1
Django 之模板篇
发布于: 2021 年 05 月 29 日
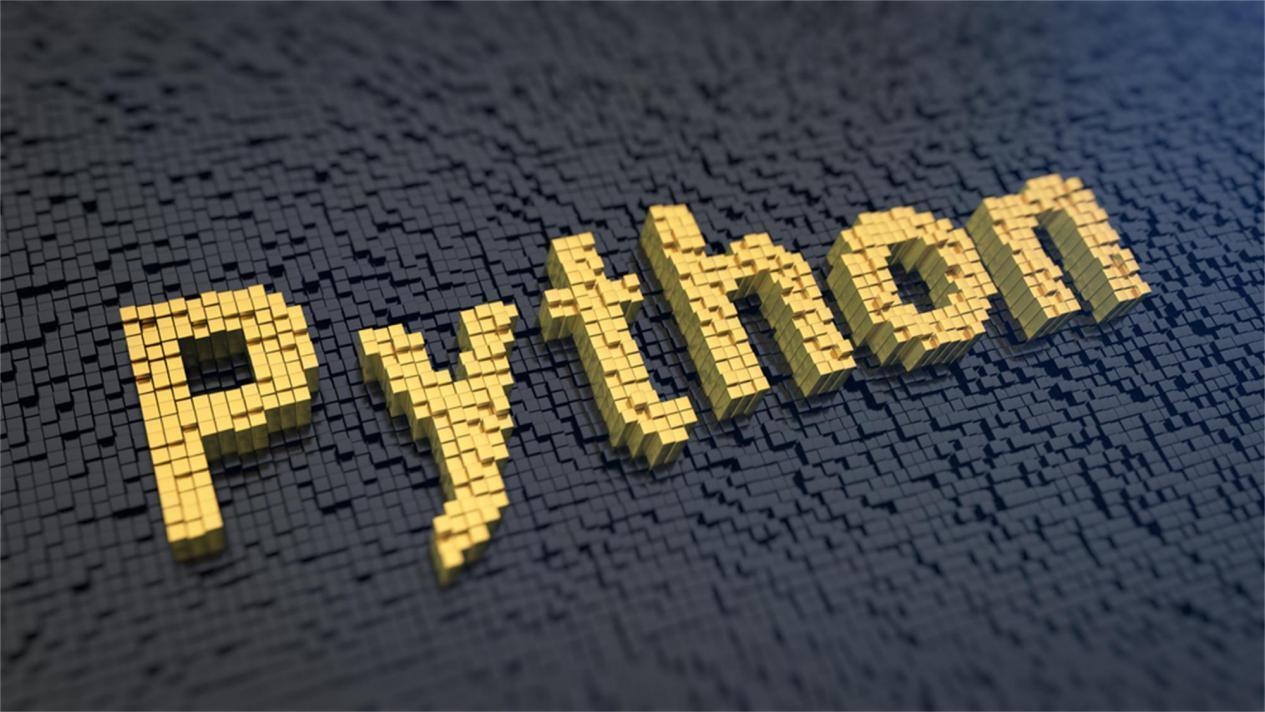
模板系统
用到的代码会放在文末
模板:一组相同或者相似的页面,在需要个性化的地方进行留白,需要的时候只是用数据填充就可以使用
步骤:
在 settings 中进行设置: TEMPLATES
在 templates 文件夹下编写模板并调用
模板-变量
变量的表示方法;{{var_name}}
在系统调用模板的时候,会用相应的数据查找相应的变量名称,如果能找到,则填充,或者叫渲染,否则,跳过
案例
two.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>案例二</title>
</head>
<body>
<h1 style="color: red">Hello {{name}}</h1>
<h1 style="color: blue">Hello {{name2}}</h1>
</body>
</html>
复制代码
模板-标签
for 标签: {% for .. in .. %}
用法:
案例
three.html
,显示班级成绩
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>案例三</title>
</head>
<body>
{% for s in score %}
<h1 style="color: deeppink">{{s}}</h1>
{% endfor %}
</body>
</html>
复制代码
if 标签
用来判断条件
代码示例:
案例
four.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>案例四</title>
</head>
<body>
{% if name == "ruochen" %}
<h1>
{{name}}, 你好,
</h1>
{% elif name == "ruo" %}
<h1>
{{name}}, 你还记得大明湖畔的下雨天的荷吗?
</h1>
{% else %}
<h1>
{{name}}, 你还有遗憾吗?
