【SpringMVC 笔记】拦截器 ,java 设计模式及实践百度云
<title>login</title>
</head>
<h1>登录页面</h1>
<hr>
<body>
<form action="${pageContext.request.contextPath}/user/login">
用户名:<input type="text" name="username"> <br>
密码:<input type="password" name="pwd"> <br>
<input type="submit" value="提交">
</form>
</body>
</html>
编写一个 Controller 处理请求
package com.yusael.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import javax.servlet.http.HttpSession;
@Controller
@RequestMapping("/user")
public class UserController {
// 跳转到登录界面
@RequestMapping("/toLogin")
public String toLogin() {
return "login";
}
// 跳转到成功页面
@RequestMapping("/toSuccess")
public String toSucess() {
return "success";
}
// 登录提交
@RequestMapping("/login")
public String login(HttpSession session, String username, String password) {
// 向 session 记录用户身份信息
System.out.println("接收前端 ===> " + username);
session.setAttribute("user", username);
return "success";
}
// 登录过期
@RequestMapping("/logout")
public String logout(HttpSession httpSession) {
// session 过期
httpSession.invalidate();
return "login";
}
}
编写一个登陆成功的页面 success.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>success</title>
</head>
<body>
<h1>登录成功页面</h1>
<hr>
${user}
<a href="${pageContext.request.contextPath}/user/logout">注销</a>
</body>
</html>
4
. 编写主页 index.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>index</title>
</head>
<body>
<h1>首页</h1>
<hr>
<%--登录--%>
<a href="${pageContext.request.contextPath}/user/toLogin">登录</a>
<a href="${pageContext.request.contextPath}/user/toSuccess">成功页面</a>
</body>
</html>
测试:
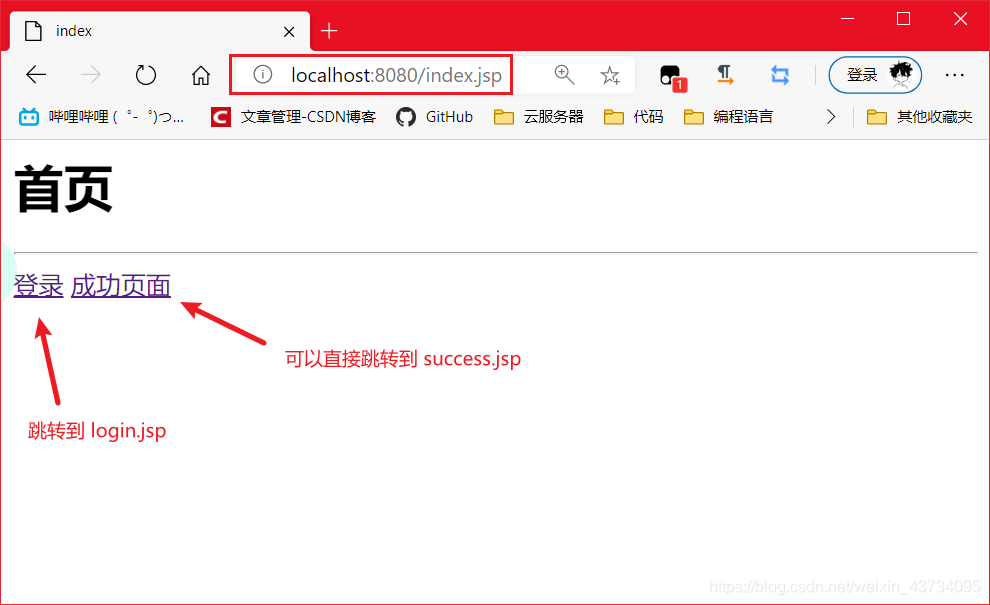
