Java 开发之 SSM 框架整合配置知识分享
一、基本环境搭建
1、新建一个 Maven 项目,不使用模板
2、在 pom.xml 文件中设置打包格式为 war
<packaging>war</packaging>
3、在 Project Structure -> Modules 中设置 webapp 目录以及 web.xml 文件位置java培训
4、导入相关的依赖
<dependency><groupId>org.springframework</groupId><artifactId>spring-jdbc</artifactId><version>5.1.9.RELEASE</version></dependency></dependencies>
5、在 pom.xml 中设置过滤器,使静态资源可以导出
<build><resources><resource><directory>src/main/java</directory><includes><include>/*.properties</include><include>/.xml</include></includes><filtering>false</filtering></resource><resource><directory>src/main/resources</directory><includes><include>**/.properties</include><include>**/*.xml</include></includes><filtering>false</filtering></resource></resources></build>
6、在 Edit Configuration 中配置 Tomcat
7、建立基本结构和框架配置
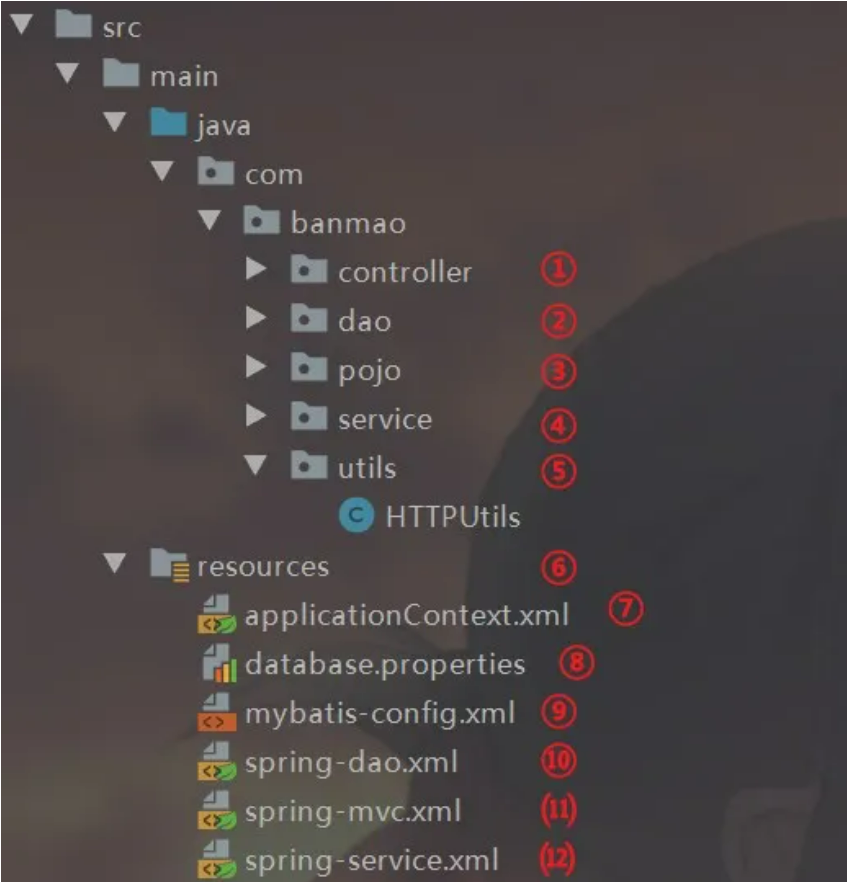
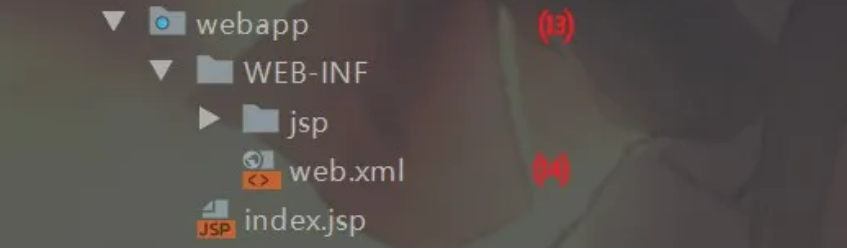
mybatis-config.xml MyBatis 配置文件<?xml version="1.0" encoding="utf-8" ?><!DOCTYPE configuration PUBLIC="-//mybatis.org//DTD Config 3.0//EN""http://mybatis.org/dtd/mybatis-3config.dtd"><configuration>
</configuration>
applicationContext.xml Spring 总配置文件<?xml version="1.0" encoding="UTF-8"?><beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
</beans>
二、整合 MyBatis
1、数据库配置文件:database.properties
jdbc.driver=com.mysql.jdbc.Driver
如果使用的是 MySQL8.0+ 增加一个时区的配置:&serverTimezone=Asia/Shanghai
jdbc.url=jdbc:mysql://localhost:3306/ssmbuild?useSSL=true&useUnicode=true&characterEncoding=utf8jdbc.username=rootjdbc.password=root
2、pojo 包下:Books 实体类
public class Books {private Integer bookID;private String bookName;private Integer bookCounts;private String detail;
}
3、dao 包下:BookMapper 接口
public interface BookMapper {// 增加一本书 int addBook(Books books);
}
4、dao 包下:BookMapper.xml
<?xml version="1.0" encoding="utf-8" ?><!DOCTYPE mapper PUBLIC="-//mybatis.org//DTD Config 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd"><mapper namespace="com.wzy.dao.BookMapper">
</mapper>
5、service 包下:BookService 接口
public interface BookService {// 增加一本书 int addBook(Books books);
}
6、service 包下:BookServiceImpl 实现类
public class BookServiceImpl implements BookService {// service 层调 dao 层:组合 DAOprivate BookMapper bookMapper;public void setBookMapper(BookMapper bookMapper) {this.bookMapper = bookMapper;}
}
三、整合 Spring
此处非常重点,建议背诵(开玩笑,直接复制就行了
1、配置 Spring 整合 MyBatis,数据源使用 c3p0 连接池
2、编写 Spring 整合 Mybatis 的相关配置文件:spring-dao.xml