</h1>
{% endif %}
</body>
</html>
复制代码
csrf 标签
csrf:跨站请求伪造
在提交表单的时候,表单页面需要加上 {% csrf_token %}
案例
five_get
,five_post
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>案例五</title>
</head>
<body>
<form action="/five_post/" method="post">
{% csrf_token %}
用户名: <input type="text" placeholder="请输入用户名" name="username">
<br>
密码: <input type="password" name="password">
<br>
<input type="submit" value="提交">
</form>
</body>
</html>
复制代码
源码
urls.py
from django.conf.urls import include, url
from django.contrib import admin
from mytpl import views as v
urlpatterns = [
# Examples:
# url(r'^$', 'django_tpl.views.home', name='home'),
# url(r'^blog/', include('blog.urls')),
url(r'^admin/', include(admin.site.urls)),
url(r'^one/', v.one),
url(r'^two/', v.two),
url(r'^three/', v.three),
url(r'^four/', v.four),
url(r'^five_get/', v.five_get),
url(r'^five_post/', v.five_post),
]
复制代码
views.py
from django.shortcuts import render
from django.http import HttpResponse
# Create your views here.
def one(request):
return render(request, r'one.html')
def two(request):
# 用来存放模板中传递的数据
ct = dict()
ct['name'] = 'ruochen'
ct['name2'] = 'ruo'
return render(request, r'two.html', context=ct)
def three(request):
ct = dict()
ct['score'] = [99, 86, 23, 100, 46]
return render(request, r'three.html', context=ct)
def four(request):
ct = dict()
ct['name'] = 'ru'
return render(request, r'four.html', context=ct)
def five_get(request):
return render(request, r'five_get.html')
def five_post(request):
print(request.POST)
return render(request, r'one.html')
复制代码
settings.py
"""
Django settings for django_tpl project.
Generated by 'django-admin startproject' using Django 1.8.
For more information on this file, see
https://docs.djangoproject.com/en/1.8/topics/settings/
For the full list of settings and their values, see
https://docs.djangoproject.com/en/1.8/ref/settings/
"""
# Build paths inside the project like this: os.path.join(BASE_DIR, ...)
import os
BASE_DIR = os.path.dirname(os.path.dirname(os.path.abspath(__file__)))
# Quick-start development settings - unsuitable for production
# See https://docs.djangoproject.com/en/1.8/howto/deployment/checklist/
# SECURITY WARNING: keep the secret key used in production secret!
SECRET_KEY = '_qwgzdbkb&m5tzm%@b*^xh7(!u-)-v&&uk896dxj)wj3^@h_xb'
# SECURITY WARNING: don't run with debug turned on in production!
DEBUG = True
ALLOWED_HOSTS = []
# Application definition
INSTALLED_APPS = (
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'mytpl',
)
MIDDLEWARE_CLASSES = (
'django.contrib.sessions.middleware.SessionMiddleware',
'django.middleware.common.CommonMiddleware',
'django.middleware.csrf.CsrfViewMiddleware',
'django.contrib.auth.middleware.AuthenticationMiddleware',
'django.contrib.auth.middleware.SessionAuthenticationMiddleware',
'django.contrib.messages.middleware.MessageMiddleware',
'django.middleware.clickjacking.XFrameOptionsMiddleware',
'django.middleware.security.SecurityMiddleware',
)
ROOT_URLCONF = 'django_tpl.urls'
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
# 告诉django,在当前项目目录下查询叫templates的文件夹,下面是模板
'DIRS': [os.path.join(BASE_DIR, 'templates')],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
WSGI_APPLICATION = 'django_tpl.wsgi.application'
# Database
# https://docs.djangoproject.com/en/1.8/ref/settings/#databases
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': os.path.join(BASE_DIR, 'db.sqlite3'),
}
}
# Internationalization
# https://docs.djangoproject.com/en/1.8/topics/i18n/
LANGUAGE_CODE = 'en-us'
TIME_ZONE = 'UTC'
USE_I18N = True
USE_L10N = True
USE_TZ = True
# Static files (CSS, JavaScript, Images)
# https://docs.djangoproject.com/en/1.8/howto/static-files/
STATIC_URL = '/static/'
复制代码
划线
评论
复制
发布于: 2021 年 05 月 29 日阅读数: 10
版权声明: 本文为 InfoQ 作者【若尘】的原创文章。
原文链接:【http://xie.infoq.cn/article/59c5695487016949759090960】。文章转载请联系作者。
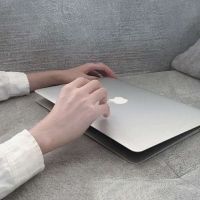
若尘
关注
还未添加个人签名 2021.01.11 加入
还未添加个人简介
评论