点击 登录,会请求跳转到 登陆页面 login.jsp;
点击 成功页面,会请求跳转到 成功页面 success.jsp
这里就出现问题了,只要在 地址栏发出请求,可以直接 越过登录,进入登录成功的界面,这个肯定是有问题的。那要如何解决这个问题呢? —— 通过 拦截器。
编写用户登录拦截器 LoginInterceptor.java
package com.yusael.interceptor;
import org.springframework.web.servlet.HandlerInterceptor;
import org.springframework.web.servlet.ModelAndView;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
public class LoginInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
// 如果是登录页面则放行
System.out.println(request.getRequestURI());
if (request.getRequestURI().contains("login")) {
return true;
}
HttpSession session = request.getSession();
// 如果用户已经登录也放行
if (session.getAttribute("user") != null) {
return true;
}
// 如果用户没有登录则跳转到登录界面
request.getRequestDispatcher("/WEB-INF/jsp/login.jsp").forward(request, response);
return false;
}
@Override
public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception {
}
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception {
}
}
在 Springmvc 的配置文件中注册拦截器:
<mvc:mapping path="/**"/>
<bean id="loginInterceptor" class="com.yusael.interceptor.LoginInterceptor"/>
</mvc:interceptor>
</mvc:interceptors>
测试:
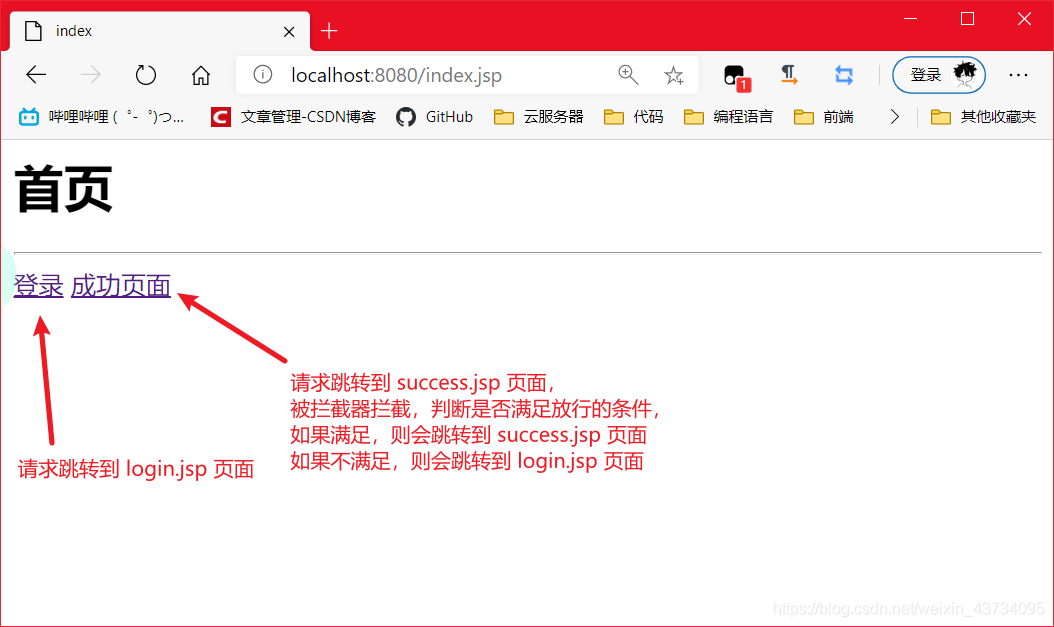
由于配置了 拦截器,直接登录 成功页面,虽然会请求跳转到 success.jsp 页面,但是由于不满足拦截器进行了判断,不满足放行的条件则会跳转到 登录界面。只有满足拦截器放行的条件才会跳转到 成功页面。
========================================================================
文件上传是项目开发中最常见的功能之一 ,SpringMVC 可以很好的支持文件上传,但是 SpringMVC 上下文中默认没有装配 MultipartResolver
,因此默认情况下其不能处理文件上传工作。如果想使用 Spring 的文件上传功能,则需要在上下文中配置 MultipartResolver
。
对前端表单的要求:为了能上传文件,必须将表单的 method
设置为 POST
,并将 enctype
设置为multipart/form-data
。只有在这样的情况下,浏览器才会把用户选择的文件以二进制数据发送给服务器;
