Java- 进阶:Java-File-&-IO—1
//try 外面声明变量,try 里面建立对象,为了提升流对象作用域//定义 FileOutputStream 的引用 FileOutputStream fos = null;try {//创建 FileOutputStream 对象 fos = new FileOutputStream(file);//写出数据 fos.write("abcde".getBytes());} catch (IOException e) {System.out.println(e.toString() + "----");throw new RuntimeException("文件写入失败,重试");} finally {//一定要判断 fos 是否为 null,只有不为 null 时,才可以关闭资源 if (fos != null) {try {fos.close();} catch (IOException e) {throw new RuntimeException("关闭资源失败");}}}}}
##四、字节输入流 ###1. 概述 java.io.InputStream: 此抽象类是表示字节输入流的所有类的超类。读取任意文件,每次只读取 1 个字节 ###2. 核心方法 abstract int read():读取一个字节 read()方法的特点:read() 执行一次,就会自动读取下一个字节,返回值是读取到的字节, 读取到结尾返回 -1
public class IoDemo {public static void main(String[] args) throws IOException {//创建字节输入流的子类对象 FileInputStream fis = new FileInputStream("E:\a.txt"); //ABCD//读取一个字节,调用 read 方法,返回 int//使用循环方式,读取文件,循环结束的条件:read() 返回 -1int len = 0; //接收 read()的返回值 while((len = fis.read()) != -1){System.out.print((char)len); //可以转换成字符}//关闭资源 fis.close();}}
int read(byte[] b):读取一定数量的字节,并将其存储在缓冲区数组 b 中 int read(byte[] b, int off, int len) :读取最多 len 个字节,存入 byte 数组。数组的作用:缓冲的作用, 提高效率 read 返回的 int,表示读取到多少个有效的字节数
public class IoDemo {public static void main(String[] args) throws IOException {//创建字节输入流的子类对象 FileInputStream fis = new FileInputStream("E:\a.txt");//创建字节数组 byte[] b = new byte[1024];
int len = 0;while((len = fis.read(b)) != -1){System.out.print(new String(b,0,len)); //使用 String 的构造方法,转为字符串}//关闭资源 fis.close();}}
###3. FileInputStream 类 ####(1)构造方法为这个流对象 绑定数据源 FileInputStream(File file)FileInputStream(String name)参数:File 对象 和 String 对象 ####(2)输入流读取文件的步骤创建字节输入流的子类对象调用读取方法 read 读取关闭资源 ####(3)读取数据的方式:第一种方式:一次读取一个字节数据第二种方式:一次读取一个字节数组第二种方式效率更高,每次读取都会和操作系统发生一次交互,第二种方式每次可以读取多个数据,提高了操作效率 ####4、释放资源的另一种方式
//1.第一种释放资源的方式 closeQuietly//释放输入流资源 closeQuietly(fis);//释放输出流对象 closeQuietly(fos);private static void closeQuietly(Closeable close) {if(close != null) {try {close.close();} catch (IOException e) {e.printStackTrace();}}}
//2.jdk7 中引入的 try-with-resource 语句
try(放申请资源的语句){
}catch() {
}
// 一旦资源的申请放在 try-with-resource 语句的()中,那么资源会保证一定自动被释放
##五、字节流文件复制 ###1. 《一线大厂 Java 面试题解析+后端开发学习笔记+最新架构讲解视频+实战项目源码讲义》开源 读取单个字节
public class Copy {public static void main(String[] args) {long s = System.currentTimeMillis();//定义两个流的对象变量 FileInputStream fis = null;FileOutputStream fos = null;try{//建立两个流的对象,绑定数据源和数据目的 fis = new FileInputStream("D:\test.mp3"); //8,003,733 字节 fos = new FileOutputStream("d:\test.mp3");//字节输入流,读取 1 个字节,输出流写 1 个字节 int len = 0 ;while((len = fis.read())!=-1){fos.write(len);}}catch(IOException e){System.out.println(e);throw new RuntimeException("文件复制失败");}finally{try{if(fos!=null)fos.close();}catch(IOException e){throw new RuntimeException("释放资源失败");}finally{try{if(fis!=null)fis.close();}catch(IOException e){throw new RuntimeException("释放资源失败");}}}long e = System.currentTimeMillis();System.out.println(e-s);}}//耗时:61817