<?xml version="1.0" encoding="UTF-8"?><beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xmlns:context="http://www.springframework.org/schema/context"xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd">
</beans>
3、Spring 整合 service 层
<?xml version="1.0" encoding="UTF-8"?><beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xmlns:context="http://www.springframework.org/schema/context"xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd">
</beans>
Spring 就是个大杂烩!!!!
四、整合 Spring MVC
1、web.xml
<?xml version="1.0" encoding="UTF-8"?><web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"version="4.0">
</web-app>
2、spring-mvc.xml
<?xml version="1.0" encoding="UTF-8"?><beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xmlns:mvc="http://www.springframework.org/schema/mvc"xmlns:context="http://www.springframework.org/schema/context"xsi:schemaLocation="http://www.springframework.org/schema/beanshttp://www.springframework.org/schema/beans/spring-beans.xsdhttp://www.springframework.org/schema/mvchttps://www.springframework.org/schema/mvc/spring-mvc.xsdhttp://www.springframework.org/schema/contexthttps://www.springframework.org/schema/context/spring-context.xsd">
</beans>
3、Spring 配置整合文件:applicationContext.xml
import 导入即可
<?xml version="1.0" encoding="UTF-8"?><beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
</beans>
五、整合 Controller 层和视图层
以下内容为前后端不分离,返回的数据直接显示在 JSP 页面上
1、BookController 类编写,方法一:查询所有书籍
@Controller@RequestMapping("/book")public class BookController {
@Autowired@Qualifier("BookServiceImpl")private BookService bookService;
@RequestMapping("/allBooks")public String list(Model model) {List<Books> list = bookService.queryAllBooks();model.addAttribute("list", list);return "allBooks";}}
2、编写首页 JSP:index.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %><!DOCTYPE HTML><html><head><title>首页</title></head><body>
<h3><a href="${pageContext.request.contextPath}/book/allBook">点击进入列表页</a></h3></body></html>
3、书籍列表页面:allBooks.jsp
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %><%@ page contentType="text/html;charset=UTF-8" language="java" %><html><head><title>书籍列表</title><meta name="viewport" content="width=device-width, initial-scale=1.0">
</head><body><div><div><table><thead><tr><th>书籍编号</th><th>书籍名字</th><th>书籍数量</th><th>书籍详情</th><th>操作</th></tr></thead>
</div></div>
其余方法可自己实现,不再赘述!
六、总结
实际上,Spring 的配置整合能够托管许多东西,其中涵盖了 MyBatis 的数据源配置、Filter 的乱码问题解决配置等等,这些都是机械化的配置操作,并不需要太多改变就可以复用。
因此可以将所有配置包装成模板,每次生成 SSM 框架项目时都可以直接使用。
但是!一定要学会 Spring 的理念和其与 Mybatis 以及 Service、MVC 整合的过程!
评论