
/**
* @Description : 自定义的广播发送器
* @Author : zq2599@gmail.com
* @Date : 2018-08-16 06:09
*/
@Service
public class CustomizePublisher implements ApplicationEventPublisherAware, ApplicationContextAware {
private ApplicationEventPublisher applicationEventPublisher;
private ApplicationContext applicationContext;
@Override
public void setApplicationEventPublisher(ApplicationEventPublisher applicationEventPublisher) {
this.applicationEventPublisher = applicationEventPublisher;
Utils.printTrack("applicationEventPublisher is set : " + applicationEventPublisher);
}
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
this.applicationContext = applicationContext;
}
/**
* 发送一条广播
*/
public void publishEvent(){
applicationEventPublisher.publishEvent(new CustomizeEvent(applicationContext));
}
}
做一个 controller,这样就能通过 web 请求来触发一次广播了:
package com.bolingcavalry.customizeapplicationevent.controller;
import com.bolingcavalry.customizeapplicationevent.publish.CustomizePublisher;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.Date;
/**
* @Description : 收到web请求后发送一条广播
* @Author : zq2599@gmail.com
* @Date : 2018-08-16 06:45
*/
@RestController
public class CustomizePublishEventController {
@Autowired
private CustomizePublisher customizePublisher;
@RequestMapping("/publish")
public String publish(){
customizePublisher.publishEvent();
return "publish finish, "
+ new Date();
}
}
自定义广播的代码已经完成了,不过现在即使把消息发出去了也不能验证是否发送成功,先不要运行工程,接下来把自定义消息监听也做了吧;
开发两个自定义监听器
我们要做两个自定义监听器;
第一个监听类在泛型中定义的类型是 ApplicationEvent,即接收所有广播消息;
package com.bolingcavalry.customizeapplicationevent.listener;
import com.bolingcavalry.customizeapplicationevent.util.Utils;
import org.springframework.context.ApplicationEvent;
import org.springframework.context.ApplicationListener;
import org.springframework.stereotype.Service;
/**
* @Description : 自定义的系统广播监听器,接收所有类型的消息
* @Author : zq2599@gmail.com
* @Date : 2018-08-15 14:53
*/
@Service
public class AllEventListener implements ApplicationListener<ApplicationEvent>{
@Override
public void onApplicationEvent(ApplicationEvent event) {
//为了便于了解调用栈,在日志中打印当前堆栈
Utils.printTrack("onApplicationEvent : " + event);
}
}
第二个监听类在泛型中定义的类型是 CustomizeEvent,即只接收 CustomizeEvent 类型的消息;
package com.bolingcavalry.customizeapplicationevent.listener;
import com.bolingcavalry.customizeapplicationevent.event.CustomizeEvent;
import com.bolingcavalry.customizeapplicationevent.util.Utils;
import org.springframework.context.ApplicationEvent;
import org.springframework.context.ApplicationListener;
import org.springframework.stereotype.Service;
/**
* @Description : 自定义的系统广播监听器,只接受CustomizeEvent类型的消息
* @Author : zq2599@gmail.com
* @Date : 2018-08-15 14:53
*/
@Service
public class CustomizeEventListener implements ApplicationListener<CustomizeEvent>{
@Override
public void onApplicationEvent(CustomizeEvent event) {
//为了便于了解调用栈,在日志中打印当前堆栈
Utils.printTrack("onApplicationEvent : " + event);
}
}
把工程运行起来,先见到 CustomizePublisher 被注入 ApplicationEventPublisher 对象的日志,然后看到 AllEventListener 收到广播后打印的日志,如下所示,可通过日志中的堆栈信息印证我们之前看过的广播监听相关源码:
2018-08-16 18:35:15.656 INFO 7864 --- [ main] c.b.c.util.Utils : applicationEventPublisher is set : org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@37e547da: startup date [Thu Aug 16 18:35:14 CST 2018]; root of context hierarchy
************************************************************
java.lang.Thread.getStackTrace() 1,556 <-
com.bolingcavalry.customizeapplicationevent.util.Utils.printTrack() 25 <-
com.bolingcavalry.customizeapplicationevent.publish.CustomizePublisher.setApplicationEventPublisher() 27 <-
org.springframework.context.support.ApplicationContextAwareProcessor.invokeAwareInterfaces() 114 <-
org.springframework.context.support.ApplicationContextAwareProcessor.postProcessBeforeInitialization() 96 <-