###2. 读取字节数组
public class Copy_1 {public static void main(String[] args) {long s = System.currentTimeMillis();FileInputStream fis = null;FileOutputStream fos = null;try{fis = new FileInputStream("D:\test.mp3");fos = new FileOutputStream("E:\test.mp3");//定义字节数组,缓冲 byte[] bytes = new byte[1024*10];//读取数组,写入数组 int len = 0 ;while((len = fis.read(bytes))!=-1){fos.write(bytes, 0, len);}}catch(IOException e){System.out.println(e);throw new RuntimeException("文件复制失败");}finally{try{if(fos!=null)fos.close();}catch(IOException e){throw new RuntimeException("释放资源失败");}finally{try{if(fis!=null)fis.close();}catch(IOException e){throw new RuntimeException("释放资源失败");}}}long e = System.currentTimeMillis();System.out.println(e-s);}}//耗时:41
##六、字符输出流 ###1. 概述 java.io.Writer 所有字符输出流的超类。写文件,写 文本文件 ###2. 方法介绍 void write(int c):写入单个字符 void write(String str) :写入字符串 void write(String str, int off, int len):写入字符串的某一部分 void write(char[] cbuf):写入字符数组 abstract void write(char[] cbuf, int off, int len) :写入字符数组的某一部分 ###3. FileWriter 类 Writer 类是抽象类,找到子类对象 FileWriter 构造方法:参数也是 File 类型对象 和 String 文件名字符输出流,写数据的时候,必须要运行一个功能,刷新功能:flush()
public class WriterDemo {public static void main(String[] args) throws IOException{FileWriter fw = new FileWriter("c:\1.txt");
//写 1 个字符 fw.write(100);fw.flush();
//写 1 个字符数组 char[] c = {'a','b','c','d','e'};fw.write(c);fw.flush();
//写字符数组一部分 fw.write(c, 2, 2);fw.flush();
//写如字符串 fw.write("hello");fw.flush();
fw.close();}}
##七、字符输入流 ###1. 概述 java.io.Reader,字符输入流读取文本文件,所有字符输入流的超类,专门读取文本文件 ###2. 方法介绍 int read(): 读取单个字符 int read(char[] cbuf):将字符读入数组 abstract int read(char[] cbuf, int off, int len) :将字符读入数组的某一部分没有读取字符串的方法 ###3. FileReader 类 Reader 类 是抽象类,找到子类对象 FileReader 构造方法:写入的数据目的。绑定数据源。参数也是 File 类型对象 和 String 文件名
public class ReaderDemo {public static void main(String[] args) throws IOException{FileReader fr Java 开源项目【ali1024.coding.net/public/P7/Java/git】 = new FileReader("c:\1.txt");//读字符/int len = 0 ;while((len = fr.read())!=-1){System.out.print((char)len);}/
//读数组 char[] ch = new char[1024];int len = 0 ;while((len = fr.read(ch))!=-1){System.out.print(new String(ch,0,len)); //转字符串}fr.close();}}
###4. flush 方法和 close 方法区别 flush()方法:用来刷新缓冲区的,刷新后可以再次写出,只有字符流才需要刷新 close()方法:用来关闭流释放资源的的,如果是带缓冲区的流对象的 close()方法,不但会关闭流,还会再关闭流之前刷新缓冲区,关闭后不能再写出
##八、字符流复制文本文件字符流复制文本文件,必须是文本文件
学习分享,共勉
这里是小编拿到的学习资源,其中包括“中高级 Java 开发面试高频考点题笔记 300 道.pdf”和“Java 核心知识体系笔记.pdf”文件分享,内容丰富,囊括了 JVM、锁、并发、Java 反射、Spring 原理、微服务、Zookeeper、数据库、数据结构等大量知识点。同时还有 Java 进阶学习的知识笔记脑图(内含大量学习笔记)!
