带你了解代理模式
@Version 1.0.0
*/
public class StaticProxyService implements PayService {
private PayService payService;
public StaticProxyService(PayService payService) {
this.payService = payService;
}
@Override
public void pay() {
before();
payService.pay();
after();
}
public void before(){
System.err.println("before 执行方法前执行");
}
public void after(){
System.err.println("after 执行方法后执行");
}
}
Main 方法测试
/**
@Auther: heng
@Date: 2021/4/25 11:14
@Description: ProxyMain
@Version 1.0.0
*/
public class ProxyMain {
public static void main(String[] args) {
PayService payService = new StaticProxyService(new PayServiceImpl());
payService.pay();
}
}
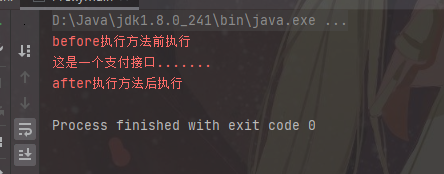
JDK 动态代理方法实现需求(被代理类必须实现接口 否则会报未实现接口错误)
需要实现 InvocationHandler
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
/**
@Auther: heng
@Date: 2021/4/25 13:11
@Description: JdkInvocationHandler jdk 动态代理
@Vers
ion 1.0.0
*/
public class PayInvocationHandler implements InvocationHandler {
public Object targerClass;
public PayInvocationHandler(Object targerClass) {
this.targerClass = targerClass;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
before();
Object result = method.invoke(targerClass, args);
after();
return result;
}
public void before(){
System.err.println("before 执行方法前执行");
}
public void after(){
System.err.println("after 执行方法后执行");
}
}
创建 JdkProxy 方法
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Proxy;
/**
@Auther: heng
@Date: 2021/4/25 13:20
@Description: JdkProxy jdk 动态代理类 执行
@Version 1.0.0
*/
public class JdkProxy {
public static <T> T newProxyInstance(T targerClass, InvocationHandler invocationHandler){
ClassLoader classLoader = targerClass.getClass().getClassLoader();
Class<?>[] interfaces = targerClass.getClass().getInterfaces();
return (T) Proxy.newProxyInstance(classLoader,interfaces,invocationHandler);
}
}
Main 测试代码
//jdk 动态代理
PayService payService = new PayServiceImpl();
PayInvocationHandler payInvocationHandler = new PayInvocationHandler(payService);
PayService proxyPayService = JdkProxy.newProxyInstance(payService, payInvocationHandler);
proxyPayService.pay();
Cglib 动态代理实现需求方法(可以代理实现接口的类和不实现接口的类)
需要引入 cglib jar
<dependency>
<groupId>cglib</groupId>
<artifactId>cglib</artifactId>
<version>3.2.9</version>
</dependency>
需要实现 MethodInterceptor
创建 CglibInvocationHandler
import net.sf.cglib.proxy.MethodInterceptor;
import net.sf.cglib.proxy.MethodProxy;
import java.lang.reflect.Method;
/**
@Auther: heng
@Date: 2021/4/25 13:37
@Description: CglibInvocationHandler cglib 动态代理
@Version 1.0.0
*/
public class CglibInvocationHandler implements MethodInterceptor {
@Override
public Object intercept(Object o, Method method, Object[] args, MethodProxy methodProxy) throws Throwable {
before();
Object result = methodProxy.invokeSuper(o, args);
after();
return result;
}
public void before(){
System.err.println("before 执行方法前执行");
}
public void after(){
System.err.println("after 执行方法后执行");
}
}
创建 CglibProxy 动态代理执行类
import net.sf.cglib.proxy.Enhancer;
import net.sf.cglib.proxy.MethodInterceptor;
/**
@Auther: heng
@Date: 2021/4/25 13:42
@Description: CglibProxy cglib 动态代理执行类
@Version 1.0.0
*/
public class CglibProxy {
public static <T> T newProxyInstance(T targerClass, MethodInterceptor methodInterceptor){
return (T) Enhancer.create(targerClass.getClass(),methodInterceptor);
}
评论