org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.applyBeanPostProcessorsBeforeInitialization() 416 <-
org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.initializeBean() 1,691 <-
org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.doCreateBean() 573 <-
org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.createBean() 495 <-
org.springframework.beans.factory.support.AbstractBeanFactory.lambda$doGetBean$0() 317 <-
org.springframework.beans.factory.support.DefaultSingletonBeanRegistry.getSingleton() 222 <-
org.springframework.beans.factory.support.AbstractBeanFactory.doGetBean() 315 <-
org.springframework.beans.factory.support.AbstractBeanFactory.getBean() 199 <-
org.springframework.beans.factory.config.DependencyDescriptor.resolveCandidate() 251 <-
org.springframework.beans.factory.support.DefaultListableBeanFactory.doResolveDependency() 1,135 <-
org.springframework.beans.factory.support.DefaultListableBeanFactory.resolveDependency() 1,062 <-
org.springframework.beans.factory.annotation.AutowiredAnnotationBeanPostProcessor$AutowiredFieldElement.inject() 583 <-
org.springframework.beans.factory.annotation.InjectionMetadata.inject() 91 <-
org.springframework.beans.factory.annotation.AutowiredAnnotationBeanPostProcessor.postProcessPropertyValues() 372 <-
org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.populateBean() 1,341 <-
org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.doCreateBean() 572 <-
org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.createBean() 495 <-
org.springframework.beans.factory.support.AbstractBeanFactory.lambda$doGetBean$0() 317 <-
org.springframework.beans.factory.support.DefaultSingletonBeanRegistry.getSingleton() 222 <-
org.springframework.beans.factory.support.AbstractBeanFactory.doGetBean() 315 <-
org.springframework.beans.factory.support.AbstractBeanFactory.getBean() 199 <-
org.springframework.beans.factory.support.DefaultListableBeanFactory.preInstantiateSingletons() 759 <-
org.springframework.context.support.AbstractApplicationContext.finishBeanFactoryInitialization() 869 <-
org.springframework.context.support.AbstractApplicationContext.refresh() 550 <-
org.springframework.boot.web.servlet.context.ServletWebServerApplicationContext.refresh() 140 <-
org.springframework.boot.SpringApplication.refresh() 762 <-
org.springframework.boot.SpringApplication.refreshContext() 398 <-
org.springframework.boot.SpringApplication.run() 330 <-
org.springframework.boot.SpringApplication.run() 1,258 <-
org.springframework.boot.SpringApplication.run() 1,246 <-
com.bolingcavalry.customizeapplicationevent.CustomizeapplicationeventApplication.main() 10
************************************************************
2018-08-16 18:35:15.732 INFO 7864 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/**/favicon.ico] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-08-16 18:35:15.875 INFO 7864 --- [ main] s.w.s.m.m.a.RequestMappingHandlerAdapter : Looking for @ControllerAdvice: org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@37e547da: startup date [Thu Aug 16 18:35:14 CST 2018]; root of context hierarchy
2018-08-16 18:35:15.919 INFO 7864 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/publish]}" onto public java.lang.String com.bolingcavalry.customizeapplicationevent.controller.CustomizePublishEventController.publish()
2018-08-16 18:35:15.924 INFO 7864 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/error]}" onto public org.springframework.http.ResponseEntity<java.util.Map<java.lang.String, java.lang.Object>> org.springframework.boot.autoconfigure.web.servlet.error.BasicErrorController.error(javax.servlet.http.HttpServletRequest)