资料整理不易,读者朋友可以转发分享下!
Java 核心知识体系笔记.pdf
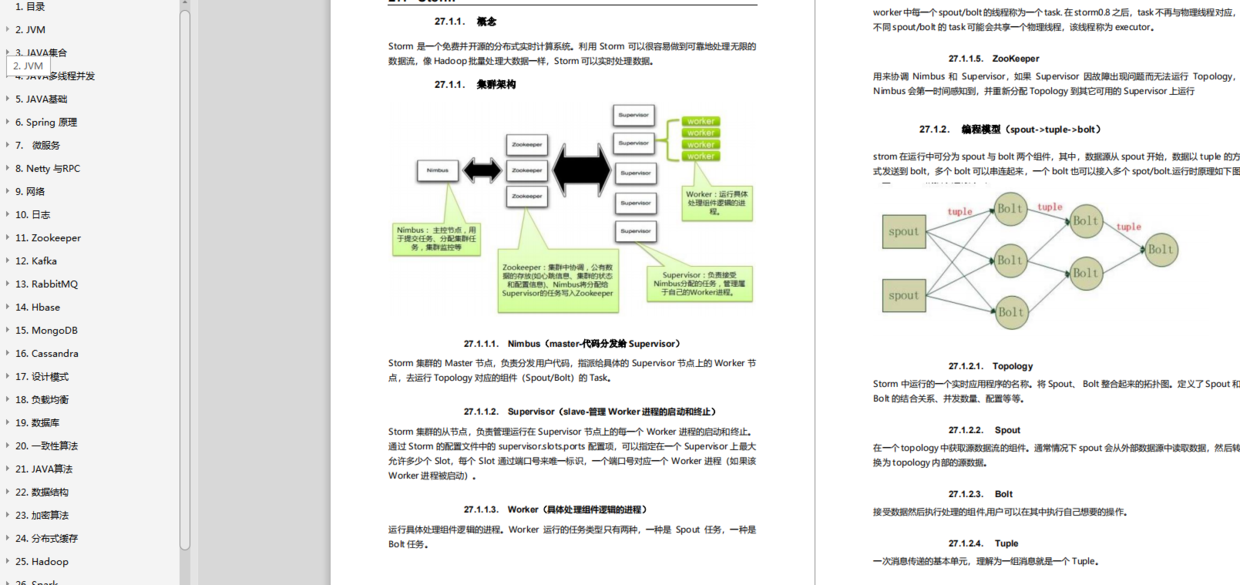
中高级 Java 开发面试高频考点题笔记 300 道.pdf