表单中的 enctype
(编码方式)属性的说明:
application/x-www=form-urlencoded
:默认方式,只处理表单域中的 value 属性值,采用这种编码方式的表单会将表单域中的值处理成 URL 编码方式。multipart/form-data
:这种编码方式会以二进制流的方式来处理表单数据,这种编码方式会把文件域指定文件的内容也封装到请求参数中,不会对字符编码。text/plain
:除了把空格转换为 “+” 号外,其他字符都不做编码处理,这种方式适用直接通过表单发送邮件。
<form action="" enctype="multipart/form-data" method="post">
<input type="file" name="file"/>
<input type="submit">
</form>
一旦设置了 enctype
为 multipart/form-data
,浏览器即会采用二进制流的方式来处理表单数据,而对于文件上传的处理则涉及在服务器端解析原始的 HTTP 响应。
在 2003 年,Apache Software Foundation 发布了开源的 Commons FileUpload 组件,其很快成为 Servlet/JSP 程序员上传文件的最佳选择。
Servlet3.0 规范已经提供方法来处理文件上传,但这种上传需要在 Servlet 中完成;而 Spring MVC 则提供了更简单的封装;
Spring MVC 为文件上传提供了直接的支持,这种支持是用即插即用的 MultipartResolver
实现的。
Spring MVC 使用 Apache Commons FileUpload 技术实现了一个 MultipartResolver
实现类:
CommonsMultipartResolver
。因此,SpringMVC 的文件上传还需要依赖 Apache Commons FileUpload 的组件。
引入 commons-fileupload 的依赖
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.3</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
</dependency>
配置
bean:multipartResolver
注:这个 bean
的 id 必须为:multipartResolver
,否则上传文件会报 400 的错误。
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="defaultEncoding" value="utf-8"/>
<property name="maxUploadSize" value="10485760"/>
<property name="maxInMemorySize" value="40960"/>
</bean>
CommonsMultipartFile
的 常用方法:
String getOriginalFilename() 获取上传文件的原名
InputStream getInputStream() 获取文件流
void transferTo(File dest) 将上传文件保存到一个目录文件中
编写前端页面:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>file</title>
</head>
<body>
<form action="/upload" enctype="multipart/form-data" method="post">