2018-08-16 18:35:15.925 INFO 7864 --- [ main] s.w.s.m.m.a.RequestMappingHandlerMapping : Mapped "{[/error],produces=[text/html]}" onto public org.springframework.web.servlet.ModelAndView org.springframework.boot.autoconfigure.web.servlet.error.BasicErrorController.errorHtml(javax.servlet.http.HttpServletRequest,javax.servlet.http.HttpServletResponse)
2018-08-16 18:35:15.945 INFO 7864 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/webjars/**] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-08-16 18:35:15.946 INFO 7864 --- [ main] o.s.w.s.handler.SimpleUrlHandlerMapping : Mapped URL path [/**] onto handler of type [class org.springframework.web.servlet.resource.ResourceHttpRequestHandler]
2018-08-16 18:35:16.059 INFO 7864 --- [ main] o.s.j.e.a.AnnotationMBeanExporter : Registering beans for JMX exposure on startup
2018-08-16 18:35:16.069 INFO 7864 --- [ main] c.b.c.util.Utils : onApplicationEvent : org.springframework.context.event.ContextRefreshedEvent[source=org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@37e547da: startup date [Thu Aug 16 18:35:14 CST 2018]; root of context hierarchy]
************************************************************
java.lang.Thread.getStackTrace() 1,556 <-
com.bolingcavalry.customizeapplicationevent.util.Utils.printTrack() 25 <-
com.bolingcavalry.customizeapplicationevent.listener.AllEventListener.onApplicationEvent() 18 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.doInvokeListener() 172 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.invokeListener() 165 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.multicastEvent() 139 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 400 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 354 <-
org.springframework.context.support.AbstractApplicationContext.finishRefresh() 888 <-
org.springframework.boot.web.servlet.context.ServletWebServerApplicationContext.finishRefresh() 161 <-
org.springframework.context.support.AbstractApplicationContext.refresh() 553 <-
org.springframework.boot.web.servlet.context.ServletWebServerApplicationContext.refresh() 140 <-
org.springframework.boot.SpringApplication.refresh() 762 <-
org.springframework.boot.SpringApplication.refreshContext() 398 <-
org.springframework.boot.SpringApplication.run() 330 <-
org.springframework.boot.SpringApplication.run() 1,258 <-
org.springframework.boot.SpringApplication.run() 1,246 <-
com.bolingcavalry.customizeapplicationevent.CustomizeapplicationeventApplication.main() 10
************************************************************
2018-08-16 18:35:16.094 INFO 7864 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path ''
2018-08-16 18:35:16.096 INFO 7864 --- [ main] c.b.c.util.Utils : onApplicationEvent : org.springframework.boot.web.servlet.context.ServletWebServerInitializedEvent[source=org.springframework.boot.web.embedded.tomcat.TomcatWebServer@1c25b8a7]
************************************************************
java.lang.Thread.getStackTrace() 1,556 <-
com.bolingcavalry.customizeapplicationevent.util.Utils.printTrack() 25 <-
com.bolingcavalry.customizeapplicationevent.listener.AllEventListener.onApplicationEvent() 18 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.doInvokeListener() 172 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.invokeListener() 165 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.multicastEvent() 139 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 400 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 354 <-
org.springframework.boot.web.servlet.context.ServletWebServerApplicationContext.finishRefresh() 164 <-
org.springframework.context.support.AbstractApplicationContext.refresh() 553 <-