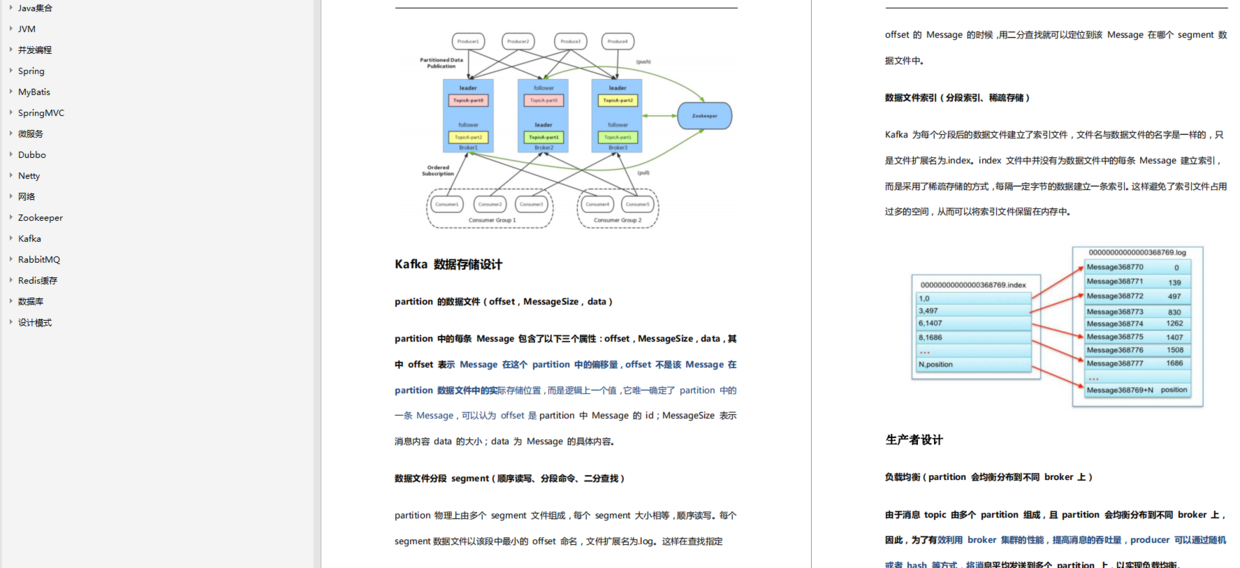
架构进阶面试专题及架构学习笔记脑图
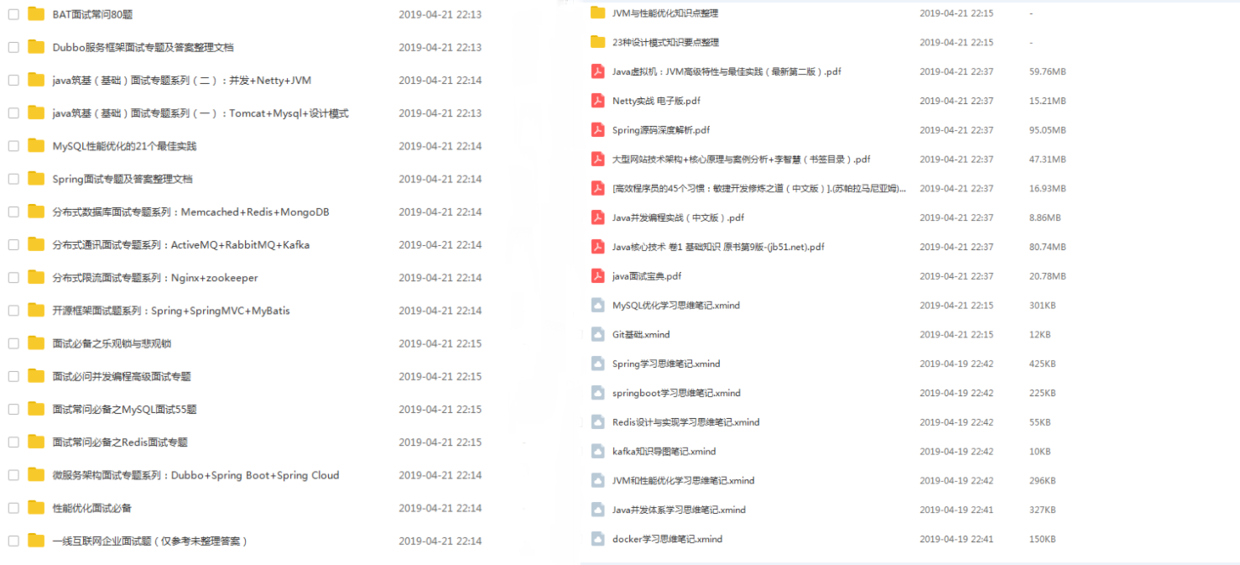
Java 架构进阶学习视频分享
评论