<input type="file" name="file"/>
<input type="submit" value="upload">
</form>
</body>
</html>
Controller
package com.yusael.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.commons.CommonsMultipartFile;
import javax.servlet.http.HttpServletRequest;
import java.io.*;
@Controller
public class FileController {
// @RequestParam("file") 将 name=file 控件得到的文件封装成 CommonsMultipartFile 对象
// 批量上传 CommonsMultipartFile 则为数组即可
@RequestMapping("/upload")
public String fileUpload(@RequestParam("file")CommonsMultipartFile file, HttpServletRequest request) throws IOException {
// 获取文件名
String uploadFileName = file.getOriginalFilename();
// 如果文件名为空, 直接回到首页
if ("".equals(uploadFileName)){
return "redirect:/index.jsp";
}
System.out.println("上传文件名: " + uploadFileName);
// 上传路径保存设置
String path = request.getServletContext().getRealPath("/upload");
// 如果路径不存在, 则创建一个
File realPath = new File(path);
if (!realPath.exists()) {
realPath.mkdir();
}
System.out.println("上传文件保存地址: " + realPath);
// 文件输入流
InputStream is = file.getInputStream();
// 文件输出流
OutputStream os = new FileOutputStream(new File(realPath, uploadFileName));
// 读取写出
int len = 0;
byte[] buffer = new byte[1024];
while ((len = is.read(buffer)) != -1) {
os.write(buffer, 0, len);
os.flush();
}
os.close();
is.close();
return "redirect:/index.jsp";
}
}
测试文件上传:
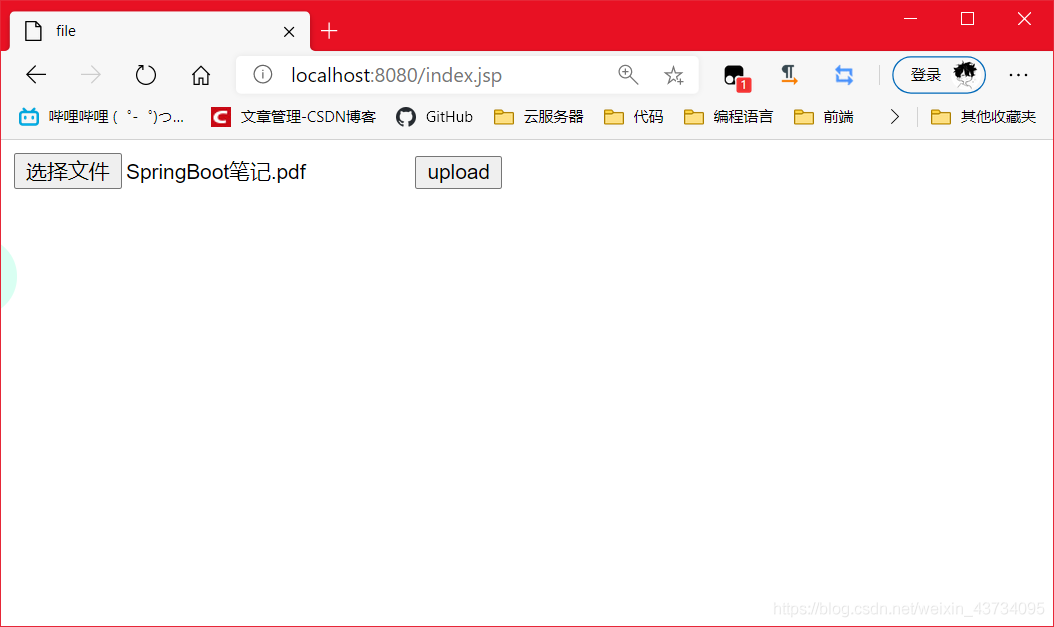
在 out 目录下可以找到我们设定的下载地址:
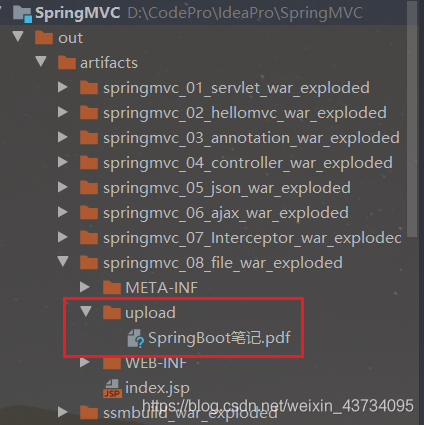
Controller:
@RequestMapping("/upload2")
public String fileUpload2(@RequestParam("file") CommonsMultipartFile file, HttpServletRequest request) throws IOException {
// 上传路径保存设置