org.springframework.boot.web.servlet.context.ServletWebServerApplicationContext.refresh() 140 <-
org.springframework.boot.SpringApplication.refresh() 762 <-
org.springframework.boot.SpringApplication.refreshContext() 398 <-
org.springframework.boot.SpringApplication.run() 330 <-
org.springframework.boot.SpringApplication.run() 1,258 <-
org.springframework.boot.SpringApplication.run() 1,246 <-
com.bolingcavalry.customizeapplicationevent.CustomizeapplicationeventApplication.main() 10
************************************************************
2018-08-16 18:35:16.099 INFO 7864 --- [ main] b.c.CustomizeapplicationeventApplication : Started CustomizeapplicationeventApplication in 2.098 seconds (JVM running for 3.161)
2018-08-16 18:35:16.101 INFO 7864 --- [ main] c.b.c.util.Utils : onApplicationEvent : org.springframework.boot.context.event.ApplicationStartedEvent[source=org.springframework.boot.SpringApplication@77b7ffa4]
************************************************************
java.lang.Thread.getStackTrace() 1,556 <-
com.bolingcavalry.customizeapplicationevent.util.Utils.printTrack() 25 <-
com.bolingcavalry.customizeapplicationevent.listener.AllEventListener.onApplicationEvent() 18 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.doInvokeListener() 172 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.invokeListener() 165 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.multicastEvent() 139 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 400 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 354 <-
org.springframework.boot.context.event.EventPublishingRunListener.started() 97 <-
org.springframework.boot.SpringApplicationRunListeners.started() 72 <-
org.springframework.boot.SpringApplication.run() 337 <-
org.springframework.boot.SpringApplication.run() 1,258 <-
org.springframework.boot.SpringApplication.run() 1,246 <-
com.bolingcavalry.customizeapplicationevent.CustomizeapplicationeventApplication.main() 10
************************************************************
2018-08-16 18:35:16.103 INFO 7864 --- [ main] c.b.c.util.Utils : onApplicationEvent : org.springframework.boot.context.event.ApplicationReadyEvent[source=org.springframework.boot.SpringApplication@77b7ffa4]
************************************************************
java.lang.Thread.getStackTrace() 1,556 <-
com.bolingcavalry.customizeapplicationevent.util.Utils.printTrack() 25 <-
com.bolingcavalry.customizeapplicationevent.listener.AllEventListener.onApplicationEvent() 18 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.doInvokeListener() 172 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.invokeListener() 165 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.multicastEvent() 139 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 400 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 354 <-
org.springframework.boot.context.event.EventPublishingRunListener.running() 103 <-
org.springframework.boot.SpringApplicationRunListeners.running() 78 <-
org.springframework.boot.SpringApplication.run() 346 <-
org.springframework.boot.SpringApplication.run() 1,258 <-
org.springframework.boot.SpringApplication.run() 1,246 <-
com.bolingcavalry.customizeapplicationevent.CustomizeapplicationeventApplication.main() 10
************************************************************
在浏览器输入地址:http://localhost:8080/publish,触发一次 CustomizeEvent 类型的消息广播,日志显示 CustomizeEventListener 和 AllEventListener 都收到了消息,如下:
2018-08-16 18:44:18.879 INFO 7864 --- [nio-8080-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring FrameworkServlet 'dispatcherServlet'
2018-08-16 18:44:18.880 INFO 7864 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : FrameworkServlet 'dispatcherServlet': initialization started
2018-08-16 18:44:18.912 INFO 7864 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : FrameworkServlet 'dispatcherServlet': initialization completed in 32 ms
2018-08-16 18:44:18.958 INFO 7864 --- [nio-8080-exec-1] c.b.c.util.Utils : onApplicationEvent : com.bolingcavalry.customizeapplicationevent.event.CustomizeEvent[source=org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@37e547da: startup date [Thu Aug 16 18:35:14 CST 2018]; root of context hierarchy]
************************************************************
java.lang.Thread.getStackTrace() 1,556 <-
com.bolingcavalry.customizeapplicationevent.util.Utils.printTrack() 25 <-
com.bolingcavalry.customizeapplicationevent.listener.AllEventListener.onApplicationEvent() 18 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.doInvokeListener() 172 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.invokeListener() 165 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.multicastEvent() 139 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 400 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 354 <-
com.bolingcavalry.customizeapplicationevent.publish.CustomizePublisher.publishEvent() 39 <-
com.bolingcavalry.customizeapplicationevent.controller.CustomizePublishEventController.publish() 24 <-
sun.reflect.NativeMethodAccessorImpl.invoke0() -2 <-
sun.reflect.NativeMethodAccessorImpl.invoke() 62 <-
sun.reflect.DelegatingMethodAccessorImpl.invoke() 43 <-
java.lang.reflect.Method.invoke() 498 <-
org.springframework.web.method.support.InvocableHandlerMethod.doInvoke() 209 <-
org.springframework.web.method.support.InvocableHandlerMethod.invokeForRequest() 136 <-
org.springframework.web.servlet.mvc.method.annotation.ServletInvocableHandlerMethod.invokeAndHandle() 102 <-
org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter.invokeHandlerMethod() 877 <-
org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter.handleInternal() 783 <-
org.springframework.web.servlet.mvc.method.AbstractHandlerMethodAdapter.handle() 87 <-
org.springframework.web.servlet.DispatcherServlet.doDispatch() 991 <-
org.springframework.web.servlet.DispatcherServlet.doService() 925 <-
org.springframework.web.servlet.FrameworkServlet.processRequest() 974 <-
org.springframework.web.servlet.FrameworkServlet.doGet() 866 <-
javax.servlet.http.HttpServlet.service() 635 <-
org.springframework.web.servlet.FrameworkServlet.service() 851 <-
javax.servlet.http.HttpServlet.service() 742 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 231 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.apache.tomcat.websocket.server.WsFilter.doFilter() 52 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.RequestContextFilter.doFilterInternal() 99 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.HttpPutFormContentFilter.doFilterInternal() 109 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.HiddenHttpMethodFilter.doFilterInternal() 93 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.CharacterEncodingFilter.doFilterInternal() 200 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.apache.catalina.core.StandardWrapperValve.invoke() 198 <-
org.apache.catalina.core.StandardContextValve.invoke() 96 <-
org.apache.catalina.authenticator.AuthenticatorBase.invoke() 493 <-
org.apache.catalina.core.StandardHostValve.invoke() 140 <-
org.apache.catalina.valves.ErrorReportValve.invoke() 81 <-
org.apache.catalina.core.StandardEngineValve.invoke() 87 <-
org.apache.catalina.connector.CoyoteAdapter.service() 342 <-
org.apache.coyote.http11.Http11Processor.service() 800 <-
org.apache.coyote.AbstractProcessorLight.process() 66 <-
org.apache.coyote.AbstractProtocol$ConnectionHandler.process() 800 <-
org.apache.tomcat.util.net.NioEndpoint$SocketProcessor.doRun() 1,471 <-