String path = request.getServletContext().getRealPath("/upload");
File realPath = new File(path);
if (!realPath.exists()) {
realPath.mkdir();
}
System.out.println("上传文件保存地址: " + realPath);
// 通过 CommonsMultipartFile 的方法直接写文件
file.transferTo(new File(realPath + "/" + file.getOriginalFilename()));
return "redirect:/index.jsp";
}
前端页面 index.jsp:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>file</title>
</head>
<body>
<form action="/upload" enctype="multipart/form-data" method="post">
<input type="file" name="file"/>
<input type="submit" value="upload">
</form>
<hr>
<form action="/upload2" enctype="multipart/form-data" method="post">
<input type="file" name="file"/>
<input type="submit" value="upload">
</form>
</body>
</html>
进行测试:
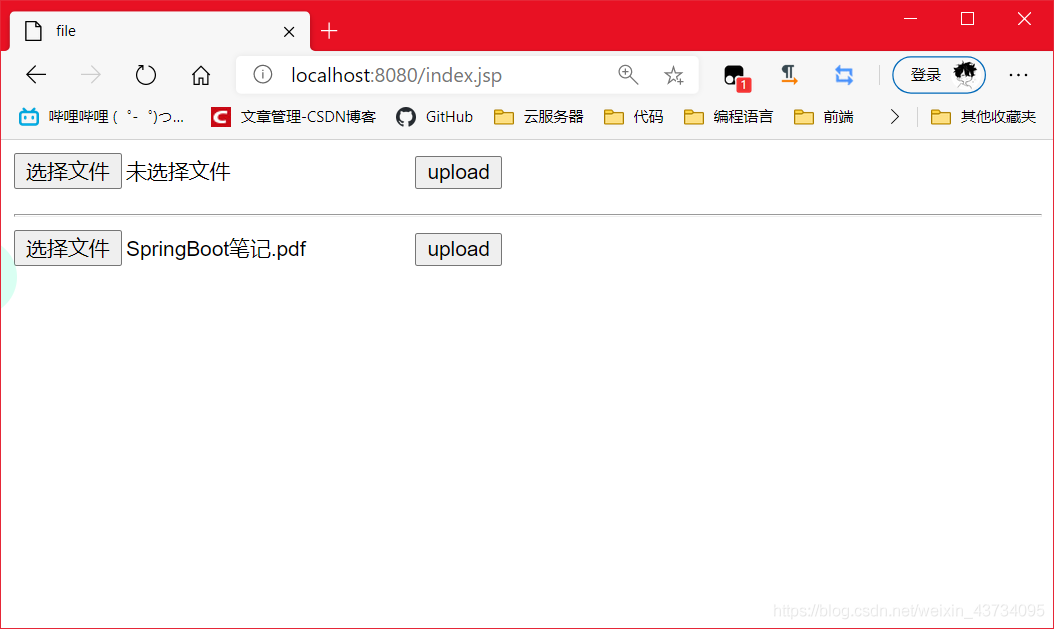
效果与上面的一模一样,同样可以在 out 目录的对应位置找到上传的文件。
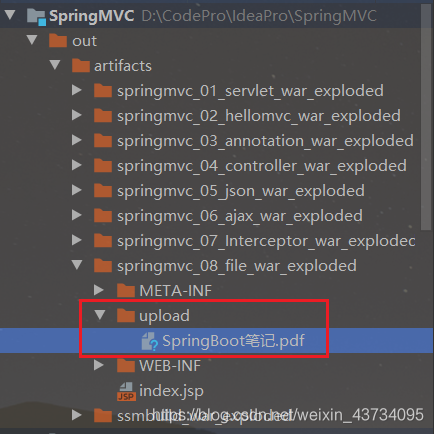
========================================================================
文件下载步骤:
设置 response 响应头
读取文件 – InputStream
写出文件 – OutputStream
执行操作
关闭流 (先开后关)
代码实现:
@RequestMapping("/download")
public String downloads(HttpServletResponse response , HttpServletRequest request) throws Exception{
// 要下载的图片地址
String path = request.getServletContext().getRealPath("/upload");
String fileName = "SpringBoot 笔记.pdf";
// 1、设置 response 响应头
response.reset(); // 设置页面不缓存, 清空 buffer
response.setCharacterEncoding("UTF-8"); // 字符编码
response.setContentType("multipart/form-data"); // 二进制传输数据
// 设置响应头
response.setHeader("Content-Disposition",
"attachment;fileName="+ URLEncoder.encode(fileName, "UTF-8"));
File file = new File(path,fileName);
评论