org.apache.tomcat.util.net.SocketProcessorBase.run() 49 <-
java.util.concurrent.ThreadPoolExecutor.runWorker() 1,142 <-
java.util.concurrent.ThreadPoolExecutor$Worker.run() 617 <-
org.apache.tomcat.util.threads.TaskThread$WrappingRunnable.run() 61 <-
java.lang.Thread.run() 745
************************************************************
2018-08-16 18:44:18.964 INFO 7864 --- [nio-8080-exec-1] c.b.c.util.Utils : onApplicationEvent : com.bolingcavalry.customizeapplicationevent.event.CustomizeEvent[source=org.springframework.boot.web.servlet.context.AnnotationConfigServletWebServerApplicationContext@37e547da: startup date [Thu Aug 16 18:35:14 CST 2018]; root of context hierarchy]
************************************************************
java.lang.Thread.getStackTrace() 1,556 <-
com.bolingcavalry.customizeapplicationevent.util.Utils.printTrack() 25 <-
com.bolingcavalry.customizeapplicationevent.listener.CustomizeEventListener.onApplicationEvent() 19 <-
com.bolingcavalry.customizeapplicationevent.listener.CustomizeEventListener.onApplicationEvent() 14 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.doInvokeListener() 172 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.invokeListener() 165 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.multicastEvent() 139 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 400 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 354 <-
com.bolingcavalry.customizeapplicationevent.publish.CustomizePublisher.publishEvent() 39 <-
com.bolingcavalry.customizeapplicationevent.controller.CustomizePublishEventController.publish() 24 <-
sun.reflect.NativeMethodAccessorImpl.invoke0() -2 <-
sun.reflect.NativeMethodAccessorImpl.invoke() 62 <-
sun.reflect.DelegatingMethodAccessorImpl.invoke() 43 <-
java.lang.reflect.Method.invoke() 498 <-
org.springframework.web.method.support.InvocableHandlerMethod.doInvoke() 209 <-
org.springframework.web.method.support.InvocableHandlerMethod.invokeForRequest() 136 <-
org.springframework.web.servlet.mvc.method.annotation.ServletInvocableHandlerMethod.invokeAndHandle() 102 <-
org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter.invokeHandlerMethod() 877 <-
org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter.handleInternal() 783 <-
org.springframework.web.servlet.mvc.method.AbstractHandlerMethodAdapter.handle() 87 <-
org.springframework.web.servlet.DispatcherServlet.doDispatch() 991 <-
org.springframework.web.servlet.DispatcherServlet.doService() 925 <-
org.springframework.web.servlet.FrameworkServlet.processRequest() 974 <-
org.springframework.web.servlet.FrameworkServlet.doGet() 866 <-
javax.servlet.http.HttpServlet.service() 635 <-
org.springframework.web.servlet.FrameworkServlet.service() 851 <-
javax.servlet.http.HttpServlet.service() 742 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 231 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.apache.tomcat.websocket.server.WsFilter.doFilter() 52 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.RequestContextFilter.doFilterInternal() 99 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.HttpPutFormContentFilter.doFilterInternal() 109 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.HiddenHttpMethodFilter.doFilterInternal() 93 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.CharacterEncodingFilter.doFilterInternal() 200 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.apache.catalina.core.StandardWrapperValve.invoke() 198 <-
org.apache.catalina.core.StandardContextValve.invoke() 96 <-
org.apache.catalina.authenticator.AuthenticatorBase.invoke() 493 <-
org.apache.catalina.core.StandardHostValve.invoke() 140 <-
org.apache.catalina.valves.ErrorReportValve.invoke() 81 <-
org.apache.catalina.core.StandardEngineValve.invoke() 87 <-
org.apache.catalina.connector.CoyoteAdapter.service() 342 <-
org.apache.coyote.http11.Http11Processor.service() 800 <-
org.apache.coyote.AbstractProcessorLight.process() 66 <-
org.apache.coyote.AbstractProtocol$ConnectionHandler.process() 800 <-
org.apache.tomcat.util.net.NioEndpoint$SocketProcessor.doRun() 1,471 <-
org.apache.tomcat.util.net.SocketProcessorBase.run() 49 <-
java.util.concurrent.ThreadPoolExecutor.runWorker() 1,142 <-
java.util.concurrent.ThreadPoolExecutor$Worker.run() 617 <-
org.apache.tomcat.util.threads.TaskThread$WrappingRunnable.run() 61 <-
java.lang.Thread.run() 745
************************************************************
2018-08-16 18:44:18.995 INFO 7864 --- [nio-8080-exec-1] c.b.c.util.Utils : onApplicationEvent : ServletRequestHandledEvent: url=[/publish]; client=[0:0:0:0:0:0:0:1]; method=[GET]; servlet=[dispatcherServlet]; session=[null]; user=[null]; time=[66ms]; status=[OK]
************************************************************
java.lang.Thread.getStackTrace() 1,556 <-
com.bolingcavalry.customizeapplicationevent.util.Utils.printTrack() 25 <-
com.bolingcavalry.customizeapplicationevent.listener.AllEventListener.onApplicationEvent() 18 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.doInvokeListener() 172 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.invokeListener() 165 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.multicastEvent() 139 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 400 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 354 <-
org.springframework.web.servlet.FrameworkServlet.publishRequestHandledEvent() 1,074 <-
org.springframework.web.servlet.FrameworkServlet.processRequest() 1,005 <-
org.springframework.web.servlet.FrameworkServlet.doGet() 866 <-
javax.servlet.http.HttpServlet.service() 635 <-
org.springframework.web.servlet.FrameworkServlet.service() 851 <-
javax.servlet.http.HttpServlet.service() 742 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 231 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.apache.tomcat.websocket.server.WsFilter.doFilter() 52 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.RequestContextFilter.doFilterInternal() 99 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.HttpPutFormContentFilter.doFilterInternal() 109 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.HiddenHttpMethodFilter.doFilterInternal() 93 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.CharacterEncodingFilter.doFilterInternal() 200 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.apache.catalina.core.StandardWrapperValve.invoke() 198 <-
org.apache.catalina.core.StandardContextValve.invoke() 96 <-
org.apache.catalina.authenticator.AuthenticatorBase.invoke() 493 <-
org.apache.catalina.core.StandardHostValve.invoke() 140 <-
org.apache.catalina.valves.ErrorReportValve.invoke() 81 <-
org.apache.catalina.core.StandardEngineValve.invoke() 87 <-
org.apache.catalina.connector.CoyoteAdapter.service() 342 <-
org.apache.coyote.http11.Http11Processor.service() 800 <-
org.apache.coyote.AbstractProcessorLight.process() 66 <-
org.apache.coyote.AbstractProtocol$ConnectionHandler.process() 800 <-
org.apache.tomcat.util.net.NioEndpoint$SocketProcessor.doRun() 1,471 <-
org.apache.tomcat.util.net.SocketProcessorBase.run() 49 <-
java.util.concurrent.ThreadPoolExecutor.runWorker() 1,142 <-
java.util.concurrent.ThreadPoolExecutor$Worker.run() 617 <-
org.apache.tomcat.util.threads.TaskThread$WrappingRunnable.run() 61 <-
java.lang.Thread.run() 745
************************************************************
2018-08-16 18:44:19.392 INFO 7864 --- [nio-8080-exec-2] c.b.c.util.Utils : onApplicationEvent : ServletRequestHandledEvent: url=[/favicon.ico]; client=[0:0:0:0:0:0:0:1]; method=[GET]; servlet=[dispatcherServlet]; session=[null]; user=[null]; time=[19ms]; status=[OK]
************************************************************
java.lang.Thread.getStackTrace() 1,556 <-
com.bolingcavalry.customizeapplicationevent.util.Utils.printTrack() 25 <-
com.bolingcavalry.customizeapplicationevent.listener.AllEventListener.onApplicationEvent() 18 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.doInvokeListener() 172 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.invokeListener() 165 <-
org.springframework.context.event.SimpleApplicationEventMulticaster.multicastEvent() 139 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 400 <-
org.springframework.context.support.AbstractApplicationContext.publishEvent() 354 <-
org.springframework.web.servlet.FrameworkServlet.publishRequestHandledEvent() 1,074 <-
org.springframework.web.servlet.FrameworkServlet.processRequest() 1,005 <-
org.springframework.web.servlet.FrameworkServlet.doGet() 866 <-
javax.servlet.http.HttpServlet.service() 635 <-
org.springframework.web.servlet.FrameworkServlet.service() 851 <-
javax.servlet.http.HttpServlet.service() 742 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 231 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.apache.tomcat.websocket.server.WsFilter.doFilter() 52 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.RequestContextFilter.doFilterInternal() 99 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.HttpPutFormContentFilter.doFilterInternal() 109 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.HiddenHttpMethodFilter.doFilterInternal() 93 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.springframework.web.filter.CharacterEncodingFilter.doFilterInternal() 200 <-
org.springframework.web.filter.OncePerRequestFilter.doFilter() 107 <-
org.apache.catalina.core.ApplicationFilterChain.internalDoFilter() 193 <-
org.apache.catalina.core.ApplicationFilterChain.doFilter() 166 <-
org.apache.catalina.core.StandardWrapperValve.invoke() 198 <-
org.apache.catalina.core.StandardContextValve.invoke() 96 <-
org.apache.catalina.authenticator.AuthenticatorBase.invoke() 493 <-
org.apache.catalina.core.StandardHostValve.invoke() 140 <-
org.apache.catalina.valves.ErrorReportValve.invoke() 81 <-
org.apache.catalina.core.StandardEngineValve.invoke() 87 <-
org.apache.catalina.connector.CoyoteAdapter.service() 342 <-
org.apache.coyote.http11.Http11Processor.service() 800 <-
org.apache.coyote.AbstractProcessorLight.process() 66 <-
org.apache.coyote.AbstractProtocol$ConnectionHandler.process() 800 <-
org.apache.tomcat.util.net.NioEndpoint$SocketProcessor.doRun() 1,471 <-
org.apache.tomcat.util.net.SocketProcessorBase.run() 49 <-
java.util.concurrent.ThreadPoolExecutor.runWorker() 1,142 <-
java.util.concurrent.ThreadPoolExecutor$Worker.run() 617 <-
org.apache.tomcat.util.threads.TaskThread$WrappingRunnable.run() 61 <-
java.lang.Thread.run() 745
************************************************************
实战完成,自定义的广播发送和监听都达到了预期;
实战源码下载
本章实战的源码可以在 github 下载,地址和链接信息如下表所示:
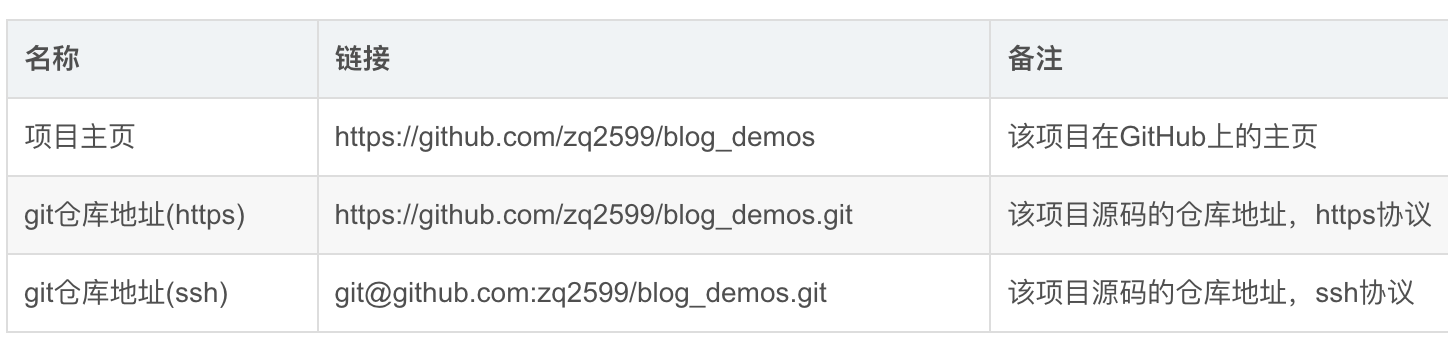
这个 git 项目中有多个文件夹,本章源码在文件夹 customizeapplicationevent 下,如下图红框所示:
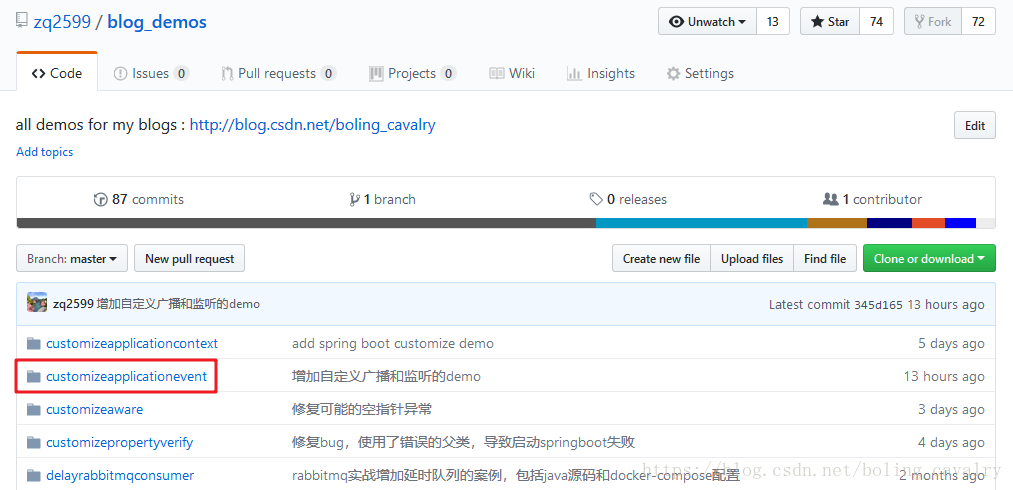
至此,spring 实战的广播与监听部分,我们通过先看源码再动手实践的方式做了比较深入的了解,希望本次实战能对你在设计进程内的消息服务时有所帮助,借助 spring 容器和扩展能力,设计出更加简洁高效的服务;
欢迎关注 InfoQ:程序员欣宸
版权声明: 本文为 InfoQ 作者【程序员欣宸】的原创文章。
原文链接:【http://xie.infoq.cn/article/773474185335bf3949951cec4】。文章转载请联系作者。
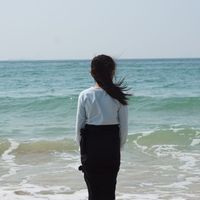
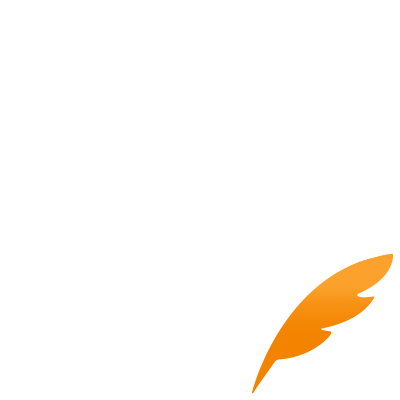
程序员欣宸
搜索"程序员欣宸",一起畅游Java宇宙 2018.04.19 加入
前腾讯、前阿里员工,从事Java后台工作,对Docker和Kubernetes充满热爱,所有文章均为作者原创,个人Github:https://github.com/zq2599/blog_demos
评论